前言
- configparser是Python标准库中的一个模块,用于解析和操作配置文件。它提供了读取和写入常见配置文件格式的功能。
- configparser模块主要支持写入 INI 文件格式。INI文件格式是一种常见的配置文件格式,它由节(section)和键值对(key-value)组成。每个键值对位于一个节内,节用方括号 []表示,键值对由键和值组成。
一、写入
import configparser
config = configparser.ConfigParser()
config.add_section('new_section')
config.set('new_section', 'option_name', 'value')
config['Database'] = {
'host': 'localhost',
'port': '3306',
'username': 'admin',
'password': 'secret'
}
with open('config.ini', 'w', encoding='utf-8') as configfile:
config.write(configfile)
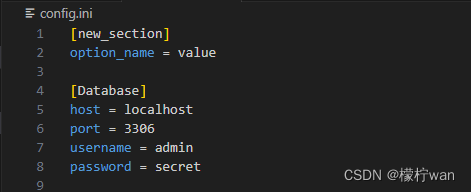
二、读取
import configparser
config = configparser.ConfigParser()
config.read('config.ini')
sections = config.sections()
print(sections)
options = config.options('Database')
print(options)
items = config.items('Database')
print(items)
value = config.get('Database', 'host')
print(type(value), value)

三、修改
import configparser
config = configparser.ConfigParser()
config.read('config.ini')
sections = config.sections()
old_section_name = 'Database'
config.add_section('mysql')
for key, value in config.items(old_section_name):
config.set('mysql', key, value)
config.remove_section('Database')
section_name = 'mysql'
old_option_name = 'username'
old_value = config.get(section_name, old_option_name)
config.remove_option(section_name, old_option_name)
new_option_name = 'user'
config.set(section_name, new_option_name, old_value)
section_name = 'mysql'
option_name = 'user'
new_value = 'root'
config.set(section_name, option_name, new_value)
with open('config.ini', 'w') as configfile:
config.write(configfile)
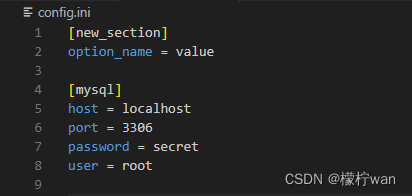
四、删除
import configparser
config = configparser.ConfigParser()
config.read('config.ini')
config.remove_section('new_section')
config.remove_option('mysql', 'user')
with open('config.ini', 'w') as configfile:
config.write(configfile)
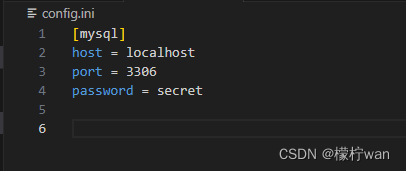
五、封装示例
import configparser
class ConfigFile:
"""
使用configparser模块读写INI格式配置文件的类
"""
def __init__(self, file_path, encoding):
"""
初始化方法,传入配置文件路径
:param file_path: 配置文件路径
:param encoding: 配置编码格式
"""
self.file_path = file_path
self.encoding = encoding
def read(self):
"""
读取配置文件内容
:return: 返回一个字典,键为节名,值为字典,键为选项名,值为选项值
"""
config = configparser.ConfigParser()
config.read(self.file_path, encoding=self.encoding)
return {section: dict(config.items(section)) for section in config.sections()}
def write(self, data_dict):
"""
写入配置文件内容
:param data_dict: 字典类型数据,包含配置文件内容
"""
config = configparser.ConfigParser()
for section, options in data_dict.items():
config.add_section(section)
for option, value in options.items():
config.set(section, option, value)
with open(self.file_path, 'w', encoding=self.encoding) as f:
config.write(f)
def update(self, section, data_dict, new_section=None):
"""
更新配置文件指定节的内容
:param section: 节名
:param data_dict: 字典类型数据,包含要更新的选项及其值
:param new_section: 新的节名,如果为None,则不修改节名
"""
config = configparser.ConfigParser()
config.read(self.file_path, encoding=self.encoding)
if not config.has_section(section):
config.add_section(section)
for option, value in data_dict.items():
config.set(section, option, value)
if new_section is not None:
config.add_section(new_section)
for option, value in data_dict.items():
config.set(new_section, option, value)
config.remove_section(section)
with open(self.file_path, 'w', encoding=self.encoding) as f:
config.write(f)
def delete(self, section, option=None):
"""
删除配置文件指定节或选项
:param section: 节名
:param option: 选项名,如果为None,则删除整个节
"""
config = configparser.ConfigParser()
config.read(self.file_path, encoding=self.encoding)
if config.has_section(section):
if option is None:
config.remove_section(section)
else:
config.remove_option(section, option)
with open(self.file_path, 'w', encoding=self.encoding) as f:
config.write(f)
else:
print("节点不存在!")
if __name__ == "__main__":
config = ConfigFile('example.ini', 'UTF-8')
new_data_dict = {
'web_server': {
'host': '127.0.0.1',
'port': '8080'
},
'user_information': {
'account': 'admin',
'password': '123'
}
}
config.write(new_data_dict)
data_dict = config.read()
print(data_dict)
print(data_dict['web_server']['port'])
update_data_dict = {
'host': 'localhost',
'port': '8888'
}
config.update('web_server', update_data_dict, new_section='new_web_server')
config.delete('new_web_server', 'host')