多边形
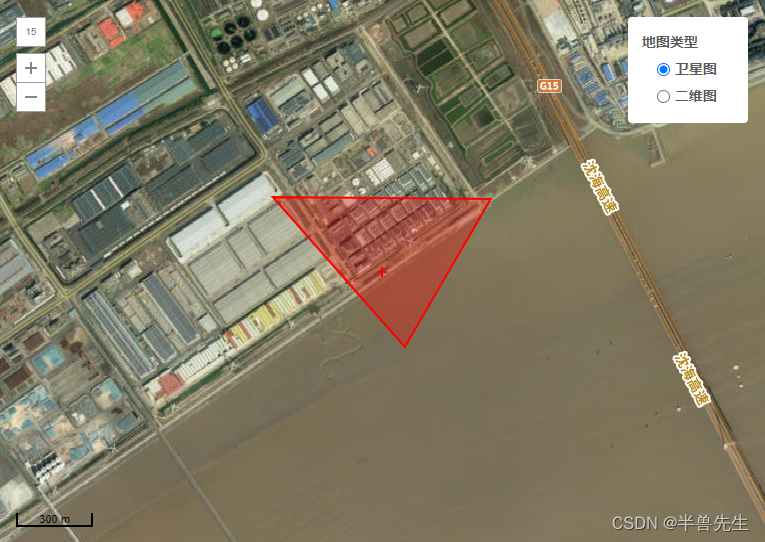
<!-- 地图组件 -->
<template>
<div :id="id" class="container"></div>
</template>
<script>
import _ from "lodash";
import "maptalks/dist/maptalks.css";
import * as maptalks from "maptalks";
export default {
//import引入的组件需要注入到对象中才能使用
name: "Map",
props: {
id: {
type: String,
default: () => {
return "map";
},
},
mapSet: {
type: Object,
default: () => {
return {};
},
},
color: {
type: String,
default: () => {
return "";
},
},
},
components: {},
data() {
//这里存放数据
return {
dataLis: [],
layer: null,
layer2: null,
map: null,
polygon: null,
drawTool: null,
// 默认中心点点位
center: [121.044372, 30.575405],
// 缩放层级
zoom: 14,
// 倾斜度
pitch: 0,
// 轴承
// bearing: 90,
// 最小缩放层级
minZoom: 1,
// 最大缩放层级
maxZoom: 18.5,
symbol: {
polygonFill: "#ff0000", // 图形覆盖颜色
polygonOpacity: 0.3, // 图形覆盖区域透明度
lineColor: "#ff0000", // 线条颜色
lineWidth: 2, // 线条宽度
},
pointSymbol: {
markerFile: "@/assets/logo/logo.png",
markerWidth: 20,
markerHeight: 20,
},
layerSwitcherControl: {
position: "top-right",
// title of base layers
baseTitle: "地图类型",
// title of layers
overlayTitle: "图层",
// layers you don't want to manage with layer switcher
excludeLayers: [],
// css class of container element, maptalks-layer-switcher by default
containerClass: "maptalks-layer-switcher",
},
// 电子地图图层
// urlTemplate: "http://{s}.basemaps.cartocdn.com/light_all/{z}/{x}/{y}.png",
// subdomains: ["a", "b", "c", "d"],
// attribution:
// '© <a href="http://osm.org">OpenStreetMap</a> contributors, © <a href="https://carto.com/">CARTO</a>'
// urlTemplate:
// "https://gss{s}.bdstatic.com/8bo_dTSlRsgBo1vgoIiO_jowehsv/tile/?qt=tile&x={x}&y={y}&z={z}&styles=pl&scaler=1&udt=20170927",
// subdomains: [0, 1, 2, 3],
// attribution:
// '© <a target="_blank" href="http://map.baidu.com">Baidu</a>'
drawMap: {
Point: "点",
LineString: "线",
Polygon: "多边形",
Circle: "圆",
Ellipse: "椭圆",
Rectangle: "长方形",
},
};
},
//监听属性 类似于data概念
computed: {},
//监控data中的数据变化
watch: {
mapSet: {
deep: true,
immediate: true,
async handler(val) {
if (Object.keys(val).length) {
let { drawMapType, drawMapArray, editable, draggable } = val;
if (this.map != undefined) this.map.remove();
await this.init();
// 循环插入多边形
if (drawMapArray && drawMapArray.length > 0) {
// console.log("回显", editable);
drawMapArray.forEach(item => {
let obj = {
// 编辑图形
drawMapArray: () => {
this.startEdit(item, {
drawMapType,
editable,
draggable,
drawMapArray: item
});
},
// 绘制图形
drawMapType: () => {
this.drawMapType({
drawMapType,
editable,
draggable,
drawMapArray: item
});
},
};
let arrayKeys = Object.keys({
drawMapType,
editable,
draggable,
drawMapArray: item
});
arrayKeys.map((item) => {
if (obj[item]) return obj[item]();
});
})
if (editable) {
// 打开新增多边形
let obj = {
// 绘制图形
drawMapType: () => {
this.drawMapType({
drawMapType,
editable,
draggable,
// drawMapArray
});
},
};
let arrayKeys = Object.keys({
drawMapType,
editable,
draggable,
// drawMapArray
});
arrayKeys.map((item) => {
if (obj[item]) return obj[item]();
});
}
} else {
// console.log("新增");
let obj = {
// 绘制图形
drawMapType: () => {
this.drawMapType({
drawMapType,
editable,
draggable,
// drawMapArray
});
},
};
let arrayKeys = Object.keys({
drawMapType,
editable,
draggable,
// drawMapArray
});
arrayKeys.map((item) => {
if (obj[item]) return obj[item]();
});
}
} else {
// console.log("销毁地图");
if (this.map != undefined) this.map.remove();
}
},
},
color: {
immediate: true,
handler(val) {
// console.log("🚀 ~ Date:2023/06/15 17:14 ~ File: index.vue ~ Line:118 ----- ", val);
if (val) {
let { drawTool, polygon } = this;
if (drawTool) this.changeSymbol("drawTool", drawTool, val);
if (polygon) this.changeSymbol("polygon", polygon, val);
// if (drawTool) {
// drawTool.setSymbol({
// polygonFill: val, // 图形覆盖颜色
// polygonOpacity: 0.3, // 图形覆盖区域透明度
// lineColor: val, // 线条颜色
// lineWidth: 2 // 线条宽度
// });
// }
// if (polygon) {
// polygon.updateSymbol({
// polygonFill: val, // 图形覆盖颜色
// lineColor: val // 线条颜色
// });
// }
}
},
},
},
//生命周期 - 创建完成(可以访问当前this实例)
// created() { },
//生命周期 - 挂载完成(可以访问DOM元素)
// mounted() {
// let a = "rgb(255, 69, 0)";
// console.log(a.slice(5, a.length - 1));
// },
// beforeDestroy() {
// let { map } = this;
// // console.log(
// // "🚀 ~ Date:2023/07/11 14:37 ~ File: index.vue ~ Line:154 ----- ",
// // 销毁地图
// // );
// if (map) map.remove();
// },
//方法集合
methods: {
// 获取到图层所有的点位
getSent(data) {
let array = []
data.forEach(res => {
let arrray2 = []
res._coordinates.forEach(res2 => {
arrray2.push({
"lngWgs": res2.x,
"latWgs": res2.y,
// "sort": index2
})
})
array.push(arrray2)
})
return array;
},
init() {
this.$nextTick(() => {
let { center, zoom, pitch, minZoom, maxZoom, symbol, layerSwitcherControl, id } = this;
this.map = new maptalks.Map(id, {
center,
zoom,
pitch,
minZoom,
maxZoom,
centerCross: true,
seamlessZoom: true,
attribution: false,
zoomControl: true, // add zoom control
scaleControl: true, // add scale control
layerSwitcherControl,
symbol,
// baseLayer 表示基础图层,它可以添加多个,逗号隔开
baseLayer: new maptalks.GroupTileLayer("Base TileLayer", [
new maptalks.TileLayer("卫星图", {
urlTemplate:
"https://t5.tianditu.gov.cn/DataServer?T=img_w&X={x}&Y={y}&L={z}&tk=074d4a21c8f34a3a20cd1f69f81b26bf",
subdomains: ["a", "b", "c", "d"],
}),
new maptalks.TileLayer("二维图", {
visible: false,
// urlTemplate:
// "https://{s}.basemaps.cartocdn.com/light_all/{z}/{x}/{y}.png",
urlTemplate:
"https://map.geoq.cn/arcgis/rest/services/ChinaOnlineCommunity/MapServer/tile/{z}/{y}/{x}",
subdomains: ["a", "b", "c", "d"],
}),
// new maptalks.TileLayer("Carto dark", {
// visible: false,
// urlTemplate:
// "https://{s}.basemaps.cartocdn.com/dark_all/{z}/{x}/{y}.png",
// subdomains: ["a", "b", "c", "d"],
// }),
// new maptalks.WMSTileLayer("survey", {
// urlTemplate: "http://10.169.61.30:8080/geoserver/gwc/service/wms",
// crs: "EPSG:3857",
// layers: "haigang",
// styles: "",
// version: "1.1.1",
// tileStackDepth: 0,
// format: "image/png",
// transparent: true,
// uppercase: true,
// zIndex: 1,
// forceRenderOnMoving: true,
// forceRenderOnZooming: true,
// }),
]),
});
// 添加-地图矢量数据图层
var tiandituLayer = new maptalks.TileLayer('tianditu', {
urlTemplate: 'http://t0.tianditu.gov.cn/cia_w/wmts?SERVICE=WMTS&REQUEST=GetTile&VERSION=1.0.0&LAYER=cia&STYLE=default&TILEMATRIXSET=w&FORMAT=tiles&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}&tk=074d4a21c8f34a3a20cd1f69f81b26bf',
subdomains: ['a', 'b', 'c']
});
this.map.addLayer(tiandituLayer);
// 监听图层切换监听
this.map.getBaseLayer().on('layerload', function (e) {
let visible = e.target.layers[0].options.visible
if (visible) {
// console.log("卫星图", this.map._layers[0].options.visible);
this.map._layers[0].options.visible = true // 显示-矢量数据图层
} else {
// console.log("二维图", this.map._layers);
this.map._layers[0].options.visible = false // 隐藏-矢量数据图层
}
});
// this.layer = new maptalks.VectorLayer("vector").addTo(this.map)
this.layer = new maptalks.VectorLayer("vector").addTo(this.map)
this.layer2 = new maptalks.VectorLayer("vector2").addTo(this.map)
// this.map.on("click", function (params) {
// console.log(666, params);
// });
this.map.on("editrecord", function (params) {
console.log(params);
});
});
},
// 绘制图形
drawMapType(val) {
!this.layer ? this.layer = new maptalks.VectorLayer("vector").addTo(this.map) : null
// console.log('绘制图形', val, this.map);
let { drawMapType, drawMapArray } = val;
if (!drawMapArray) {
let { map, symbol, color } = this;
let that = this;
// var layer = new maptalks.VectorLayer("vector").addTo(map);
var drawTool = new maptalks.DrawTool({
mode: drawMapType,
// once: true,
// once: false,
symbol: drawMapType == "Point" ? null : symbol,
})
.addTo(map)
.disable();
drawTool.on("drawend", function (param) {
// console.log(param.geometry);
let { _coordinates } = param.geometry;
let array = null;
if (drawMapType == "Point") {
array = [_coordinates.x, _coordinates.y];
} else if (drawMapType == "Polygon") {
array = _coordinates.map((item) => {
return [item.x, item.y];
});
}
that.dataLis.push(array)
// console.log(123, array);
that.layer.addGeometry(param.geometry);
that.startEdit(array, val, that.dataLis.length - 1);
that.layer.remove();
that.layer.setZIndex(6);
that.$nextTick(() => {
// console.log("绘制好了 发送", that.getSent(that.layer2._geoList));
that.$emit("drawPointChange", that.getSent(that.layer2._geoList));
})
});
this.drawTool = drawTool;
drawTool.setMode(drawMapType).enable();
// 改变覆盖物设置
if (color) this.changeSymbol("drawTool", drawTool, color);
// if (color) {
// drawTool.setSymbol({
// polygonFill: color, // 图形覆盖颜色
// polygonOpacity: 0.3, // 图形覆盖区域透明度
// lineColor: color, // 线条颜色
// lineWidth: 2 // 线条宽度
// });
// }
}
},
// 编辑绘制图形
startEdit(array, val) {
// let nun = Math.random();
// console.log("编辑绘制图形", array, val);
let that = this;
let { drawMapType, editable, draggable } = val;
let { symbol, map, pointSymbol, color } = that;
let type = null;
let pointArray = _.clone(array);
// that.$emit("drawPointChange", pointArray);
let objArray = [
[() => drawMapType == "Polygon", () => {
type = "Polygon"
},
() => {
polygon.startEdit();
// 更改形状
polygon.on("shapechange", function (param) {
let { _coordinates } = param.target;
pointArray = _coordinates.map((item) => {
return [item.x, item.y];
});
// 更新原始多边形对象的位置
param.target.getCoordinates()
// polygon.setCoordinates(_coordinates);
that.$emit("drawPointChange", that.getSent(that.layer2._geoList));
// console.log(111, that.getSent(that.layer2._geoList));
});
// 更改位置
polygon.on("positionchange", function (param) {
let { _coordinates } = param.target;
pointArray = _coordinates.map((item) => {
return [item.x, item.y];
});
// 更新原始多边形对象的位置
param.target.getCoordinates()
// polygon.setCoordinates(_coordinates);
that.$emit("drawPointChange", that.getSent(that.layer2._geoList));
// console.log(222);
// console.log("send", that.getSent(that.layer2._geoList));
});
// 监听删除
polygon.on("remove", function (param) {
let { _coordinates } = param.target;
pointArray = _coordinates.map((item) => {
return [item.x, item.y];
});
// 更新原始多边形对象的位置
param.target.getCoordinates()
// polygon.setCoordinates(_coordinates);
that.$emit("drawPointChange", that.getSent(that.layer2._geoList));
// console.log(333);
});
// 监听右键点击事件
// polygon.on("contextmenu", function (e) {
// console.log(112233, e);
// // 判断点击的是否为多边形
// if (e.target instanceof maptalks.Polygon) {
// // 删除多边形
// map.removeGeometry(e.target);
// }
// });
},
],
];
let state = objArray.find((m) => m[0]());
if (state) state[1]();
let polygon = new maptalks[type](array, {
visible: true,
editable,
cursor: "pointer",
draggable,
dragShadow: false,
drawOnAxis: null,
symbol,
});
let options = {
'items': [
{
item: '删除', click: function (e) {
that.layer2.removeGeometry(polygon);
}
}
]
};
polygon.setMenu(options).openMenu();
// new maptalks.VectorLayer("vectorEdit" + nun, polygon).addTo(map);
that.layer2.addGeometry(polygon);
// that.layer.addGeometry(polygon);
map.fitExtent(polygon.getExtent(), -1); // 自动适配区域
if (color) this.changeSymbol("polygon", polygon, color);
if (state) state[2]();
that.polygon = polygon;
},
// 覆盖物设置更改
changeSymbol(type, mapObj, color) {
let obj = {
drawTool: () => {
mapObj.setSymbol({
polygonFill: color, // 图形覆盖颜色
polygonOpacity: 0.3, // 图形覆盖区域透明度
lineColor: color, // 线条颜色
lineWidth: 2, // 线条宽度
});
},
polygon: () => {
mapObj.updateSymbol({
polygonFill: color, // 图形覆盖颜色
lineColor: color, // 线条颜色
});
},
};
if (obj[type]) return obj[type]();
},
// 绘制多边形
// addPoint(val) {
// // console.log(this.map,66);
// let layer = new maptalks.VectorLayer("vector").addTo(this.map);
// // console.log(layer,"tu");
// val.forEach((item) => {
// let polygon = new maptalks.Polygon(item, {
// visible: true,
// editable: false,
// cursor: "pointer",
// draggable: false,
// dragShadow: false,
// drawOnAxis: null,
// // properties: {
// // name,
// // },
// symbol: [
// {
// lineColor: "skyblue",
// lineWidth: 1,
// polygonFill: "skyblue",
// polygonOpacity: 0.1,
// },
// ],
// }).addTo(layer);
// // polygon.setZIndex(0)
// layer.setZIndex(1);
// });
// },
// 绘制2dmark
// addMark(val) {
// console.log(val, 6677);
// this.layer = new maptalks.VectorLayer("vector2").addTo(this.map);
// val.forEach((item) => {
// let sort = item.sort;
// let file = require("@/assets/warehouseWeb/equipment/001.png");
// let marker = new maptalks.Marker(item.list, {
// properties: {
// sort,
// },
// symbol: [
// {
// markerType: "path",
// markerFile: file,
// markerWidth: {
// stops: [
// [7, 15],
// [18, 25],
// ],
// },
// markerHeight: {
// stops: [
// [7, 15],
// [18, 25],
// ],
// },
// markerDx: 0,
// markerDy: 20,
// markerOpacity: 1,
// },
// {
// textFaceName: "sans-serif",
// textName: "{sort}",
// textSize: 12,
// textDy: 30,
// textFill: "#7ee6ed",
// },
// ],
// });
// this.layer.addGeometry(marker);
// });
// },
// 绘制3dmark
// addMark3d(val) {
// console.log(val, 6677);
// this.layer2 = new maptalks.VectorLayer("vector3").addTo(this.map);
// val.forEach((item) => {
// let sort = item.sort;
// let file = require("@/assets/warehouseWeb/equipment/001.png");
// let marker = new maptalks.Marker(item.list, {
// properties: {
// sort,
// },
// symbol: [
// {
// markerType: "path",
// markerFile: file,
// markerWidth: {
// stops: [
// [7, 15],
// [18, 25],
// ],
// },
// markerHeight: {
// stops: [
// [7, 15],
// [18, 25],
// ],
// },
// markerDx: 0,
// markerDy: 20,
// markerOpacity: 1,
// },
// {
// textFaceName: "sans-serif",
// textName: "{sort}",
// textSize: 12,
// textDy: 30,
// textFill: "#7ee6ed",
// },
// ],
// });
// this.layer2.addGeometry(marker);
// });
// },
},
};
</script>
<style scoped>
.container {
width: calc(100% - 50px);
/* width: 550px; */
min-height: 550px;
margin: 15px;
}
/deep/ .maptalks-layer-switcher .panel .group:nth-child(2) {
display: none !important;
}
/deep/ .maptalks-layer-switcher .panel {
padding: 15px 0;
}
</style>
点位
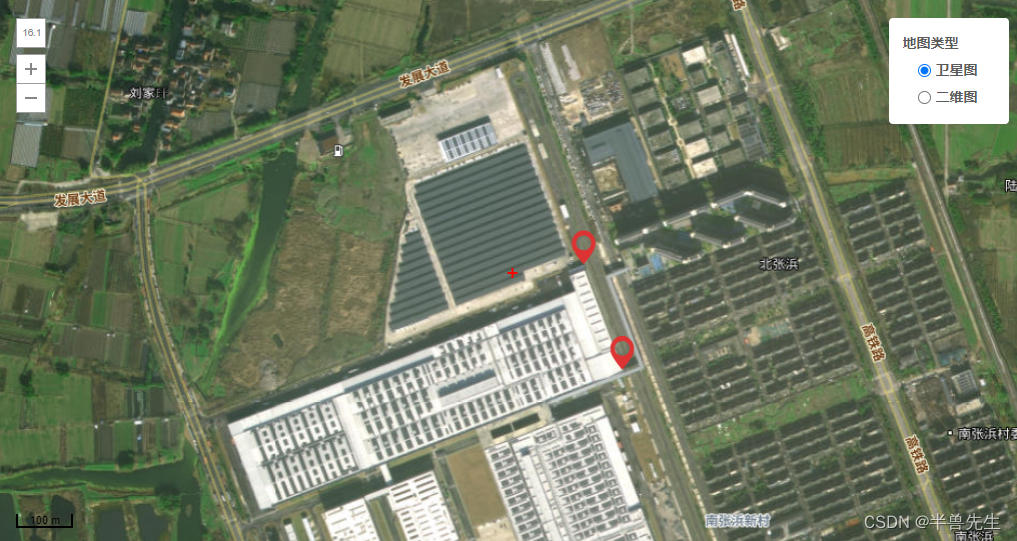
<!-- 地图组件 -->
<template>
<div :id="id" class="container"></div>
</template>
<script>
import _ from "lodash";
import "maptalks/dist/maptalks.css";
import * as maptalks from "maptalks";
export default {
//import引入的组件需要注入到对象中才能使用
name: "Map",
props: {
id: {
type: String,
default: () => {
return "map";
},
},
mapSet: {
type: Object,
default: () => {
return {};
},
},
color: {
type: String,
default: () => {
return "";
},
},
},
components: {},
data() {
//这里存放数据
return {
dataLis: [],
layer: null,
layer2: null,
map: null,
polygon: null,
drawTool: null,
// 默认中心点点位
center: [121.044372, 30.575405],
// 缩放层级
zoom: 14,
// 倾斜度
pitch: 0,
// 轴承
// bearing: 90,
// 最小缩放层级
minZoom: 1,
// 最大缩放层级
maxZoom: 18.5,
symbol: {
polygonFill: "#ff0000", // 图形覆盖颜色
polygonOpacity: 0.3, // 图形覆盖区域透明度
lineColor: "#ff0000", // 线条颜色
lineWidth: 2, // 线条宽度
},
pointSymbol: {
markerFile: "@/assets/logo/logo.png",
markerWidth: 20,
markerHeight: 20,
},
layerSwitcherControl: {
position: "top-right",
// title of base layers
baseTitle: "地图类型",
// title of layers
overlayTitle: "Layers",
// layers you don't want to manage with layer switcher
excludeLayers: [],
// css class of container element, maptalks-layer-switcher by default
containerClass: "maptalks-layer-switcher",
},
drawMap: {
Point: "点",
LineString: "线",
Polygon: "多边形",
Circle: "圆",
Ellipse: "椭圆",
Rectangle: "长方形",
},
};
},
//监听属性 类似于data概念
// computed: {},
//监控data中的数据变化
watch: {
mapSet: {
deep: true,
immediate: true,
async handler(val) {
if (Object.keys(val).length) {
let { drawMapType, drawMapArray, editable, draggable } = val;
if (this.map) this.map.remove();
await this.init();
if (drawMapArray && drawMapArray.length > 0) {
// console.log("地图获取到所有点位", drawMapArray);
drawMapArray.map(i => {
// console.log(123, i[0]);
let items = i[0]
let obj = {
drawMapArray: () => {
this.startEdit(items, {
drawMapType, //点位
editable: true,//可编辑的
draggable: true, //拖动
drawMapArray: items //数据
});
},
drawMapType: () => {
this.drawMapType({
drawMapType, //点位
editable: true,//可编辑的
draggable: true, //拖动
drawMapArray: items //数据
});
},
};
let arrayKeys = Object.keys({
drawMapType, //点位
editable: true,//可编辑的
draggable: true, //拖动
drawMapArray: items //数据
});
arrayKeys.map((items) => {
if (obj[items]) return obj[items]();
});
})
// let items2 = [121.720448, 29.978434]
// console.log(9992, items2);
// let obj2 = {
// drawMapArray: () => {
// this.startEdit(items2, {
// drawMapType, //点位
// editable: true,//可编辑的
// draggable: true, //拖动
// drawMapArray: items2 //数据
// });
// },
// drawMapType: () => {
// this.drawMapType({
// drawMapType, //点位
// editable: true,//可编辑的
// draggable: true, //拖动
// drawMapArray: items2 //数据
// });
// },
// };
// let arrayKeys2 = Object.keys({
// drawMapType, //点位
// editable: true,//可编辑的
// draggable: true, //拖动
// drawMapArray: items2 //数据
// });
// arrayKeys2.map((items2) => {
// if (obj2[items2]) return obj2[items2]();
// });
// 出现
// this.drawMapType({
// drawMapType, //点位
// editable: true,//可编辑的
// draggable: true, //拖动
// drawMapArray: undefined //数据
// });
}
this.drawMapType({
drawMapType, //点位
editable: true,//可编辑的
draggable: true, //拖动
drawMapArray: undefined //数据
});
// if (drawMapArray && drawMapArray.length > 0) {
// drawMapArray.forEach((item, indexs) => {
// console.log(999, item);
// let obj = {
// drawMapArray: () => {
// this.startEdit(item, {
// drawMapType, //点位
// editable: true,//可编辑的
// draggable: true, //拖动
// drawMapArray: item //数据
// });
// },
// drawMapType: () => {
// this.drawMapType({
// drawMapType, //点位
// editable: true,//可编辑的
// draggable: true, //拖动
// drawMapArray: item //数据
// });
// },
// };
// let arrayKeys = Object.keys({
// drawMapType, //点位
// editable: true,//可编辑的
// draggable: true, //拖动
// drawMapArray: item //数据
// });
// arrayKeys.map((item) => {
// if (obj[item]) return obj[item]();
// });
// })
// } else {
// let obj = {
// // 绘制图形
// drawMapType: () => {
// this.drawMapType({
// drawMapType, //点位
// editable: true,//可编辑的
// draggable: true, //拖动
// drawMapArray: item //数据
// });
// },
// };
// let arrayKeys = Object.keys({
// drawMapType, //点位
// editable: true,//可编辑的
// draggable: true, //拖动
// drawMapArray: item //数据
// });
// arrayKeys.map((item) => {
// if (obj[item]) return obj[item]();
// });
// }
// ----------------
// // 循环插入多边形
// if (drawMapArray && drawMapArray.length > 0) {
// drawMapArray.forEach(item => {
// let obj = {
// // 编辑图形
// drawMapArray: () => {
// this.startEdit(item, {
// drawMapType,
// editable,
// draggable,
// drawMapArray: item
// });
// },
// // 绘制图形
// drawMapType: () => {
// this.drawMapType({
// drawMapType,
// editable,
// draggable,
// drawMapArray: item
// });
// },
// };
// let arrayKeys = Object.keys({
// drawMapType,
// editable,
// draggable,
// drawMapArray: item
// });
// arrayKeys.map((item) => {
// if (obj[item]) return obj[item]();
// });
// })
// } else {
// let obj = {
// // 编辑图形
// // drawMapArray: () => {
// // this.startEdit(item, {
// // drawMapType,
// // editable,
// // draggable,
// // drawMapArray
// // });
// // },
// // 绘制图形
// drawMapType: () => {
// this.drawMapType({
// drawMapType,
// editable,
// draggable,
// drawMapArray
// });
// },
// };
// let arrayKeys = Object.keys({
// drawMapType,
// editable,
// draggable,
// drawMapArray
// });
// arrayKeys.map((item) => {
// if (obj[item]) return obj[item]();
// });
// }
} else {
// console.log("销毁地图");
if (this.map != undefined) this.map.remove();
}
},
},
color: {
immediate: true,
handler(val) {
if (val) {
let { drawTool, polygon } = this;
if (drawTool) this.changeSymbol("drawTool", drawTool, val);
if (polygon) this.changeSymbol("Point", polygon, val);
// if (drawTool) {
// drawTool.setSymbol({
// polygonFill: val, // 图形覆盖颜色
// polygonOpacity: 0.3, // 图形覆盖区域透明度
// lineColor: val, // 线条颜色
// lineWidth: 2 // 线条宽度
// });
// }
// if (polygon) {
// polygon.updateSymbol({
// polygonFill: val, // 图形覆盖颜色
// lineColor: val // 线条颜色
// });
// }
}
},
},
},
//生命周期 - 创建完成(可以访问当前this实例)
// created() { },
//生命周期 - 挂载完成(可以访问DOM元素)
// mounted() {
// let a = "rgb(255, 69, 0)";
// console.log(a.slice(5, a.length - 1));
// },
// beforeDestroy() {
// let { map } = this;
// // console.log(
// // "🚀 ~ Date:2023/07/11 14:37 ~ File: index.vue ~ Line:154 ----- ",
// // 销毁地图
// // );
// if (map) map.remove();
// },
//方法集合
methods: {
// 获取到图层所有的点位
extractCoordinates(data) {
// console.log("获取到图层所有的点位", data);
let datas = []
data.forEach(res => {
datas.push({
"lngWgs": res._coordinates.x,
"latWgs": res._coordinates.y
})
})
// console.log(111111, datas);
return datas;
},
// getSent(data) {
// let array = []
// data.forEach(res => {
// let arrray2 = []
// res._coordinates.forEach(res2 => {
// arrray2.push({
// "lngWgs": res2.x,
// "latWgs": res2.y,
// // "sort": index2
// })
// })
// array.push(arrray2)
// })
// return array;
// },
init() {
this.$nextTick(() => {
let { center, zoom, pitch, minZoom, maxZoom, symbol, layerSwitcherControl, id } = this;
this.map = new maptalks.Map(id, {
center,
zoom,
pitch,
minZoom,
maxZoom,
centerCross: true,
seamlessZoom: true,
attribution: false,
zoomControl: true, // add zoom control
scaleControl: true, // add scale control
layerSwitcherControl,
symbol,
// baseLayer 表示基础图层,它可以添加多个,逗号隔开
baseLayer: new maptalks.GroupTileLayer("Base TileLayer", [
// 卫星图
new maptalks.TileLayer("卫星图", {
urlTemplate: "https://t5.tianditu.gov.cn/DataServer?T=img_w&X={x}&Y={y}&L={z}&tk=074d4a21c8f34a3a20cd1f69f81b26bf",
subdomains: ["a", "b", "c", "d"], //负载均衡-以提高地图数据加载的效率
}),
// 二维图
new maptalks.TileLayer("二维图", {
visible: false,
urlTemplate:
"https://map.geoq.cn/arcgis/rest/services/ChinaOnlineCommunity/MapServer/tile/{z}/{y}/{x}",
subdomains: ["a", "b", "c", "d"],
})
]),
});
// 添加-地图矢量数据图层
var tiandituLayer = new maptalks.TileLayer('tianditu', {
urlTemplate: 'http://t0.tianditu.gov.cn/cia_w/wmts?SERVICE=WMTS&REQUEST=GetTile&VERSION=1.0.0&LAYER=cia&STYLE=default&TILEMATRIXSET=w&FORMAT=tiles&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}&tk=074d4a21c8f34a3a20cd1f69f81b26bf',
subdomains: ['a', 'b', 'c']
});
this.map.addLayer(tiandituLayer);
// 监听图层切换监听
this.map.getBaseLayer().on('layerload', function (e) {
let visible = e.target.layers[0].options.visible
if (visible) {
// console.log("卫星图", this.map._layers[0].options.visible);
this.map._layers[0].options.visible = true // 显示-矢量数据图层
} else {
// console.log("二维图", this.map._layers);
this.map._layers[0].options.visible = false // 隐藏-矢量数据图层
}
});
// 创建地图容器
// var map = new maptalks.Map('map', {
// center: [116.3975, 39.9085],
// zoom: 10,
// baseLayer: new maptalks.TileLayer('base', {
// urlTemplate: 'http://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png',
// subdomains: ['a', 'b', 'c']
// })
// });
// this.layer = new maptalks.VectorLayer("vector").addTo(this.map)
this.layer = new maptalks.VectorLayer("vector").addTo(this.map)
this.layer2 = new maptalks.VectorLayer("vector2").addTo(this.map)
// this.map.on("click", function (params) {
// });
// this.map.on("editrecord", function (params) {
// console.log(params);
// });
});
},
// 绘制图形
drawMapType(val) {
// !this.layer ? this.layer = new maptalks.VectorLayer("vector").addTo(this.map) : null
// console.log('绘制图形', val, this.map);
let { drawMapType, drawMapArray } = val;
if (!drawMapArray) {
let { map, symbol, color } = this;
let that = this;
// var layer = new maptalks.VectorLayer("vector").addTo(map);
var drawTool = new maptalks.DrawTool({
mode: drawMapType,
// once: true,
// once: false,
symbol: drawMapType == "Point" ? null : symbol,
})
.addTo(map)
.disable();
drawTool.on("drawend", function (param) {
// console.log(param.geometry);
let { _coordinates } = param.geometry;
let array = null;
if (drawMapType == "Point") {
array = [_coordinates.x, _coordinates.y];
} else if (drawMapType == "Polygon") {
array = _coordinates.map((item) => {
return [item.x, item.y];
});
}
that.dataLis.push(array)
// console.log(123, array);
that.layer.addGeometry(param.geometry);
that.startEdit(array, val, that.dataLis.length - 1);
that.layer.remove();
that.layer.setZIndex(6);
// that.$nextTick(() => {
// console.log("绘制好了 发送", that.getSent(that.layer2._geoList));
// that.$emit("drawPointChange", that.getSent(that.layer2._geoList));
// })
// console.log(666666, that.extractCoordinates(that.layer._geoList));
// that.$emit("drawPointChange", that.extractCoordinates(that.layer._geoList));
// console.log(666666, that.layer._geoList);
});
this.drawTool = drawTool;
drawTool.setMode(drawMapType).enable();
// 改变覆盖物设置
if (color) this.changeSymbol("drawTool", drawTool, color);
// if (color) {
// drawTool.setSymbol({
// polygonFill: color, // 图形覆盖颜色
// polygonOpacity: 0.3, // 图形覆盖区域透明度
// lineColor: color, // 线条颜色
// lineWidth: 2 // 线条宽度
// });
// }
}
},
// 编辑绘制图形
startEdit(array, val) {
// let nun = Math.random();
// console.log("编辑绘制图形", array, val);
let that = this;
let { drawMapType, editable, draggable } = val;
let { symbol, map, pointSymbol, color } = that;
let type = null;
// let pointArray = _.clone(array);
// that.$emit("drawPointChange", pointArray);
// setTimeout(()=>{
// that.$emit("drawPointChange", that.extractCoordinates(that.layer2._geoList));
// },3000)
let objArray = [
[() => drawMapType == "Point",
() => {
type = "Marker";
},
() => {
// 更改位置
// polygon.on("shapechange", function (param) {
// console.log(6666666666, param, that.extractCoordinates(that.layer2._geoList));
// // that.extractCoordinates(that.layer._geoList)
// // let { _coordinates } = param.target;
// // pointArray = [_coordinates.x, _coordinates.y];
// // that.$emit("drawPointChange", pointArray);
// that.$emit("drawPointChange", that.extractCoordinates(that.layer2._geoList));
// });
// 更改位置
polygon.on("positionchange", function (param) {
// console.log(110, param, that.extractCoordinates(that.layer2._geoList));
// that.extractCoordinates(that.layer._geoList)
// let { _coordinates } = param.target;
// pointArray = [_coordinates.x, _coordinates.y];
// that.$emit("drawPointChange", pointArray);
that.$emit("drawPointChange", that.extractCoordinates(that.layer2._geoList));
});
// 更改位置
polygon.on("remove", function (param) {
// console.log(110, param, that.extractCoordinates(that.layer2._geoList));
// that.extractCoordinates(that.layer._geoList)
// let { _coordinates } = param.target;
// pointArray = [_coordinates.x, _coordinates.y];
// that.$emit("drawPointChange", pointArray);
that.$emit("drawPointChange", that.extractCoordinates(that.layer2._geoList));
});
},
]
];
let state = objArray.find((m) => m[0]());
if (state) state[1]();
let polygon = new maptalks[type](array, {
visible: true,
editable,
cursor: "pointer",
draggable,
dragShadow: false,
drawOnAxis: null,
symbol: drawMapType == "Point" ? null : symbol,
});
var options = {
'items': [
{
item: '删除', click: function (e) {
that.layer2.removeGeometry(polygon);
}
}
]
};
polygon.setMenu(options).openMenu();
// new maptalks.VectorLayer("vectorEdit" + nun, polygon).addTo(map);
that.layer2.addGeometry(polygon);
that.$emit("drawPointChange", that.extractCoordinates(that.layer2._geoList));
// that.layer.addGeometry(polygon);
map.fitExtent(polygon.getExtent(), -1); // 自动适配区域
if (color) this.changeSymbol("Point", polygon, color);
if (state) state[2]();
that.polygon = polygon;
},
// 覆盖物设置更改
changeSymbol(type, mapObj, color) {
let obj = {
drawTool: () => {
mapObj.setSymbol({
polygonFill: color, // 图形覆盖颜色
polygonOpacity: 0.3, // 图形覆盖区域透明度
lineColor: color, // 线条颜色
lineWidth: 2, // 线条宽度
});
},
polygon: () => {
mapObj.updateSymbol({
polygonFill: color, // 图形覆盖颜色
lineColor: color, // 线条颜色
});
},
};
if (obj[type]) return obj[type]();
},
},
};
</script>
<style scoped>
.container {
width: calc(100% - 50px);
/* width: 550px; */
min-height: 550px;
margin: 15px;
}
/deep/ .maptalks-layer-switcher .panel .group:nth-child(2) {
display: none !important;
}
/deep/ .maptalks-layer-switcher .panel {
padding: 15px 0;
}
</style>