数据结构》树(3)树 完整源码
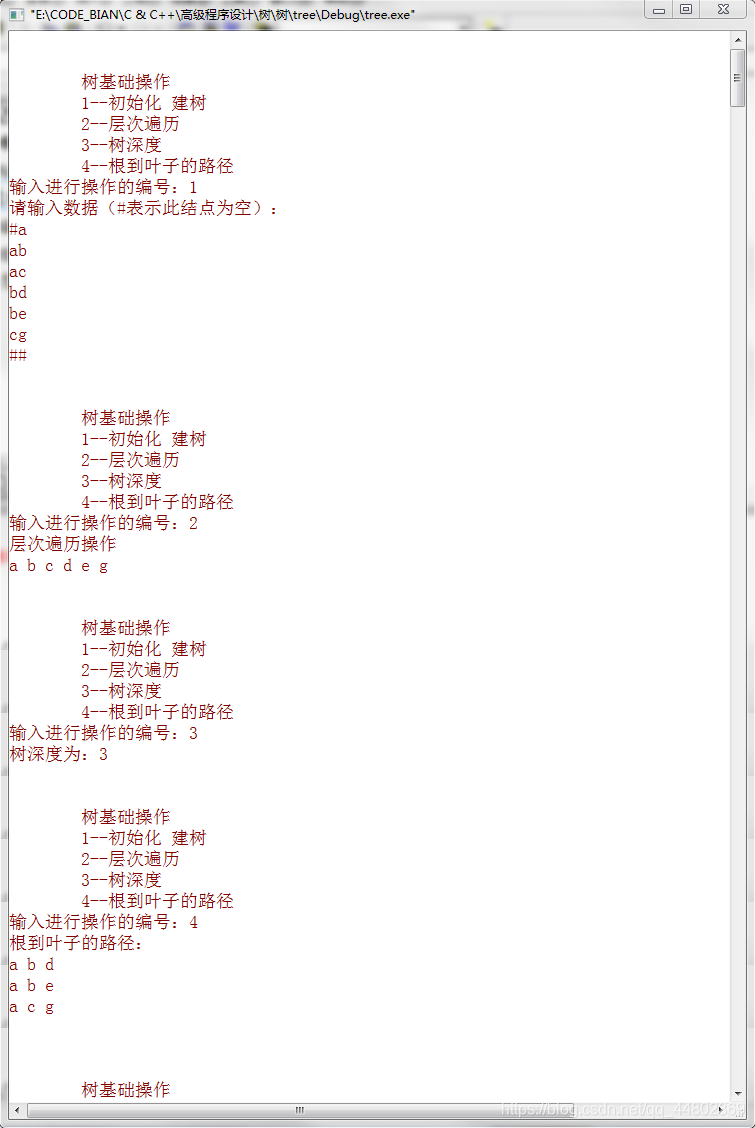
#include <iostream>
#include <windows.h>
using namespace std;
#define MAXSIZE 100
typedef char ElemType;
typedef struct csnode{
ElemType data;
struct csnode *firstchild;
struct csnode *nextsibling;
}CSNode,*CSTree;
typedef struct squeue{
CSTree *base;
int front;
int rear;
}SqQueue;
typedef struct sqstack{
CSTree *base;
CSTree *top;
int stack_size;
}SqStack;
bool init_queue(SqQueue &q);
bool en_queue(SqQueue &q,CSTree e);
bool de_queue(SqQueue &q,CSTree &e);
bool judge_empty(SqQueue q);
bool init_stack(SqStack &s);
bool push(SqStack &s,CSTree e);
bool pop(SqStack &s,CSTree &e);
bool judge_empty(SqStack s);
bool get_top(SqStack s,CSTree &e);
bool print_stack(SqStack s);
bool create_tree(CSTree &t);
bool layer_order(CSTree t);
int tree_depth(CSTree t);
void all_path(CSTree t,SqStack &s);
void show();
void switch_channel(int channel);
CSTree t;
SqStack s;
int main()
{
int channel;
do{
show();
cout<<"输入进行操作的编号:";
cin>>channel;
switch_channel(channel);
}while(1);
return 0;
}
void show()
{
cout<<endl<<endl<<"\t树基础操作\n";
cout<<"\t1--初始化 建树\n";
cout<<"\t2--层次遍历\n";
cout<<"\t3--树深度\n";
cout<<"\t4--根到叶子的路径\n";
}
void switch_channel(int channel)
{
switch(channel)
{
case 1:
{
cout<<"请输入数据(#表示此结点为空):\n";
create_tree(t);
}break;
case 2:
{ cout<<"层次遍历操作\n";
layer_order(t);
cout<<endl;
}break;
case 3:
{
cout<<"树深度为:";
cout<<tree_depth(t);
cout<<endl;
}break;
case 4:
{
cout<<"根到叶子的路径:\n";
all_path(t,s);
cout<<endl;
}break;
default:exit(1);break;
}
}
bool create_tree(CSTree &t)
{
SqQueue q;
init_queue(q);
ElemType fa,ch;
CSTree p;
CSTree s,r;
t=NULL;
for(cin>>fa>>ch;ch!='#';cin>>fa>>ch)
{
p = new CSNode;
p->data = ch;
p->firstchild = NULL;
p->nextsibling = NULL;
en_queue(q,p);
if(fa == '#')
t = p;
else
{
s = q.base[q.front];
while(s->data != fa)
{
de_queue(q,s);
s = q.base[q.front];
}
if(!(s->firstchild))
{
s->firstchild = p;
r = p;
}
else
{
r->nextsibling = p;
r = p;
}
}
}
return true;
}
bool layer_order(CSTree t)
{
SqQueue q;
init_queue(q);
CSTree p1,p2;
if(t)
en_queue(q,t);
while(!judge_empty(q))
{
de_queue(q,p1);
cout<<p1->data<<" ";
for(p2=p1->firstchild;p2;p2=p2->nextsibling)
if(p2)
en_queue(q,p2);
}
return true;
}
int tree_depth(CSTree t)
{
if(!t)
return 0;
int hight_c = tree_depth(t->firstchild);
int hight_s = tree_depth(t->nextsibling);
return (hight_c + 1 > hight_s ? hight_c + 1 : hight_s);
}
void all_path(CSTree t,SqStack &s)
{
CSTree p;
while(t)
{
push(s,t);
if(!t->firstchild) print_stack(s);
else all_path(t->firstchild,s);
pop(s,p);
t = t->nextsibling;
}
}
bool init_queue(SqQueue &q)
{
q.base = new CSTree[MAXSIZE];
if(!q.base)
{
cout<<"分配空间失败"<<endl;
exit(-1);
}
q.front=q.rear=0;
return true;
}
bool en_queue(SqQueue &q,CSTree e)
{
if((q.rear + 1) % MAXSIZE == q.front)
{
cout<<"队列已满,入队失败"<<endl;
return false;
}
q.base[q.rear] = e;
q.rear = (q.rear + 1) % MAXSIZE;
return true;
}
bool de_queue(SqQueue &q,CSTree &e)
{
if(judge_empty(q))
return false;
e = q.base[q.front];
q.front = (q.front + 1) % MAXSIZE;
return true;
}
bool judge_empty(SqQueue q)
{
if(q.front == q.rear)
return true;
return false;
}
bool init_stack(SqStack &s)
{
s.base = new CSTree[MAXSIZE];
if(!s.base)
{
cout<<"分配空间失败"<<endl;
exit(-1);
}
s.top=s.base;
s.stack_size = MAXSIZE;
return true;
}
bool push(SqStack &s,CSTree e)
{
if(s.top - s.base >= s.stack_size)
{
s.base = (CSTree *)realloc(s.base,(s.stack_size+MAXSIZE)*sizeof(CSTree));
if(!s.base)
{
cout<<"分配空间失败"<<endl;
exit(-1);
}
s.top = s.base + s.stack_size;
s.stack_size = s.stack_size + MAXSIZE;
}
*s.top = e;
s.top++;
return true;
}
bool pop(SqStack &s,CSTree &e)
{
if(judge_empty(s))
return false;
s.top--;
e = *s.top;
return true;
}
bool judge_empty(SqStack s)
{
if(s.top == s.base)
return true;
return false;
}
bool get_top(SqStack s,CSTree &e)
{
if(judge_empty(s))
return false;
e = *(s.top - 1);
return true;
}
bool print_stack(SqStack s)
{
if(judge_empty(s))
return false;
CSTree *p;
p = s.base;
while(p != s.top)
{
cout<<(*p)->data<<" ";
p++;
}
cout<<endl;
return true;
}