短信服务模块:
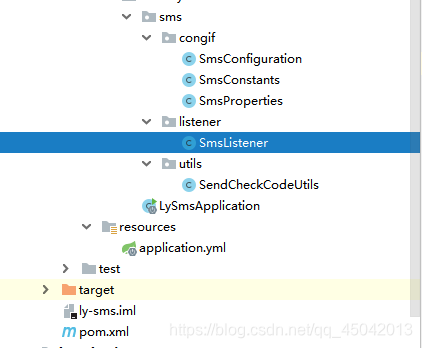
pom
<artifactId>ly-sms</artifactId>
<dependencies>
<!--springboot的web的支持-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!--rabbitMQ消息队列支持-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-amqp</artifactId>
</dependency>
<!--测试-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
</dependency>
<!--阿里云短信的支持-->
<dependency>
<groupId>com.aliyun</groupId>
<artifactId>aliyun-java-sdk-core</artifactId>
<version>4.1.1</version>
</dependency>
<!--工具类支持-->
<dependency>
<groupId>com.leyou</groupId>
<artifactId>ly-common</artifactId>
<version>1.0-SNAPSHOT</version>
</dependency>
</dependencies>
<!--打包springboot插件-->
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
application.yml
server:
port: 8086
spring:
application:
name: sms-service
rabbitmq:
host: 127.0.0.1
username: lyou116
password: lyou
virtual-host: /lyou116 # 队列名称
# 阿里云短信服务配置
ly:
sms:
accessKey: LTAI4Fd2VpKsDUa3MuH # 阿里云创建的子账号
accessKeySecret: hU0f5OL9HjsQmP6tccFfHZAhBb
signName: 乐优平台 # 签名名称
verifyCodeTemplate: SMS_17653921 # 模板名称
domain: dysmsapi.aliyuncs.com # 域名
action: SendSMS # API类型,发送短信
version: 2017-05-25 # API版本,固定值
regionID: cn-hangzhou # 区域id
配置文件 属性的解析
import lombok.Data;
import org.springframework.boot.context.properties.ConfigurationProperties;
@Data
@ConfigurationProperties(prefix = "ly.sms")
public class SmsProperties {
String accessKey;
String accessKeySecret;
String signName;
String verifyCodeTemplate;
String domain;
String version;
String action;
String regionID;
}
加载配置文件到IOC容器
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.context.properties.EnableConfigurationProperties;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
@EnableConfigurationProperties(SmsProperties.class)
public class SmsConfiguration {
@Autowired
private SmsProperties prop;
@Bean
public IAcsClient acsClient(){
DefaultProfile profile = DefaultProfile.getProfile(
prop.getRegionID(),
prop.getAccessKey(),
prop.getAccessKeySecret()
);
return new DefaultAcsClient(profile);
}
}
发送短信工具类
import com.aliyuncs.CommonRequest;
import com.aliyuncs.CommonResponse;
import com.aliyuncs.IAcsClient;
import com.aliyuncs.exceptions.ClientException;
import com.aliyuncs.exceptions.ServerException;
import com.aliyuncs.http.MethodType;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.StringUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import java.util.Map;
@Slf4j
@Component
public class SendCheckCodeUtils {
@Autowired
private IAcsClient client;
@Autowired
private SmsProperties prop;
public void sendCodeMsg(String phone, String code) {
CommonRequest request = new CommonRequest();
request.setMethod(MethodType.POST);
request.setDomain(prop.getDomain());
request.setVersion(prop.getVersion());
request.setAction(prop.getAction());
request.putQueryParameter(SmsConstants.SMS_PARAM_KEY_REGION_ID,prop.getRegionID());
request.putQueryParameter(SmsConstants.SMS_PARAM_KEY_PHONE,phone);
request.putQueryParameter(SmsConstants.SMS_PARAM_KEY_SIGN_NAME,prop.getSignName());
request.putQueryParameter(SmsConstants.SMS_PARAM_KEY_TEMPLATE_CODE,prop.getVerifyCodeTemplate());
request.putQueryParameter(SmsConstants.SMS_PARAM_KEY_TEMPLATE_PARAM,"{\"code\":\""+code+"\"}");
try {
CommonResponse response = client.getCommonResponse(request);
Map<String,String> repsData = JsonUtils.toMap(response.getData(),String.class,String.class);
if (!StringUtils.equals(repsData.get(SmsConstants.SMS_RESPONSE_KEY_CODE),SmsConstants.OK)){
log.error("手机号:"+phone+",接收短信验证码失败!原因为:"+repsData.get(SmsConstants.SMS_RESPONSE_KEY_MESSAGE));
}
log.info("手机号:"+phone+",接收短信验证码成功");
} catch (ServerException e) {
log.error("阿里云短信服务器异常!");
e.printStackTrace();
} catch (ClientException e) {
log.error("本地阿里云短信客户端异常!");
e.printStackTrace();
}
}
}
提取所有阿里云工具类中的key
public class SmsConstants {
public static final String SMS_PARAM_KEY_REGION_ID = "RegionId";
public static final String SMS_PARAM_KEY_PHONE = "PhoneNumbers";
public static final String SMS_PARAM_KEY_SIGN_NAME = "SignName";
public static final String SMS_PARAM_KEY_TEMPLATE_CODE = "TemplateCode";
public static final String SMS_PARAM_KEY_TEMPLATE_PARAM= "TemplateParam";
public static final String SMS_RESPONSE_KEY_CODE = "Code";
public static final String SMS_RESPONSE_KEY_MESSAGE = "Message";
public static final String OK = "OK";
}
RabbitMQ消息队列 短信信息的监听
import lombok.extern.slf4j.Slf4j;
import org.springframework.amqp.core.ExchangeTypes;
import org.springframework.amqp.rabbit.annotation.Exchange;
import org.springframework.amqp.rabbit.annotation.Queue;
import org.springframework.amqp.rabbit.annotation.QueueBinding;
import org.springframework.amqp.rabbit.annotation.RabbitListener;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import java.util.Map;
@Slf4j
@Component
public class SmsListener {
@Autowired
private SendCheckCodeUtils checkCodeUtils;
@RabbitListener(bindings = @QueueBinding(
value = @Queue(value = MQConstants.Queue.SMS_VERIFY_CODE_QUEUE,durable = "true"),
exchange = @Exchange(value = MQConstants.Exchange.SMS_EXCHANGE_NAME,type = ExchangeTypes.TOPIC),
key = MQConstants.RoutingKey.VERIFY_CODE_KEY
))
public void sendCheckCodeMsg(Map<String,String> codeMap){
try {
String phone = codeMap.get("phone");
String code = codeMap.get("code");
checkCodeUtils.sendCodeMsg(phone,code);
}catch (Exception e){
log.error("MQ发送短信验证码失败!异常信息:{}",e);
}
}
}
短信服务的启动类
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class LySmsApplication {
public static void main(String[] args) {
SpringApplication.run(LySmsApplication.class,args);
}