设计一个算法用于判断带头结点的循环双链表是否对称
#include<stdio.h>
#include<stdlib.h>
typedef struct DNode {
int data;
struct DNode *prior,*next;
} DNode, *DLinkList;
void Symmetry(DLinkList L) {
DNode *p=L->next,*q=L->prior;
while(p!=q&&q->next!=p) {
if(p->data==q->data) {
p=p->next;
q=q->prior;
} else{
printf("\n该表不对称\n");
return;
}
}
printf("\n该表对称\n");
}
DLinkList CreateList_DL() {
DNode *head, *p, *s;
int x;
head = (DNode *)malloc(sizeof(DNode));
p=head;
printf("请输入元素(输入9999结束):\n");
scanf("%d",&x);
while(x!=9999) {
s=(DNode *)malloc(sizeof(DNode));
s->data=x;
p->next=s;
s->prior=p;
p=s;
scanf("%d",&x);
}
head->prior=s;
p->next=head;
return head;
}
void PrintList(DLinkList head) {
DNode *p;
p=head->next;
while(p!=head) {
printf("%d ",p->data);
p=p->next;
}
}
int main() {
DLinkList L=CreateList_DL();
PrintList(L);
Symmetry(L);
}
运行结果
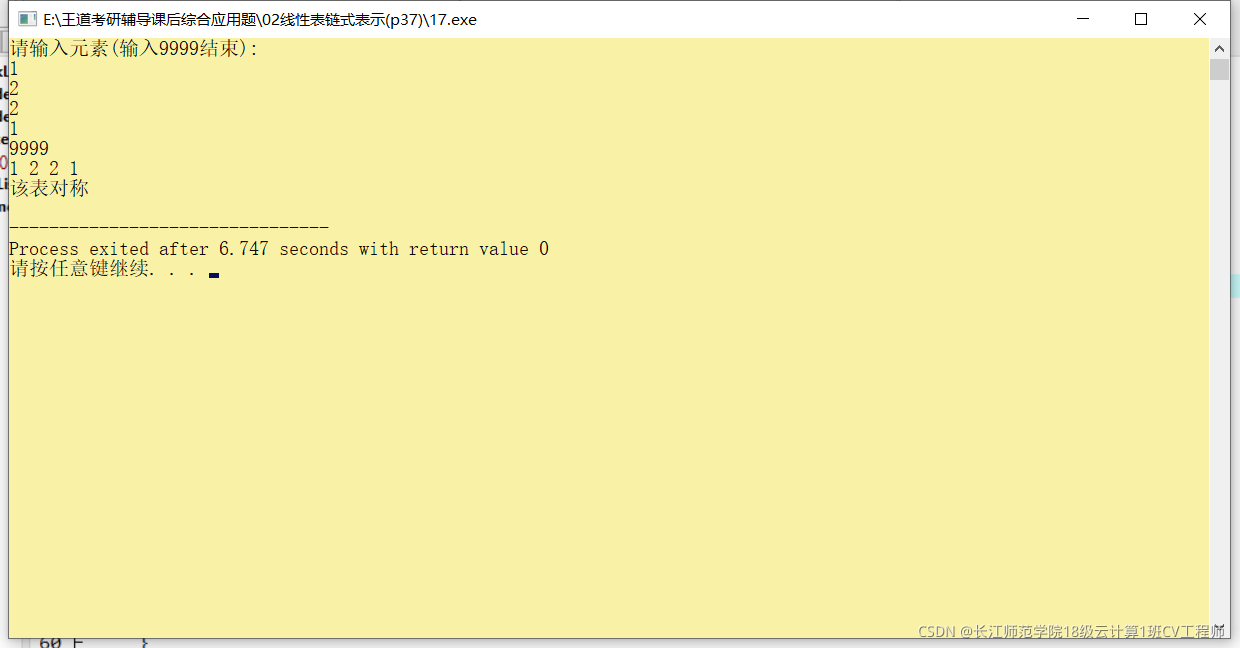