python
前提准备
- 安装好python及其环境
- 安装好Oracle数据库
- python中安装好cx_Oracle包,且能与数据库正常联立交互
注: 前提准备部分的内容不做描述,百度均有教程
步骤:
第一步:在oracle中创建以下表:
下面展示 建表语句
。
--ID_FLAG表是用以记录单词进度
create table ID_FLAG
(
id_value NUMBER(5),
insert_time VARCHAR2(50)
)
-- EXAM_FUN2表用以记录模块2中测验的结果
create table EXAM_FUN2
(
id NUMBER(5),
word VARCHAR2(100),
chinese_value VARCHAR2(1024),
insert_time VARCHAR2(50),
isright VARCHAR2(10)
)
-- ALL_WORDS表是单词的记录储存表
create table ALL_WORDS
(
id NUMBER(5) not null,
word VARCHAR2(100),
chinese_value VARCHAR2(1024)
)
--
-- 这是用以储存自已写的例句表
create table EXAMPLE_SENTENCE_TABLE
(
id_scope VARCHAR2(10),
example_sentence VARCHAR2(500),
insert_time VARCHAR2(50)
)
第二步:运行脚本向数据库中导入文件里的单词数据
下面这个是单词的txt文件:
链接: https://pan.baidu.com/s/1Ga2sHQJrpg3LGvLDt_i4vQ?pwd=1234
这是导入数据库的脚本代码:
注:代码中的数据库账号密码端口以及文件路径等信息需要自行修改。
import cx_Oracle as cx
def condba():
global conn
username = 'briup' #数据库的账号
password = 'briup' #数据库的密码
host = '127.0.0.1:1521/XE'
conn = cx.connect(username,password,host)
cursor = conn.cursor()
return cursor
def run(con):
'''
准备写一个方法可以读取指定文件夹中的文件,
并将文件名和指定数放在两个list中
'''
#============拆分文件模块开始==============
#print('=======进入run方法========')
#print(content)
list_id=[]
list_word=[]
list_chineseValue=''
strlist = con.split()
#print(strlist)
list_id = strlist[0]
list_word = strlist[1]
list_len = len(strlist)
#print(list_len)
if list_len == 3:
list_chineseValue = strlist[2]
else:
i = 2
temp_str=''
while i < list_len:
temp_str = temp_str+str(strlist[i])
i = i+1
#print(temp_str)
list_chineseValue=temp_str
#print('==========拆分结束=========')
# #========已将文件拆到三个列表中========
# #============拆分文件模块结束==============
return list_id,list_word,list_chineseValue
if __name__ == '__main__':
try:
print('==========开始插入数据=========')
dba = condba()
filepath = r'D:\记得修改路径\1.txt'
fp = open(filepath,'r',encoding='UTF-8')
#读取文件内容
content = fp.readlines()
#print(content)
delete_sql = "truncate table all_words"
dba.execute(delete_sql)
print('all_words原数据已删除')
for con in content:
id,word,cv = run(con)
insert_sql = str_temp = "insert into all_words values('%s','%s','%s')" % (id,word,cv)
#print(insert_sql)
dba.execute(insert_sql)
except Exception as e:
conn.rollback()
print(e)
finally:
conn.commit()
conn.close()
fp.close()
print('==========数据插入结束=========')
出现以下显示时表明数据插入完成。
注:其实若愿意的话,也可以将前面的建表语句放到后面的数据插入脚本中一次性执行,写个str字符串,将sql放进去后,用ora.execute(str)执行后再将其commit即可,此处没写进去是因为在plsql中用习惯了,方便修改。
数据插入完成后,便可以执行以下python脚本了
import cx_Oracle as cx
import random
def condba():
global conn
username = 'briup'
password = 'briup'
host = '127.0.0.1:1521/XE'
conn = cx.connect(username,password,host)
cursor = conn.cursor()
return cursor
'''
分4种模式:
1.第一种按顺序五个五个出现word和cv ,可以输入数字5尝试写例句用以加强记忆
2.第二种里共有三种测试方式,前两种只出现翻译,填写word,并进行检测是否匹配,第1中是每15个进行一次检测,第2种是从开头一直检测到已学部分,第3种是有单词有翻译,进行选择,所有检测的结果会记录在表exam_fun2中
3.第三种将所有false的进行再次复习与测验,1是进行复习,2是对二模式中测验错误的进行再次测验,若对了会更新记录
4.将复习的表进行重置 --保留表中id = 1的值,方便重置后方法的运行,一切重新开始
'''
def fun1(ora):
count = 1
print('您已选择第一种模式:')
id_sql = "select * from (select * from id_flag order by id_value desc) where rownum = 1"
ora.execute(id_sql)
result = ora.fetchall()
#print(result[0][0])
order = '0' #'0'继续学习,stop()结束学习
id = result[0][0] #读取数据库中表id_flag中最后一次记录的id值
while id <= 5384:
if(count == 4):
print('已满15个,是否进行一次测验? Y/N')
isCheck = input('请输入:')
while isCheck:
if isCheck == 'Y':
exam3(ora)
break
elif isCheck == 'N':
print('继续学习')
break
else:
print('请不要输入除了Y或N以外的内容:')
isCheck = input('请输入:')
count = 1
if order == 'stop()':
break
elif order == '5':
print('请写您的例句:',end='')
example_sentence = input()
id_str = '%d~%d' % (id-5,id)
ex_sql = "insert into example_sentence_table values('%s','%s',to_char(sysdate,'yyyymmdd hh24:mi:ss'))" % (id_str,example_sentence);
ora.execute(ex_sql)
conn.commit()
elif order == '0':
print('===========================分隔符===========================')
count = count + 1
strsql = "select word,chinese_value from all_words where id >= %d and id < %d" % (id,id+5)
#print(strsql)
ora.execute(strsql)
strsql_result = ora.fetchall()
for v in strsql_result:
#print(v[1])
a ="%s : %s" % (v[0],v[1])
print(a)
else:
print('请输入正确的指令!')
insertId_sql = "insert into id_flag values(%d,to_char(sysdate,'yyyymmdd hh24:mi:ss'))" % (id)
ora.execute(insertId_sql)
conn.commit() #每学习一次就要记录一次,方便方法2在测验的时候进行读取id范围
id = id+5
print('请输入指令:--stop()--退出,--5--自写例句,--0--下一个')
print('===========================分隔符===========================')
order = input('请输入:')
print('第一种模式结束')
#2.第二种只出现cv,填写word,并进行检测是否匹配,从id_flag表中读取id进行
def fun2(ora):
print('*******模块2测验开始*******')
print('**********分界线************')
print('请输入数字1对已学进行阶段测验')
print('请输入数字2对已学进行全面测验')
print('请输入数字3对已学进行新的测验')
print('**********分界线************')
print('请输入一个数字或输入quit退出:')
num = input('请输入:')
while num != 'quit':
if num == '1':
exam1(ora)
elif num == '2':
exam2(ora)
elif num == '3':
exam3(ora)
else :
print('输入有误!')
print('**********分界线************')
print('请输入数字1对已学进行阶段测验')
print('请输入数字2对已学进行全面测验')
print('请输入数字3对已学进行新的测验')
print('**********分界线************')
print('请输入一个数字或输入quit退出:')
num = input('请输入:')
print('*****阶模块2测验结束*****')
def exam1(ora):
print('*****阶段性测验开始*****')
str_sql = "select min(id_value),max(id_value) from (select * from id_flag order by id_value desc) where rownum < 5 order by 1" #读取记录表中的最后两条记录的id值
ora.execute(str_sql)
result = ora.fetchall()
#print(result)
id1 = result[0][0]
id2 = result[0][1]
str_sql2 = "select * from all_words where id >= %d and id <= %d" % (id1,id2+5)
#print(str_sql2)
ora.execute(str_sql2)
sql2_cv = ora.fetchall()
#print(sql2_cv)
for t in sql2_cv:
print(t[2])
print('请输入对应的word值:')
word = input('请输入:')
if(word == t[1]):
sql_check = "insert into exam_fun2 values(%d,'%s','%s',to_char(sysdate,'yyyymmdd hh24:mi:ss'),'true')" % (t[0],t[1],t[2])
print('correct!')
else:
sql_check = "insert into exam_fun2 values(%d,'%s','%s',to_char(sysdate,'yyyymmdd hh24:mi:ss'),'false')" % (t[0],t[1],t[2])
print('wrong! the correct answer is :'+ t[1])
#print(sql_check)
print('**********************分隔符*****************************')
ora.execute(sql_check)
conn.commit()
print('*****阶段性测验结束*****')
def exam2(ora):
print('*****整体测验开始*****')
str_sql2 = "select * from all_words where id <= (select max(id_value) from id_flag a)"
#print(str_sql2)
ora.execute(str_sql2)
sql2_cv = ora.fetchall()
#print(sql2_cv)
for t in sql2_cv:
print(t[2])
print('请输入对应的word值:')
word = input('请输入:')
if(word == t[1]):
sql_check = "insert into exam_fun2 values(%d,'%s','%s',to_char(sysdate,'yyyymmdd hh24:mi:ss'),'true')" % (t[0],t[1],t[2])
print('correct!')
else:
sql_check = "insert into exam_fun2 values(%d,'%s','%s',to_char(sysdate,'yyyymmdd hh24:mi:ss'),'false')" % (t[0],t[1],t[2])
print('wrong! the correct answer is :'+ t[1])
#print(sql_check)
print('**********************分隔符*****************************')
ora.execute(sql_check)
conn.commit()
print('*****整体测验结束*****')
def exam3(ora):
print('*****选汉语测验开始*****')
str_sql = "select min(id_value),max(id_value) from (select * from id_flag order by id_value desc) where rownum < 5 order by 1" #读取记录表中的最后两条记录的id值
ora.execute(str_sql)
result = ora.fetchall()
#print(result)
id1 = result[0][0]
id2 = result[0][1]
str_sql2 = "select * from all_words where id >= %d and id <= %d" % (id1,id2) #获取最后两个id值
#print(str_sql2)
ora.execute(str_sql2)
sql2_cv = ora.fetchall()
#print(sql2_cv)
value = {} #将单词和翻译放在字典中
value2 = [] #但翻译放在字典中并序号,方便下面抽取
for t in sql2_cv:
value[t[1]] = t[2] # 将这十几个里面抽取三个,与当前选择的值放在一起组成选项
value2.append(t[2])
#print(random.sample(value2, 3))
temp = []
new_temp = []
for t in sql2_cv:
temp = value2.copy() #将所有的翻译给复制到一个临时列表temp中
temp.remove(t[2]) #这临时列表中将当前的单词翻译删掉
new_temp = random.sample(temp,3) #在没有当前翻译的剩下值中随机筛选3个组成新的列表
new_temp.append(t[2]) #将当前单词的翻译添加进去
new_temp = random.sample(new_temp,len(new_temp)) #再将组好的这个新列表进行随机排列
print(t[1])
count = 1
for ft in new_temp:
print(count,end='')
print(' : ',end='')
print(ft)
count = count + 1
print('请从上列选项中选择对应的选项:')
num = input('请输入选项(1~4)或者quit退出:')
flag = 1
while flag == 1 and num != 'quit':
num = int(num) - 1
if num >= 0 and num <= 3 :
flag = 0
else:
print('您输入的值不在1~4之间,请重新输入!')
num = input('请输入选项(1~4)或者quit退出:')
#num = int(num) - 1
if num == 'quit':
break
elif(new_temp[num] == t[2]):
sql_check = "insert into exam_fun2 values(%d,'%s','%s',to_char(sysdate,'yyyymmdd hh24:mi:ss'),'true')" % (t[0],t[1],t[2])
print('correct!')
else:
sql_check = "insert into exam_fun2 values(%d,'%s','%s',to_char(sysdate,'yyyymmdd hh24:mi:ss'),'false')" % (t[0],t[1],t[2])
print('wrong! the correct answer is :')
print(t[2])
#print(sql_check)
print('**********************分隔符*****************************')
ora.execute(sql_check)
conn.commit()
print('*****选汉语测验结束*****')
def fun3(ora):
print('*****错重复习开始*****')
err_sql = " select count(*) \
from exam_fun2 a ,(select word,max(insert_time) as max_time from exam_fun2 group by word) b \
where a.word = b.word \
and a.insert_time = b.max_time \
and a.isright = 'false'\
order by insert_time desc "
ora.execute(err_sql)
err_count = ora.fetchall()
print('**********分界线************')
if err_count:
print('已积累错误个数:',end='')
print(err_count[0][0])
print('请输入数字1对错误进行再次复习')
print('请输入数字2对复习进行再次测验')
print('**********分界线************')
print('请输入选择或者quit退出:')
num = input('请输入:')
while num != 'quit':
if num == '1':
print('**********分界线************')
print('您输入的是1,开始对错误进行复习')
str_sql = " select a.* \
from exam_fun2 a ,(select word,max(insert_time) as max_time from exam_fun2 group by word) b \
where a.word = b.word \
and a.insert_time = b.max_time \
and a.isright = 'false'\
order by insert_time desc " #读取测验表中的测验错误的单词
ora.execute(str_sql)
result = ora.fetchall()
for v in result:
a ="%s : %s" % (v[1],v[2])
print(a)
print('**********分界线************')
elif num == '2':
print('**********分界线************')
print('您输入的是2,开始对错误进行检验')
str_sql = " select a.* \
from exam_fun2 a ,(select word,max(insert_time) as max_time from exam_fun2 group by word) b \
where a.word = b.word \
and a.insert_time = b.max_time \
and a.isright = 'false'\
order by insert_time desc "
#print(str_sql)
ora.execute(str_sql)
sql_cv = ora.fetchall()
#print(sql_cv)
for t in sql_cv:
print(t[2])
print('请输入对应的word值:')
word = input('请输入:')
if(word == t[1]):
sql_check = "insert into exam_fun2 values(%d,'%s','%s',to_char(sysdate,'yyyymmdd hh24:mi:ss'),'true')" % (t[0],t[1],t[2])
print('correct!')
else:
sql_check = "insert into exam_fun2 values(%d,'%s','%s',to_char(sysdate,'yyyymmdd hh24:mi:ss'),'false')" % (t[0],t[1],t[2])
print('wrong! the correct answer is :'+ t[1])
#print(sql_check)
print('**********分界线************')
ora.execute(sql_check)
conn.commit()
else:
print('输入有误,请重新输入!')
print('**********分界线************')
print('请输入数字1对错误进行再次复习')
print('请输入数字2对复习进行再次测验')
print('**********分界线************')
print('请输入数字选择或者quit退出:')
num = input('请输入:')
print('*****错重复习结束*****')
def fun4(ora):
delete_exam_fun2 = 'delete from exam_fun2 where id > 1'
delete_id_flag = 'delete from id_flag where id_value > 1'
ora.execute(delete_exam_fun2)
ora.execute(delete_id_flag)
conn.commit()
print('表中记录已重置')
if __name__ == '__main__':
ora = condba()
print("*****请输入指令1或者2或者3或者4,输入exit()退出:*****")
print('1. 开始学习单词')
print('2. 来一次小测验')
print('3. 复习一下上次测验的错误')
print('4. 记录重置')
print("********************分界线*********************")
print('请输入一个选项:')
str = input('请输入:')
while str != 'exit()':
#print(str)
if str == '1':
fun1(ora)
elif str == '2':
fun2(ora)
elif str == '3':
fun3(ora)
elif str == '4':
fun4(ora)
else:
print('您输入的选项无效,请重新输入!')
str = input('请输入:')
continue
print("*****请输入指令1或者2或者3或者4,输入exit()退出:*****")
str = input('请输入:')
print('bye')
conn.close()
这里一共写了四个小功能,分为1,2,3,4四个选项:
第一个功能是直接显示单词与翻译,每五个为一组,按0进行下一组,按5可以对当前的单词自写例句,例句会保存在表EXAMPLE_SENTENCE_TABLE中。满15个就进行一次小测验,结果无论对错都会将其记录在表exam_fun2中,方便后面进行复习和再检测,输入stop()进行退出第一种模式。
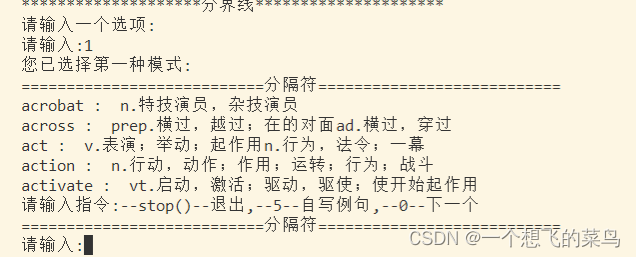
第二个功能是测验功能,分三种:
第一种其实就是在1模式中的小测验,第二种是从第一个单词一直到你所学的单词为止全部进行一次测验。这前两种都是根据翻译写单词,第三种是根据单词选择翻译,有四种选项,正确答案是其中的一个。
第三个功能是将前面测验错误的进行重新学习与测验,当重新测验正确后,再下次学习错误单词时便不会再计入内。
第四个功能是将所有学习过的记录,或者错误的记录全部清除掉,一切重新开始,因此便可以进行重复学习
自此便是全部的功能,后续还将添加阅读题,以及对应的题目讲解等功能。
注:本脚本的编写环境均由vscode所实现。