string类介绍
string类用于初始化单字节字符和字符产类型的数据。
string的底层实现
string继承自basic_string,其实是对char进行了封装,封装的string包含了char数组,容量,长度等等属性。
string不是一个单独的容器,只是basic_string模板类中的一个typedef,相对应的还有wstring等。在实际使用过程中,并不区分他们的区别。
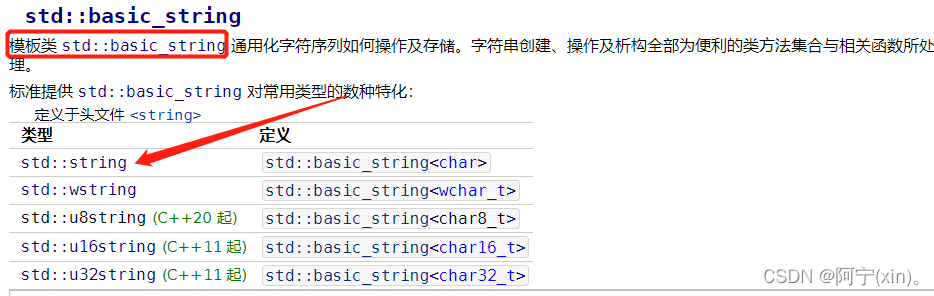
string相对于一个保存字符的序列容器,除了有字符串的一些常用操作外,还包含了所有其他容器的操作,包括:增加,删除,修改,查找比较,输入,输出等。
在函数使用过程中,可认为basic_string就是string。
string可以进行动态扩展,在每次扩展的时候另外申请一块原空间大小两倍的空间(2^n),然后将原字符串拷贝过去,并加上新增的内容。
string扩容
string中的成员类型
成员类型 | 定义 |
---|
value_type | 值类型(char) |
size_type | size_t |
difference_type | 差值类型(int) |
reference | value_type& |
const_reference | const value_type& |
pointer | value_type* |
const_pointer | const value_type* |
iterator | 随机访问迭代器 |
const_iterator | 随机访问迭代器 |
reverse_iterator | std::reverse_iterator |
const_reverse_iterator | std::reverse_iterator<const_iterator> |
string函数介绍
构造函数
成员函数 | 功能 |
---|
basic_string(); | 默认构造函数,构造空string(零大小和未指定的容量) |
basic_string(size_type count,CharT ch); | 构造具有count个字符ch的string |
basic_string(const CharT* s); | 以s指向的字符串构造string |
basic_string(const CharT* s,size_type count); | 以s指向的字符串的前count个字符构造string |
basic_string(const CharT* s,size_type pos,size_type count); | 以s指向的字符串的[pos,pos + count)的几个字符构造string |
basic_string(const basic_string&other); | 复制构造函数构造string |
basic_string(const basic_string&other,size_type pos); | 复制构造函数从pos位置构造string |
basic_string(const basic_string&other,size_type pos,size_type count); | 复制构造函数从pos位置起count个字符构造string |
basic_string(std::initializer_list ilist); | 构造拥有initializer_list ilist 内容的string |
basic_string& operator=(const basic_string& str); | 以str的内容替换内容 |
basic_string& operator=(std::initializer_list ilist); | 以initializer_list ilist 的内容替换内容 |
basic_string& assign(const CharT* s); | 以s所指向的空终止字符串的内容替换内容 |
basic_string& assign(size_type count,CharT ch); | 以count个ch的内容替换内容 |
basic_string& assign(const basic_string& str); | 以str的内容替换内容 |
basic_string& assign(const basic_string& str,size_type pos,size_type count); | 以str的字串[pos,pos + count]内容替换内容 |
#include<iostream>
#include<string>
using namespace std;
int main()
{
string s1;
string s2(10, 'a');
string s3("hello world");
string s4("hello world", 5);
string s5("hello world", 3,5);
string s6(s3);
string s7(s3, 3);
string s8(s3, 3, 5);
string s9 = "hello world";
string s10;
s10.assign("hello world", 3, 5);
cout << "s1:" << s1 << endl;
cout << "s2:" << s2 << endl;
cout << "s3:" << s3 << endl;
cout << "s4:" << s4 << endl;
cout << "s5:" << s5 << endl;
cout << "s6:" << s6 << endl;
cout << "s7:" << s7 << endl;
cout << "s8:" << s8 << endl;
cout << "s9:" << s9 << endl;
cout << "s10:" << s10 << endl;
return 0;
}
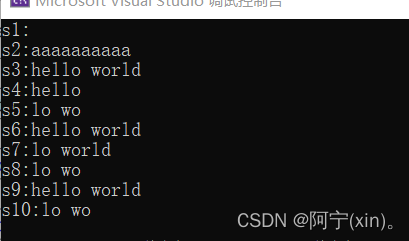
元素访问
元素访问 | 功能 |
---|
reference at( size_type pos ); | 返回到位于指定位置 pos 的字符的引用。进行边界检查,非法访问时抛出 std::out_of_range 类型的异常。 |
const_reference at( size_type pos ); | 返回到位于指定位置 pos 的字符的引用。进行边界检查,非法访问时抛出 std::out_of_range 类型的异常。 |
reference operator[]( size_type pos ); | 返回到位于指定位置 pos 的字符的引用。不进行边界检查。若 pos > size() ,则行为未定义。 |
const_reference operator[]( size_type pos ) const; | 返回到位于指定位置 pos 的字符的引用。不进行边界检查。若 pos > size() ,则行为未定义。 |
CharT& front(); | 返回到 string 中首字符的引用。若 empty() == true 则行为未定义。 |
const CharT& front() const; | 返回到 string 中首字符的引用。若 empty() == true 则行为未定义。 |
CharT& back(); | 返回string中的末字符。若 empty() == true 则行为未定义。 |
const CharT& back() const; | 返回string中的末字符。若 empty() == true 则行为未定义。 |
const CharT* data() const; | 返回指向作为字符存储工作的底层数组的指针。data() 与 c_str() 进行同一功能。 |
CharT* data() ; | 返回指向作为字符存储工作的底层数组的指针。data() 与 c_str() 进行同一功能。 |
const CharT* c_str() const; | 返回指向拥有数据等价于存储于字符串中的空终止字符数组的指针。data() 与 c_str() 进行同一功能。 |
#include<iostream>
#include<string>
using namespace std;
int main()
{
string s1 = "hello world";
char ch1 = s1.at(3);
char ch2 = s1[3];
char ch3 = s1.front();
char ch4 = s1.back();
const char* str1 = s1.data();
const char* cstr = s1.c_str();
cout << "s1:" << s1 << endl;
cout << "ch1:" << ch1 << endl;
cout << "ch2:" << ch2 << endl;
cout << "ch3:" << ch3 << endl;
cout << "ch4:" << ch4 << endl;
cout << "str1:" << str1 << endl;
cout << "cstr:" << cstr << endl;
return 0;
}
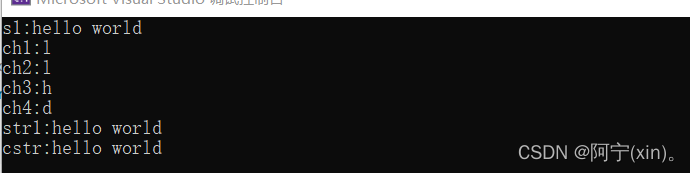
迭代器(注意越界)
迭代器 | 功能 |
---|
iterator begin(); | 返回指向string首字符的迭代器。 |
const_iterator begin() const; | 返回指向string首字符的迭代器。 |
iterator end(); | 返回指向后随string末字符的字符的迭代器。 |
const_iterator end() const; | 返回指向后随string末字符的字符的迭代器。 |
reverse_iterator rbegin(); | 返回指向逆转string首字符的逆向迭代器。 |
const_reverse_iterator rbegin() const; | 返回指向逆转string首字符的逆向迭代器。 |
reverse_iterator rend(); | 返回指向后随逆向string末字符的字符的逆向迭代器。 |
const_reverse_iterator rend() const; | 返回指向后随逆向string末字符的字符的逆向迭代器。 |
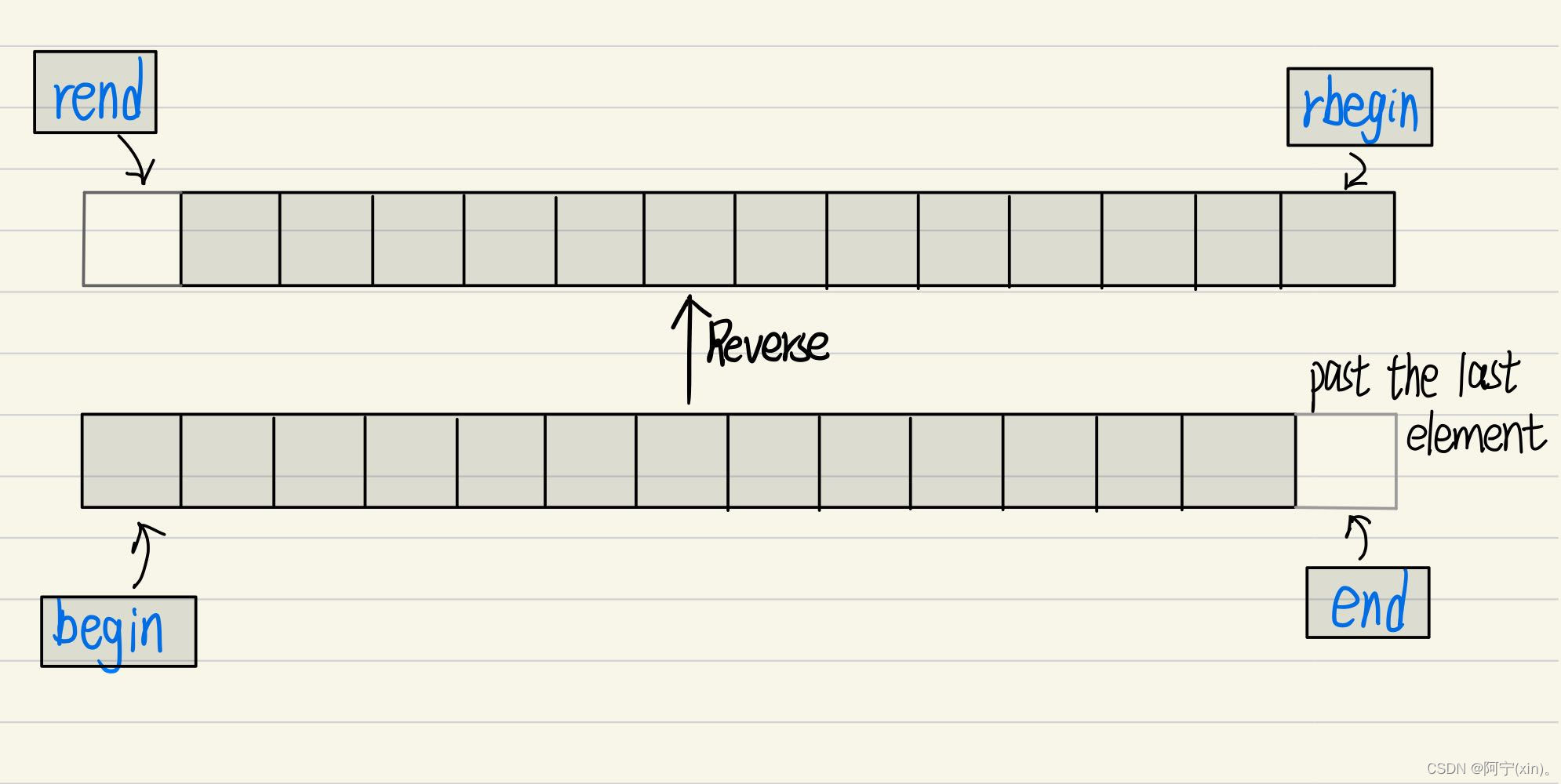
#include<iostream>
#include<string>
using namespace std;
int main()
{
string s1 = "hello world";
auto ch1 = *s1.begin();
auto ch2 = *(s1.end() - 1);
auto ch3 = *s1.rbegin();
auto ch4 = *(s1.rend() - 1) ;
*s1.begin() = 'x';
cout << "ch1:" << ch1 << endl;
cout << "ch2:" << ch2 << endl;
cout << "ch3:" << ch3 << endl;
cout << "ch4:" << ch4 << endl;
cout << "s1:" << s1 << endl;
return 0;
}
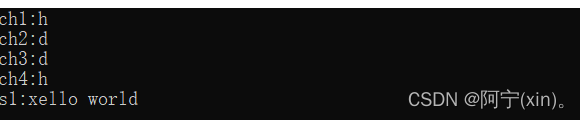
插入(insert,push_back)和删除(erase,pop_back,clear)
插入和删除 | 功能 |
---|
basic_string& insert( size_type index, size_type count, CharT ch ); | 在位置 index 插入 count 个字符 ch 的副本。 |
basic_string& insert( size_type index, const CharT* s ); | 在位置 index 插入 s 所指向的空终止字符串。 |
basic_string& insert( size_type index, const CharT* s, size_type count ); | 在位置 index 插入范围 [s, s+count) 中的字符。范围能含有空字符。 |
basic_string& insert( size_type index, const basic_string& str ); | 在位置 index 插入 string str 。 |
basic_string& insert( size_type index, const basic_string& str, size_type index_str, size_type count ); | 在位置 index 插入由 str.substr(index_str, count) 获得的 string 。 |
basic_string& erase( size_type index = 0, size_type count = npos ); | 移除始于 index 的 min(count, size() - index) 个字符。 |
iterator erase( iterator position ); | 移除位于 position 的字符。 |
iterator erase( iterator first, iterator last ); | 移除范围 [first, last) 中的字符。 |
void push_back( CharT ch ); | 后附给定字符 ch 到字符串尾。 |
void pop_back(); | 从字符串移除末字符。 |
void clear(); | 从 string 移除所有字符。 |
#include<iostream>
#include<string>
using namespace std;
int main()
{
string s1 = "hello world";
s1.insert(1, "this");
cout << "s1:" << s1 << endl;
s1.erase(1, 3);
cout << "s1:" << s1 << endl;
s1.push_back('a');
cout << "s1:" << s1 << endl;
s1.pop_back();
cout << "s1:" << s1 << endl;
s1.clear();
cout << "s1:" << s1 << endl;
return 0;
}
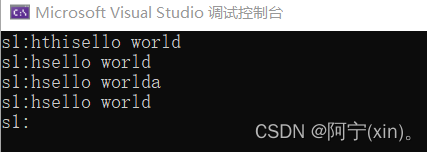
插入(append,+=(尾部附加数据))
插入(尾部附加数据) | 功能 |
---|
basic_string& append( size_type count, CharT ch ); | 后附 count 个 ch 的副本 |
basic_string& append( const basic_string& str ); | 后附 string str |
basic_string& append( const basic_string& str,size_type pos, size_type count ); | 后附 str 的子串 [pos, pos+count) 。若 pos > str.size() ,则抛出 std::out_of_range 。 |
basic_string& operator+=( const basic_string& str ); | 后附 string str 。 |
basic_string& operator+=( CharT ch ); | 后附字符 ch 。 |
basic_string& operator+=( const CharT* s ); | 后附 s 所指向的空终止字符串。 |
basic_string& operator+=( std::initializer_list ilist ); | 后附 initializer_list ilist 中的字符。 |
#include<iostream>
#include<string>
using namespace std;
int main()
{
string s1 = "hello world";
string s2 = "is";
s1.append(2,'a');
cout << "s1:" << s1 << endl;
s1.append("This");
cout << "s1:" << s1 << endl;
s1 += s2;
cout << "s1:" << s1 << endl;
s1 += 'a';
cout << "s1:" << s1 << endl;
return 0;
}
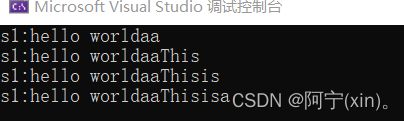
字典比较函数
比较函数 | 功能 |
---|
int compare( const basic_string& str ) const; | 比较此 string 与 str 。 |
int compare( size_type pos1, size_type count1, const basic_string& str ) const; | 比较此 string 的 [pos1, pos1+count1) 子串与 str 。若 count1 > size() - pos1 则子串为 [pos1, size()) 。 |
int compare( size_type pos1, size_type count1, const basic_string& str, size_type pos2, size_type count2 ) const; | 比较此 string 的 [pos1, pos1+count1) 子串与 str 的子串 [pos2, pos2+count2) 。若 count1 > size() - pos1 则第一子串为 [pos1, size()) 。若 count2 > str.size() - pos2 则第二子串为 [pos2, str.size()) 。 |
int compare( const CharT* s ) const; | 比较此 string 与始于 s 所指向字符的长度为 Traits::length(s) 的空终止字符序列。 |
int compare( size_type pos1, size_type count1, const CharT* s ) const; | 比较此 string 的 [pos1, pos1+count1) 子串与始于 s 所指向字符的长度为 Traits::length(s) 的空终止字符序列。若 count1 > size() - pos1 则子串为 [pos1, size()) 。 |
int compare( size_type pos1, size_type count1, const CharT* s, size_type count2 ) const; | 比较此 string 的 [pos1, pos1+count1) 子串与范围 [s, s + count2) 中的字符。(注意:范围 [s, s + count2) 中的字符可包含空字符。) |
compare结果描述

#include<iostream>
#include<string>
using namespace std;
int main()
{
string s1 = "hello world";
string s2 = "he";
string s3 = "hello nihao";
cout << s1.compare(s2) << endl;
cout << s1.compare(0, 3, s3) << endl;
return 0;
}

前缀后缀(C++20)
前缀、后缀函数 | 功能 |
---|
bool starts_with( std::basic_string_view<CharT,Traits> sv ) const noexcept; | 检查 string 是否始于给定前缀。 |
bool starts_with( CharT c ) const ; | 检查 string 是否始于给定前缀。 |
bool starts_with( const CharT* s ) const; | 检查 string 是否始于给定前缀。 |
constexpr bool ends_with( std::basic_string_view<CharT,Traits> sv ) const noexcept; | 检查 string 是否终于给定后缀 |
bool ends_with( CharT c ) const ; | 检查 string 是否终于给定后缀 |
bool ends_with( const CharT* s ) const; | 检查 string 是否终于给定后缀 |
返回值
若 string 终于给定后缀则为 true ,否则为 false 。
##加粗样式 replace,substr,copy,resize,swap函数(只列出部分)
函数名称 | 功能 |
---|
basic_string& replace( size_type pos, size_type count, const basic_string& str ); | 以新字符串替换 [pos, pos + count) 所指示的 string 部分。 |
basic_string& replace( const_iterator first, const_iterator last,const basic_string& str ); | 以新字符串替换[first, last) 所指示的 string 部分。 |
basic_string substr( size_type pos = 0, size_type count = npos ) const; | 返回子串 [pos, pos+count) 。若请求的字串越过字符串的结尾,即 count 大于 size() - pos (例如若 count == npos ),则返回的子串为 [pos, size()) 。 |
size_type copy( CharT* dest, size_type count, size_type pos = 0 ) const; | 复制子串 [pos, pos+count) 到 dest 所指向的字符串。 |
void resize( size_type count ); | 重设 string 大小以含 count 个字符。 |
void resize( size_type count, CharT ch ); | 初始化新字符为 ch 。 |
void swap( basic_string& other ); | 交换 string 与 other 的内容。 |
#include<iostream>
#include<string>
using namespace std;
int main()
{
string s1 = "hello world";
string s2 = "This is";
cout << s1.replace(0, 3, "re") << endl;
cout << s1.substr(3, 7) << endl;
s1.resize(7);
cout << s1 << endl;
s1.swap(s2);
cout << s1 << endl;
return 0;
}
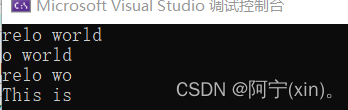
查找函数
查找函数 | 功能 |
---|
size_type find( const basic_string& str, size_type pos = 0 ) const; | 寻找等于 str 的首个子串。 |
size_type find( const CharT* s, size_type pos, size_type count ) const; | 寻找等于范围 [s, s+count) 的首个子串。此范围能含空字符。 |
size_type find( const CharT* s, size_type pos = 0 ) const; | 寻找等于 s 所指向的字符串的首个子串。 |
size_type find( CharT ch, size_type pos = 0 ) const; | 寻找首个字符 ch (由后述规则当作单字节子串)。 |
size_type rfind( const basic_string& str, size_type pos = npos ) const; | 寻找等于 str 的最后子串。 |
size_type rfind( const CharT* s, size_type pos, size_type count ) const; | 寻找等于范围 [s, s+count) 的最后子串。此范围能包含空字符。 |
size_type rfind( const CharT* s, size_type pos = npos ) const; | 寻找等于 s 所指向的字符串的最后子串。 |
size_type rfind( CharT ch, size_type pos = npos ) const; | 寻找等于 ch 的最后字符。 |
size_type find_first_of( const basic_string& str, size_type pos = 0 ) const; | 寻找等于 str 中字符之一的首个字符。 |
size_type find_first_of( const CharT* s, size_type pos, size_type count ) const; | 寻找等于范围 [s, s+count) 中字符中字符之一的首个字符。 |
size_type find_first_of( const CharT* s, size_type pos = 0 ) const; | size_type find_first_of( CharT ch, size_type pos = 0 ) const; |
寻找等于 s 所指向的字符串中字符之一的首个字符。 | 寻找等于 ch 的首个字符。 |
size_type find_first_not_of( const basic_string& str, size_type pos = 0 ) const; | 寻找不等于 str 中任何字符的首个字符。 |
size_type find_first_not_of( const CharT* s, size_type pos, size_type count ) const; | 寻找不等于范围 [s, s+count) 中任何字符的首个字符。此范围能包含空字符。 |
size_type find_first_not_of( const CharT* s, size_type pos = 0 ) const; | 寻找不等于 s所指向的字符串中任何字符的首个字符。 |
size_type find_first_not_of( CharT ch, size_type pos = 0 ) const; | 寻找不等于 ch 的首个字符。 |
size_type find_last_of( const basic_string& str, size_type pos = npos ) const; | |
寻找等于给定字符序列中字符之一的最后字符。str 中。若区间中不存在这种字符,则返回 npos 。 | |
size_type find_last_of( const CharT* s, size_type pos, size_type count ) const; | 寻找等于范围 [s, s+count) 中字符之一的最后字符。 |
size_type find_last_of( const CharT* s, size_type pos = npos ) const; | 寻找等于范围 [s, s+count) 中字符之一的最后字符。 |
size_type find_last_of( CharT ch, size_type pos = npos ) const; | 寻找等于范围 [s, s+count) 中字符之一的最后字符。 |
size_type find_last_not_of( const basic_string& str, size_type pos = npos ) const; | 寻找不等于 str 中任何字符的最后字符。 |
size_type find_last_not_of( const CharT* s, size_type pos, size_type count ) const; | 寻找不等于范围 [s, s+count) 中任何字符的最后字符。 |
size_type find_last_not_of( const CharT* s, size_type pos = npos ) const; | 寻找不等于 s 所指向的字符串中任何字符的最后字符。 |
size_type find_last_not_of( CharT ch, size_type pos = npos ) const; | 寻找不等于 ch 的最后字符。 |
#include<iostream>
#include<string>
using namespace std;
int main()
{
string s1 = "hello world";
cout << s1.find("llo") << endl;
cout << s1.find_first_of('l') << endl;
cout << s1.find_first_not_of('l') << endl;
return 0;
}

非成员函数
函数 | 功能 |
---|
operator+ | 连接两个字符串或者一个字符串和一个字符 |
operator<< | 执行字符串的流输入与输出 |
operator>> | 执行字符串的流输入与输出 |
template< class CharT, class Traits, class Allocator > | |
std::basic_istream<CharT,Traits>& getline( std::basic_istream<CharT,Traits>& input, std::basic_string<CharT,Traits,Allocator>& str, CharT delim ); | getline 从输入流读取字符并将它们放进 string |
#include<iostream>
#include<string>
using namespace std;
int main()
{
string s1 ;
cout << s1 << endl;
getline(std::cin, s1);
cout << s1 << endl;
return 0;
}
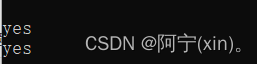
数值转换
str - 要转换的字符串
pos - 存储已处理字符数的整数的地址
base - 数的底
数值转换 | 功能 |
---|
int stoi( const std::string& str, std::size_t* pos = 0, int base = 10 ); | 转换字符串为有符号整数 |
long stol( const std::string& str, std::size_t* pos = 0, int base = 10 ); | 转换字符串为有符号整数 |
long long stoll( const std::string& str, std::size_t* pos = 0, int base = 10 ); | 转换字符串为有符号整数 |
unsigned long stoul( const std::string& str, std::size_t* pos = 0, int base = 10 ); | 转换字符串为无符号整数 |
unsigned long long stoull( const std::string& str, std::size_t* pos = 0, int base = 10 ); | 转换字符串为无符号整数 |
float stof( const std::string& str, std::size_t* pos = 0 ); | 转换字符串为浮点值 |
double stod( const std::string& str, std::size_t* pos = 0 ); | 转换字符串为浮点值 |
long double stold( const std::string& str, std::size_t* pos = 0 ); | 转换字符串为浮点值 |
std::string to_string( int value ); | 把有符号十进制整数转换为字符串,与 std::sprintf(buf, “%d”, value) 在有足够大的 buf 时产生的内容相同。(也可以转换为float等,替换关键字即可) |
std::wstring to_wstring( int value ); | 转换有符号十进制整数为宽字符串,其内容与 std::swprintf(buf, sz, L"%d", value) 对于充分大的 buf 将会生成的内容相同。(也可以转换float等,替换关键字即可) |
#include<iostream>
#include<string>
using namespace std;
int main()
{
string s1 = "123" ;
string s2 = "123.33";
string s3 = "127a with ";
float a = 5.6789;
cout << stoi(s1) << endl;
cout << stof(s2) << endl;
cout << stoi(s3) << endl;
cout << to_string(a) << endl;
return 0;
}
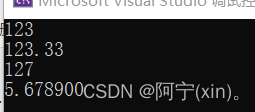
string仿写(见下篇)
END!