SpringBoot 结合 thymeleaf模板页面技术实战
1.thymeleaf
1.1 thymeleaf 介绍
- Thymeleaf是个XML/XHTML/HTML5模板引擎,可以用于Web与非Web应用。
- Thymeleaf的主要目标是提供一种可被浏览器正确显示的、格式良好的模板创建方式,因此也可以用作静态建模。
- 相对于编写逻辑或代码,开发者只需将标签属性添加到模板中即可。接下来,这些标签属性就会在DOM(文档对象模型)上执行预先制定好的逻辑。
- Thymeleaf的可扩展性非常棒:可以使用它定义自己的模板属性集合,这样就可以计算自定义表达式并使用自定义逻辑。
1.2 相关依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
1.3 标签
- 当 HTML 页面上使用到 thymeleaf 时,需要添加标签,页面加上:
<html xmlns:th="http://www.thymeleaf.org">
2.项目实战
2.1 配置文件配置
server:
port: 8888
spring:
application:
name: springboot-mybatis
datasource:
driver-class-name: com.mysql.jdbc.Driver
url: jdbc:mysql://192.168.159.133:3306/springbootdata?useUnicode=true&characterEncoding=UTF-8&serverTimezone=UTC
username: liuyunfei
password: Lyf123456!
type: com.alibaba.druid.pool.DruidDataSource
redis:
host: 192.168.159.133
port: 6379
database: 0
jedis:
pool:
max-active: 10
max-wait: 6000
max-idle: 5
min-idle: 2
timeout: 5000
thymeleaf:
cache: true
encoding: UTF-8
mode: HTML5
prefix: classpath:/templates/
suffix: .html
CommentList:
name: commentList
mybatis:
configuration:
map-underscore-to-camel-case: true
mapper-locations: classpath:mapper/*.xml
type-aliases-package: com.lagou.entity
2.2 提供服务的 controller
package com.lagou.controller;
import com.alibaba.fastjson.JSON;
import com.lagou.dao.CommentDao;
import com.lagou.entity.Comment;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.servlet.ModelAndView;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
@RestController
@RequestMapping(path = "/comment", produces = "application/json;charset=UTF-8")
public class CommentController {
@Autowired
private CommentDao commentDao;
@Autowired
private RedisTemplate<String, String> redisTemplate;
@Value("${CommentList.name}")
private String commentListName;
@RequestMapping("/{id}")
public Comment getCommentById(@PathVariable("id") Integer id){
Object o = redisCache(String.valueOf(id));
Comment comment = null;
if(o != null){
comment = JSON.parseObject(String.valueOf(o), Comment.class);
}
System.out.println("输入 id:" + id);
System.out.println("查询结果:" + comment);
return comment;
}
@RequestMapping("/all")
public List<Comment> getAll(){
Object o = redisCache(String.valueOf(Integer.MAX_VALUE));
List<Comment> commentList = null;
if (o != null){
commentList = JSON.parseArray(String.valueOf(o), Comment.class);
}
return commentList;
}
private Object redisCache(String id){
Boolean b1 = redisTemplate.hasKey(commentListName);
if (!b1){
List<Comment> commentList = commentDao.findAll();
Map<String, String> commentMap = new LinkedHashMap<>();
for (Comment comment: commentList){
commentMap.put(String.valueOf(comment.getId()), JSON.toJSONString(comment));
}
commentMap.put(String.valueOf(Integer.MAX_VALUE), JSON.toJSONString(commentList));
redisTemplate.opsForHash().putAll(commentListName, commentMap);
}
b1 = redisTemplate.opsForHash().hasKey(commentListName, id);
if (b1){
return redisTemplate.opsForHash().get(commentListName, id);
} else {
return null;
}
}
}
2.3 提供页面访问的 controller
@RequestMapping(path = "/index")
public ModelAndView toIndex(ModelAndView modelAndView, HttpServletRequest request, HttpServletResponse response){
Object o = redisCache(String.valueOf(Integer.MAX_VALUE));
List<Comment> commentList = null;
if (o != null){
commentList = JSON.parseArray(String.valueOf(o), Comment.class);
}
modelAndView.addObject("username", request.getParameter("username"));
modelAndView.addObject("commentList", commentList);
modelAndView.setViewName("index");
return modelAndView;
}
2.4 编写数据页 HTML
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org"><head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1,shrink-to-fit=no">
<title>评论首页</title>
</head>
<body>
<div style="background-color: #ffbc54; width: 500px;height: 30px">
<span style="float:left;color: blue">评论管理系统</span>
<span style="float:right;color: red" th:text="'登录用户:' + ${username}">匿名用户</span>
</div>
<div style="background-color: #196373; width: 500px">
<h3>评论列表</h3>
<ol type="1" start="1">
<li th:each="comment:${commentList}">
<p th:text="${comment.toString()}">评论信息</p>
</li>
</ol>
</div>
<div style="background-color: #067a12; width: 500px">
<h3>评论表格</h3>
<table border="1px" width="400px">
<thead>
<tr>
<td>id</td>
<td>content</td>
<td>author</td>
<td>aId</td>
</tr>
</thead>
<thead>
<tr th:each="comment:${commentList}">
<td th:text="${comment.getId()}"></td>
<td th:text="${comment.getContent()}"></td>
<td th:text="${comment.getAuthor()}"></td>
<td th:text="${comment.getAId()}"></td>
</tr>
</thead>
</table>
</div>
</body>
</html>
3.项目启动及测试
3.1 项目启动
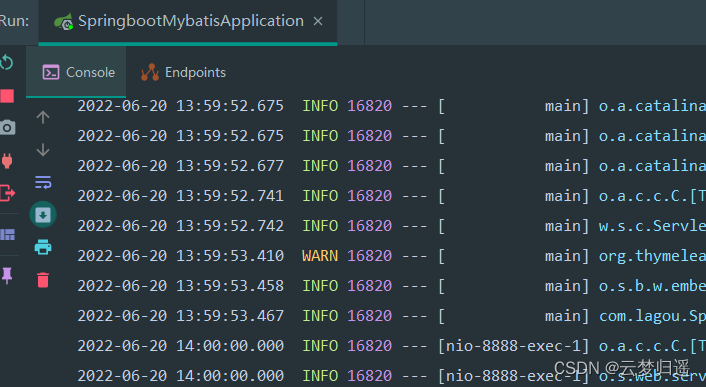
3.2 浏览器访问服务接口进行测试
3.3 浏览器访问 html 页面服务接口
3.4 查看 Linux 服务器上 Redis 服务是否缓存成功数据
127.0.0.1:6379> keys *
1) "commentList"
127.0.0.1:6379> hgetall "commentList"
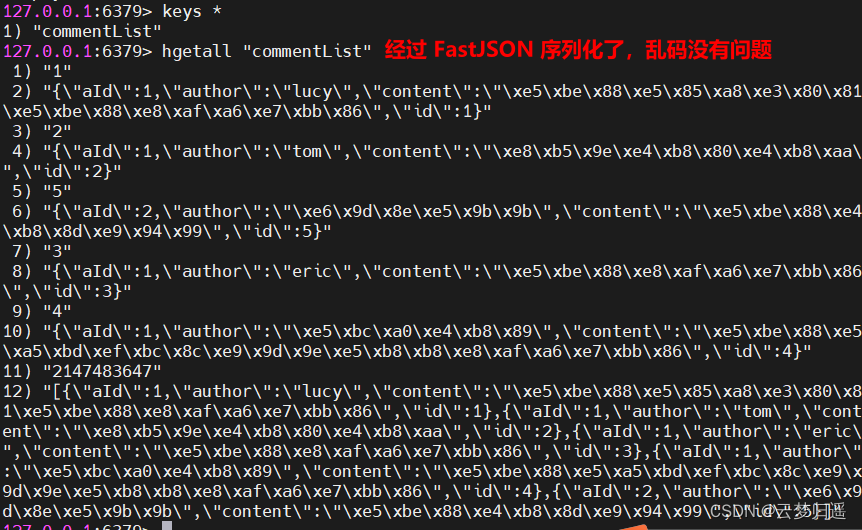
4.总结
- MyBatis 负责对应数据库进行数据查询
- RedisTemplate 负责对接 Redis 进行数据缓存,减小 DB 的压力
- ModelAndView 负责存储模板页面需要的数据,动态加载到 thymeleaf 中进行动态展示