gson框架
implementation 'com.google.code.gson:gson:2.8.6'
效果图
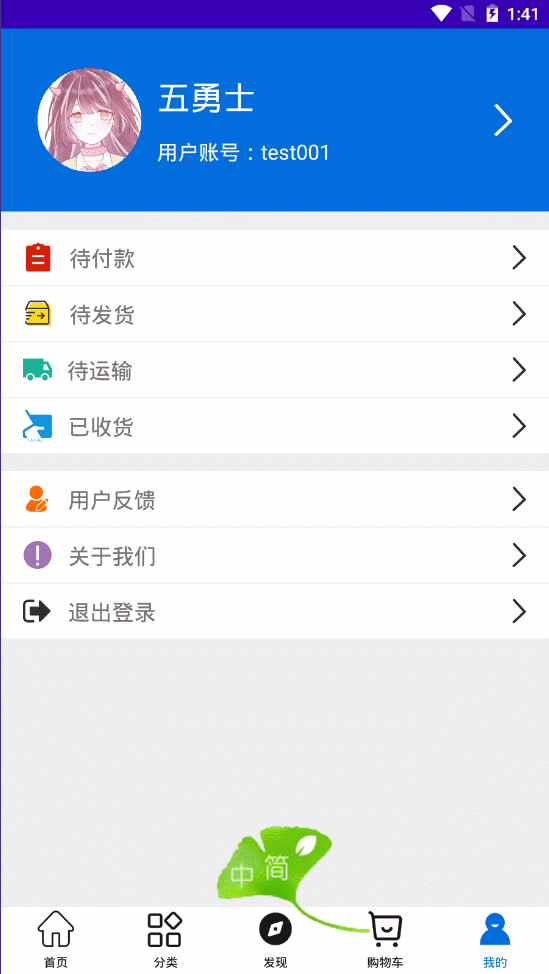
定义按钮
<Button
android:id="@+id/button"
android:layout_width="120dp"
android:layout_height="60dp"
android:text="选择地区"/>
逻辑文件
Button button = findViewById(R.id.button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
AlertDialog.Builder builder=new AlertDialog.Builder(Info.this);
View view = LayoutInflater.from(Info.this).inflate(R.layout.addressdialog, null);
builder.setView(view);
LinearLayout addressdialog_linearlayout = (LinearLayout)view.findViewById(R.id.addressdialog_linearlayout);
final ScrollerNumberPicker provincePicker = (ScrollerNumberPicker)view.findViewById(R.id.province);
final ScrollerNumberPicker cityPicker = (ScrollerNumberPicker)view.findViewById(R.id.city);
final ScrollerNumberPicker counyPicker = (ScrollerNumberPicker)view.findViewById(R.id.couny);
final AlertDialog dialog = builder.show();
addressdialog_linearlayout.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
button.setText(provincePicker.getSelectedText()+cityPicker.getSelectedText()+counyPicker.getSelectedText());
save.setVisibility(View.VISIBLE);
Log.i("kkkk",provincePicker.getSelectedText()+cityPicker.getSelectedText()+counyPicker.getSelectedText());
dialog.dismiss();
}
});
}
});
自定义的dialog样式
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:id="@+id/myaddress_linerlayout"
android:layout_width="match_parent"
android:layout_height="45dp"
android:orientation="horizontal"
android:gravity="center"
android:background="#1290DC">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1"
android:orientation="vertical"
android:gravity="left|center">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="3dp"
android:layout_marginBottom="5dp"
android:layout_marginLeft="15dp"
android:text="选择地区"
android:textColor="#fff"
android:textSize="17dp"/>
</LinearLayout>
<LinearLayout
android:id="@+id/addressdialog_linearlayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1"
android:orientation="vertical"
android:gravity="center|right">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="3dp"
android:layout_marginBottom="5dp"
android:layout_marginRight="15dp"
android:text="确定"
android:textColor="#fff"
android:textSize="17dp"/>
</LinearLayout>
</LinearLayout>
<LinearLayout
android:layout_below="@+id/myaddress_linerlayout"
android:layout_width="match_parent"
android:layout_height="180dp"
android:orientation="horizontal"
android:gravity="center"
android:background="#ffff">
<com.czhoujunlv.drug.city.CityPicker
android:id="@+id/citypicker"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:gravity="center"
android:orientation="vertical"/>
</LinearLayout>
</RelativeLayout>
自定义view类CityPicker
public class CityPicker extends LinearLayout {
private ScrollerNumberPicker provincePicker;
private ScrollerNumberPicker cityPicker;
private ScrollerNumberPicker counyPicker;
private OnSelectingListener onSelectingListener;
private static final int REFRESH_VIEW = 0x001;
private int tempProvinceIndex = -1;
private int temCityIndex = -1;
private int tempCounyIndex = -1;
private Context context;
private List<Cityinfo> province_list = new ArrayList<Cityinfo>();
private HashMap<String, List<Cityinfo>> city_map = new HashMap<String, List<Cityinfo>>();
private HashMap<String, List<Cityinfo>> couny_map = new HashMap<String, List<Cityinfo>>();
private CitycodeUtil citycodeUtil;
private String city_code_string;
private String city_string;
public CityPicker(Context context, AttributeSet attrs) {
super(context, attrs);
this.context = context;
getaddressinfo();
}
public CityPicker(Context context) {
super(context);
this.context = context;
getaddressinfo();
}
private void getaddressinfo() {
JSONParser parser = new JSONParser();
String area_str = FileUtil.readAssets(context, "area.json");
province_list = parser.getJSONParserResult(area_str, "area0");
city_map = parser.getJSONParserResultArray(area_str, "area1");
couny_map = parser.getJSONParserResultArray(area_str, "area2");
}
public static class JSONParser {
public ArrayList<String> province_list_code = new ArrayList<String>();
public ArrayList<String> city_list_code = new ArrayList<String>();
public List<Cityinfo> getJSONParserResult(String JSONString, String key) {
List<Cityinfo> list = new ArrayList<Cityinfo>();
JsonObject result = new JsonParser().parse(JSONString)
.getAsJsonObject().getAsJsonObject(key);
Iterator<?> iterator = result.entrySet().iterator();
while (iterator.hasNext()) {
@SuppressWarnings("unchecked")
Entry<String, JsonElement> entry = (Entry<String, JsonElement>) iterator
.next();
Cityinfo cityinfo = new Cityinfo();
cityinfo.setCity_name(entry.getValue().getAsString());
cityinfo.setId(entry