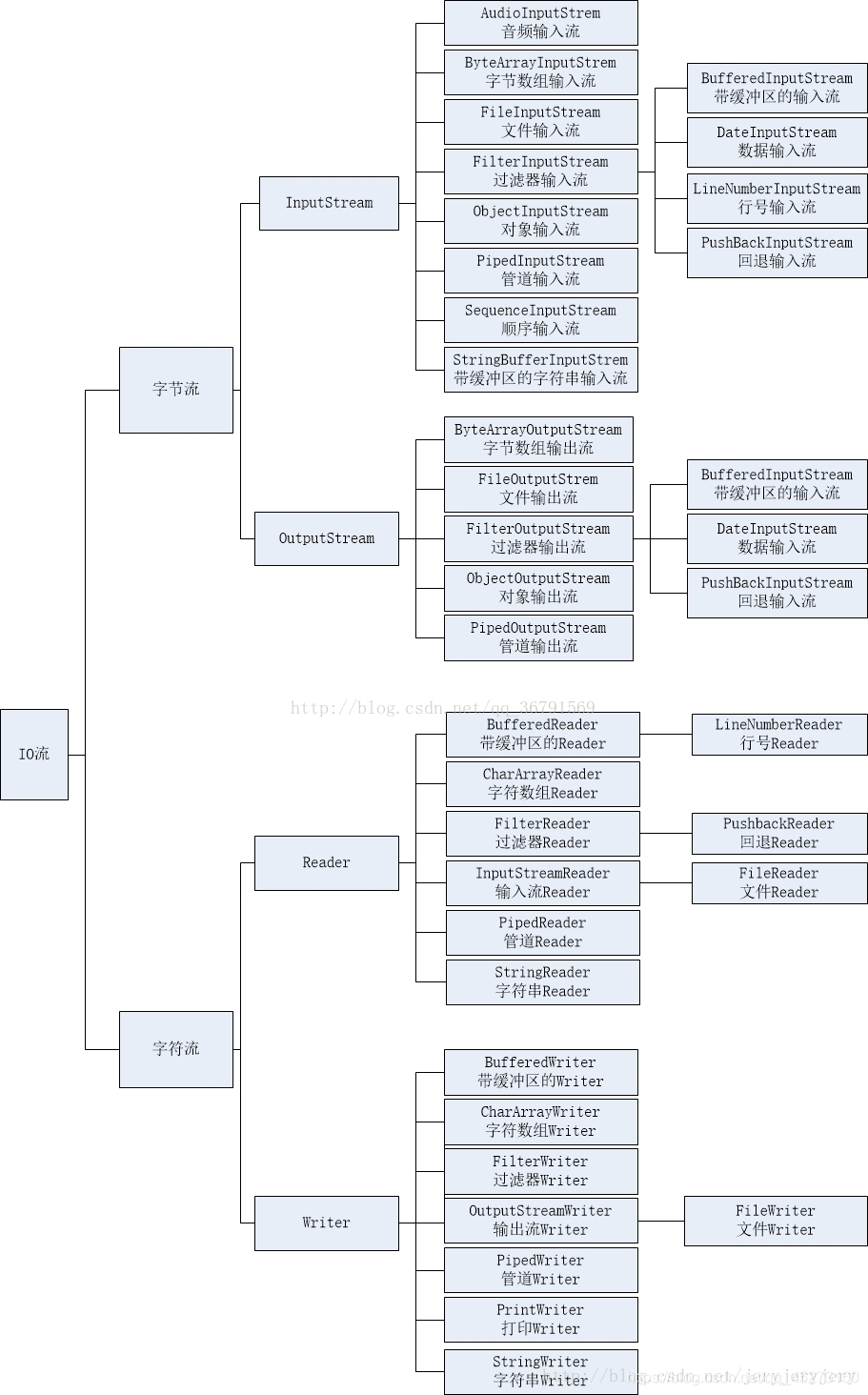
package com.llb.io;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
public class MyByteIO {
public static void main(String[] args) throws Exception {
myFileInPut();
}
private static void myFileOutPut()throws Exception{
FileOutputStream fos =
new FileOutputStream(
new File("E:\\itheima\\test\\aaa\\c.txt"),true);
fos.write(97);
fos.write("\r\n".getBytes());
String str = "云想衣裳花想容,\t春风拂槛露华浓。\n若非群玉山头见,\t会向瑶台月下逢。";
fos.write(str.getBytes());
fos.close();
}
private static void myFileInPut()throws Exception{
FileInputStream fis =
new FileInputStream(
new File("E:\\itheima\\test\\aaa\\c.txt"));
int b ;
while ((b = fis.read()) != -1){
System.out.println((char)b);
}
fis.close();
}
}
package com.llb.io;
import java.io.*;
public class MyBuffered {
public static void main(String[] args) throws Exception {
BufferedInputStream bis =
new BufferedInputStream(
new FileInputStream("E:\\itheima\\test\\aaa\\a.mp4"));
BufferedOutputStream bos =
new BufferedOutputStream(
new FileOutputStream("E:\\itheima\\test\\copy.mp4"));
int len ;
byte[] bytes = new byte[1024];
while ((len=bis.read(bytes))!=-1){
bos.write(bytes,0,len);
}
bos.close();
bis.close();
}
}
package com.llb.io;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
public class FileCopy {
public static void main(String[] args)throws Exception {
copyBig();
}
private static void copy()throws Exception{
FileInputStream fis =
new FileInputStream(
new File("E:\\itheima\\test\\aaa\\a.mp4"));
FileOutputStream fos =
new FileOutputStream(
new File("E:\\itheima\\test\\a.mp4"));
int b ;
while ((b=fis.read())!=-1){
fos.write(b);
}
fos.close();
fis.close();
}
private static void copyBig() throws Exception {
FileInputStream fis =
new FileInputStream(
new File("E:\\itheima\\test\\aaa\\a.mp4"));
FileOutputStream fos =
new FileOutputStream(
new File("E:\\itheima\\test\\a.mp4"));
byte[] bytes = new byte[1024];
int count=0;
int len;
while ((len = fis.read(bytes))!=-1){
fos.write(bytes,0,len);
count++;
}
System.out.println(count);
fos.close();
fis.close();
}
}
package com.llb.io;
import java.io.*;
public class BufferedCopy {
public static void main(String[] args) throws Exception {
BufferedInputStream bis =
new BufferedInputStream(
new FileInputStream("E:\\itheima\\test\\aaa\\a.mp4"));
BufferedOutputStream bos =
new BufferedOutputStream(
new FileOutputStream("E:\\itheima\\test\\copy.mp4"));
byte[] bytes = new byte[1024];
int len;
while ((len=bis.read(bytes))!=-1){
bos.write(bytes,0,len);
}
bos.close();
bis.close();
}
}
package com.llb.转换流;
import java.io.*;
public class Demo {
public static void main(String[] args) throws Exception {
OutputStreamWriter osw =
new OutputStreamWriter(new FileOutputStream("Day12\\c.txt"),
"UTF-8");
osw.write("悲莫悲兮生别离");
osw.write("乐莫乐兮新相知");
osw.close();
InputStreamReader isr =
new InputStreamReader(
new FileInputStream("Day12\\c.txt"),
"UTF-8");
int len;
char[] chars = new char[1024];
while ((len=isr.read())!=-1){
System.out.println(new String(chars,0,len));
}
isr.close();
}
}
package com.llb.对象操作流;
import java.io.*;
import java.util.ArrayList;
public class Exercise {
public static void main(String[] args) throws Exception {
Student student1 = new Student("刘备",18);
Student student2 = new Student("关羽",19);
Student student3 = new Student("张飞",17);
ObjectOutputStream oos =
new ObjectOutputStream(new FileOutputStream("Day12\\e.txt"));
ArrayList<Student> list = new ArrayList<Student>();
list.add(student1);
list.add(student2);
list.add(student3);
oos.writeObject(list);
oos.close();
ObjectInputStream ois =
new ObjectInputStream(new FileInputStream("Day12\\e.txt"));
ArrayList<Student> o = (ArrayList<Student>) ois.readObject();
for (Student student : o) {
System.out.println(student);
}
ois.close();
}
}
class Student implements Serializable {
private static final long serialVersionUID=1L;
private String username;
private Integer age;
@Override
public String toString() {
return "Student{" +
"username='" + username + '\'' +
", age=" + age +
'}';
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
public Student(String username, Integer age) {
this.username = username;
this.age = age;
}
public Student() {
}
}
package com.llb.对象操作流;
import java.io.*;
public class Demo {
public static void main(String[] args) throws IOException, ClassNotFoundException {
ObjectInputStream ois =
new ObjectInputStream(new FileInputStream("Day12\\d.txt"));
User user = (User) ois.readObject();
System.out.println(user);
ois.close();
}
}
class User implements Serializable {
private String username;
private transient String password;
private static final long serialVersionUID=1L;
@Override
public String toString() {
return "User{" +
"username='" + username + '\'' +
", password='" + password + '\'' +
'}';
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public User(String username, String password) {
this.username = username;
this.password = password;
}
public User() {
}
}
package com.llb.字符流;
import java.io.*;
import java.util.Scanner;
public class Exercise {
public static void main(String[] args) throws IOException {
write();
}
private static void write()throws IOException{
Scanner sc = new Scanner(System.in);
System.out.println("请录入用户名");
String username = sc.next();
System.out.println("请录入密码");
String password = sc.next();
FileWriter fw = new FileWriter(new File("Day12\\a.txt"));
fw.write(username);
fw.write("=");
fw.write(password);
fw.flush();
fw.close();
}
private static void read() throws FileNotFoundException {
FileReader fr = new FileReader(new File("Day12\\a.txt"));
int len;
}
}
package com.llb.字符流;
import javax.xml.transform.Source;
import java.io.*;
import java.util.Arrays;
public class BufferedExe {
public static void main(String[] args) throws IOException {
BufferedReader br =
new BufferedReader(new FileReader("Day12\\b.txt"));
String s = br.readLine();
String[] s1 = s.split(" ");
int[] arr = new int[s1.length];
for (int i = 0; i < s1.length; i++) {
String s3 = s1[i];
int num = Integer.parseInt(s3);
arr[i] = num;
}
System.out.println("排序前的数组 : "+Arrays.toString(arr));
Arrays.sort(arr);
System.out.println("排序后的数组 : "+Arrays.toString(arr));
BufferedWriter bw =
new BufferedWriter(new FileWriter("Day12\\b.txt"));
for (int i : arr) {
bw.write(i+" ");
bw.flush();
}
bw.close();
br.close();
}
}
package com.llb.字符流;
import java.io.*;
public class BufferedDemo {
public static void main(String[] args) throws Exception {
BufferedReader br =
new BufferedReader(new FileReader("Day12\\a.txt"));
String line;
while ((line=br.readLine())!=null){
System.out.println(line);
}
br.close();
}
private static void reader() throws Exception {
BufferedReader br =
new BufferedReader(new FileReader("Day12\\a.txt"));
char[] chars = new char[1024];
int len;
while ((len=br.read(chars))!=-1){
System.out.println(new String(chars,0,len));
}
br.close();
}
private static void writer() throws Exception{
BufferedWriter bw =
new BufferedWriter(new FileWriter("Day12\\b.txt"));
bw.write(97);
char[] chars = {98,99,100,101,102,103};
bw.write(chars);
bw.write(chars,0,chars.length);
bw.close();
}
}
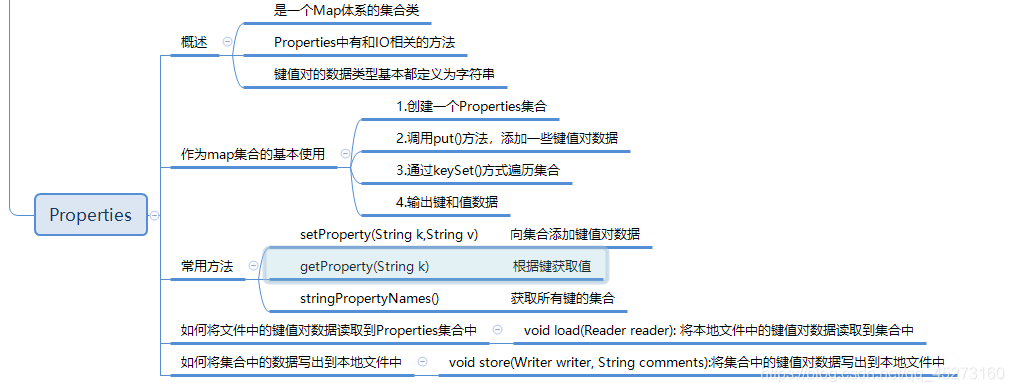
package com.llb.properties;
import java.util.Map;
import java.util.Properties;
import java.util.Set;
public class Demo {
public static void main(String[] args) {
Properties prop = new Properties();
prop.put("1003","hinata3");
prop.put("1001","hinata1");
prop.put("1002","hinata2");
prop.setProperty("中国","北京");
System.out.println(prop);
prop.remove("1001");
prop.put("1002","naruto");
System.out.println(prop);
Set<String> set = prop.stringPropertyNames();
for (String s : set) {
System.out.print("key's set "+s+"\t");
}
System.out.println();
Set<Map.Entry<Object, Object>> entries = prop.entrySet();
for (Map.Entry<Object, Object> entry : entries) {
Object key = entry.getKey();
Object value = entry.getValue();
System.out.print(key);
System.out.println(value);
}
}
}