UI展示
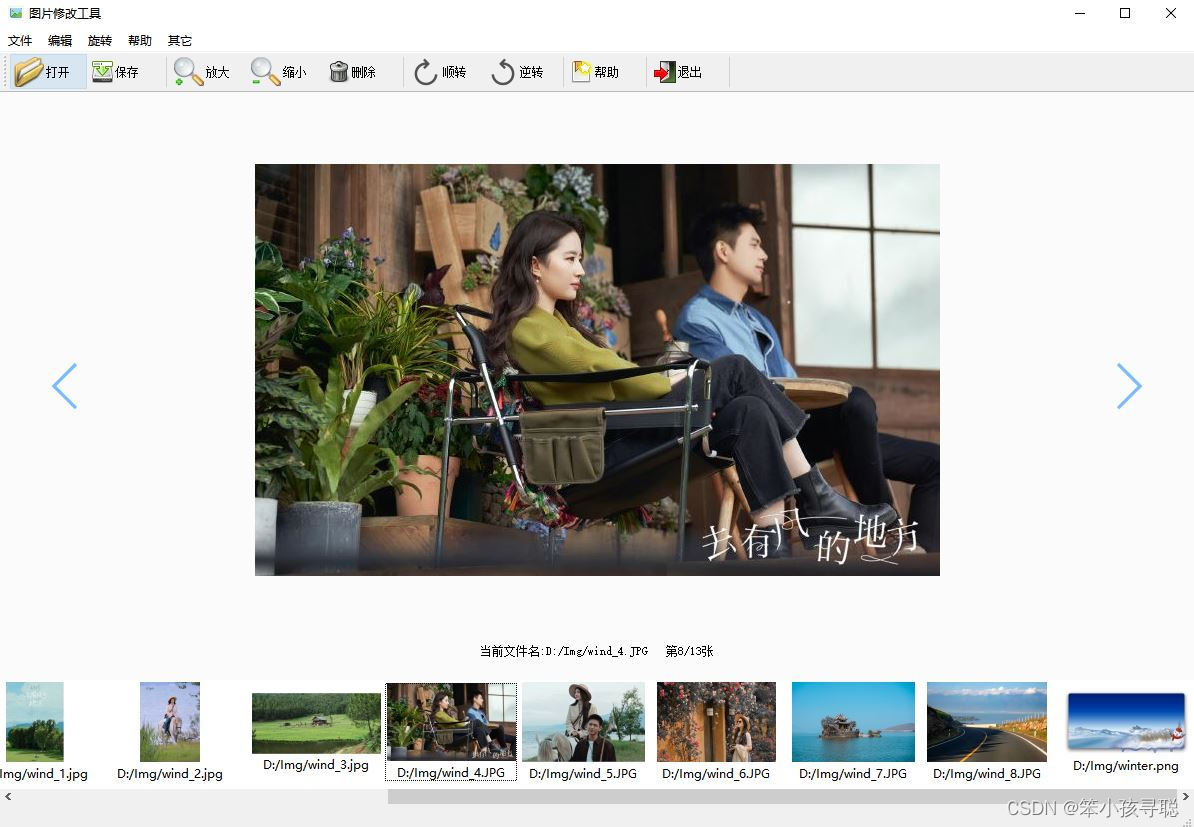
功能概述
一、图片导入:图片导入方式分为两种,QAction打开导入与拖拽导入;追加导入时界面新追加打开列表中的首张图片;
二、信息显示:导入图片列表后,页面中心的label中默认显示导入列表中的第一张图片和其全路径信息以及它在导入的所有图片中的位置序号信息,页面底部的listWidget中显示导入的所有图片的缩略图及其全路径名称;
三、图片操作:
1、切换操作:图片切换方式分两种,点击上一张下一张按钮切换与点击底部列表中条目进行切换,切换后,将在页面的label中显示图片及其相关信息;
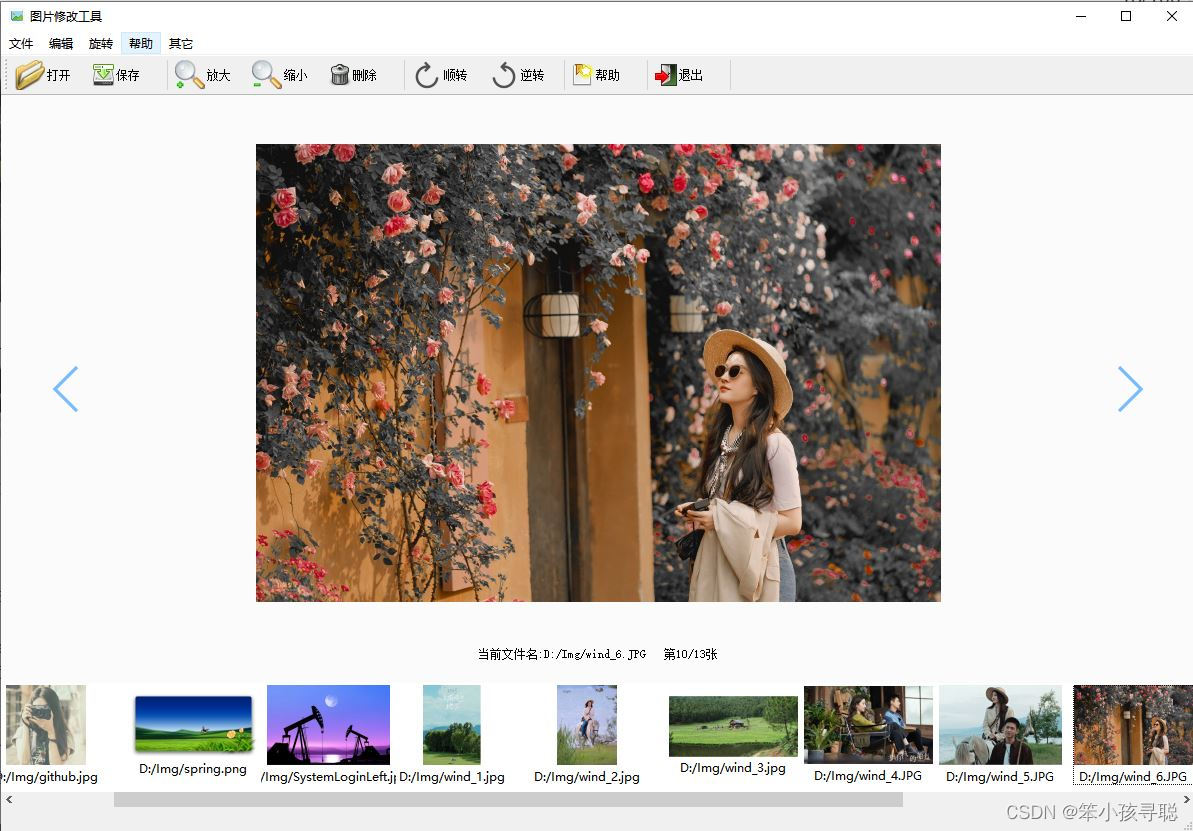
2、缩放操作:点击工具栏或菜单栏下拉列表中对应的QAction,使用Qt中QTransform进行缩放操作,缩放时模糊度较大,质量较差;
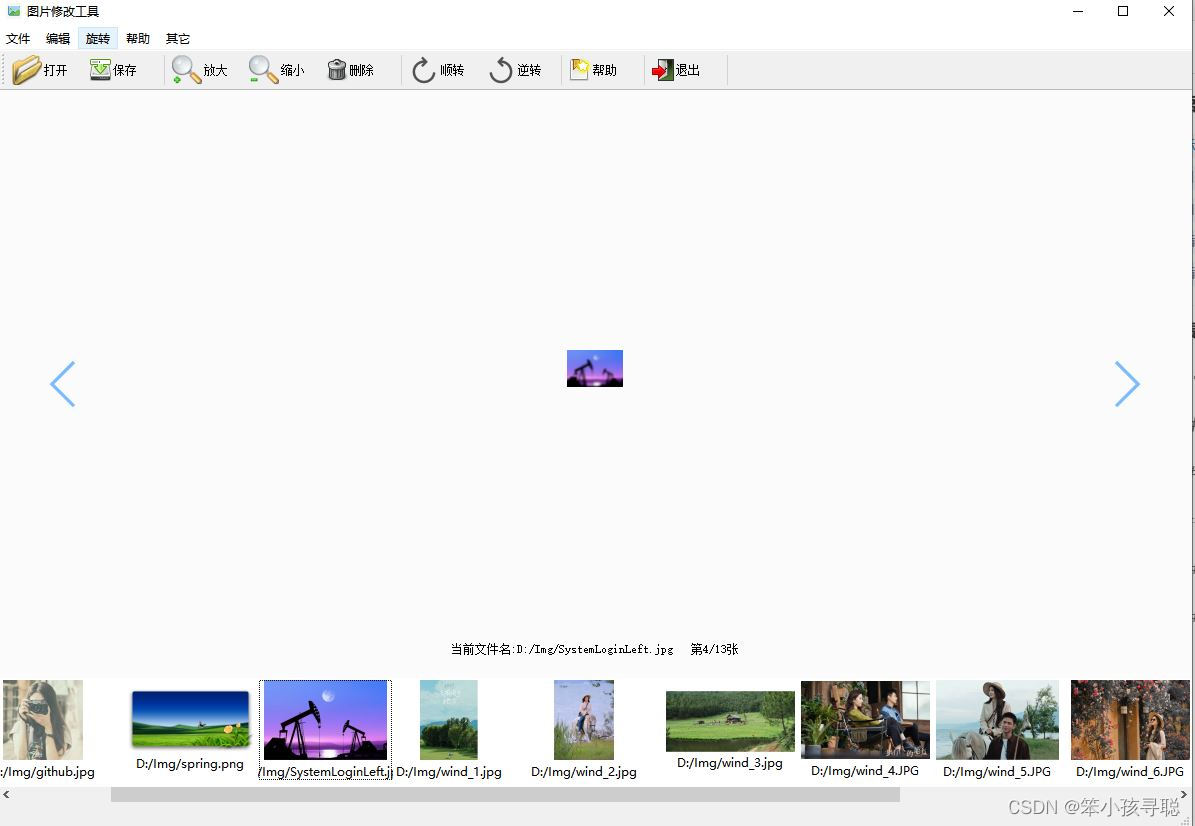
3、旋转操作:点击工具栏或菜单栏下拉列表中对应的QAction,默认的顺转与逆转均为90度;
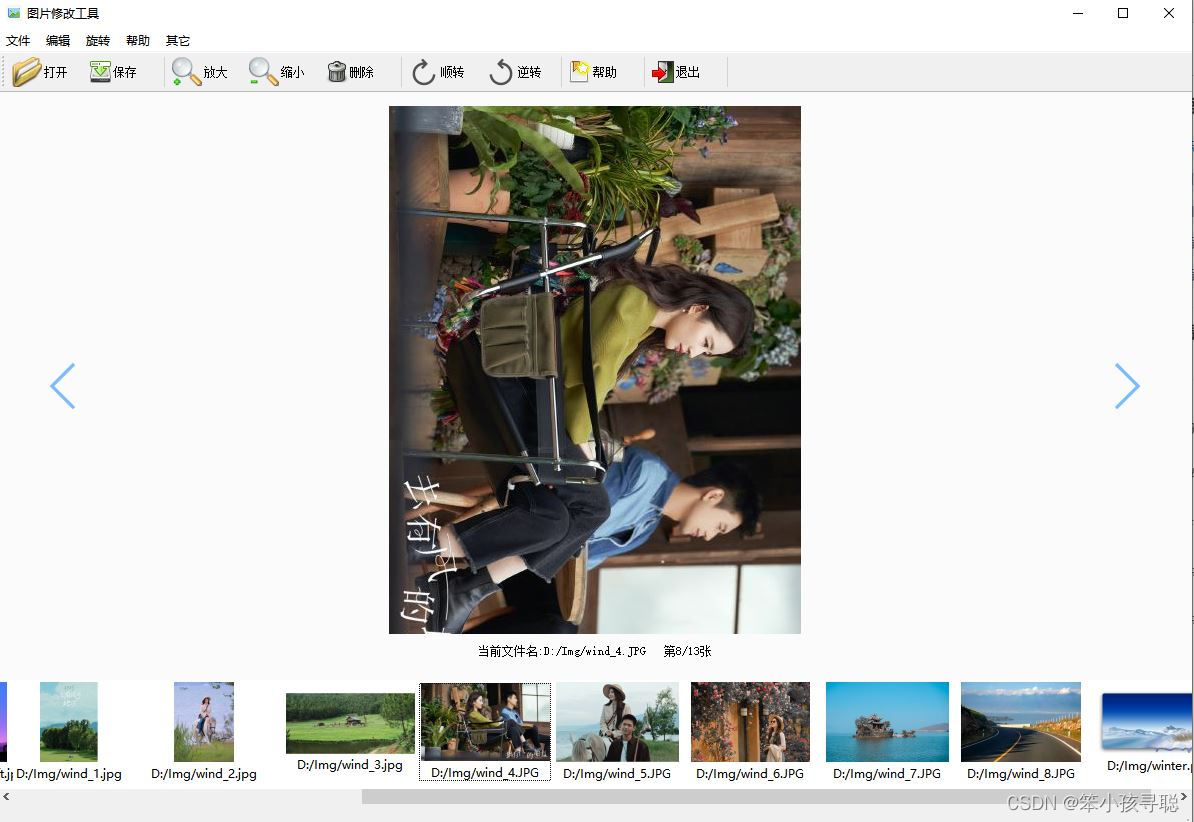
4、其它说明:保存与帮助按钮仅放置在工具条,未有任何实现,退出按钮实现退出程序;
程序源码:
头文件
#ifndef PICOPERATE_H
#define PICOPERATE_H
#include <QMainWindow>
#include <QStringList>
#include <QImage>
#include <QPixmap>
#include <QMimeData>
#include <QDropEvent>
#include <QDragEnterEvent>
#include <QWheelEvent>
#include <QUrl>
namespace Ui {
class PicOperate;
}
class PicOperate : public QMainWindow
{
Q_OBJECT
public:
explicit PicOperate(QWidget *parent = 0);
~PicOperate();
void connectionSlots();
void laodLabelPic(QString fillAllPath);
void adaptPicLabelShow(QImage img);
void loadSwitchPic(int index);
void pinInfoShow(QString picRoad, int curIndex, int totalNum = 0);
void tipInformation(QString tipStr);
protected:
void dragEnterEvent(QDragEnterEvent *event);
void dropEvent(QDropEvent *dropedEvent);
void wheelEvent(QWheelEvent *event);
private slots:
void listWidgetRowChanged(int currentRow);
void openFileTriggered();
void deletePicTriggered();
void zoomInTriggered();
void zoomOutTriggered();
void upPicToolBtnClicked();
void downPicToolBtnClicked();
void leftRotateTriggered();
void rightRotateTriggered();
private:
QString picFileRoad;
QStringList fullPathOfFiles;
int picNumber;
bool fileDirOpen;
QImage zoomImg;
int picIndex;
int picImportIndex = 0;
private:
Ui::PicOperate *ui;
};
#endif
源文件
#include "picoperate.h"
#include "ui_picoperate.h"
#include <QFileDialog>
#include <QListWidget>
#include <QPushButton>
#include <QMessageBox>
#include <QTransform>
#include <QList>
#include <QAction>
#include <QDir>
#include <QDebug>
#define ISNULL(IMG) if(IMG.isNull()){return;}
constexpr int rotateAngle = 90;
PicOperate::PicOperate(QWidget *parent) :
QMainWindow(parent)
, picNumber(0)
, fileDirOpen(false)
, picIndex(0)
, ui(new Ui::PicOperate)
{
ui->setupUi(this);
this->setWindowTitle(tr("图片修改工具"));
this->setAcceptDrops(true);
connectionSlots();
}
PicOperate::~PicOperate()
{
delete ui;
}
void PicOperate::connectionSlots()
{
connect(ui->action_OpenFile, &QAction::triggered, this, &PicOperate::openFileTriggered);
connect(ui->action_ZoomIn, &QAction::triggered, this, &PicOperate::zoomInTriggered);
connect(ui->action_ZoomOut, &QAction::triggered, this, &PicOperate::zoomOutTriggered);
connect(ui->action_Delete, &QAction::triggered, this, &PicOperate::deletePicTriggered);
connect(ui->action_LeftRotate, &QAction::triggered, this, &PicOperate::leftRotateTriggered);
connect(ui->action_RightRotate, &QAction::triggered, this, &PicOperate::rightRotateTriggered);
connect(ui->action_Exit, &QAction::triggered, this, []{exit(0);});
connect(ui->upPicToolButton, &QPushButton::clicked, this, &PicOperate::upPicToolBtnClicked);
connect(ui->downPicToolButton, &QPushButton::clicked, this, &PicOperate::downPicToolBtnClicked);
connect(ui->PiclistWidget, &QListWidget::currentRowChanged, this, &PicOperate::listWidgetRowChanged);
}
void PicOperate::laodLabelPic(QString fillAllPath)
{
QImage imgTemp;
fileDirOpen = true;
imgTemp.load(fillAllPath);
zoomImg.load(fillAllPath);
ISNULL(imgTemp)
adaptPicLabelShow(imgTemp);
pinInfoShow(fillAllPath, picImportIndex, picNumber);
picImportIndex = picNumber;
}
void PicOperate::adaptPicLabelShow(QImage img)
{
int fitWidth = img.width() <= ui->PicShowLabel->width() ? img.width() : ui->PicShowLabel->width();
int fitHeight = img.height() <= ui->PicShowLabel->height() ? img.height() : ui->PicShowLabel->height();
ui->PicShowLabel->setPixmap(QPixmap::fromImage(img.scaled(fitWidth, fitHeight)));
}
void PicOperate::loadSwitchPic(int index)
{
QImage img;
QString picName = fullPathOfFiles.at(index);
zoomImg.load(picName);
img.load(picName);
ISNULL(img)
adaptPicLabelShow(img);
pinInfoShow(picName, index, picNumber);
ui->PiclistWidget->setCurrentRow(index);
}
void PicOperate::pinInfoShow(QString picRoad, int curIndex, int totalNum)
{
QString PicInfoLabel = "当前文件名:" + picRoad;
PicInfoLabel = PicInfoLabel + " " + "第" + QString::number(curIndex + 1) + "/" + QString::number(totalNum) + "张";
ui->picInfolabel->setText(PicInfoLabel);
}
void PicOperate::tipInformation(QString tipStr)
{
QMessageBox::warning(this,tr("文件提示:"), tipStr, QMessageBox::Ok);
}
void PicOperate::dragEnterEvent(QDragEnterEvent *event)
{
event->mimeData()->hasUrls() ? event->acceptProposedAction()
: event->ignore();
}
void PicOperate::dropEvent(QDropEvent *dropedEvent)
{
if (dropedEvent->mimeData()->hasUrls()) {
QList<QUrl> urlList = dropedEvent->mimeData()->urls();
for (int i = 0; i < urlList.size(); i++) {
QString curFile = urlList.at(i).toLocalFile();
picNumber == 0 ? fullPathOfFiles.insert(i, curFile)
: fullPathOfFiles.insert(picNumber + i, curFile);
QListWidgetItem *signalPicItem = new QListWidgetItem(QIcon(curFile), curFile);
ui->PiclistWidget->addItem(signalPicItem);
}
ui->PiclistWidget->setCurrentRow(picNumber);
picNumber += urlList.size();
laodLabelPic(urlList.at(0).toLocalFile());
}
}
void PicOperate::wheelEvent(QWheelEvent *event)
{
if (QApplication::keyboardModifiers() == Qt::ControlModifier) {
if (event->angleDelta().y() > 0) {
zoomInTriggered();
} else {
zoomOutTriggered();
}
}
}
void PicOperate::openFileTriggered()
{
picFileRoad = QFileDialog::getExistingDirectory(this, "Open Dir", "../");
if (picFileRoad.isEmpty()) {
return;
}
QDir openPicDir(picFileRoad);
QStringList filter;
filter<<"*.jpg"<<"*.png"<<"*.bmp"<<"*.jpeg"<<"*.ppm"<<
"*.JPG"<<"*.PNG"<<"*.JPEG";
openPicDir.setNameFilters(filter);
QStringList picFileList = openPicDir.entryList(filter);
for(int i = 0; i < picFileList.size(); i++) {
QString picFullDir = picFileRoad + "/" + picFileList.at(i);
picNumber == 0 ? fullPathOfFiles.insert(i, picFullDir)
: fullPathOfFiles.insert(picNumber + i, picFullDir);
QListWidgetItem *signalPicItem=new QListWidgetItem(QIcon(picFullDir), picFullDir);
ui->PiclistWidget->addItem(signalPicItem);
}
ui->PiclistWidget->setCurrentRow(picNumber);
picNumber += picFileList.size();
laodLabelPic(fullPathOfFiles.at(0));
}
void PicOperate::deletePicTriggered()
{
int curIndex = ui->PiclistWidget->currentRow();
if (curIndex < 0) {
fileDirOpen = false;
return;
}
fullPathOfFiles.removeAt(curIndex);
ui->PiclistWidget->takeItem(curIndex);
picNumber = fullPathOfFiles.size();
picImportIndex = picNumber;
if (picNumber > 0) {
QString str = ui->PiclistWidget->currentItem()->text();
pinInfoShow(str, ui->PiclistWidget->currentRow(), picNumber);
} else {
ui->PicShowLabel->setPixmap(QPixmap(":/new/images/labelBg.jpg"));
ui->picInfolabel->clear();
return;
}
}
void PicOperate::listWidgetRowChanged(int currentRow)
{
if (currentRow == -1) {
return;
}
QImage currentImg;
QString currentPic = ui->PiclistWidget->item(currentRow)->text();
zoomImg.load(currentPic);
currentImg.load(currentPic);
ISNULL(currentImg)
adaptPicLabelShow(currentImg);
pinInfoShow(currentPic, currentRow, picNumber);
}
void PicOperate::zoomInTriggered()
{
ISNULL(zoomImg)
QTransform zoomInTransform;
zoomInTransform.scale(1.1,1.1);
zoomImg = zoomImg.transformed(zoomInTransform, Qt::SmoothTransformation);
ui->PicShowLabel->setPixmap(QPixmap::fromImage(zoomImg));
}
void PicOperate::zoomOutTriggered()
{
ISNULL(zoomImg)
QTransform zoomOutTransform;
zoomOutTransform.scale(0.9, 0.9);
zoomImg = zoomImg.transformed(zoomOutTransform, Qt::SmoothTransformation);
ui->PicShowLabel->setPixmap(QPixmap::fromImage(zoomImg));
}
void PicOperate::upPicToolBtnClicked()
{
if (false == fileDirOpen) {
tipInformation("请先选择您需要打开的图片文件");
return;
}
picIndex = ui->PiclistWidget->currentRow();
--picIndex;
if (picIndex < 0) {
tipInformation("您当前浏览的是列表中第一张图片,将从列表中最后一张重新开始");
picIndex = fullPathOfFiles.size() - 1;
}
loadSwitchPic(picIndex);
}
void PicOperate::downPicToolBtnClicked()
{
if (false == fileDirOpen) {
tipInformation("请先选择您需要打开的图片文件");
return;
}
picIndex = ui->PiclistWidget->currentRow();
picIndex++;
if (picIndex > fullPathOfFiles.size() - 1) {
tipInformation("已浏览至最后一张图片,再次点击将从第一张重新开始");
picIndex = 0;
}
loadSwitchPic(picIndex);
}
void PicOperate::leftRotateTriggered()
{
ISNULL(zoomImg)
QTransform leftTransform;
leftTransform.rotate(-rotateAngle);
zoomImg = zoomImg.transformed(leftTransform, Qt::SmoothTransformation);
ui->PicShowLabel->setPixmap(QPixmap::fromImage(zoomImg));
}
void PicOperate::rightRotateTriggered()
{
ISNULL(zoomImg)
QTransform rightTransform;
rightTransform.rotate(rotateAngle);
zoomImg = zoomImg.transformed(rightTransform, Qt::SmoothTransformation);
ui->PicShowLabel->setPixmap(QPixmap::fromImage(zoomImg));
}
完整版代码及图片资源链接:https://download.csdn.net/download/qq_44896246/87377757