项目简介:
- 实现功能:开始、暂停、重置;
- 项目进行了模块化封装,易于修改界面;
- 暂停按钮需要长按1s或者点按,因为使用了Sleep()函数;
- 适合小白或者刚接触C++的EasyX图形化编程的小伙伴;
- 我会将源文件上传到百度网盘(链接在文末)和CSDN资源社区,供小伙伴自行下载;
- 注意事项:项目属性-》高级-》字符集-》使用多字节字符集;
- 关于EasyX库可自行搜索下载安装,否则部分代码会报错;
- 如有疑问或者下载链接失效,可评论留言,我将在第一时间为你解忧;
封装的类
class Button
{
public:
Button(int x, int y, int width, int length) :
x(x), y(y), width(width), length(length), white(WHITE)
{
this->str = new char[12];
}
int Draw();
int setColor(COLORREF);
char& setText(const char*,COLORREF);
bool Clicked(ExMessage);
protected:
int x;
int y;
int width;
int length;
char* str;
COLORREF white;
};
class Time
{
public:
Time() :hour(0), minute(6), second(0),SWITCH(false),btn(nullptr) {}
void outtext_Hour(int hour);
void outtext_Minute(int minute);
void outtext_Second(int second);
int& getHour();
int& getMinute();
int& getSecond();
bool& getSwitch();
int start();
protected:
int hour;
int minute;
int second;
bool SWITCH;
Button* btn;
};
class MyWin
{
public:
MyWin(int width, int length) :Win_width(width), Win_length(length) {}
int DrawWin();
protected:
int Win_width;
int Win_length;
};
- 这就是几个主要的类的封装,没有实现具体的功能,具体功能的实现我只展示部分主要的函数;
倒计时函数:
int Time::start()
{
Sleep(250);
if (this->hour >= 0 || this->minute >= 0 || this->second >= 0)
{
if (this->second >= 0 || this->minute >= 0 || this->hour >= 0)
{
if (this->second == 0 && this->minute > 0)
{
this->second = 60;
this->minute--;
}
if (this->second == 0 && this->minute <= 0 && this->hour > 0)
{
this->hour--;
this->minute = 59;
this->second = 60;
}
}
this->second--;
Sleep(500);
cout << this->hour << " : " << this->minute << " : " << this->second << endl;
if (this->hour <= 0 && this->minute <= 0 && this->second <= 0)
{
this->getHour() = 0;
this->getMinute() = 6;
this->getSecond() = 0;
this->getSwitch() = false;
this->SWITCH = false;
this_thread::sleep_for(3s);
}
Sleep(250);
}
return 0;
}
设置文字显示并居中
char& Button::setText(const char* str1,COLORREF color)
{
int str_length = strlen(str1) + 1;
strcpy_s(this->str, str_length, str1);
settextstyle(32, 0, "楷体");
settextcolor(color);
setbkmode(TRANSPARENT);
int textW = textwidth(this->str);
int textH = textheight(this->str);
int t_X = this->x + (this->width - textW) / 2;
int t_Y = this->y + (this->length - textH) / 2;
outtextxy(t_X, t_Y, this->str);
return *this->str;
}
判断按钮点击事件
bool Button::Clicked(ExMessage m)
{
if (m.message == WM_LBUTTONDOWN &&
m.x >= this->x && m.x <= this->x + this->width &&
m.y >= this->y && m.y <= this->y + this->length)
{
return true;
}
return false;
}
主函数逻辑部分
ExMessage m;
BeginBatchDraw();
while (1)
{
putimage(-10, 0, &img_bk);
while (peekmessage(&m, EM_MOUSE));
if (btn1->Clicked(m))
{
tim_1.getSwitch() = true;
cout << "click message..." << endl;
}
if (btn2->Clicked(m))
{
tim_1.getSwitch() = false;
cout << "stop message..." << endl;
}
if (btn3->Clicked(m))
{
tim_1.getHour() = 0;
tim_1.getMinute() = 6;
tim_1.getSecond() = 0;
tim_1.getSwitch() = false;
cout << "repeat time..." << endl;
}
if (tim_1.getSwitch())
{
tim_1.start();
}
settextcolor(LIGHTRED);
tim_1.outtext_Minute(tim_1.getMinute());
tim_1.outtext_Second(tim_1.getSecond());
btn1->setColor(YELLOW);
btn1->Draw();
btn1->setText("开始", RED);
btn2->setColor(RED);
btn2->Draw();
btn2->setText("暂停", YELLOW);
btn3->setColor(BLUE);
btn3->Draw();
btn3->setText("重置", WHITE);
FlushBatchDraw();
}
EndBatchDraw();
项目运行截图
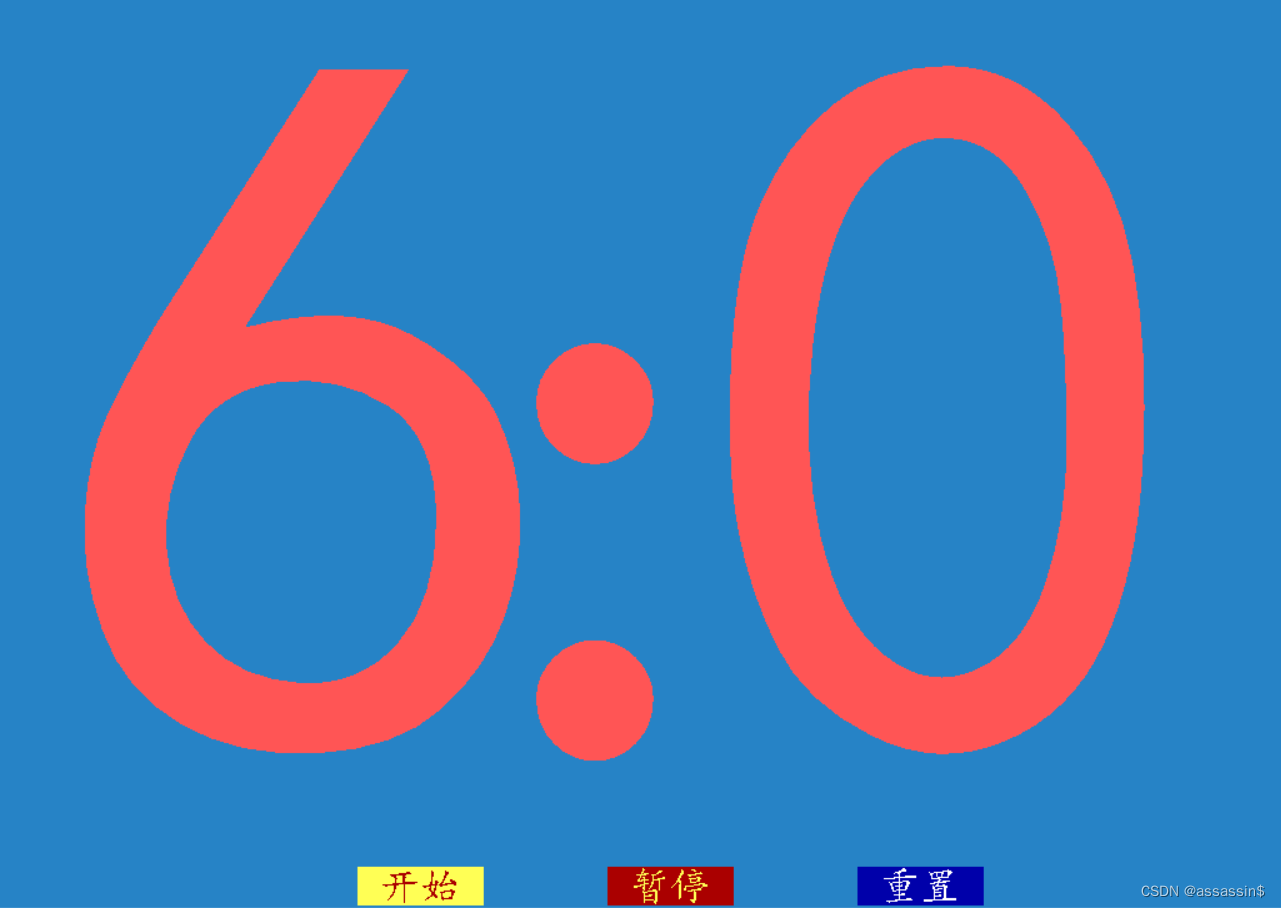
可将项目设置为如下以减小电脑负担

- 文件已上传至我的CSDN资源处,欢迎下载;
- 源代码网盘链接:链接: https://pan.baidu.com/s/1KrEz33EPLavtPFVsUpdi1Q?pwd=vmjr 提取码: vmjr;(如遇链接失效可评论留言)
- 注意:如有疑问欢迎留言讨论!!!其他的问题在我能力范围以内的也欢迎各位小伙伴私信或留言讨论!!!