import threading
import time
import queue
#第一节
# def run(threadname,n):
# lock.acquire()
# while n>=0:
# print(threadname+":"+str(n))
# time.sleep(1)
# n-=1
# lock.release()
# Thread1=threading.Thread(target=run,args=("Thread_1",5,))
# Thread2=threading.Thread(target=run,args=("Thread_2",5,))
# Thread3=threading.Thread(target=run,args=("Thread_3",5,))
# Thread1.start()
# Thread2.start()
# Thread3.start()
# Thread1.join()
# Thread2.join()
# Thread3.join()
#
#第二节
#多个线程执行一个操作
# lock=threading.Lock()#上锁,上锁防止多个线程操作,造成数据混乱!
# class myThread(threading.Thread):
# def __init__(self):
# threading.Thread.__init__(self)
# name=threading.Thread.getName(self)
# self.name=name
# self.count=5
#
# def run(self):
# lock.acquire()
# while self.count >= 0:
# print(self.name + ":" + str(self.count))
# time.sleep(1)
# self.count -= 1
# lock.release()
# # 创建新线程
# thread1 = myThread()
# thread2 = myThread()
# thread1.start()#启动线程
# thread2.start()
# thread1.join()#阻塞的作用,放在不同线程启动后的位置有不同德效果!比如放在thread1.start()后面,在放thread2.start(),需要等待thread1执行完后才会执行thread2
# thread2.join()
# print("退出主线程")
#第三节
#生产者和消费者的模式,生产完一个数据,等消费者消费完在生产第二个
#创建队列
qu=queue.Queue()
lock=threading.Lock()#上锁,上锁防止多个线程操作,造成数据混乱!上锁后,需要等待解锁才能操作
class mythrade_01(object):
def __init__(self):
self.count=10
#生产者
def producer(self):
while self.count>=0:
lock.acquire()#上锁
print("producer:",self.count)
qu.put(self.count)#往队列里面传参
# time.sleep(1)
self.count-=1
#消费者的消费
def consumer(self):
while self.count>=0:
print("consumer:",qu.get())#往队列里面拿数据
# time.sleep(1)
lock.release()解锁
#生产者线程
def producer_thread(self):
producer_thread=threading.Thread(target=self.producer,args=())
producer_thread.start()
#消费者线程
def consumer_thread(self):
consumer_thread = threading.Thread(target=self.consumer, args=())
consumer_thread.start()#启动线程
# thread=mythrade_01()
# thrade1=thread.producer_thread()
# thrade12=thread.consumer_thread()
python-简单线程
最新推荐文章于 2022-08-31 16:32:03 发布
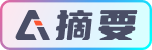