顺序结构
#define _CRT_SECURE_NO_WARNINGS
#define MAX_SIZE 1000
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
typedef struct peo
{
char name[20];
char number[20];
char tele[20];
}peo;
typedef struct contact
{
peo arr[MAX_SIZE];
int count;
}contact;
void print_menu()
{
printf("-------------------------------------------------\n");
printf("* 1.添加信息 2.删除信息 3.查找信息 *\n");
printf("* *\n");
printf("* 4.修改信息 5.打印信息 0.退出 *\n");
printf("-------------------------------------------------\n");
}
void init_contact(contact *pcon)
{
pcon->count = 0;
memset(pcon->arr, 0, MAX_SIZE * sizeof(peo));
}
void Add(contact *pcon)
{
if (pcon->count == MAX_SIZE)
{
printf("顺序表是满的!!!\n");
exit(1);
}
printf("请输入名字>>>");
scanf("%s", pcon->arr[pcon->count].name);
printf("请输入学号>>>");
scanf("%s", pcon->arr[pcon->count].number);
printf("请输入电话号码>>>");
scanf("%s", pcon->arr[pcon->count].tele);
pcon->count++;
}
void print_xinxi(contact *pcon)
{
int i = 0;
if (!pcon->count)
{
printf("顺序表是空的,请输入信息!!!\n");
}
else
{
printf("序号 姓名 学号 电话号码\n");
for (i = 0; i < pcon->count; i++)
{
printf("%d %s %s %s\n", i+1,pcon->arr[i].name, pcon->arr[i].number, pcon->arr[i].tele);
}
}
}
int find(contact *pcon)
{
int i = 0;
char name[20];
int ret = 0;
printf("请输入想要查找的名字:");
scanf("%s", name);
for (i = 0; i < pcon->count; i++)
{
ret = strcmp(name, pcon->arr[i].name);
if (ret == 0)
{
return i;
}
}
return -1;
}
int deletel(contact *pcon)
{
int i = 0;
int ret = 0;
printf("删除联系人\n");
ret = find(pcon);
if (ret == -1)
{
printf("你要删除的姓名不存在!!!\n");
return -1;
}
else
{
for (i = ret; i < pcon->count - 1; i++)
{
pcon->arr[i] = pcon->arr[i + 1];
}
pcon->count--;
return 0;
}
}
int revise(contact *pcon)
{
int ret = 0;
printf("修改联系人信息>");
ret = find(pcon);
if (ret == -1)
{
printf("你要修改的联系人不存在!!!\n");
return -1;
}
else
{
printf("请输入要修改的数据:\n");
printf("请输入名字>>>");
scanf("%s", pcon->arr[ret].name);
printf("请输入学号>>>");
scanf("%s", pcon->arr[ret].number);
printf("请输入电话>>>");
scanf("%s", pcon->arr[ret].tele);
return 0;
}
}
int find_contacts(contact *pcon)
{
int ret = 0;
ret = find(pcon);
if (ret == -1)
{
printf("你要查找的名字不存在!!!\n");
return -1;
}
else
{
printf("姓名:%s\n学号:%s\n电话:%s\n",pcon->arr[ret].name,pcon->arr[ret].number,pcon->arr[ret].tele);
return 0;
}
}
int main()
{
contact con;
int input = 1;
init_contact(&con);
do
{
print_menu();
printf("请选择>");
scanf("%d", &input);
switch (input)
{
case 1:
Add(&con);
break;
case 2:
deletel(&con);
break;
case 3:
find_contacts(&con);
break;
case 4:
revise(&con);
break;
case 5:
print_xinxi(&con);
break;
default:
break;
}
} while (input);
return 0;
}
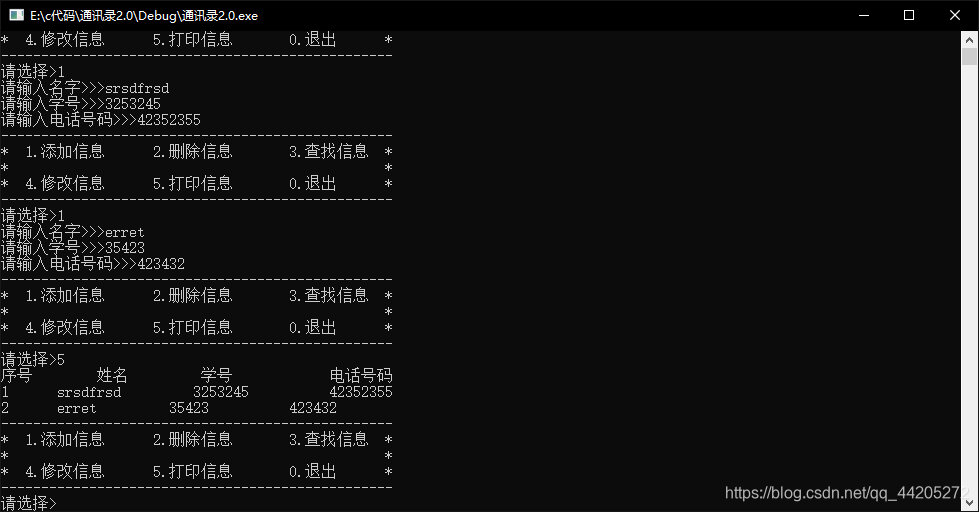
链式结构
#define _CRT_SECURE_NO_WARNINGS
#include<stdio.h>
#include<malloc.h>
#include<stdlib.h>
#include<string.h>
#include<Windows.h>
typedef struct node
{
char name[20];
char id[20];
char tel[20];
struct node *next;
}lnode, *LinkList;
void menue()
{
printf("************************************\n");
printf("***** 1-打印 2-添加 3-插入 *****\n");
printf("***** 4-删除 5-查找 6-修改 *****\n");
printf("***** 7-清屏 8-人数 0-退出 *****\n");
printf("************************************\n");
}
lnode* head = (lnode*)malloc(sizeof(lnode));
int add( )
{
struct node *p , *pre;
int n, i;
printf("请输入你想要的添加几个学生>>>");
scanf("%d", &n);
p = (struct node *)malloc(sizeof(struct node));
head = p;
pre = p;
printf("请输入第1个学生的数据(中间用空格分开)>>>");
scanf("%s%s%s", p->name,p->id,p->tel);
p->next = NULL;
for (i = 2; i <= n; i++)
{
p = (struct node *)malloc(sizeof(struct node));
printf("请输入第%d个学生的数据(中间用空格分开)>>>", i);
scanf("%s%s%s", p->name, p->id, p->tel);
p->next = NULL;
pre->next = p;
pre = p;
}
return 0;
}
int Length()
{
lnode * p = head;
int j = 0;
while (p->next)
{
p = p->next;
j++;
}
printf("通讯录里总共有%d个学生\n",j+1);
return 0;
}
void print()
{
lnode *p;
p = head;
if (p != NULL)
{
printf("已有成员如下:\n");
printf(" 姓名 学号 电话\n");
while (p != NULL)
{
printf("%10s%10s%10s\n", p->name, p->id, p->tel);
p = p->next;
}
}
else
{
printf("通讯录目前无信息!\n\n");
}
}
void find()
{
lnode *p;
p = head;
int k = 0;
printf("请输入你想要的查找第几个人>>>");
scanf("%d", &k);
int j = 1;
while (p->next != NULL && j < k)
{
p = p->next;
j++;
}
printf("你想查找的学生信息如下>>>\n");
printf("姓名>>>%s\n学号>>>%s\n电话>>>%s\n", p->name, p->id, p->tel);
}
int insert()
{
int k = 0;
printf("请输入你想要插入第几个人后面>>>");
scanf("%d", &k);
lnode *q;
lnode *p = head;
int j = 1;
while (p->next != NULL && j < k)
{
p = p->next;
j++;
}
if (j == k)
{
q = (lnode*)malloc(sizeof(lnode));
printf("请输入想要插入的数据>>>");
scanf("%s%s%s", q->name, q->id, q->tel);
q->next = p->next;
p->next = q;
}
else
printf("查找的人不存在!!!不能插入");
return 0;
}
int dele()
{
lnode *r,*pre;
lnode *p = head;
int k = 1;
printf("请输入你想要删除第几个人>>>");
scanf("%d", &k);
int j = 1;
pre = head;
r = head;
while (p->next != NULL && j < k)
{
p = pre;
p = p->next;
p = r;
j++;
}
if (j == k)
{
if (p == head)
{
head = p->next;
free(p);
}
else if(r->next != NULL)
{
pre = p;
p->next = r->next;
free(r);
}
else
{
p->next = NULL;
free(r);
}
}
else
printf("查找的人不存在!!!不能删除");
return 0;
}
int xiugai()
{
int k = 0;
printf("请输入你想要修改第几位学生>>>");
scanf("%d", &k);
lnode *p = head;
int j = 1;
while (p->next != NULL && j < k)
{
p = p->next;
j++;
}
if (j == k)
{
printf("请输入想要修改的数据>>>");
scanf("%s%s%s", p->name, p->id, p->tel);
}
else
printf("查找的人不存在!!!不能修改");
return 0;
}
void init()
{
system("cls");
}
int main()
{
lnode* head = (lnode*)malloc(sizeof(lnode));
if (head == NULL)
{
printf("malloc error!\n");
return -1;
}
else
{
while(1)
{
int temp = 0;
menue();
printf("请输入你想要的操作:");
scanf("%d", &temp);
switch (temp)
{
case 1: {
print();
break;
}
case 2: {
add();
system("cls");
break;
}
case 3: {
insert();
system("cls");
break;
}
case 4: {
dele();
system("cls");
break;
}
case 5: {
find();
break;
}
case 6: {
xiugai();
system("cls");
break;
}
case 7:
{
init();
system("cls");
break;
}
case 8: {
Length();
break;
}
case 0:
{
exit(0);
}
default:
{
printf("输入错误!");
break;
}
}
}
}
return 0;
}
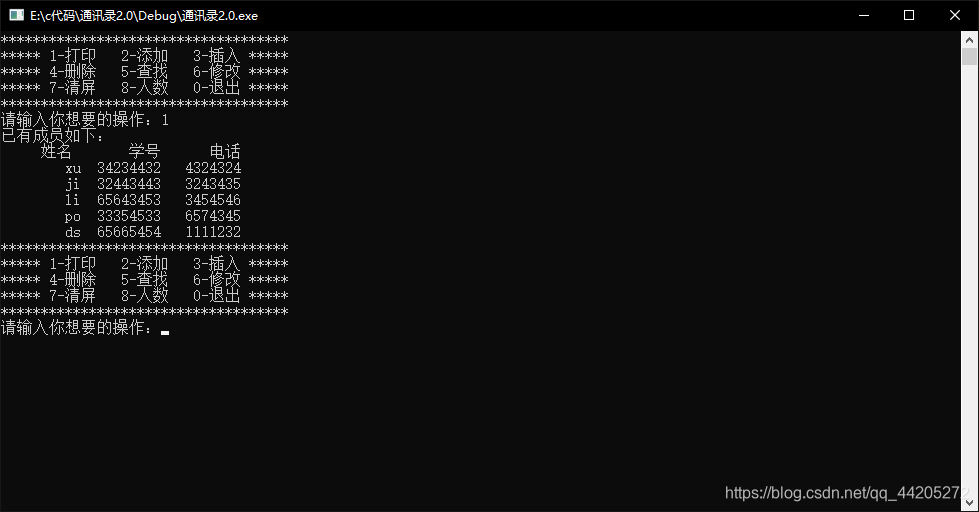