队列:
/**********队列**********/
/*本例子中需要注意的是,指针的访问;head指向的地址,*head可以理解为指向的具体数值*/
/*指针的内容还是需要去多多的理解和使用,指针head=&a,那么head是指向a的地址,*head就是代表了a,也就是代表了a代表的值*/
#include <stdio.h>
#include <stdlib.h>
#define length 3
typedef struct Line{ //队列的数据结构
int buffer[length]; //缓冲队列
int *head,*tail,*end; //head指向队头,tail指向队尾
//head= tail=&buffer[0];
}Queue;
Queue *Queue_Create(void) //队列的创建函数
{
int n,data;
Queue *queue=(Queue *)malloc(sizeof(Queue));
queue->head=queue->tail=queue->buffer; //取队列中缓冲数组的头地址
queue->end=&queue->buffer[length-1]; //取队列中缓冲数组的尾地址
printf("请输入需要创建的队列的大小:\n");
scanf("%d",&n);
printf("请输入%d个数值;\n",n);
while(n)
{
scanf("%d",&data);
if(queue->tail<=queue->end) //判断缓冲数组的尾指针是否已经指向数组的尾地址,如果不是就直接插入数据;否则要改变tail指向buffer的头地址
{
*queue->tail=data;
queue->tail++;
}
else{ //这里的创建的循环队列,在队列满了的情况下会采取“先进先出”,会逐渐覆盖进入队列中最久时间的数据
printf("队列已满\n");
queue->tail=queue->buffer;
*queue->tail=data;
printf("%d\n",*queue->tail);
queue->tail++;
queue->head++;
}
n--;
}
return queue;
}
/*这里的出队列函数,在上面的创建队列函数中,tail是指向队列的下一个该插入数据的地址,但是在存在tail的指向地址一开始就和head重合的情况;
经过分析发现,tail有两种情况,在head的左边和右边,左边时对应的是,插入的数据超过了缓冲队列的大小;在右边时对应的是,插入的数据没有超过
缓冲队列的大小;*/
void Queue_Out(Queue *queue) //出队操作函数
{
int data;
if(queue->tail<=queue->head)
{
printf("%d ",*queue->head);
queue->head++; //为了避免head和tail重合的情况,当tail<=head时,先打印*head,并且head++
//printf("%d\n",*queue->tail);
while(queue->head!=queue->tail) //tail指向队列的下一个该插入数据的地址,此时这个地址是没有数据的
{
if(queue->head<=queue->end)
{
data=*queue->head;
printf("%d ",data);
queue->head++;
}
else{
queue->head=queue->buffer;
//data=*queue->head;
//printf("%d ",data);
//queue->head++;
}
}
}
else{
while(queue->head!=queue->tail)
{
printf("%d ",*queue->head);
queue->head++;
}
}
printf("\n");
}
/*void Queue_Insert(Queue *queue)
{
}*/
int main()
{
Queue *line;
line=Queue_Create();
Queue_Out(line);
return 0;
}
运行结果:
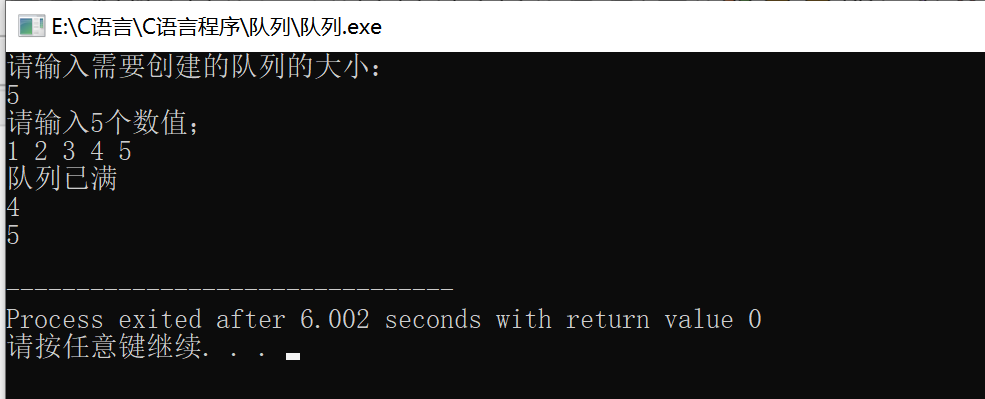