1 使用步骤
- 从配置文件中读取类字符串,配置文件可以是properties , 和xml
- 实例化类:使用
Class.forName(String className)
加载字符串类名, 使用 cls.newInstance()
进行类的实例化 - 获取方法对象:使用
Class.getMethod(String methodName)
获取方法对象 - 使用方法对象的
invoke(Object o)
函数,传入实例化的对象,实现反射
2 代码
- 从配置文件中读取 字符串配置
InputStream re = new FileInputStream("Chapter23_reflection/src/re.properties");
Properties properties = new Properties();
properties.load(re);
String classPath = properties.get("class_path").toString();
String menthodName= properties.get("method").toString();
System.out.println(classPath + ", " + menthodName);
- 实例化类
Class cls = Class.forName(classPath);
Object o = cls.newInstance();
- 获取方法对象,实现反射
Method method = cls.getMethod(menthodName);
method.invoke(o);
3 全部代码
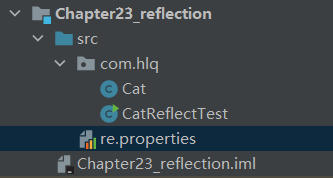
- JavaBean
public class Cat {
private String name;
public Cat(String name) {
this.name = name;
}
public Cat() {
this.name = "Tom";
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public void hi() {
System.out.println(name + "say: \'Hi\'");
}
public void cry() {
System.out.println(name + ", 喵~");
}
}
- 配置文件
class_path=com.hlq.Cat
method=cry
- 使用反射
public class CatReflectTest {
public static void main(String[] args) throws IOException, ClassNotFoundException, IllegalAccessException,
InstantiationException, NoSuchMethodException, InvocationTargetException {
InputStream re = new FileInputStream("Chapter23_reflection/src/re.properties");
Properties properties = new Properties();
properties.load(re);
String classPath = properties.get("class_path").toString();
String menthodName= properties.get("method").toString();
System.out.println(classPath + ", " + menthodName);
Class cls = Class.forName(classPath);
Object o = cls.newInstance();
if (o instanceof Cat) {
((Cat)o).setName("Tomm");
}
Method method = cls.getMethod(menthodName);
method.invoke(o);
}
}