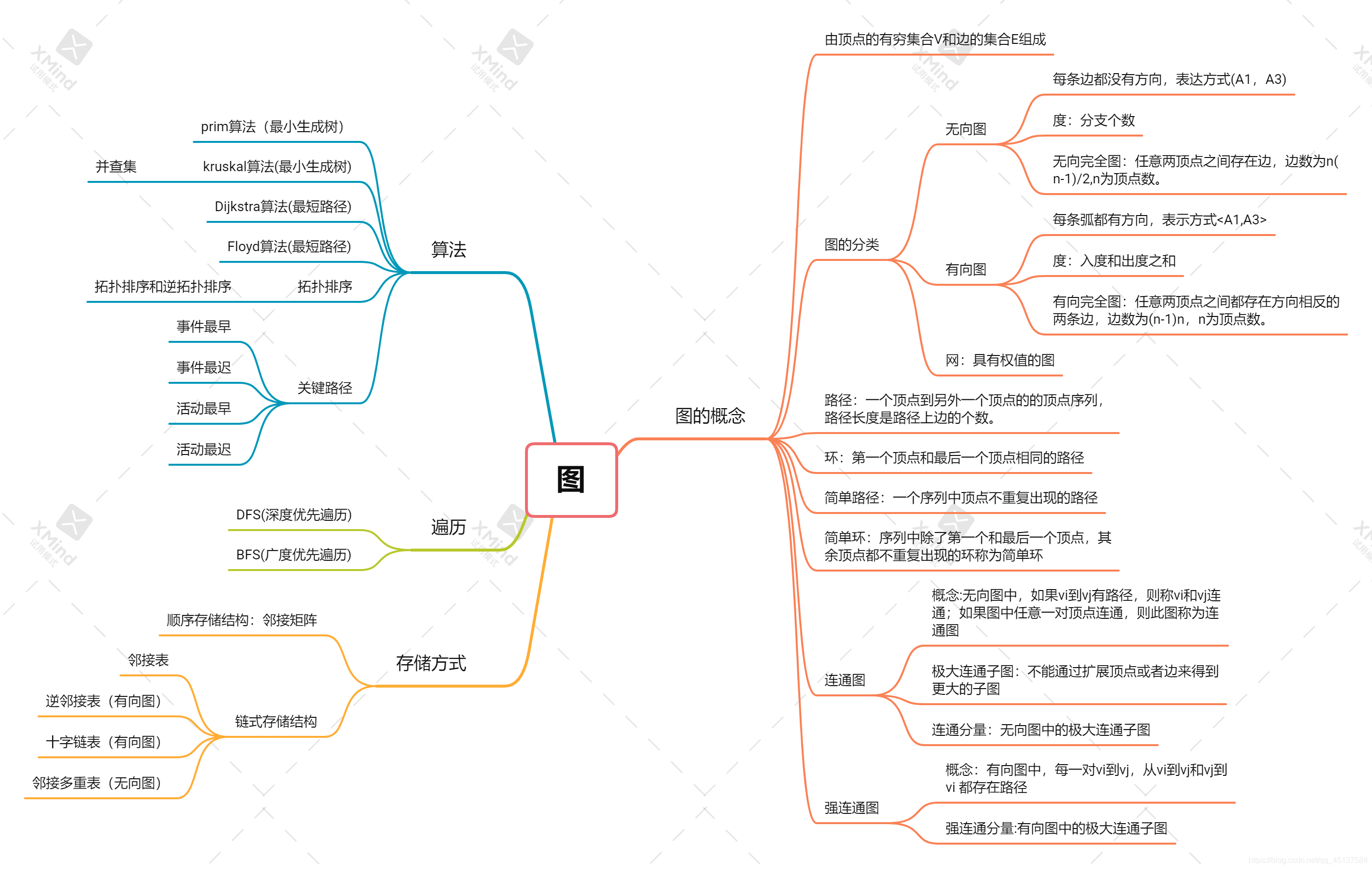
1.邻接矩阵
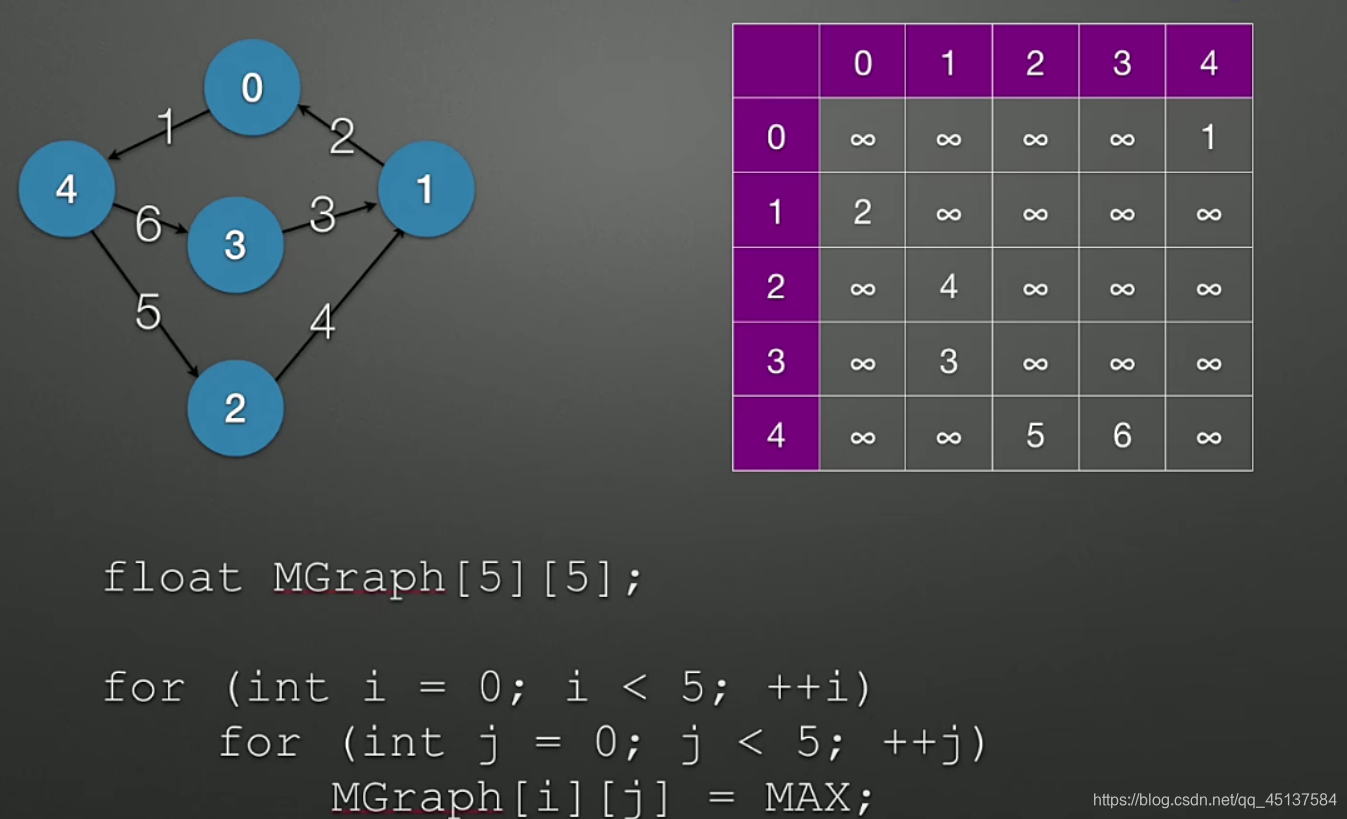
2.邻接表和逆接表
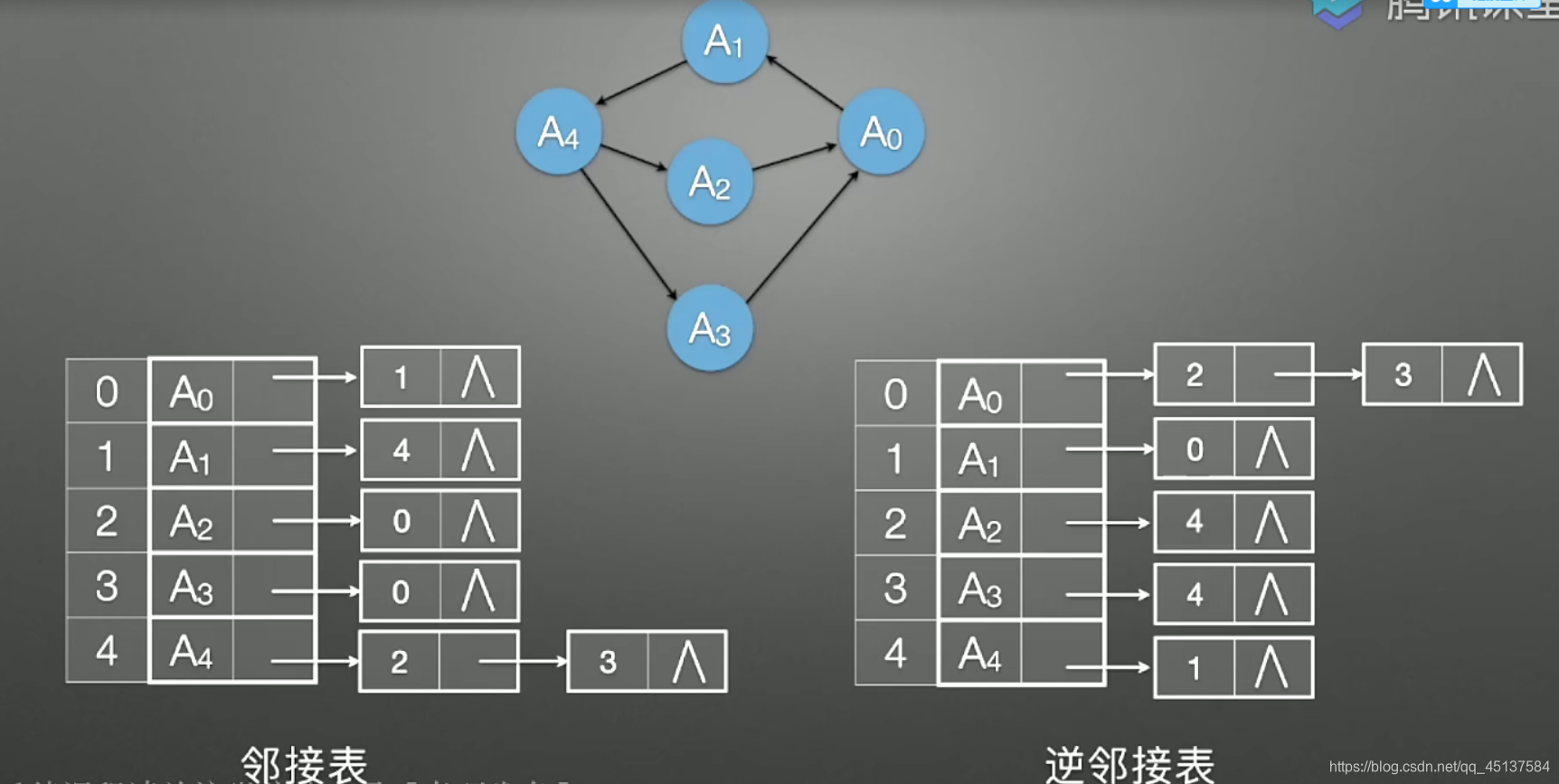
typedef struct ArcNode
{
int adjV;
struct ArcNode* next;
}ArcNode;
typedef struct
{
int data;
ArcNode* first;
}VNode;
typedef struct
{
VNode adjList[maxSize];
int n,e;
}AGraph;
3.十字链表
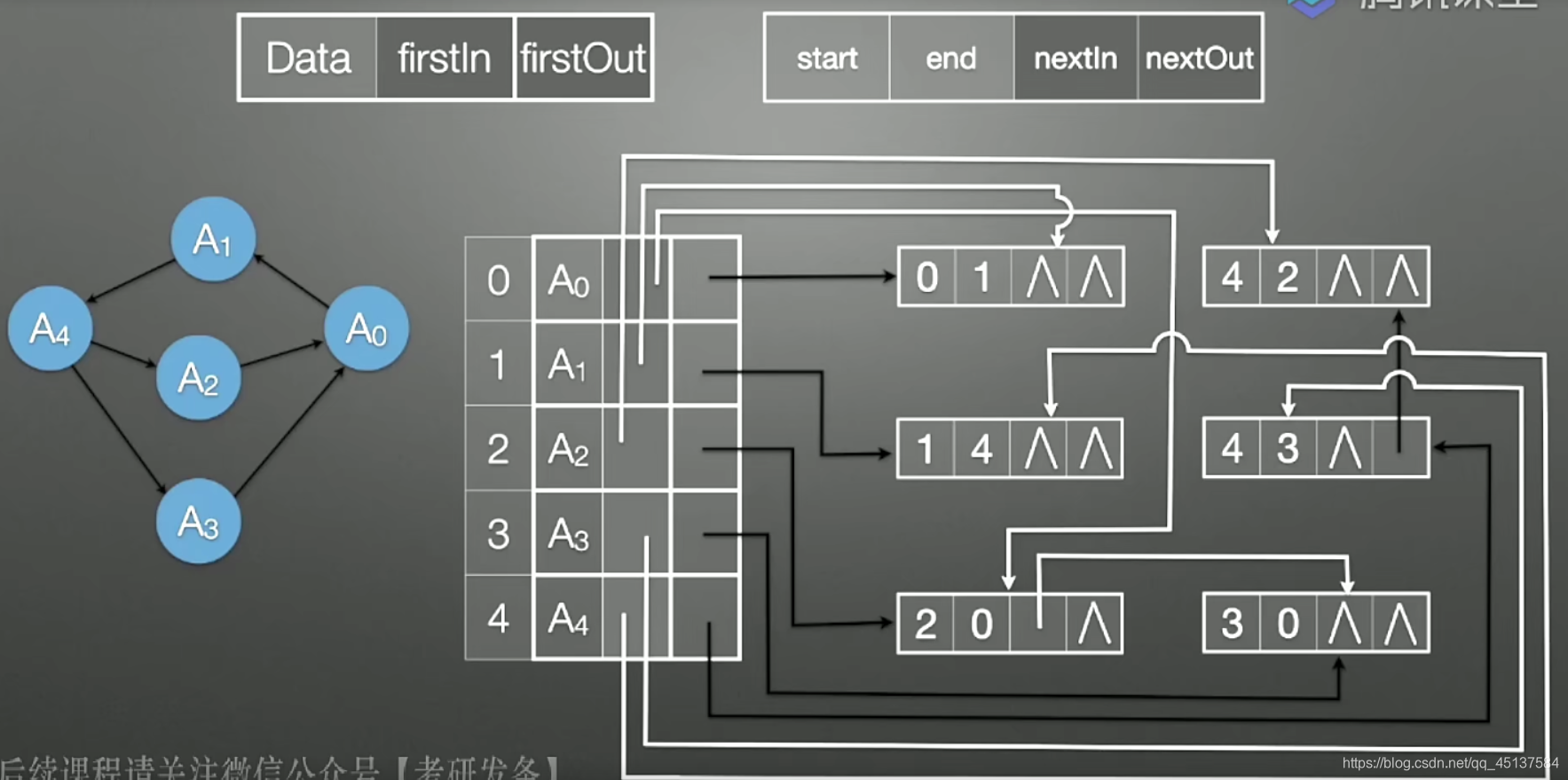
4.邻接多重表
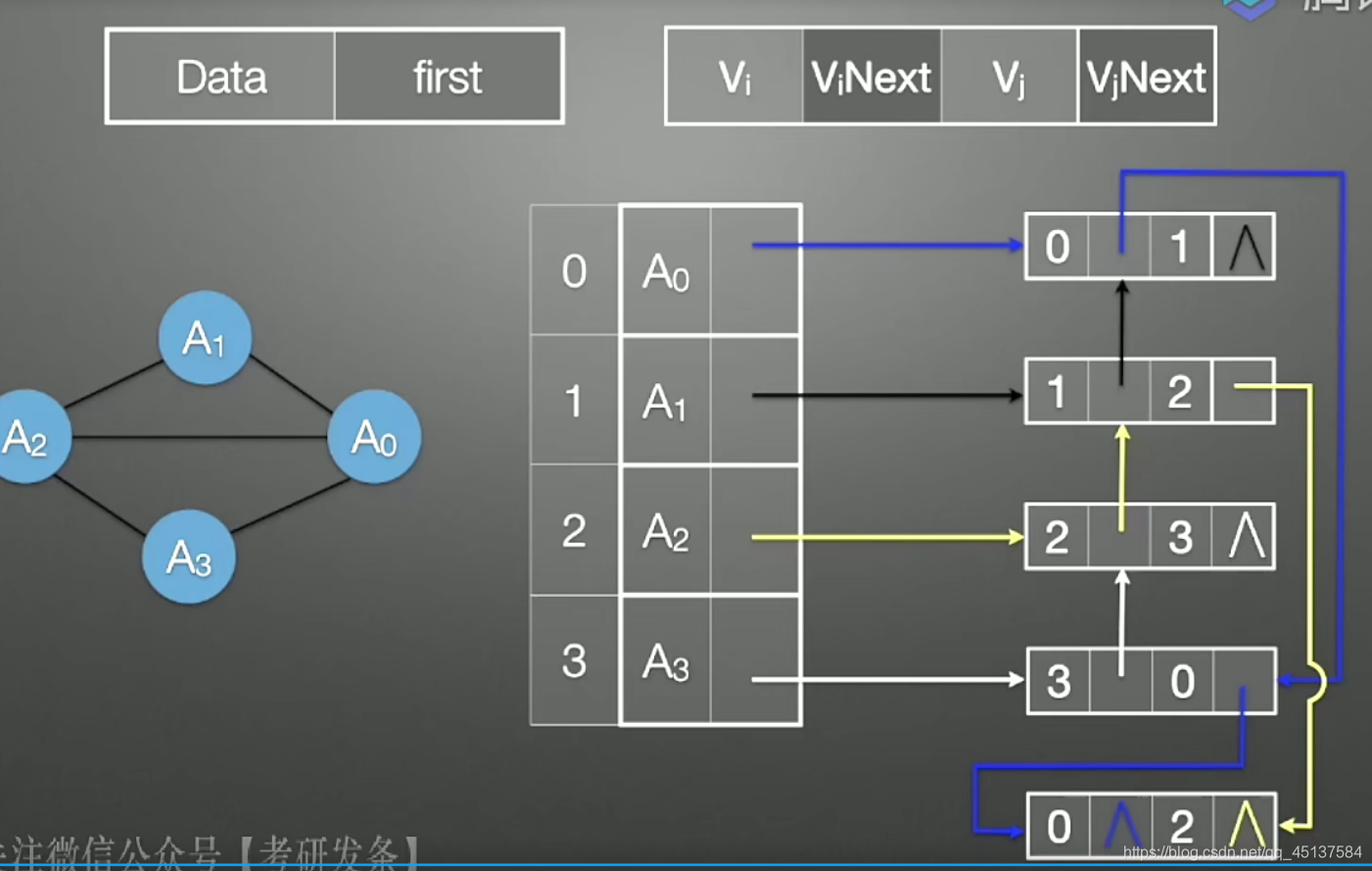
5.DFS(深度优先遍历)
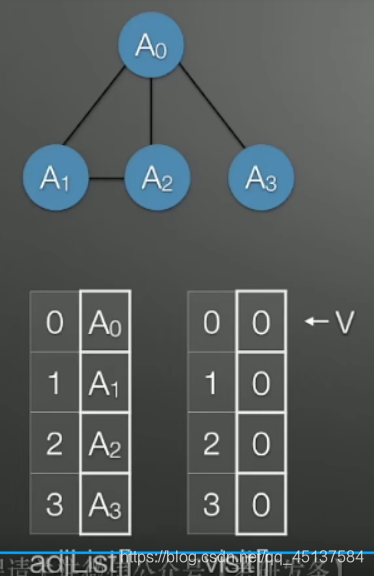
void DFS(int v,AGraph *G)
{
visit[v]=1;
cout<<v<<endl;
ArcNode *q=G->adjList[v].first;
while(q!=NULL)
{
if(visit[q->adjV]==0)
DFS(q->adjV,G);
q=q->next;
}
}
6.BFS(广度优先遍历)
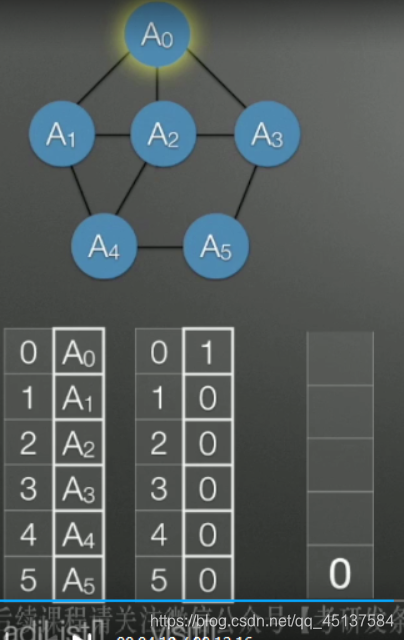
void BFS(AGraph *G,int v)
{
ArcNode *p;
int que[maxSize],front=0,rear=0;
int j;
cout<<v<<endl;
rear=(rear+1)%maxSize;
que[rear]=v;
while(front!=rear)
{
front=(front+1)%maxSize;
j=que[front];
p=G->adjList[j].first;
while(p!=NULL)
{
if(visit[p->adjV]==0)
{
cout<<p->adjV<<endl;
visit[p->adjV]=1;
rear=(rear+1)%maxSize;
que[rear]=p->adjV;
}
p=p->next;
}
}
}
7.prim算法
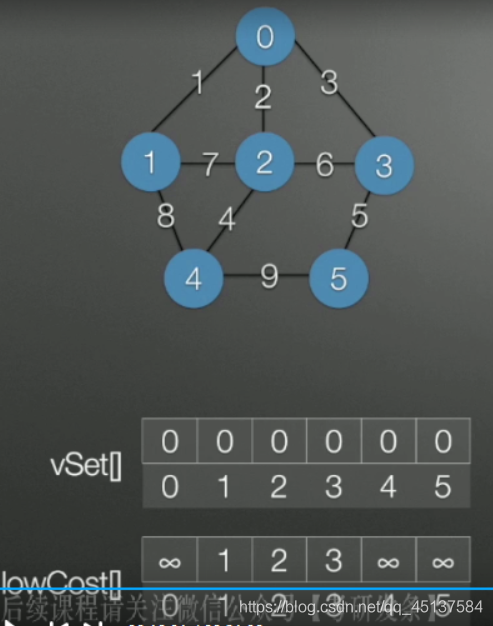
void Prim(int n,float MGraph[][maxSize],int v0,float &sum)
{
int lowCost[n],vSet[n];
int v,k,min;
for(int i=0;i<n;++i)
{
lowCost[i]=MGraph[v0][i];
vSet[i]=0;
}
v=v0;
vSet[v]=1;
sum=0;
for(int i=0;i<n-1;++i)
{
min=INT_FAST16_MAX;
for(int j=0;j<n;++j)
{
if(vSet[j]==0&&lowCost[j]<min)
{
min=lowCost[j];
k=j;
}
vSet[k]=1;
v=k;
sum+=min;
for(int j=0;j<n;++j)
if(vSet[j]==0&&MGraph[v][j]<lowCost[j])
lowCost[j]==MGraph[v][j];
}
}
}
8.kruskal算法
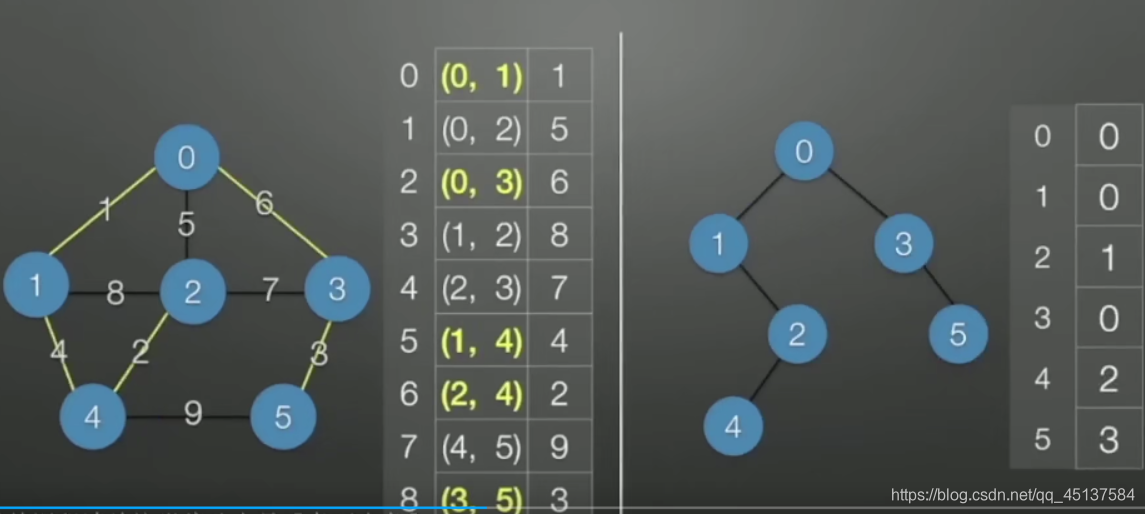
int v[maxSize];
int getRoot(int p)
{
while(p!=v[p])
p=v[p];
return p;
}
void selectionSort1(Road arr[],int n)
{
for(int i=0;i<n;i++)
{
int minIndex=i;
for(int j=i+1;j<n;j++)
if(arr[j]<arr[minIndex])
minIndex=j;
swap(arr[i],arr[minIndex]);
}
}
void Kruskal(Road road[],int n,int e,int &sum)
{
int a,b;
sum=0;
for(int i=0;i<n;++i)
v[i]=i;
selectionSort1(road,e);
for(int i=0;i<e;++i)
{
a=getRoot(road[i].a);
b=getRoot(road[i].b);
if(a!=b)
{
v[a]=b;
sum+=road[i].w;
}
}
}
9.Dijkstra算法
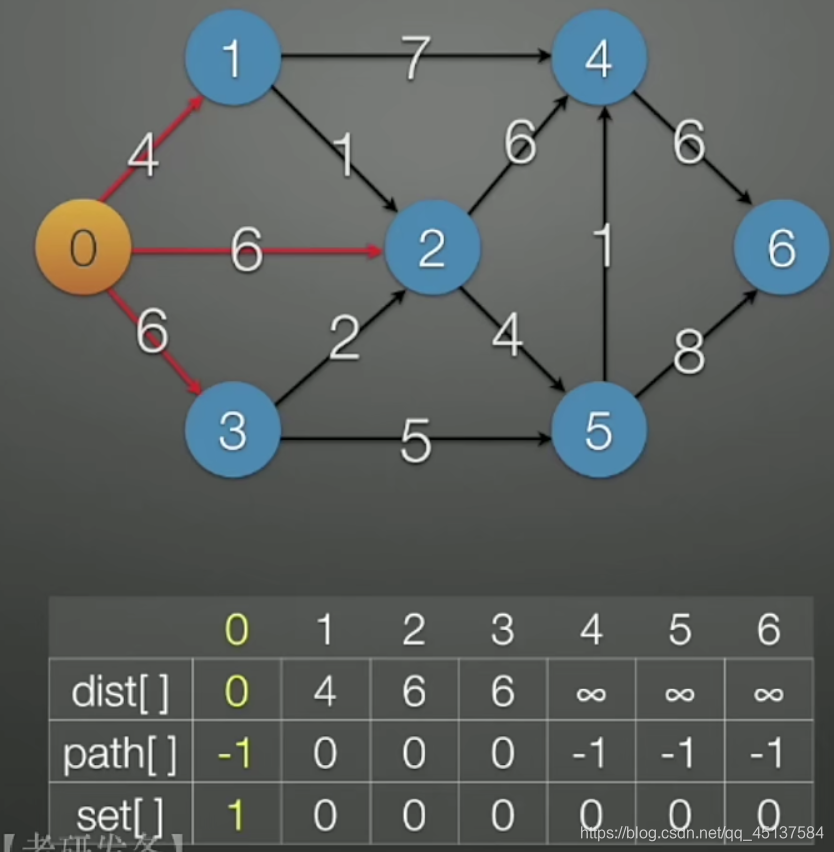
void Dijkstra(int n,float MGrph[][maxSize],int v0,int dist[],int path[])
{
int set[maxSize];
int min,v;
for(int i=0;i<n;++i)
{
dist[i]=MGrph[v0][i];
set[i]=0;
if(MGrph[v0][i]<INFINITY)
path[i]=v0;
else
path[i]=-1;
}
set[v0]=1;path[v0]=-1;
for(int i=0;i<n-1;++i)
{
min=INFINITY;
for(int j=0;j<n;++j)
if(set[j]==0&&dist[j]<min)
{
v=j;
min=dist[j];
}
set[v]=1;
for(int j=0;j<n;++j)
{
if(set[j]==0&&dist[v]+MGrph[v][j]<dist[j])
{
dist[j]=dist[v]+MGrph[v][j];
path[j]=v;
}
}
}
}
10.Floyd算法
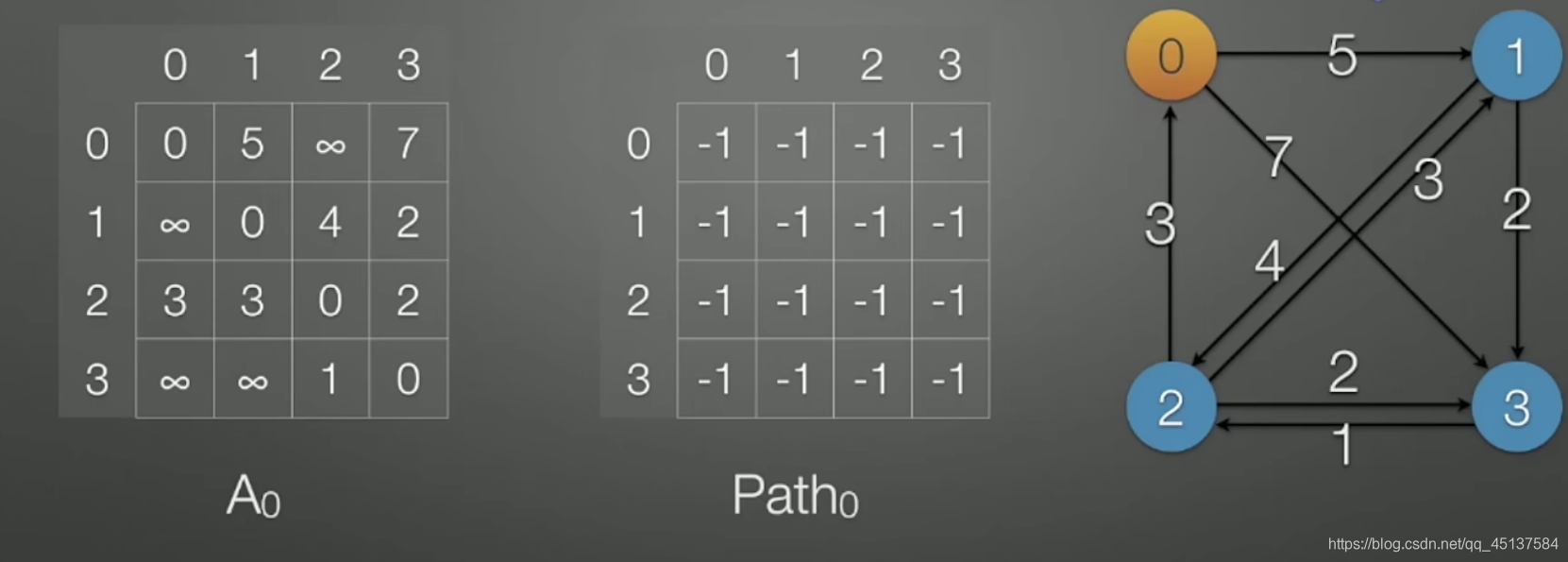
void Floyd(int n,float MGraph[][maxSize],int path[][maxSize])
{
int i,j,v;
int A[n][n];
for(i=0;i<n;++j)
for(j=0;j<n;++j)
{
A[i][j]=MGraph[i][j];
path[i][j]=-1;
}
for(v=0;v<n;++v)
for(i=0;i<n;++i)
for(j=0;j<n;++j)
if(A[i][j]>A[i][v]+A[v][j])
{
A[i][j]=A[i][v]+A[v][j];
path[i][j]=v;
}
}
void printPath(int u,int v,int path[][maxSize])
{
if(path[u][v]==-1)
cout<<1<<endl;
else{
int mid =path[u][v];
printPath(u,mid,path);
printPath(mid,v,path);
}
}
11.拓扑排序
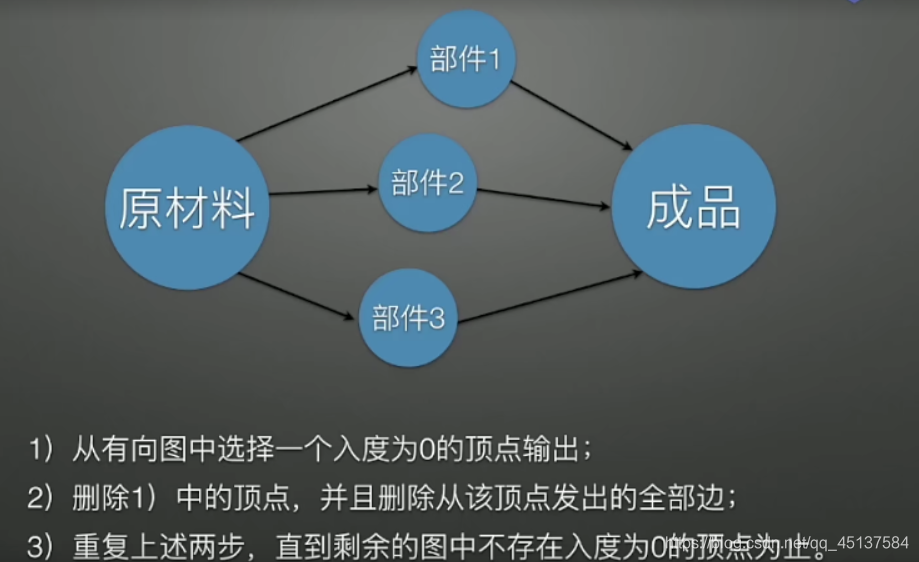
typedef struct ArcNode
{
int adjV;
struct ArcNode* next;
}ArcNode;
typedef struct
{
int data;
int count;
ArcNode* first;
}VNode;
typedef struct
{
VNode adjList[maxSize];
int n,e;
}AGraph;
int TopSort(AGraph *G)
{
int i,j,n=0;
int stack[maxSize],top=-1;
ArcNode *p;
for(i=0;i<G->n;++i)
{
if(G->adjList[i].count==0)
stack[++top]=i;
}
while(top!=-1)
{
i=stack[top--];
++n;
cout<<i<<endl;
p=G->adjList[i].first;
while(p!=NULL)
{
j=p->adjV;
--(G->adjList[j].count);
if(G->adjList[j].count==0)
stack[++top]=j;
p=p->next;
}
}
if(n==G->n)
return 1;
else
return 0;
}
12.逆拓扑排序
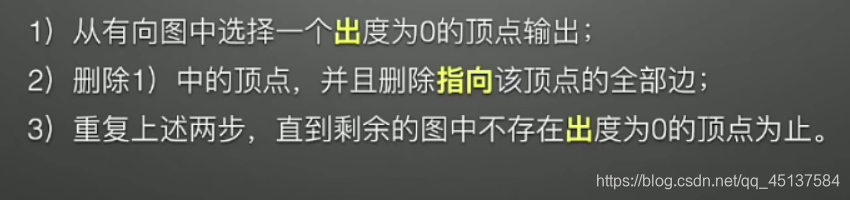
typedef struct ArcNode
{
int adjV;
struct ArcNode* next;
}ArcNode;
typedef struct
{
int data;
int count;
ArcNode* first;
}VNode;
typedef struct
{
VNode adjList[maxSize];
int n,e;
}AGraph;
void endSort(int v,AGraph *G)
{
visit[v]=1;
ArcNode* q=G->adjList[v].first;
while(q!=NULL)
{
if(visit[q->adjV]==0)
DFS(q->adjV,G);
q=q->next;
}
cout<<v<<endl;
}
13.关键路径
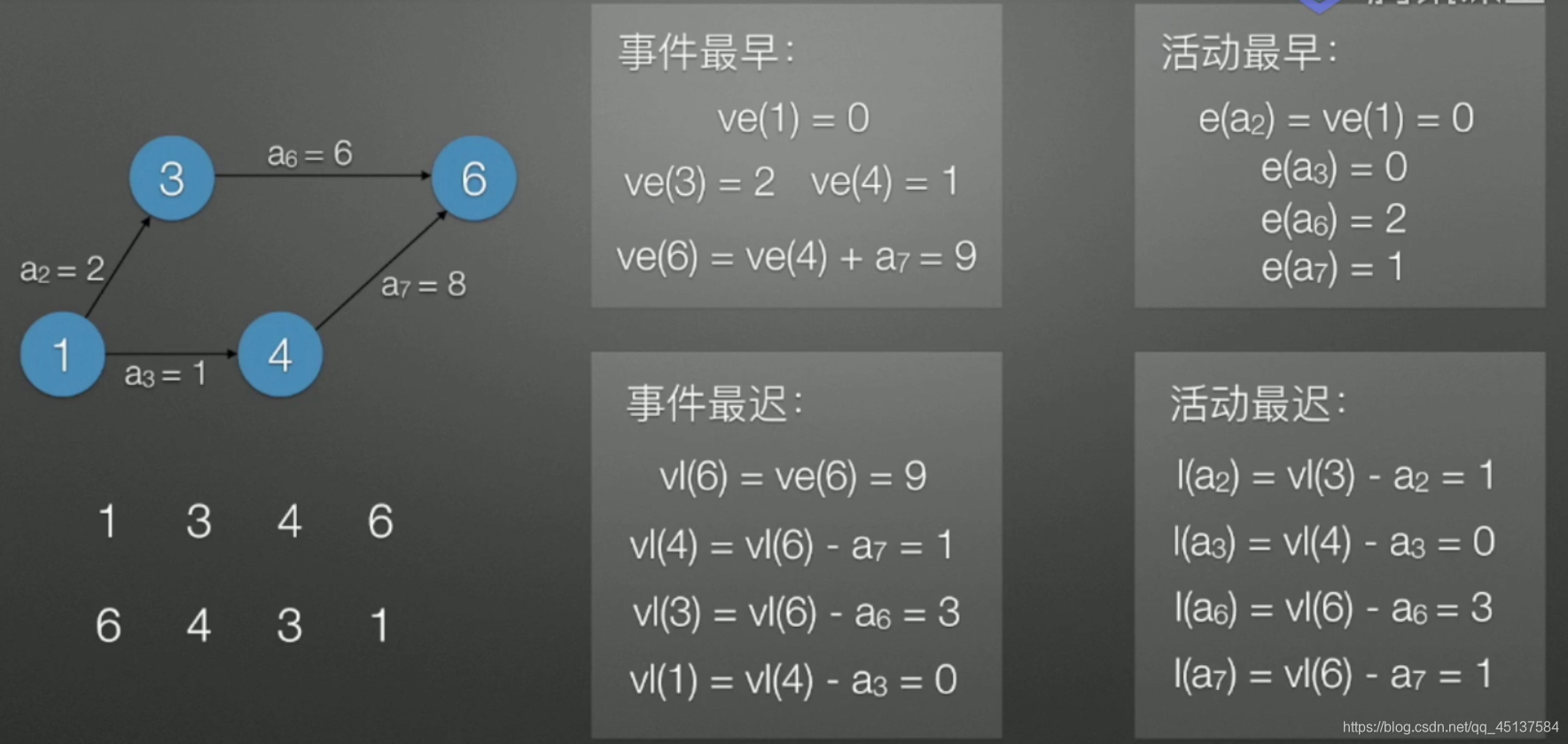