<!DOCTYPE html>
<html>
<head>
<title>GPU加速示例</title>
<style>
#canvas {
width: 400px;
height: 300px;
}
</style>
</head>
<body>
<canvas id="canvas"></canvas>
<script>
// 获取canvas元素
const canvas = document.getElementById('canvas');
const ctx = canvas.getContext('webgl');
// 创建顶点着色器
const vertexShaderSource = `
attribute vec2 a_position;
void main() {
gl_Position = vec4(a_position, 0, 1);
}
`;
const vertexShader = ctx.createShader(ctx.VERTEX_SHADER);
ctx.shaderSource(vertexShader, vertexShaderSource);
ctx.compileShader(vertexShader);
// 创建片元着色器
const fragmentShaderSource = `
precision mediump float;
uniform float u_time;
void main() {
gl_FragColor = vec4(sin(u_time), cos(u_time), tan(u_time), 1.0);
}
`;
const fragmentShader = ctx.createShader(ctx.FRAGMENT_SHADER);
ctx.shaderSource(fragmentShader, fragmentShaderSource);
ctx.compileShader(fragmentShader);
// 创建着色器程序
const program = ctx.createProgram();
ctx.attachShader(program, vertexShader);
ctx.attachShader(program, fragmentShader);
ctx.linkProgram(program);
ctx.useProgram(program);
// 设置顶点数据
const positionAttributeLocation = ctx.getAttribLocation(program, "a_position");
const positionBuffer = ctx.createBuffer();
ctx.bindBuffer(ctx.ARRAY_BUFFER, positionBuffer);
const positions = [
-1, -1,
1, -1,
-1, 1,
1, 1
];
ctx.bufferData(ctx.ARRAY_BUFFER, new Float32Array(positions), ctx.STATIC_DRAW);
ctx.enableVertexAttribArray(positionAttributeLocation);
ctx.vertexAttribPointer(positionAttributeLocation, 2, ctx.FLOAT, false, 0, 0);
// 获取uniform变量位置
const timeUniformLocation = ctx.getUniformLocation(program, "u_time");
// 渲染函数
let startTime = Date.now();
function render() {
// 计算运行时间
const currentTime = (Date.now() - startTime) / 1000;
// 设置uniform变量值并渲染
ctx.uniform1f(timeUniformLocation, currentTime);
ctx.clearColor(0, 0, 0, 0);
ctx.clear(ctx.COLOR_BUFFER_BIT);
ctx.drawArrays(ctx.TRIANGLE_STRIP, 0, 4);
// 请求下一帧渲染
requestAnimationFrame(render);
}
// 开始渲染
render();
</script>
</body>
</html>
GPU-gpu测试网页
最新推荐文章于 2024-06-24 18:02:59 发布
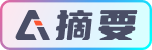