question
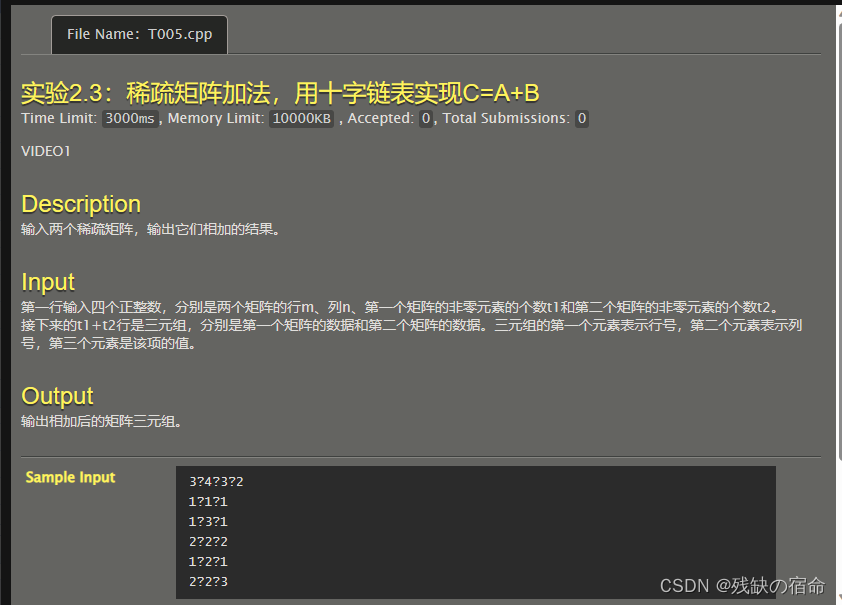
完整代码
#include <iostream>
#include <vector>
#include <iomanip>
using DataType = int;
struct TridaNode
{
DataType val;
int row, col;
TridaNode *right, *down;
};
using TridaList = TridaNode *;
struct CrossNode
{
TridaList *row_head, *col_head;
int m, n, len;
};
using CrossList = CrossNode *;
void CreatCrossList(CrossNode &Mat);
void InsertNode(CrossNode &Mat, int row, int col, DataType val);
void AddCrossList(CrossNode A, CrossNode B, CrossNode &C);
void showSparseMatrix(CrossNode Mat);
void SparsetoNormal(CrossNode Mat);
int main()
{
CrossNode A, B, C;
int m, n, t1, t2;
std::cin >> m >> n >> t1 >> t2;
A.m = B.m = m, A.n = B.n = n, A.len = t1, B.len = t2;
CreatCrossList(A);
CreatCrossList(B);
AddCrossList(A, B, C);
showSparseMatrix(C);
system("pause");
return 0;
}
void CreatCrossList(CrossNode &Mat)
{
Mat.col_head = new TridaList[Mat.n + 1]{nullptr};
Mat.row_head = new TridaList[Mat.m + 1]{nullptr};
if (!Mat.col_head || !Mat.row_head)
{
std::cout << "分配内存失败!" << std::endl;
return;
}
int row, col;
DataType val;
for (auto i = 0; i < Mat.len; ++i)
{
std::cin >> row >> col >> val;
InsertNode(Mat, row, col, val);
}
}
void InsertNode(CrossNode &Mat, int row, int col, DataType val)
{
TridaList p = new TridaNode;
if (!p)
{
std::cout << "分配内存失败!" << std::endl;
return;
}
p->row = row, p->col = col, p->val = val, p->right = nullptr, p->down = nullptr;
if (!Mat.row_head[row])
Mat.row_head[row] = p;
else
{
TridaList q = Mat.row_head[row];
while (q->right && q->right->col < col)
q = q->right;
p->right = q->right, q->right = p;
}
if (!Mat.col_head[col])
Mat.col_head[col] = p;
else
{
TridaList q = Mat.col_head[col];
while (q->down && q->down->row < row)
q = q->down;
p->down = q->down, q->down = p;
}
}
void AddCrossList(CrossNode A, CrossNode B, CrossNode &C)
{
int size = 0;
if (A.m != B.m || A.n != B.n)
{
std::cout << "输入矩阵不合法!" << std::endl;
return;
}
C.m = A.m, C.n = A.n;
C.col_head = new TridaList[C.n + 1]{nullptr};
C.row_head = new TridaList[C.m + 1]{nullptr};
for (auto i = 0; i < A.m; ++i)
{
TridaList A_temp = A.row_head[i], B_temp = B.row_head[i];
if (!B_temp)
{
while (A_temp)
{
InsertNode(C, A_temp->row, A_temp->col, A_temp->val);
++size;
A_temp = A_temp->right;
}
}
else
{
if (!A_temp)
{
while (B_temp)
{
InsertNode(C, B_temp->row, B_temp->col, B_temp->val);
++size;
B_temp = B_temp->right;
}
}
else
{
while (A_temp && B_temp)
{
if (A_temp->row < B_temp->row || (A_temp->row == B_temp->row && A_temp->col < B_temp->col))
{
InsertNode(C, A_temp->row, A_temp->col, A_temp->val);
++size;
A_temp = A_temp->right;
}
else if (B_temp->row < A_temp->row || (B_temp->row == A_temp->row && B_temp->col < A_temp->col))
{
InsertNode(C, B_temp->row, B_temp->col, B_temp->val);
++size;
B_temp = B_temp->right;
}
else
{
int sum = A_temp->val + B_temp->val;
if (sum != 0)
{
InsertNode(C, A_temp->row, A_temp->col, sum);
++size;
}
A_temp = A_temp->right;
B_temp = B_temp->right;
}
}
while (A_temp)
{
InsertNode(C, A_temp->row, A_temp->col, A_temp->val);
++size;
A_temp = A_temp->right;
}
while (B_temp)
{
InsertNode(C, B_temp->row, B_temp->col, B_temp->val);
++size;
B_temp = B_temp->right;
}
}
}
}
C.len = size;
}
void showSparseMatrix(CrossNode Mat)
{
for (auto i = 0; i < Mat.m; ++i)
{
TridaList p = Mat.row_head[i];
while (p)
{
std::cout << p->row << " " << p->col << " " << p->val << std::endl;
p = p->right;
}
}
}
void SparsetoNormal(CrossNode Mat)
{
std::vector<std::vector<int>> temp(Mat.m, std::vector<int>(Mat.n, 0));
for (auto i = 0; i < Mat.m; ++i)
{
TridaList p = Mat.row_head[i];
while (p)
{
temp[p->row][p->col] = p->val;
p = p->right;
}
}
for (auto i = 0; i < Mat.m; ++i)
{
for (auto j = 0; j < Mat.n; ++j)
{
std::cout << std::setw(3) << temp[i][j] << ' ';
}
std::cout << std::endl;
}
}