#include<iostream>
using namespace std;
template<typename T>
class vector{
private:
int __capacity,__size;
T* __a;
public:
vector():__capacity(0),__size(0),__a(nullptr){
//cout<<"默认构造\n";
}
vector(const vector& temp):__capacity(temp.__capacity),__size(temp.__size){
__a=new T[__capacity];
for(int i=0;i<__size;i++){
__a[i]=temp.__a[i];
}
//cout<<"拷贝构造\n";
}
vector(vector&& temp):__capacity(temp.__capacity),__size(temp.__size),__a(temp.__a){
temp.__a=nullptr;
// cout<<"移动构造\n";
}
vector& operator=(const vector& temp){
if(this==&temp) return *this;
__capacity=temp.__capacity;
__size=temp.__size;
delete[] __a;
__a=new T[__capacity];
for(int i=0;i<__size;i++){
__a[i]=temp.__a[i];
}
//cout<<"拷贝赋值\n";
return *this;
}
vector& operator=(vector&& temp){
if(this==&temp) return *this;
__capacity=temp.__capacity;
__size=temp.__size;
delete[] __a;
__a=temp.__a;
temp.__a=nullptr;
//cout<<"移动赋值\n";
return *this;
}
void resize(int x){
if(!x) x=1;
__capacity=x;
T* nxt=new T[__capacity];
for(int i=0;i<__size;i++){
nxt[i]=__a[i];
}
delete[] __a;
__a=nxt;
}
void push_back(T x){
if(__size==__capacity){
resize(__size*2);
}
__a[__size++]=x;
}
void pop_back(){
--__size;
}
T& operator[](int idx){
return __a[idx];
}
int size(){
return __size;
}
int capacity(){
return __capacity;
}
~vector(){
delete[] __a;
}
};
int main(){
vector<int> a;//默认构造
vector<int> b(a);//拷贝构造
vector<int> c;//默认构造
c=a;//拷贝赋值
cout<<endl<<endl;
vector<int> d(move(c));//移动构造
vector<int> e;//默认构造
e=move(c);//移动赋值
return 0;
}
C++ 手写vector
最新推荐文章于 2024-09-10 16:26:50 发布
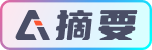