java反射示例演示代码
反射是什么?
介绍: Java的反射(reflection)机制是指在程序的运行状态中,可以构造任意一个类的对象,可以了解任意一个对象所属的类,可以了解任意一个类的成员变量和方法,可以调用任意一个对象的属性和方法。这种动态获取程序信息以及动态调用对象的功能称为Java语言的反射机制。反射被视为动态语言的关键。
这种机制在很多的如Spring的框架中得到应用,所以还是需要了解一下的。写了一个段代码简单介绍一下这种机制,reflect()方法是通过反射拿到一个类的基本信息,如方法名称,参数,构造器等, 然后拼接字符串打印出来。method()是通过反射实例化对象,然后给对象赋值。
代码示例
1.用于反射的类
public class Customer {
private int custid;
private String custname;
private String contacts;
private String tel;
private String email;
private int empid;
public Customer() {
System.out.println("Customer无参构造器");
}
public Customer(int custid, String custname, String contacts, String tel, String email) {
this.custid = custid;
this.custname = custname;
this.contacts = contacts;
this.tel = tel;
this.email = email;
}
public Customer(String custname, String contacts, String tel, String email, int empid) {
this.custname = custname;
this.contacts = contacts;
this.tel = tel;
this.email = email;
this.empid = empid;
}
public Customer(int custid, String custname, String contacts, String tel, String email, int empid) {
this.custid = custid;
this.custname = custname;
this.contacts = contacts;
this.tel = tel;
this.email = email;
this.empid = empid;
}
@Override
public String toString() {
return "Customer{" +
"custid=" + custid +
", custname='" + custname + '\'' +
", contacts='" + contacts + '\'' +
", tel='" + tel + '\'' +
", email='" + email + '\'' +
", empid=" + empid +
'}';
}
public int getCustid() {
return custid;
}
public void setCustid(int custid) {
this.custid = custid;
}
public String getCustname() {
return custname;
}
public void setCustname(String custname) {
this.custname = custname;
}
public String getContacts() {
return contacts;
}
public void setContacts(String contacts) {
this.contacts = contacts;
}
public String getTel() {
return tel;
}
public void setTel(String tel) {
this.tel = tel;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public int getEmpid() {
return empid;
}
public void setEmpid(int empid) {
this.empid = empid;
}
}
2.reflect()方法
public static void reflect() {
String className = "pro.reflect.Customer";
try {
//得到反射对象
Class cls = Class.forName(className);
//根据反射对象获得修饰符
String modify = Modifier.toString(cls.getModifiers());
//得到反射类名称
String name = cls.getName();
System.out.println(modify + " class " + name + "{");
System.out.println("****************得到类属性*****************");
//得到类的所有的属性字段custid,custname,contacts...
Field[] declaredFields = cls.getDeclaredFields();
//循环遍历输出
for (Field field : declaredFields) {
//根据字段属性获得修饰符private,public...
modify = Modifier.toString(field.getModifiers());
//得到字段类型名称string,int...
String type = field.getType().getName();
//得到属性字段名称custid,custname,contacts
name = field.getName();
System.out.println(modify + " " + type + " " + name + ";");
}
System.out.println("****************得到所有的构造器*****************");
//得到所有的构造器
Constructor[] declaredConstructors = cls.getDeclaredConstructors();
//循环输出
for (Constructor constructor : declaredConstructors) {
//根据构造器获得修饰符private,public...
modify = Modifier.toString(constructor.getModifiers());
//得到构造器字段名称Customer
name = constructor.getName();
//根据构造器得到所以参数的类型
Class[] parameterTypes = constructor.getParameterTypes();
System.out.print(modify + " " + name + "(");
for (Class parameterType : parameterTypes) {
System.out.print(parameterType.getName() + ",");
}
System.out.println("){}");
}
System.out.println("****************得到所有的声明的方法*****************");
//得到所有的声明的方法
Method[] declaredMethods = cls.getDeclaredMethods();
//循环输出
for (Method method : declaredMethods) {
//根据构造器获得修饰符private,public...
modify = Modifier.toString(method.getModifiers());
//得到返回类型
String type = method.getReturnType().getName();
//得到方法名称
name = method.getName();
//得到参数类型
Class[] parameterTypes = method.getParameterTypes();
//格式输出
System.out.print(modify + " " + type + " " + name + "(");
for (Class parameterType : parameterTypes) {
System.out.print(parameterType.getName() + ",");
}
System.out.println("){}");
}
System.out.println("}");
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
输出
public class pro.reflect.Customer{
****************得到类属性*****************
private int custid;
private java.lang.String custname;
private java.lang.String contacts;
private java.lang.String tel;
private java.lang.String email;
private int empid;
****************得到所有的构造器*****************
public pro.reflect.Customer(int,java.lang.String,java.lang.String,java.lang.String,java.lang.String,int,){}
public pro.reflect.Customer(java.lang.String,java.lang.String,java.lang.String,java.lang.String,int,){}
public pro.reflect.Customer(int,java.lang.String,java.lang.String,java.lang.String,java.lang.String,){}
public pro.reflect.Customer(){}
****************得到所有的声明的方法*****************
public java.lang.String toString(){}
public void setCustid(int,){}
public java.lang.String getContacts(){}
public void setContacts(java.lang.String,){}
public java.lang.String getTel(){}
public void setCustname(java.lang.String,){}
public java.lang.String getCustname(){}
public java.lang.String getEmail(){}
public int getEmpid(){}
public void setEmail(java.lang.String,){}
public void setEmpid(int,){}
public int getCustid(){}
public void setTel(java.lang.String,){}
}
3.method()方法
public static void method() {
String className = "pro.reflect.Customer";
String fieldName = "custname";
String fieldValue = "张三";
String methodName = "setTel";
String argsTypes = "java.lang.String";
String argsValues = "13833546346";
try {
//得到反射对象
Class cls = Class.forName(className);
//Object obj = cls.newInstance();
//得到构造器
Constructor declaredConstructor = cls.getDeclaredConstructor();
//根据构造器得到对象实例
Object obj = declaredConstructor.newInstance();
//根据字段名称custname得到字段
Field field = cls.getDeclaredField(fieldName);
//设置字段可见,注意现在的customer是由private修饰的
field.setAccessible(true);
//字段赋值
field.set(obj, fieldValue);
//得到参数字段类型argsTypes-》java.lang.String
Class argsTypeClass = Class.forName(argsTypes);
//声明的方法
Method declaredMethod = null;
//得到方法通过方法名和参数类型methodName——》setTel,并传入参数argsTypeClass
declaredMethod = cls.getDeclaredMethod(methodName, argsTypeClass);
//为对象实例赋值;argsValues->13833546346
declaredMethod.invoke(obj, argsValues);
//打印实例
System.out.println(obj);
} catch (Exception e) {
e.printStackTrace();
}
}
输出
Customer无参构造器
Customer{custid=0, custname='张三', contacts='null', tel='13833546346', email='null', empid=0}
希望对你有帮助,打工人冲冲冲!
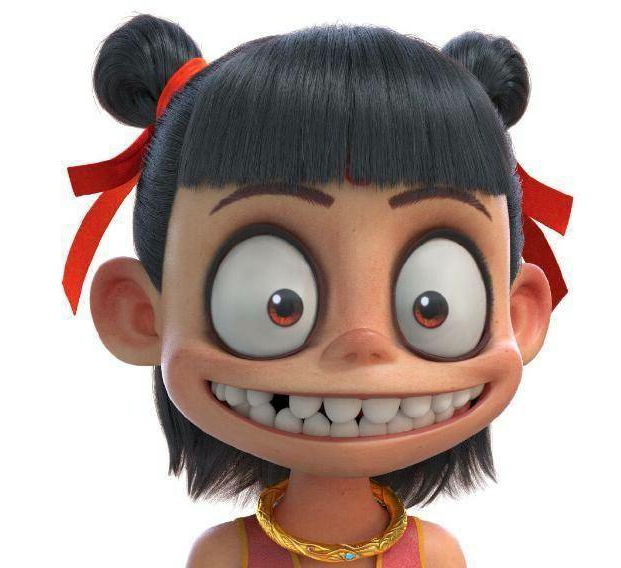