Jquery介绍
1.jQuery是一个封装的库,也可以称为一个框架。将js中复杂的方法进行了封装改良,让开发人员更加专注于逻辑开发
2.jQuery做好了兼容,但是仅仅封装了DOM操作,操作不了bom
注意:前面两个是js和jQuery的区别
3.jQuery使用时必须进行引入(必须引入在自己的js之前,建议引入min)
引入本地的js文件
cdn加载(引入在线网址上 网址上具有框架)
4.没有DOM,需要用原生js写
5.在线手册:https://jquery.cuishifeng.cn/?from=singlemessage
<body>
<!-- 引入本地jquery -->
<script src="./jquery-3.5.1/jquery-3.5.1.min.js"></script>
<!-- 引入网上的 -->
<!-- <script src="https://code.jquery.com/jquery-3.5.1.min.js"></script> -->
<script type="text/javascript">
console.log(jQuery)//如果有表示引入成功
// 在jQuery中可以使用$代替jQuery
console.log($===jQuery)//true
// 方式一
// 入口文件 允许存在多个(不推荐)
$(document).ready(function(){
console.log(111)//111
})
// 方式二 (推荐使用,可以多个)
$(function(){
// 要求所有的jQuery代码均需要放在入口文件之内
console.log(333)//333
})
</script>
</body>
jQuery选择符
区别
不用用jQuery 用jQuery
document.queSeletorAll('div') $('div')
this $(this)
<body>
<div id="box">第一次杰出</div>
<ul>
<li>1111111</li>
<li>1111111</li>
<li>1111111</li>
<li>1111111</li>
<li>1111111</li>
<li>1111111</li>
<li>1111111</li>
</ul>
<h1>昨天巴南有人查出新冠</h1>
<h2>昨天巴南有人查出新冠</h2>
<h3>昨天巴南有人查出新冠</h3>
<h4>昨天巴南有人查出新冠</h4>
<h5>昨天巴南有人查出新冠</h5>
<h6>昨天巴南有人查出新冠</h6>
<script src='jquery-3.5.1/jquery-3.5.1.min.js'>
</script>
<script type="text/javascript">
// 原生js代码
// var box=document.querySelector('#box');
// box.style.color='red';
// 利用jQuery
$(function(){
$('#box').css('color','green');
$('ul li:first').css('color','red')//第一个li变色
$('ul li:last').css('color','red')//第一个li变色
})
// :lt(index)小于指定的值,index从0计数
$('ul li:lt(2)').css('border','1px solid black')
// :gt(index)大于指定的值,index从0计数
$('ul li:gt(3)').css('border','1px solid blue')
// :even匹配所有偶数,从下标0开始计数(重要)
$('ul li:even').css('color','gray')
// :odd 匹配所有的奇数行,下标从0开始计数(重要)
$('ul li:odd').css('color','pink')
// :eq(index)找到指定的某一个元素
$('ul li:eq(1)').css('background','orange')
// :header选择所有的标题标签
$(':header').css('color','orange')
</script>
</body>
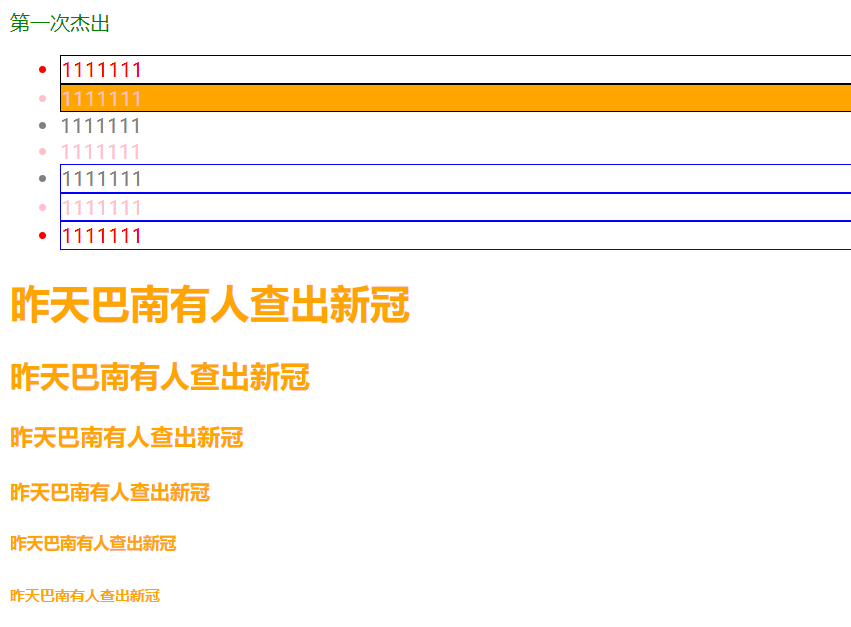
基本选择器
常用
1.选择器
#id
element
class
*
selector1,selector2,selector3
2.层级
a b
a>b
a+b(相邻的)
a~b(兄弟)
3.基本筛选器
:first
:eq(index)
:last
:even
:odd
:lt(index)
:gt(index)
4.表单
:input
:password
属性选择符
css的使用
css()获取或则设置css样式
设置多个
设置单个
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
div{
width: 300px;
height: 300px;
background: green;
}
</style>
</head>
<body>
<div></div>
<script src="jquery-3.5.1/jquery-3.5.1.min.js"></script>
<script type="text/javascript">
$(function(){
// css()获取或则设置css样式
console.log($('div').css('width'))//300px
console.log($('div').css('height'))//300px
// 设置css样式 单个样式(不推荐)
$('div').css('position','relative').css('left','200px').css('top','200px')//div位置移动了
// 同时设置多个css样式 写对象
$('div').css({background:'orange',bordeRadius:'50%',border:'1px solid black'})
})
</script>
</body>
</html>
筛选
过滤(常用)
eq
first
last
is(value)与hasClass等价的,就是写法不同
hasClass(value)判断是否具有特定的class类,返回boolean值,只能判断有没有类名
链式操作
这五个是重点(常用)
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
</head>
<body>
<ul>
<li class="active">1111</li>
<li>1112</li>
<li>1113</li>
<li>1114</li>
<li>1115</li>
</ul>
<script src="jquery-3.5.1/jquery-3.5.1.min.js"></script>
<script>
$(function(){
// 链式操作(方便切换对象)
$('ul li').css({width:'300px',background:'gray',margin:'1px 0px'}).first().css('background','green').html('你变了')
$('ul li').last().css('background','pink');
$('ul li').eq(1).css('background','red');
// hasClass()判断是否具有特定的class类,返回boolean值,只能判断有没有类名
var res=$('ul li').hasClass('active');
console.log(res)//true
// is()与hasClass等价的,就是写法不同
var res=$('ul li').first().is('.active');
console.log(res)//true
console.log($('ul li').parent().is('ul'))//true
// map循环遍历出来
$('ul li').map(function(){
var value=$(this).html()
console.log(value)
})
//遍历每个li,因为数组自动绑定全部元素
$('ul li').click(function(){
console.log($(this).html())
})
})
</script>
</body>
</html>
查找
1.sibling()找到除了自己的所有兄弟(轮播图可以用) 经常用
2.查找子元素
find()找到自己所包含的所有元素
children()找到自己的子元素(不包括孙子)
3.查找前一个
prev()相当于previousSibling 紧邻这个元素之前的(同等级)
prevAll()找到之前所有的
prevUntil()找到直到什么为止
4.查找下一个
next() 相当于nextSibling 紧邻这个元素之后的(同等级)
nextAll()之后所有的
nextUntil()直到某个元素为止
5.查找父级
parent()第一个父亲
partents()所有的父亲
partentUntil()直到什么为止
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
</head>
<body>
<div id="box">
<p>1111</p>
<b><p>2222</p></b>
</div>
<ul>
<li>111</li>
<li>111</li>
<li class="active">111</li>
<li>111</li>
<li>111</li>
</ul>
<script src="jquery-3.5.1/jquery-3.5.1.min.js"></script>
<script type="text/javascript">
$(function(){
console.log($('div').children())
// children()查找指定的子元素
var res=$('div').children('p')
console.log(res)//<p>11111</p>
// find()查找指定的某个元素,包含关系
$('div').find('p').css('background','red');
// next() 相当于nextSibling
$('ul li:first').next().css('color','red')
$('ul li:first').nextAll().css('background','lightblue')
$('ul li:first').nextUntil('.active').css('background','pink')
var res=$('ul li').parents();
console.log(res)
var res=$('ul li').parentUntil('html');
console.log(res)
// prev()相当于previousSibling
$('ul li:last').prev().css('color','red')
$('ul li:last').prevAll().css('background','lightblue')
$('ul li:last').prevUntil('.active').css('background','pink')
// siblings()查找所有的兄弟
$('ul li.active').siblings().css('background','#00FFFF')
})
</script>
</body>
</html>
串联
end(回到上一个破坏性操作)(推荐)
用的parent()和find()(太复杂)
<body>
<ul>
<li>111111</li>
<li>111111</li>
<li>111111</li>
<li>111111</li>
</ul>
<script src="../0817/jquery-3.5.1/jquery-3.5.1.min.js"></script>
<script type="text/javascript">
$(function(){
// end(回到上一个破坏性操作)(推荐)
$('ul li').first().css('color','red').end().last().css('color','green').end().eq(2).css('color','blue')
// 用的parent()和find()(太复杂)
// $('ul li').first().css('color','red').parent().find('li').last().css('color','lightblue')
})
</script>
</body>
属性的操作(最重要)
1.attr()获取、修改、设置属性(推荐用)
attr(key)获取指定属性的值
attr(key,value) 当属性名不存在,则为添加属性
attr(key,value)当属性名存在,则为修改属性
2.removeAttr(key)移除指定的属性节点
3.prop()不推荐用,因为有很多限制
4.prop()获取属性值,只能获取集合中的第一个
prop(key)获取指定属性的值
prop(key,value)当属性名不存在,则为添加属性
prop(key,value)当属性名存在,则为修改属性
removeProp(key,value)移除属性
用attr
例子
<body>
<p name="text" id="one">有空多复习</p>
<p name="text" id="two">有空多复习</p>
<p name="text" id="three">有空多复习</p>
<script src="../0817/jquery-3.5.1/jquery-3.5.1.min.js"></script>
<script type="text/javascript">
$(function(){
// attr()获取、修改、设置属性(推荐用)
// attr(key)获取指定属性的值
console.log($('p').attr('name'))//text
// attr(key,value) 当属性名不存在,则为添加属性
$('p').attr('class','active')//增加属性
// attr(key,value)当属性名存在,则为修改属性
$('p').attr('name','txt')
// removeAttr(key)移除指定的属性节点
setTimeout(function(){
$('p').removeAttr('id')
},1000)
})
</script>
</body>
用prop
的例子
<body>
<p name="text" id="one">有空多复习</p>
<p name="text" id="two">有空多复习</p>
<p name="text" id="three">有空多复习</p>
<script src="../0817/jquery-3.5.1/jquery-3.5.1.min.js"></script>
<script type="text/javascript">
$(function(){
// prop()不推荐用
// prop()获取属性值,只能获取集合中的第一个
console.log($('p').prop("id"))//one
// 增加属性
// $('p').prop('class','active')
// 里面可以写对象
$('p').prop({class:'active'})
// 修改属性
$('p').prop('id','txt')
// 移除不了
setTimeout(function(){
$('p').removeProp('class','active')
})
})
</script>
</body>
操作Class
Class的操作(最重要)
addClass(value)
removeClass(value)
toggleClass(value) 在不知道状态的时候用
切换class样式 若存在,则删除,若不存在,则添加
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
*{margin:0;padding:0;}
ul{
list-style:none;
display:flex;
/* 让远点居中 */
justify-content:center;
}
ul>li{
width:20px;
height:20px;
border-radius:50%;
color:white;
background:#000000;
text-align:center;
margin:3px;
}
ul>li.active{
background:darkred;
}
</style>
</head>
<body>
<ul>
<li class="active">1</li>
<li>2</li>
<li>3</li>
<li>4</li>
</ul>
<script src="../0817/jquery-3.5.1/jquery-3.5.1.min.js"></script>
<script>
$(function(){
$('ul li').click(function(){
$(this).addClass('active').siblings().removeClass('active')
})
})
</script>
</body>
</html>
切换class样式
方式一:
用hasClass和removeClass(value)或则addClass(value)切换
方式二:
toggleClass(value)
切换class样式 若存在,则删除,若不存在,则添加
用toggleClass(value) ----推荐使用(简单)
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style type="text/css">
p{
color: green;
}
.active{
color: red;
}
</style>
</head>
<body>
<button>点击切换</button>
<p>明天开始检查健康码</p>
<script src="../0817/jquery-3.5.1/jquery-3.5.1.min.js"></script>
<script type="text/javascript">
$(function(){
$('button').click(function(){
// 方式一 切换class样式
// var res=$('p').hasClass('active');
// if(res){
// $('p').removeClass('active');
// }else{
// $('p').addClass('active');
// }
// 方式二 切换class样式 若存在,则删除,若不存在,则添加
$('p').toggleClass('active');
})
})
</script>
</body>
</html>
文本值操作
html() 相当于innerHTML
text() 相当于innerText
html() text()获取和设置值
val()获取输入框、文本框、下拉框的值
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
</head>
<body>
<div>
<p>段落中的文字</p>
</div>
<input type="text"/>
<script src="../0817/jquery-3.5.1/jquery-3.5.1.min.js"></script>
<script type="text/javascript">
$(function(){
// html() 相当于innerHTML
// html() text()获取和设置值
var text=$('div').html();
console.log(text)//<p>段落中的文字</p>
var text=$('div').text();
console.log(text)//段落中的文字
// $('div').text('text设置的字')
$('div').html('html设置的字')
// val()获取输入框、文本框、下拉框的值
$('input').blur(function(){
var value=$('input').val()
console.log(value)
})
})
</script>
</body>
</html>
位置的获取
offset()获取当前对象相对于可视口(client)的偏移,返回一个对象,包含两个属性,分别为left,top
positon获取相对父元素的偏移,返回一个对象,包含两个属性,分别为left,top
scrollTop()和scrollLeft获取滚动条到顶部和左侧的距离
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style type="text/css">
*{
margin: 0;
padding: 0;
}
body{
width: 200%;
height: 3000px;
background: #eee;
}
div.d1{
width: 500px;
height: 500px;
background: orange;
margin: 50px 100px;
position: relative;
}
div.d2{
width: 300px;
height: 300px;
background: #0000FF;
/* 设置盒子居中
1.设置父级定位
2.设置自己定位和margin:auto、四个方向的值
*/
position: absolute;
margin: auto;
left: 0;
top: 0;
right: 0;
bottom: 0;
}
div.d3{
width: 200px;
height: 200px;
background: green;
position: absolute;
left: 600px;
top:100px
}
</style>
</head>
<body>
<div class="d1">
<div class="d2"></div>
</div>
<div class="d3"></div>
<script src="../../0817/jquery-3.5.1/jquery-3.5.1.min.js"></script>
<script type="text/javascript">
$(function(){
// offset获取距离视口的相对偏移
console.log($('div.d1').offset())
console.log($('div.d1').offset().left)
console.log($('div.d1').offset().top)
// position()获取相对父级的偏移
console.log($('div.d3').position())
console.log($('div.d3').position().left)
console.log($('div.d3').position().top)
// scrollTop()和scrollLeft获取滚动条距离底部和左侧的距离
$(document).scroll(function(){
console.log($(document).scrollTop())
console.log($(document).scrollLeft())
})
})
</script>
</body>
</html>
尺寸的获取
width():获取元素的宽度
height():获取元素的高度
innerWidth():获取元素内部宽度(包括补白,不包括边框)
innerHeight():获取元素内部高度(包括补白,不包括边框)
outerWidth():获取元素外部宽度(包括补白边框)
outerHeight():获取元素外部高度(包括补白边框)
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style type="text/css">
div{
width: 200px;
height: 300px;
background: orange;
}
</style>
</head>
<body>
<div></div>
<script src="../0817/jquery-3.5.1/jquery-3.5.1.min.js"></script>
<script type="text/javascript">
$(function(){
console.log($('div').width())//200
console.log($('div').height())//300
console.log($(document).innerWidth())//获取元素内部宽度(包括补白,不包括边框)
console.log($(document).innerHeight())
console.log($(document).outerWidth())//获取元素外部宽度(包括补白边框)
console.log($(document).outerHeight())
})
</script>
</body>
</html>
效果
显示隐藏
show(speed,切换效果,回调函数):显示
speed:slow normal fast 也可以写毫秒数
hide(speed,切换效果,回调函数):隐藏
speed:slow normal fast 也可以写毫秒数
toggle(speed,切换效果,回调函数):切换
speed:slow normal fast 也可以写毫秒数
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
div{
width: 200px;
height: 200px;
background: green;
}
</style>
</head>
<body>
<button>显示show</button>
<button>隐藏hide</button>
<button>切换toggle</button>
<div></div>
<script src="../0817/jquery-3.5.1/jquery-3.5.1.min.js"></script>
<script type="text/javascript">
$(function(){
// 显示
$('button').eq(0).click(function(){
/*
speed:slow normal fast
*/
// $('div').show();
// $('div').show('normal');
// $('div').show(1000);
$('div').show(1000,'linear',function(){
console.log('显示完全了才触发这个函数')
});
})
// 隐藏
$('button').eq(1).click(function(){
/*
speed:slow normal fast
*/
$('div').hide();
})
// 切换
$('button').eq(2).click(function(){
$('div').toggle();
})
})
</script>
</body>
</html>
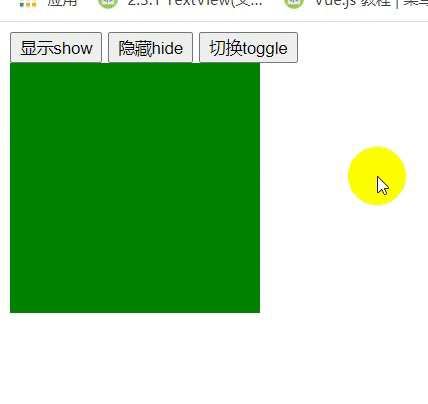
滑动
slideDown():高度增加
slideUp():高度减小
slideToggle():高度减小和增加之间切换
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
div{
width: 200px;
height: 200px;
background: green;
}
</style>
</head>
<body>
<button>slideDown</button>
<button>slideUp</button>
<button>slideToggle</button>
<div></div>
<script src="../0817/jquery-3.5.1/jquery-3.5.1.min.js"></script>
<script type="text/javascript">
$(function(){
// 高度增加slideDown()
$('button').eq(0).click(function(){
$('div').slideDown()//高度增加
})
// 高度减小slideUp()
$('button').eq(1).click(function(){
$('div').slideUp()//高度减小
})
// 切换slideToggle()
$('button').eq(2).click(function(){
$('div').slideToggle();
})
})
</script>
</body>
</html>
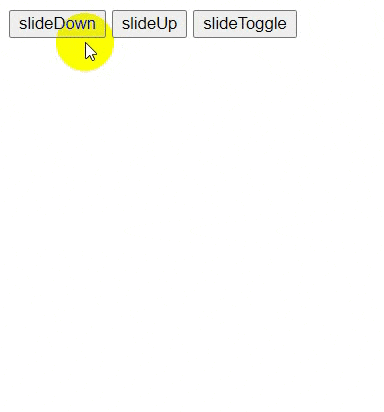
index()
直接当前操作的下标
淡入淡出
fadeIn(speed,easing,fn):淡入,出现
fadeOut(speed,easing,fn):淡出:消失
fadeTo(speed,opcity,easing,fn):匹配元素的不透明度以渐进方式调整到指定的不透明度
fadeToggle(speed,easing,fn):淡入淡出之间切换
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
div{
width: 200px;
height: 200px;
background: green;
display: none;
}
</style>
</head>
<body>
<button>fadeIn</button>
<button>fadeOut</button>
<button>fadeTo</button>
<button>fadeToggle</button>
<div></div>
<script src="../../0817/jquery-3.5.1/jquery-3.5.1.min.js"></script>
<script type="text/javascript">
$(function(){
$('button').eq(0).click(function(){
$('div').fadeIn(1000)//1秒后出现
})
$('button').eq(1).click(function(){
$('div').fadeOut(1000)//1秒后消失
})
$('button').eq(2).click(function(){
$('div').fadeTo(1000,0.5)//1秒后变为透明度为0.5
})
$('button').eq(3).click(function(){
$('div').fadeToggle(1000)//出现和消失之间切换
})
})
</script>
</body>
</html>
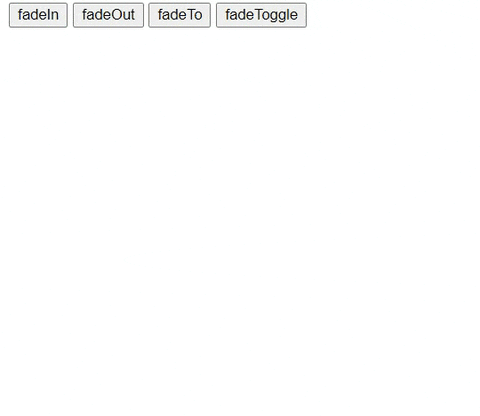
动画animate()
animate(params,[speed],[easing],[fn])
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
div{
width: 200px;
height: 200px;
background: green;
position: absolute;
left: 0;
top: 30px;
}
</style>
</head>
<body>
<button>开始动画</button>
<button>结束动画</button>
<div></div>
<script src="../../0817/jquery-3.5.1/jquery-3.5.1.min.js"></script>
<script type="text/javascript">
$(function(){
$('button').click(function(){
// $('div').animate({borderRadius:'50%',left:'300px'},1000)//1秒完成变圆和右移动
$('div').animate({borderRadius:'50%'},1000).delay(500).animate({left:'300px'},1000)//1秒完成变圆,停留0.5秒,1秒完成移动
})
})
</script>
</body>
</html>
停止stop和完成finish
1.stop()
stop()结束当前正在执行的动画,立即执行下一个动画,这个例子是停止变圆,立即执行移动 相当于stop(false,false)
stop(false,true)//立即完成当前动画,立即执行下一动画 这里是直接变圆,继续移动
stop(true)//立即结束当前当前动画,取消下一个动画 相当于stop(true,false)
stop(true,true)立即完成当前动画,取消下一个动画
总结:
第一个参数:false则为立即执行下一个动画,true则为立即取消下一个动画
第二个参数:false则为立即结束当前正在执行的动画,true立即完成当前执行的动画
2.finish()
清空所有动画,直接将所有动画定义完成
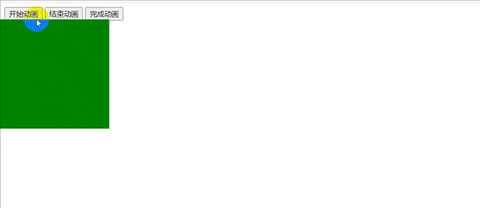
对象访问
1.each(function)以每一个匹配的元素作为上下文来执行一个函数
2.length获取长度
3.get()获取指定下标的元素,0开始,返回DOM元素 可以转化为js对象
jQuery对象转换为js对象
var oli=$('ul li').get(1);
oli.style.color='green';
4.DOM转换为jQuery对象
var h1=document.querySelector('h1');
var $h1=$('h1');
$h1.css('color','red')
5.data()暂时给标签设置个index数据,不是js的缓存
<script type="text/javascript">
$(function(){
// each()
$('ul li').each(function(index,value){
console.log(index)//0 1 2 3
console.log(value)//111 2222 3333 4444
})
// length获取长度
console.log($('ul li').length)//4
$('ul li').eq(0).css('color','red');
// jQuery对象转换为js对象 get()获取指定下标的元素,0开始,返回DOM元素、
var oli=$('ul li').get(1);
oli.style.color='green';
// DOM转换为jQuery对象
var h1=document.querySelector('h1');
var $h1=$('h1');
$h1.css('color','red')
// data()暂时给标签设置个index数据,不是js的缓存
$('h1').data('index',2)
console.log($('h1').data('index'))
// toArray()转为一个DOM数组
console.log($('ul li').toArray())
})
</script>
文档处理
内部插入
1.append(content|fn)在内部追加内容 相当于appendChild
2.appendTo(content)将所有匹配到的元素追加到指定集合中
3.prepend(content|fn)在匹配元素内部前置内容
4.prependTo(content)把所有匹配的元素前置到另一个、指定的元素元素集合中。
<body>
<ul></ul>
<div>
<h1>重大通知</h1>
</div>
<li>今天不上晚自习</li>
<li>今天不上晚自习</li>
<li>今天不上晚自习</li>
<li>今天不上晚自习</li>
<script src="../0817/jquery-3.5.1/jquery-3.5.1.min.js"></script>
<script type="text/javascript">
$(function(){
// append()在内部追加内容 相当于appendChild
$('li').append('联合会')//今天不上晚自习联合会
// appendTo()将所有匹配到的元素追加到指定集合中
$('li').appendTo($('ul'))//li到ul中了
// prepend()在匹配元素内部前置内容
$('li').prepend('姐说:')//姐说:今天不上晚自习
// prependTo()把所有匹配的元素前置到另一个、指定的元素元素集合中。
$('li').prependTo($('div'))
})
</script>
</body>
外部插入
1.after(content|fn)在每个匹配的元素之后插入内容。
2.before(content|fn)在每个匹配的元素之前插入内容。
3.insertAfter(content)把所有匹配的元素插入到另一个、指定的元素元素集合的后面。
4.insertBefore(content)把所有匹配的元素插入到另一个、指定的元素元素集合的前面。
<body>
<div></div>
<ul>
<li>111111</li>
<li>111111</li>
<li>111111</li>
<li>111111</li>
</ul>
<script src="../0817/jquery-3.5.1/jquery-3.5.1.min.js"></script>
<script type="text/javascript">
$(function(){
$('ul li').after('<input />')//li后面加了input
$('ul li').before('<input />')//li前面加了input
$('ul li').insertAfter($('div'))
$('ul li').insertBefore($('div'))
})
</script>
</body>
包裹
1.wrap(html|element|fn)给所有匹配的元素多个元素包裹起来
2.unwrap()给匹配的元素去掉包裹
3.wrapAll(html|ele)将所有匹配的元素用单个元素包裹起来
4.wrapInner(htm|element|fnl)将每一个匹配的元素的子内容(包括文本节点)用一个HTML结构包裹起来
<body>
<li>111111</li>
<li>111111</li>
<li>111111</li>
<li>111111</li>
<script src="../0817/jquery-3.5.1/jquery-3.5.1.min.js"></script>
<script type="text/javascript">
$(function(){
// wrap()给所有匹配的元素多个元素包裹起来
$('li').wrap('<ul></ul>')
// 给匹配的元素去掉包裹
$('li').unwrap();
// 将所有匹配的元素用单个元素包裹起来
$('li').wrapAll('<ul></ul>')
// wrapInner()将每一个匹配的元素的子内容(包括文本节点)用一个HTML结构包裹起来
$('li').wrapInner('<a></a>')
})
</script>
</body>
替换
1.replaceWith(content|fn)将所有匹配的元素替换成指定的HTML或DOM元素。 用这种
2.replaceAll(selector)用匹配的元素替换掉所有 selector匹配到的元素。
<body>
<p>111</p>
<p>111</p>
<p>111</p>
<p>111</p>
<script src="../0817/jquery-3.5.1/jquery-3.5.1.min.js"></script>
<script type="text/javascript">
$(function(){
$('p').replaceWith('<h2>2222222</h2>');
$('<h3>333333</h3>').replaceAll('h2');
})
</script>
</body>
删除和克隆
1.empty()清空匹配的元素的内容
2.remove([expr])移除所有匹配的元素
3.detach([expr])删除匹配的元素
4.clone()
<body>
<p>111</p>
<p>111</p>
<p>111</p>
<p>111</p>
<script src="../0817/jquery-3.5.1/jquery-3.5.1.min.js"></script>
<script type="text/javascript">
// empty()清空匹配的元素的内容
$('p').empty();//文本清空了,标签还在
// 移除所有匹配的元素
$('p').remove();
// 删除匹配的元素
$('p').detach()
$('div').clone().appendTo('body')
</script>
</body>
事件
事件处理
1.on(events,[selector],[data],fn)绑定一个或则多个事件
2.off(events,[selector],[fn])取消on的事件
<body>
<button>按钮</button>
<script src="../../0817/jquery-3.5.1/jquery-3.5.1.min.js"></script>
<script type="text/javascript">
$(function(){
function listener1{
console.log(111)
}
function listener2{
console.log(222)
}
// on()绑定一个或则多个事件
$('button').on('click mouseover',listener1)
// off()取消on的事件
setTimeout(function(){
$('button').off('mouseover',listener1)
},2000)
$('button').one('click',listener2)
})
</script>
</body>
事件切换
hover([over,]out)鼠标移入和移除分别触发的函数
<body>
<div></div>
<script src="../../0817/jquery-3.5.1/jquery-3.5.1.min.js"></script>
<script type="text/javascript">
$(function(){
$('div').hover(function(){
// 鼠标移入触发
console.log(111)
},//鼠标移除触发
function(){
console.log(222)
}
)
})
</script>
</body>
补充
//css()获取或者设置css样式
console.log($('div').css('width'))//300px
//设置css ,单个样式
$('div').css('width','200px').css('background','lightblue')
//同时设置多个css样式
$('div').css({height:'200px',background:'orange',borderRadius:'50%'})
//链式操作 ,方便切换对象
$('ul li').css({width:'300px',background:'#ccc',margin:'1px 0px'}).first().css('color','red')
$('ul li').eq(2).css({background:'pink'})
//判断是否具有某个属性,返回一个布尔值
var res =$('ul li').hasClass('active')
console.log(res)
//is适用性更强
var res =$('ul li').is('.active')
console.log(res)
//map遍历
$('ul li').map(function(){
var value=$(this).html()
console.log(value)
})
//循环点击事件
$('ul li').click(function(){
console.log($(this).html())
})
$('button').off('mouseover',listener1)
},2000)
$('button').one('click',listener2)
})
</script>
```
事件切换
hover([over,]out)鼠标移入和移除分别触发的函数
<body>
<div></div>
<script src="../../0817/jquery-3.5.1/jquery-3.5.1.min.js"></script>
<script type="text/javascript">
$(function(){
$('div').hover(function(){
// 鼠标移入触发
console.log(111)
},//鼠标移除触发
function(){
console.log(222)
}
)
})
</script>
</body>
补充
//css()获取或者设置css样式
console.log($('div').css('width'))//300px
//设置css ,单个样式
$('div').css('width','200px').css('background','lightblue')
//同时设置多个css样式
$('div').css({height:'200px',background:'orange',borderRadius:'50%'})
//链式操作 ,方便切换对象
$('ul li').css({width:'300px',background:'#ccc',margin:'1px 0px'}).first().css('color','red')
$('ul li').eq(2).css({background:'pink'})
//判断是否具有某个属性,返回一个布尔值
var res =$('ul li').hasClass('active')
console.log(res)
//is适用性更强
var res =$('ul li').is('.active')
console.log(res)
//map遍历
$('ul li').map(function(){
var value=$(this).html()
console.log(value)
})
//循环点击事件
$('ul li').click(function(){
console.log($(this).html())
})