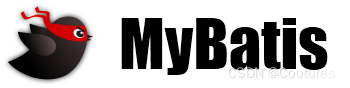
1. 回顾 JDBC
@Test
public void testJdbc() throws Exception {
String url = "jdbc:mysql://localhost:3306/javaee_***";
String username = "root";
String password = "123456";
Class.forName("com.mysql.jdbc.Driver");
Connection connection = DriverManager.getConnection(url, username, password);
String sql = "SELECT * FROM javaee_2203.student WHERE id = 1";
PreparedStatement statement = connection.prepareStatement(sql);
ResultSet resultSet = statement.executeQuery();
Student student = new Student();
if (resultSet.next()) {
student.setId(resultSet.getInt("id"));
student.setName(resultSet.getString("name"));
student.setAge(resultSet.getInt("age"));
student.setScore(resultSet.getFloat("score"));
}
System.out.println(student);
resultSet.close();
statement.close();
connection.close();
}
2. MyBatis 概述
2.1 ORM 思想
ORM 对象关系映射
Object Relational Mapping
不止于数据库
数据库 <==> Java 对象之间的映射关系(JavaBean Java Map)
JSON <==> Java 对象
XML <==> Java 对象
字符串数据信息 <==> Java 对象
url 参数 username=张三&password=123456
键值对形式的数据是极为方便,操作简单,使用便捷。
2.2 MyBatis 框架
基于 ORM 思想,全世界范围以内流行的数据库框架结构。
MyBatis 原本是 Apache组织下的一个 ibatis 项目。2010年 Google 接手 ibatis ==> 改名为 MyBatis。
2013年项目迁移到 Github
非常优秀的,效率极高,操作方便,Java数据库持久层框架,支持动态 SQL,缓存机制,高级映射。。。MyBatis也是基于 JDBC 进行封装开发。对于开发者不需要在关注 JDBC 的执行过程,而是关注 SQL语句本身,参数(parameterType)所需和对应的结果产出(returnType)。
MyBatis提供了 XML文件 和 Annotation 注解两种方式来处理 SQL,并且自动完成 ORM 操作。
3. MyBatis 开发步骤【重点】
3.1 Maven 导入 MyBatis 依赖
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.6</version>
</dependency>
3.2 创建数据表
CREATE TABLE `student`
(
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(32) NOT NULL,
`age` int(11) NOT NULL,
`score` float(5, 2) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE = InnoDB
AUTO_INCREMENT = 17
DEFAULT CHARSET = utf8
3.3 定义实体类
package com.qfedu.entity;
import lombok.*;
@Data
@NoArgsConstructor
@AllArgsConstructor
public class Student {
private Integer id;
private String name;
private Integer age;
private Float score;
}
3.4 创建对应Dao接口
package com.qfedu.dao;
import com.qfedu.entity.Student;
public interface StudentDao {
Student selectStudentById(Integer id);
}
3.5 配置 MyBatis
需要完成 MyBatis-config.xml 文件。配置文件要求的路径
src/main/resources
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<environments default="MySQL_Develop">
<environment id="MySQL_Develop">
<transactionManager type="JDBC"/>
<dataSource type="POOLED">
<property name="driver" value="com.mysql.jdbc.Driver"/>
<property name="url"
value="jdbc:mysql://localhost:3306/javaee_2203?serverTimezone=Asia/Shanghai&useUnicode=true&characterEncoding=utf8&useSSL=false"/>
<property name="username" value="root"/>
<property name="password" value="123456"/>
</dataSource>
</environment>
</environments>
</configuration>
3.6 编写 Mapper 文件
对应 StudentDao 接口的 mapper 文件需要存储在
src/main/resources
一般情况下 mapper 文件名称和对应 Dao 接口名称有相关性
interface StudentDao ==> mapper 文件 ==> StudentDaoMapper.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.qfedu.dao.StudentDao">
<select id="selectStudentById" resultType="com.qfedu.entity.Student">
SELECT * FROM javaee_2203.student WHERE id = #{arg0}
</select>
</mapper>
一定要在 mybatis-config.xml 文件中,注册 mapper 文件
<mappers>
<mapper resource="StudentDaoMapper.xml"/>
</mappers>
3.7 测试
package com.qfedu.test;
import com.qfedu.dao.StudentDao;
import com.qfedu.entity.Student;
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
import org.junit.Test;
import java.io.IOException;
import java.io.InputStream;
public class MyBatisStudentDaoTest {
@Test
public void testSelectStudent() throws IOException {
InputStream in = Resources.getResourceAsStream("mybatis-config.xml");
SqlSessionFactory factory = new SqlSessionFactoryBuilder().build(in);
SqlSession sqlSession = factory.openSession();
StudentDao studentDao = sqlSession.getMapper(StudentDao.class);
Student student = studentDao.selectStudentById(1);
System.out.println(student);
}
}
4. 问题总结
4.1 SQL 语句参数问题
方法参数个数为 1
Mapper 文件中 SQL 语句对应参数可以使用 arg0 、param1、自定义名称都可以,MyBatis 自动识别,不限制参数名称
方法参数为多个
a. 未参数绑定的情况下
Mapper中 SQL 语句原生参数可以采用
#{arg0} #{arg1}
#{param1} #{param2}
b. 参数使用 @Param 注解绑定参数名称
@Param("id") Integer id, @Param("name") String name
要求 Mapper 文件中的 SQL 语句参数使用 #{id} #{name}
4.2 jdbc.properties 文件管理数据连接必要资源
# jdbc.properties 数据库连接必要资源配置文件 存储在 src/main/resources
jdbc.driver=com.mysql.jdbc.Driver
jdbc.url=jdbc:mysql://localhost:3306/javaee_2203?serverTimezone=Asia/Shanghai&useUnicode=true&characterEncoding=utf8&useSSL=false
jdbc.username=root
jdbc.password=123456
<properties resource="jdbc.properties"/>
...
<dataSource type="POOLED">
<property name="driver" value="${jdbc.driver}"/>
<property name="url" value="${jdbc.url}"/>
<property name="username" value="${jdbc.username}"/>
<property name="password" value="${jdbc.password}"/>
</dataSource>
4.3 类型别名/简拼
修改 MyBatis-Config 配置文件
<typeAliases>
<typeAlias type="com.qfedu.entity.Student" alias="Student"/>
</typeAliases>
5. MyBatis CRUD 操作【重点】
5.1 StudentDao.java
package com.qfedu.dao;
import com.qfedu.entity.Student;
import org.apache.ibatis.annotations.Param;
import java.util.Map;
public interface StudentDao {
Student selectStudentById(Integer id);
Student selectStudentByIdAndName(Integer id, String name);
Student selectStudentByIdAndNameTwo(@Param("id") Integer id, @Param("name") String name);
void insertStudent(Student student);
void insertStudentMap(Map<String, Object> map);
void updateStudent(Student stu);
void updateStudentMap(Map<String, Object> map);
void deleteStudent(@Param("id") int id);
}
5.2 StudentDaoMapper.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.qfedu.dao.StudentDao">
<select id="selectStudentById" resultType="Student">
SELECT * FROM javaee_2203.student WHERE id = #{id}
</select>
<select id="selectStudentByIdAndName" resultType="Student">
SELECT * FROM javaee_2203.student WHERE id = #{param1} and name = #{param2}
</select>
<select id="selectStudentByIdAndNameTwo" resultType="Student">
SELECT * FROM javaee_2203.student WHERE id = #{id} and name = #{name}
</select>
<insert id="insertStudent" parameterType="Student">
INSERT INTO javaee_2203.student VALUES (null, #{name}, #{age}, #{score})
</insert>
<insert id="insertStudentMap">
INSERT INTO javaee_2203.student(name, age, score) VALUES (#{mapName}, #{mapAge}, #{mapScore})
</insert>
<update id="updateStudent" parameterType="Student">
UPDATE javaee_2203.student
SET name = #{name},
age = #{age},
score = #{score}
WHERE id = #{id}
</update>
<update id="updateStudentMap">
UPDATE javaee_2203.student
SET name = #{mapName},
age = #{mapAge},
score = #{mapScore}
WHERE id = #{mapId}
</update>
<delete id="deleteStudent" parameterType="int">
DELETE FROM javaee_2203.student WHERE id = #{id}
</delete>
</mapper>