一个简单的WebRtc的例子
创建项目
// 创建项目
// 1. 进入发布目录 ,这里要进入自己设置的发布路径
cd public
// 创建项目
mkdir demo01
cd demo01
// 创建 两文件 css 和 js ,用来对html 界面进行渲染
mkdir css js
// 返回上一级目录,书写html文件
cd ..
vim index.html
// index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>一个简单WebRTC例子</title>
<link href="./css/main.css" rel="stylesheet" />
</head>
<body>
<div>
<div>
<button type="button" id="start">Start</button>
<button type="button" id="call">Call</button>
<button id="hangup" disabled>HangUp</button>
</div>
<div id="preview">
<div id="preview">
<div >
<h2>Local:</h2>
<video id="localvideo" autoplay playsinline></video>
<h2>Local SDP:</h2>
<textarea id="offer"></textarea>
</div>
<div>
<h2>Remote:</h2>
<video id="remotevideo" autoplay playsinline></video>
<h2>Remote SDP:</h2>
<textarea id="answer"></textarea>
</div>
</div>
</div>
</div>
<script src="https://webrtc.github.io/adapter/adapter-latest.js"></script>
<script src="js/main.js"></script>
</body>
</html>
// main.css
button {
margin: 0 20px 25px 0;
vertical-align: top;
width: 134px;
}
textarea {
color: #444;
font-size: 0.9em;
font-weight: 300;
height: 20.0em;
padding: 5px;
width: calc(100% - 10px);
}
div#getUserMedia {
padding: 0 0 8px 0;
}
div.input {
display: inline-block;
margin: 0 4px 0 0;
vertical-align: top;
width: 310px;
}
div.input > div {
margin: 0 0 20px 0;
vertical-align: top;
}
div.output {
background-color: #eee;
display: inline-block;
font-family: 'Inconsolata', 'Courier New', monospace;
font-size: 0.9em;
padding: 10px 10px 10px 25px;
position: relative;
top: 10px;
white-space: pre;
width: 270px;
}
div#preview {
border-bottom: 1px solid #eee;
margin: 0 0 1em 0;
padding: 0 0 0.5em 0;
}
div#preview > div {
display: inline-block;
vertical-align: top;
width: calc(50% - 12px);
}
section#statistics div {
display: inline-block;
font-family: 'Inconsolata', 'Courier New', monospace;
vertical-align: top;
width: 308px;
}
section#statistics div#senderStats {
margin: 0 20px 0 0;
}
section#constraints > div {
margin: 0 0 20px 0;
}
h2 {
margin: 0 0 1em 0;
}
section#constraints label {
display: inline-block;
width: 156px;
}
section {
margin: 0 0 20px 0;
padding: 0 0 15px 0;
}
video {
background: #222;
margin: 0 0 0 0;
--width: 100%;
width: var(--width);
height: 225px;
}
@media screen and (max-width: 720px) {
button {
font-weight: 500;
height: 56px;
line-height: 1.3em;
width: 90px;
}
div#getUserMedia {
padding: 0 0 40px 0;
}
section#statistics div {
width: calc(50% - 14px);
}
}
'use strict'
var localVideo = document.querySelector('video#localvideo');
var remoteVideo = document.querySelector('video#remotevideo');
var btnStart = document.querySelector('button#start');
var btnCall = document.querySelector('button#call');
var btnHangup = document.querySelector('button#hangup');
var offerSdpTextarea = document.querySelector('textarea#offer');
var answerSdpTextarea = document.querySelector('textarea#answer');
var localStream;
var pc1;
var pc2;
function getMediaStream(stream){
localVideo.srcObject = stream;
localStream = stream;
}
function handleError(err){
console.error('Failed to get Media Stream!', err);
}
function start(){
if(!navigator.mediaDevices ||
!navigator.mediaDevices.getUserMedia){
console.error('the getUserMedia is not supported!');
return;
}else {
var constraints = {
video: true,
audio: false
}
navigator.mediaDevices.getUserMedia(constraints)
.then(getMediaStream)
.catch(handleError);
btnStart.disabled = true;
btnCall.disabled = false;
btnHangup.disabled = true;
}
}
function getRemoteStream(e){
remoteVideo.srcObject = e.streams[0];
}
function handleOfferError(err){
console.error('Failed to create offer:', err);
}
function handleAnswerError(err){
console.error('Failed to create answer:', err);
}
function getAnswer(desc){
pc2.setLocalDescription(desc);
answerSdpTextarea.value = desc.sdp
pc1.setRemoteDescription(desc);
}
function getOffer(desc){
pc1.setLocalDescription(desc);
offerSdpTextarea.value = desc.sdp
pc2.setRemoteDescription(desc);
pc2.createAnswer()
.then(getAnswer)
.catch(handleAnswerError);
}
function call(){
pc1 = new RTCPeerConnection();
pc2 = new RTCPeerConnection();
pc1.onicecandidate = (e)=>{
pc2.addIceCandidate(e.candidate);
}
pc2.onicecandidate = (e)=>{
pc1.addIceCandidate(e.candidate);
}
pc2.ontrack = getRemoteStream;
localStream.getTracks().forEach((track)=>{
pc1.addTrack(track, localStream);
});
var offerOptions = {
offerToRecieveAudio: 0,
offerToRecieveVideo: 1
}
pc1.createOffer(offerOptions)
.then(getOffer)
.catch(handleOfferError);
btnCall.disabled = true;
btnHangup.disabled = false;
}
function hangup(){
pc1.close();
pc2.close();
pc1 = null;
pc2 = null;
btnCall.disabled = false;
btnHangup.disabled = true;
}
btnStart.onclick = start;
btnCall.onclick = call;
btnHangup.onclick = hangup;
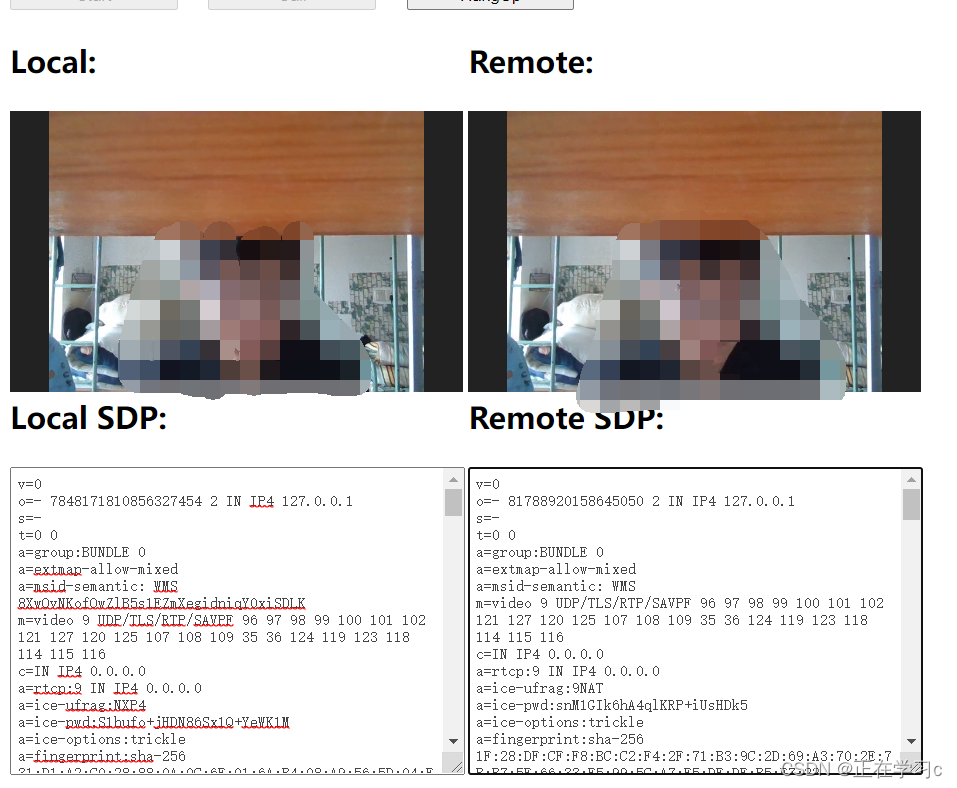