一,显示商品分类
1.业务接口文档
- 请求路径: /itemCat/findItemCatList/{level}
- 请求类型: get
- 请求参数: level
参数名称 | 参数说明 | 备注 |
---|
level | 查询级别 | 1查询一级分类 2查询1-2 级商品分类 3查询1-2-3级商品分类 |
- 业务说明: 查询3级分类菜单数据 要求三层结构嵌套
- 返回值: SysResult对象
参数名称 | 参数说明 | 备注 |
---|
status | 状态信息 | 200表示服务器请求成功 201表示服务器异常 |
msg | 服务器返回的提示信息 | 可以为null |
data | 服务器返回的业务数据 | 3级商品分类信息 |
2.编辑ItemCatController
@GetMapping("/findItemCatList/{level}")
public SysResult findItemCatList(@PathVariable Integer level){
List<ItemCat> itemCatList = itemCatService.findItemCatList(level);
return SysResult.success(itemCatList);
}
3.编辑ItemCatService
@Override
@Transactional
public List<ItemCat> findItemCatList(Integer level) {
long startTime = System.currentTimeMillis();
QueryWrapper<ItemCat> queryWrapper = new QueryWrapper<>();
queryWrapper.le("level",level)
.orderByDesc("level");
List<ItemCat> itemCatList = itemCatMapper.selectList(queryWrapper);
int s = itemCatList.size();
int a = 0;
int b = 0;
ItemCat itemCat;
ItemCat itemCat1;
for (int i = 0; i < s; i++) {
itemCat = itemCatList.get(i);
if (itemCat.getLevel() > 1 ) {
for (int j = i + 1; j < s; j++) {
itemCat1 = itemCatList.get(j);
a = itemCat.getParentId();
b = itemCat1.getId();
if (a == b) {
List<ItemCat> itemCatList1 = new ArrayList<>();
if (itemCat1.getChildren() != null) {
itemCatList1 = itemCat1.getChildren();
}
itemCatList1.add(itemCat);
itemCat1.setChildren(itemCatList1);
}
}
}
}
List<ItemCat> itemCatList2 = new ArrayList<>();
for (ItemCat itemCat2 : itemCatList) {
if (itemCat2.getLevel() == 1) {
itemCatList2.add(itemCat2);
}
}
long endTime = System.currentTimeMillis();
System.out.println("耗时:"+(endTime-startTime));
return itemCatList2;
}
嵌套逻辑分析点这里!!!
4.实现效果
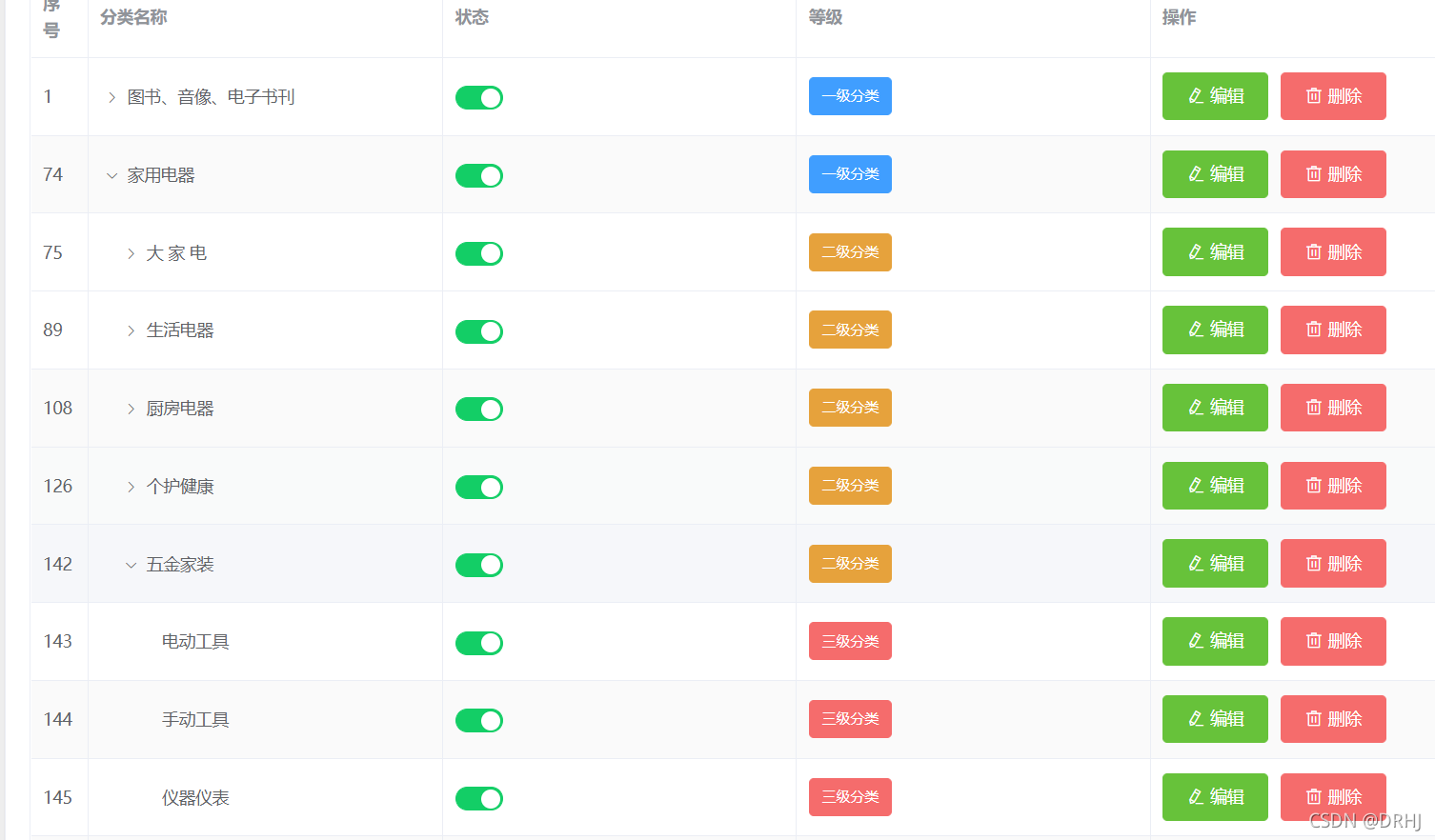
二,商品分类状态修改
1.业务分析
当用户点击状态码时,应该实现数据的修改操作.
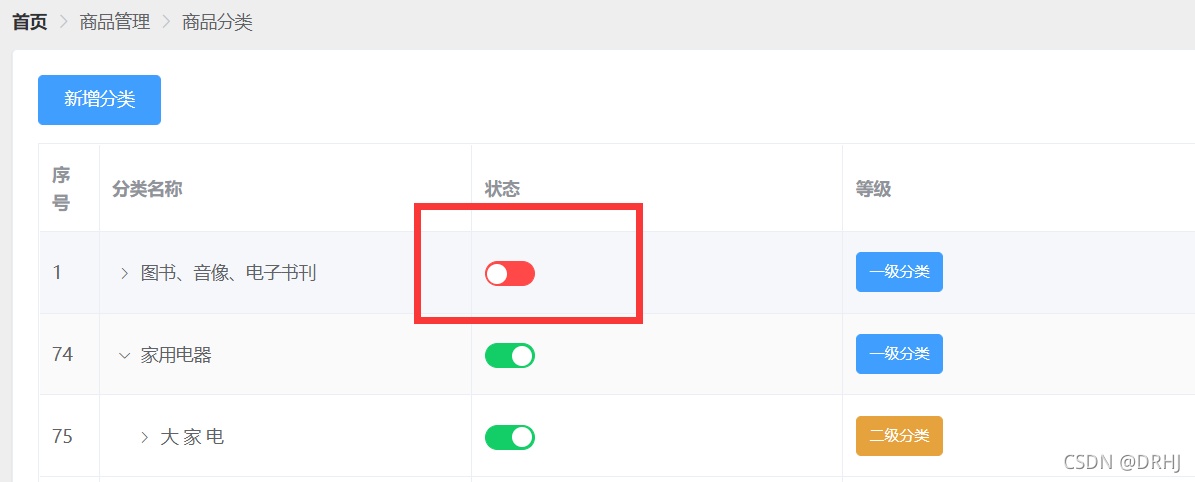
2.页面js分析
<el-table-column prop="status" label="状态">
<!-- 定义作用域插槽 展现数据 scope.row展现行级元素 -->
<template slot-scope="scope">
<el-switch v-model="scope.row.status" active-color="#13ce66" inactive-color="#ff4949"
@change="updateStatus(scope.row)"></el-switch>
</template>
</el-table-column>
async updateStatus(itemCat) {
const {
data: result
} = await this.$http.put(`/itemCat/status/${itemCat.id}/${itemCat.status}`)
if (result.status !== 200) return this.$message.error("修改状态失败")
this.$message.success("状态修改成功")
},
3.业务接口实现
- 请求路径: /itemCat/status/{id}/{status}
- 请求类型: put
- 请求参数:
参数名称 | 参数说明 | 备注 |
---|
id | 用户ID值 | 不能为null |
status | 用户的状态信息 | 不能为null |
参数名称 | 参数说明 | 备注 |
---|
status | 状态信息 | 200表示服务器请求成功 201表示服务器异常 |
msg | 服务器返回的提示信息 | 可以为null |
data | 服务器返回的业务数据 | 可以为null |
4.编辑ItemCatController
@PutMapping("/status/{id}/{status}")
public SysResult updateStatus(ItemCat itemCat){
itemCatService.updateStatus(itemCat);
return SysResult.success();
}
5.编辑ItemCatService
@Override
@Transactional
public void updateStatus(ItemCat itemCat) {
itemCatMapper.updateById(itemCat);
}
三,新增
1.业务说明
商品分类实现中,需要添加一级/二级/三级分类信息. 但是父级下拉框中勾选1-2级菜单. 因为三级菜单不能当作父级. 当用户编辑完成之后,点击确定实现商品分类入库.
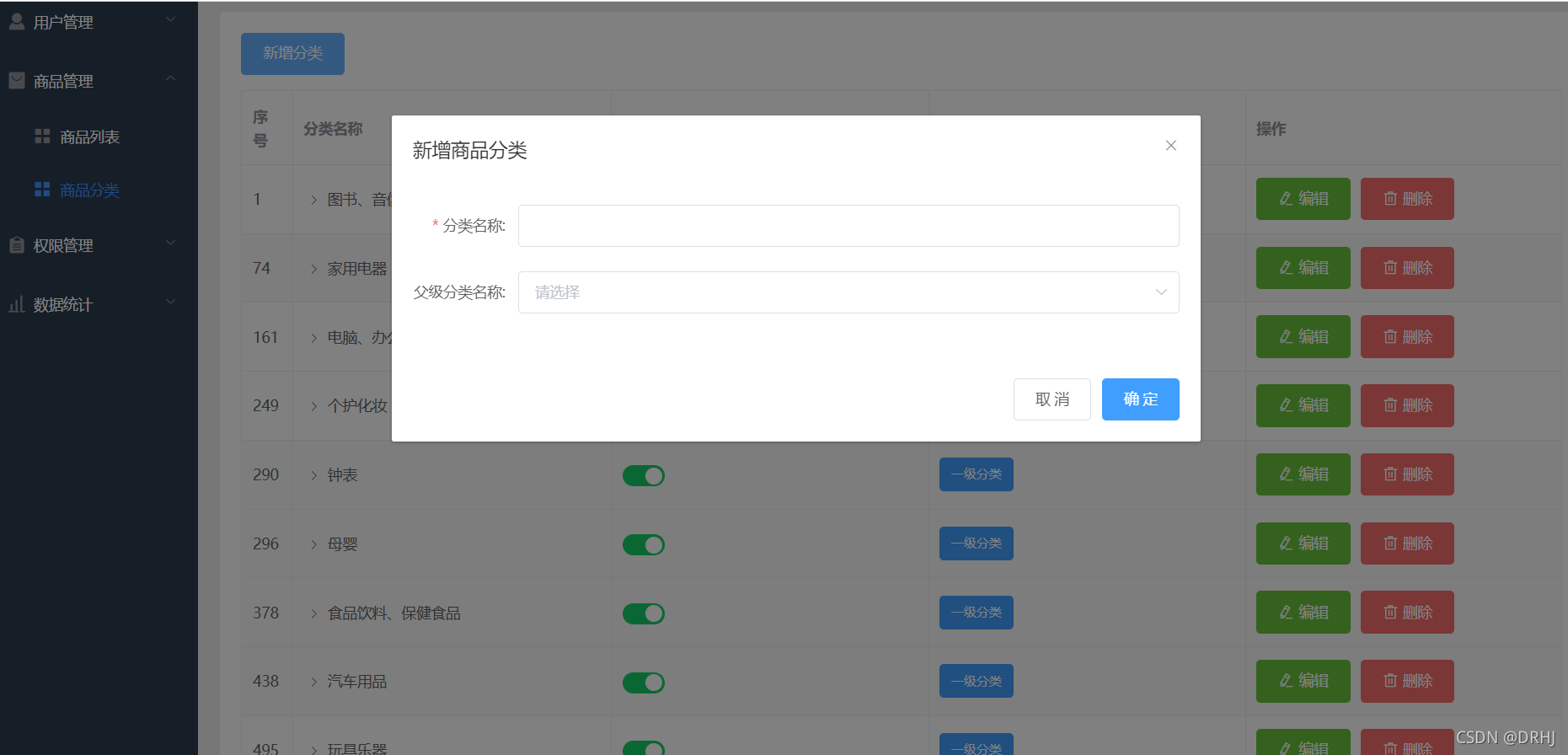
2.页面js分析
<!-- 2.1定义一行 使用栅格-->
<el-row>
<el-col :span="24">
<el-button type="primary" @click="showAddItemCatDialog">新增分类</el-button>
</el-col>
</el-row>
showAddItemCatDialog() {
this.findParentItemCatList()
this.addItemCatDialogVisible = true
},
async findParentItemCatList() {
const {
data: result
} = await this.$http.get("/itemCat/findItemCatList/2")
if (result.status !== 200) return this.$message.error("获取商品分类列表失败!!")
this.parentItemCatList = result.data
},
<span slot="footer" class="dialog-footer">
<el-button @click="addItemCatDialogVisible = false">取 消</el-button>
<el-button type="primary" @click="addItemCatForm">确 定</el-button>
</span>
async addItemCatForm() {
this.$refs.itemCatFormRef.validate(async validate => {
if (!validate) return
const {
data: result
} = await this.$http.post("/itemCat/saveItemCat", this.itemCatForm)
if (result.status !== 200) return this.$message.error("新增商品分类失败")
this.$message.success("新增商品分类成功!!!")
this.findItemCatList();
this.addItemCatDialogVisible = false
})
},
3.业务接口文档
- 请求路径: /itemCat/saveItemCat
- 请求类型: post
- 请求参数: 表单数据
参数名称 | 参数说明 | 备注 |
---|
name | 商品分类名称 | 不能为null |
parentId | 用户父级ID | 不能为null |
level | 分类级别 | 1 2 3 商品分类级别 |
参数名称 | 参数说明 | 备注 |
---|
status | 状态信息 | 200表示服务器请求成功 201表示服务器异常 |
msg | 服务器返回的提示信息 | 可以为null |
data | 服务器返回的业务数据 | 可以为null |
4.编辑ItemCatController
@PostMapping("/saveItemCat")
public SysResult saveItemCat(@RequestBody ItemCat itemCat){
itemCatService.saveItemCat(itemCat);
return SysResult.success();
}
5.编辑ItemCatService
@Override
@Transactional
public void saveItemCat(ItemCat itemCat) {
itemCat.setStatus(true) ;
itemCatMapper.insert(itemCat);
}
四,修改操作
1.修改按钮JS分析
<el-table-column label="操作">
<!-- 定义作用域插槽 定义标签等级-->
<template slot-scope="scope">
<el-button type="success" icon="el-icon-edit" @click="updateItemCatBtn(scope.row)">编辑</el-button>
<el-button type="danger" icon="el-icon-delete" @click="deleteItemCatBtn(scope.row)">删除</el-button>
</template>
</el-table-column>
updateItemCatBtn(itemCat) {
this.updateItemCatForm = itemCat
this.updateItemCatDialogVisible = true
},
2.修改-确定按钮实现
<!-- 添加修改分类对话框 -->
<el-dialog title="修改商品分类" :visible.sync="updateItemCatDialogVisible" width="50%">
<!-- 定义分类表单 -->
<el-form :model="updateItemCatForm" :rules="rules" ref="upDateItemCatForm" label-width="100px">
<el-form-item label="分类名称:" prop="name">
<el-input v-model="updateItemCatForm.name"></el-input>
</el-form-item>
</el-form>
<span slot="footer" class="dialog-footer">
<el-button @click="updateItemCatDialogVisible = false">取 消</el-button>
<el-button type="primary" @click="updateItemCat">确 定</el-button>
</span>
</el-dialog>
async updateItemCat() {
const {
data: result
} = await this.$http.put('/itemCat/updateItemCat', this.updateItemCatForm)
if (result.status !== 200) return this.$message.error("更新商品分类失败")
this.$message.success("更新商品分类成功")
this.findItemCatList();
this.updateItemCatDialogVisible = false;
},
3.业务接口文档
- 请求路径: /itemCat/updateItemCat
- 请求类型: put
- 请求参数: 表单数据 ItemCat对象
- 返回值: SysResult对象
参数名称 | 参数说明 | 备注 |
---|
status | 状态信息 | 200表示服务器请求成功 201表示服务器异常 |
msg | 服务器返回的提示信息 | 可以为null |
data | 服务器返回的业务数据 | 可以为null |
4.编辑ItemCatController
@PutMapping("/updateItemCat")
public SysResult updateItemCat(@RequestBody ItemCat itemCat){
itemCatService.updateItemCat(itemCat);
return SysResult.success();
}
5.编辑ItemCatService
@Override
@Transactional
public void updateItemCat(ItemCat itemCat) {
itemCatMapper.updateById(itemCat);
}
五,删除操作
1.业务说明
规则:
1.如果删除的商品分类是三级,则可以直接删除.
2.如果删除的商品分类是二级,则先删除三级,在删除二级.
3.如果删除的商品分类是一级,则先删除三级/二级/一级
注意事务的控制.
2.页面JS分析
deleteItemCatBtn(itemCat) {
this.$confirm('此操作将永久删除该数据, 是否继续?', '提示', {
confirmButtonText: '确定',
cancelButtonText: '取消',
type: 'warning'
}).then(async () =>{
const {data: result} = await this.$http.delete("/itemCat/deleteItemCat",{params:{id:itemCat.id,level:itemCat.level}})
if(result.status !== 200) return this.$message.error("删除商品分类失败")
this.$message.success("删除数据成功")
this.findItemCatList()
}).catch(() => {
this.$message({
type: 'info',
message: '已取消删除AAAAAAA'
});
});
}
3.业务接口实现
- 请求路径: /itemCat/deleteItemCat
- 请求类型: delete
- 业务描述: 当删除节点为父级时,应该删除自身和所有的子节点
- 请求参数:
参数名称 | 参数说明 | 备注 |
---|
id | 用户id号 | 不能为null |
level | 商品分类级别 一级,二级,三级 | |
参数名称 | 参数说明 | 备注 |
---|
status | 状态信息 | 200表示服务器请求成功 201表示服务器异常 |
msg | 服务器返回的提示信息 | 可以为null |
data | 服务器返回的业务数据 | 可以为null |
4.编辑ItemCatController
@DeleteMapping("/deleteItemCat")
public SysResult deleteItemCats(ItemCat itemCat){
itemCatService.deleteItemCats(itemCat);
return SysResult.success();
}
5.编辑ItemCatService
@Override
@Transactional
public void deleteItemCat(ItemCat itemCat) {
long start = System.currentTimeMillis();
int level = itemCat.getLevel();
itemCatMapper.deleteById(itemCat.getId());
List<Integer> ids = new ArrayList<>();
ids.add(itemCat.getId());
while (level < 3) {
ids = itemCatMapper.selectIdsByPIDs(ids);
System.out.println(ids);
if (ids.isEmpty()) {
break;
}
itemCatMapper.deleteBatchIds(ids);
level++;
}
long end = System.currentTimeMillis();
System.out.println("耗时:" + (end - start));
}
六,完整代码
页面
<template>
<div>
<!-- 1.定义面包屑导航-->
<el-breadcrumb separator-class="el-icon-arrow-right">
<el-breadcrumb-item :to="{ path: '/home' }">首页</el-breadcrumb-item>
<el-breadcrumb-item>商品管理</el-breadcrumb-item>
<el-breadcrumb-item>商品分类</el-breadcrumb-item>
</el-breadcrumb>
<!-- 2.定义卡片视图 -->
<el-card class="box-card">
<!-- 2.1定义一行 使用栅格-->
<el-row>
<el-col :span="24">
<el-button type="primary" @click="showAddItemCatDialog">新增分类</el-button>
</el-col>
</el-row>
<!-- 2.2定义表格数据-->
<el-table :data="itemCatList" style="width: 100%;margin-bottom: 20px;" row-key="id" border stripe>
<el-table-column type="index" label="序号">
</el-table-column>
<el-table-column prop="name" label="分类名称">
</el-table-column>
<el-table-column prop="status" label="状态">
<!-- 定义作用域插槽 展现数据 scope.row展现行级元素 -->
<template slot-scope="scope">
<el-switch v-model="scope.row.status" active-color="#13ce66" inactive-color="#ff4949"
@change="updateStatus(scope.row)"></el-switch>
</template>
</el-table-column>
<el-table-column prop="level" label="等级">
<!-- 定义作用域插槽 定义标签等级-->
<template slot-scope="scope">
<el-tag effect="dark" v-if="scope.row.level == 1">一级分类</el-tag>
<el-tag effect="dark" type="warning" v-if="scope.row.level == 2">二级分类</el-tag>
<el-tag effect="dark" type="danger" v-if="scope.row.level == 3">三级分类</el-tag>
</template>
</el-table-column>
<el-table-column label="操作">
<!-- 定义作用域插槽 定义标签等级-->
<template slot-scope="scope">
<el-button type="success" icon="el-icon-edit" @click="updateItemCatBtn(scope.row)">编辑</el-button>
<el-button type="danger" icon="el-icon-delete" @click="deleteItemCatBtn(scope.row)">删除</el-button>
</template>
</el-table-column>
</el-table>
</el-card>
<!-- 添加新增分类对话框-->
<el-dialog title="新增商品分类" :visible.sync="addItemCatDialogVisible" width="50%" @close="closeAddItemCatDialog">
<!-- 定义分类表单 -->
<el-form :model="itemCatForm" :rules="rules" ref="itemCatFormRef" label-width="100px">
<el-form-item label="分类名称:" prop="name">
<el-input v-model="itemCatForm.name"></el-input>
</el-form-item>
<!-- 定义父级分类选项 -->
<el-form-item label="父级分类名称:">
<!-- 通过级联选择器定义1/2级商品分类
步骤: 1.注意标签导入
2.options 表示数据的来源
3.指定props属性指定数据配置
-->
<el-cascader v-model="selectedKeys" :props="props" :options="parentItemCatList" clearable
@change="parentItemCatChange"></el-cascader>
</el-form-item>
</el-form>
<span slot="footer" class="dialog-footer">
<el-button @click="addItemCatDialogVisible = false">取 消</el-button>
<el-button type="primary" @click="addItemCatForm">确 定</el-button>
</span>
</el-dialog>
<!-- 添加修改分类对话框 -->
<el-dialog title="修改商品分类" :visible.sync="updateItemCatDialogVisible" width="50%">
<!-- 定义分类表单 -->
<el-form :model="updateItemCatForm" :rules="rules" ref="upDateItemCatForm" label-width="100px">
<el-form-item label="分类名称:" prop="name">
<el-input v-model="updateItemCatForm.name"></el-input>
</el-form-item>
</el-form>
<span slot="footer" class="dialog-footer">
<el-button @click="updateItemCatDialogVisible = false">取 消</el-button>
<el-button type="primary" @click="updateItemCat">确 定</el-button>
</span>
</el-dialog>
</div>
</template>
<script>
export default {
created() {
this.findItemCatList()
},
data() {
return {
itemCatList: [],
addItemCatDialogVisible: false,
itemCatForm: {
name: '',
parentId: 0,
level: 1
},
rules: {
name: [{
required: true,
message: '请输入分类名称',
trigger: 'blur'
}]
},
props: {
expandTrigger: "hover",
value: "id",
label: "name",
children: "children",
checkStrictly: true
},
selectedKeys: [],
parentItemCatList: [],
updateItemCatDialogVisible: false,
updateItemCatForm: {}
}
},
methods: {
async findItemCatList() {
const {
data: result
} = await this.$http.get("/itemCat/findItemCatList/3")
if (result.status !== 200) return this.$message.error("获取商品分类列表失败!!")
this.itemCatList = result.data
},
async updateStatus(itemCat) {
const {
data: result
} = await this.$http.put(`/itemCat/status/${itemCat.id}/${itemCat.status}`)
if (result.status !== 200) return this.$message.error("修改状态失败")
this.$message.success("状态修改成功")
},
showAddItemCatDialog() {
this.findParentItemCatList()
this.addItemCatDialogVisible = true
},
async findParentItemCatList() {
const {
data: result
} = await this.$http.get("/itemCat/findItemCatList/2")
if (result.status !== 200) return this.$message.error("获取商品分类列表失败!!")
this.parentItemCatList = result.data
},
parentItemCatChange() {
console.log(this.selectedKeys)
console.log(this.itemCatForm)
if (this.selectedKeys.length > 0) {
this.itemCatForm.parentId = this.selectedKeys[this.selectedKeys.length - 1]
this.itemCatForm.level = this.selectedKeys.length + 1
} else {
this.itemCatForm.parentId = 0
this.itemCatForm.level = 1
}
},
async addItemCatForm() {
this.$refs.itemCatFormRef.validate(async validate => {
if (!validate) return
const {
data: result
} = await this.$http.post("/itemCat/saveItemCat", this.itemCatForm)
if (result.status !== 200) return this.$message.error("新增商品分类失败")
this.$message.success("新增商品分类成功!!!")
this.findItemCatList();
this.addItemCatDialogVisible = false
})
},
closeAddItemCatDialog() {
this.initItemCatForm()
},
initItemCatForm() {
this.$refs.itemCatFormRef.resetFields()
this.itemCatForm.parentId = 0
this.itemCatForm.level = 1
this.selectedKeys = []
},
updateItemCatBtn(itemCat) {
this.updateItemCatForm = itemCat
this.updateItemCatDialogVisible = true
},
async updateItemCat() {
const {
data: result
} = await this.$http.put('/itemCat/updateItemCat', this.updateItemCatForm)
if (result.status !== 200) return this.$message.error("更新商品分类失败")
this.$message.success("更新商品分类成功")
this.findItemCatList();
this.updateItemCatDialogVisible = false;
},
deleteItemCatBtn(itemCat) {
this.$confirm('此操作将永久删除该数据, 是否继续?', '提示', {
confirmButtonText: '确定',
cancelButtonText: '取消',
type: 'warning'
}).then(async () =>{
const {data: result} = await this.$http.delete("/itemCat/deleteItemCat",{params:{id:itemCat.id,level:itemCat.level}})
if(result.status !== 200) return this.$message.error("删除商品分类失败")
this.$message.success("删除数据成功")
this.findItemCatList()
}).catch(() => {
this.$message({
type: 'info',
message: '已取消删除'
});
});
}
}
}
</script>
<style lang="less" scoped>
.el-cascader {
width: 100%;
}
</style>
业务层
@Service
public class ItemCatServiceImpl implements ItemCatService{
@Autowired
private ItemCatMapper itemCatMapper;
@Override
public List<ItemCat> findAll() {
long startTime = System.currentTimeMillis();
QueryWrapper<ItemCat> queryWrapper = new QueryWrapper<>();
queryWrapper.orderByDesc("level");
List<ItemCat> itemCatList = itemCatMapper.selectList(queryWrapper);
int s = itemCatList.size();
for (int i = 0; i < s; i++) {
ItemCat itemCat = itemCatList.get(i);
if (itemCat.getLevel() > 1 ) {
for (int j = i + 1; j < s; j++) {
ItemCat itemCat1 = itemCatList.get(j);
if (itemCat1.getId().equals(itemCat.getParentId())) {
List<ItemCat> itemCatList1 = new ArrayList<>();
if (itemCat1.getChildren() != null) {
itemCatList1 = itemCat1.getChildren();
}
itemCatList1.add(itemCat);
itemCat1.setChildren(itemCatList1);
}
}
}
}
List<ItemCat> itemCatList2 = new ArrayList<>();
for (int i = 0; i < itemCatList.size(); i++) {
ItemCat itemCat = itemCatList.get(i);
if (itemCat.getLevel() == 1) {
itemCatList2.add(itemCat);
}
}
long endTime = System.currentTimeMillis();
System.out.println("耗时:"+(endTime - startTime));
return itemCatList2;
}
@Override
@Transactional
public List<ItemCat> findItemCatList(Integer level) {
long startTime = System.currentTimeMillis();
QueryWrapper<ItemCat> queryWrapper = new QueryWrapper<>();
queryWrapper.le("level",level)
.orderByDesc("level");
List<ItemCat> itemCatList = itemCatMapper.selectList(queryWrapper);
int s = itemCatList.size();
int a = 0;
int b = 0;
ItemCat itemCat;
ItemCat itemCat1;
for (int i = 0; i < s; i++) {
itemCat = itemCatList.get(i);
if (itemCat.getLevel() > 1 ) {
for (int j = i + 1; j < s; j++) {
itemCat1 = itemCatList.get(j);
a = itemCat.getParentId();
b = itemCat1.getId();
if (a == b) {
List<ItemCat> itemCatList1 = new ArrayList<>();
if (itemCat1.getChildren() != null) {
itemCatList1 = itemCat1.getChildren();
}
itemCatList1.add(itemCat);
itemCat1.setChildren(itemCatList1);
}
}
}
}
List<ItemCat> itemCatList2 = new ArrayList<>();
for (ItemCat itemCat2 : itemCatList) {
if (itemCat2.getLevel() == 1) {
itemCatList2.add(itemCat2);
}
}
long endTime = System.currentTimeMillis();
System.out.println("耗时:"+(endTime-startTime));
return itemCatList2;
}
@Override
public void updateStatus(ItemCat itemCat) {
itemCatMapper.updateById(itemCat);
}
@Override
public void saveItemCat(ItemCat itemCat) {
itemCat.setStatus(true);
itemCatMapper.insert(itemCat);
}
@Override
public void updateItemCat(ItemCat itemCat) {
itemCatMapper.updateById(itemCat);
}
@Override
@Transactional
public void deleteItemCat(ItemCat itemCat) {
long start = System.currentTimeMillis();
int level = itemCat.getLevel();
itemCatMapper.deleteById(itemCat.getId());
List<Integer> ids = new ArrayList<>();
ids.add(itemCat.getId());
while (level < 3) {
ids = itemCatMapper.selectIdsByPIDs(ids);
System.out.println(ids);
if (ids.isEmpty()) {
break;
}
itemCatMapper.deleteBatchIds(ids);
level++;
}
long end = System.currentTimeMillis();
System.out.println("耗时:" + (end - start));
}
private Map<Integer,List<ItemCat>> getMap() {
Map<Integer,List<ItemCat>> map = new HashMap<>();
List<ItemCat> list = itemCatMapper.selectList(null);
for (ItemCat itemCat : list) {
int parentId = itemCat.getParentId();
if (map.containsKey(parentId)) {
map.get(parentId).add(itemCat);
} else {
List<ItemCat> children = new ArrayList<>();
children.add(itemCat);
map.put(parentId,children);
}
}
return map;
}
private List<ItemCat> getTwoList(Map<Integer,List<ItemCat>> map) {
List<ItemCat> oneList = map.get(0);
for (ItemCat oneItemCat : oneList) {
int parentId = oneItemCat.getId();
List<ItemCat> twoList = map.get(parentId);
oneItemCat.setChildren(twoList);
}
return oneList;
}
private List<ItemCat> getThreeList(Map<Integer,List<ItemCat>> map) {
List<ItemCat> oneList = getTwoList(map);
for (ItemCat oneItemCat : oneList) {
List<ItemCat> twoList = oneItemCat.getChildren();
if (twoList == null || twoList.size() == 0) {
continue;
}
for (ItemCat twoItemCat : twoList) {
int parentId = twoItemCat.getId();
List<ItemCat> threeList = map.get(parentId);
twoItemCat.setChildren(threeList);
}
}
return oneList;
}
}
控制层
@RestController
@RequestMapping("/itemCat")
@CrossOrigin
public class ItemCatController {
@Autowired
private ItemCatService itemCatService;
@GetMapping("/findAll")
public List<ItemCat> findAll() {
return itemCatService.findAll();
}
@GetMapping("/findItemCatList/{level}")
public SysResult findItemCatList(@PathVariable Integer level) {
return SysResult.success(itemCatService.findItemCatList(level));
}
@PutMapping("/status/{id}/{status}")
public SysResult updateStatus(ItemCat itemCat) {
itemCatService.updateStatus(itemCat);
return SysResult.success();
}
@PostMapping("/saveItemCat")
public SysResult saveItemCat(@RequestBody ItemCat itemCat) {
itemCatService.saveItemCat(itemCat);
return SysResult.success();
}
@PutMapping("/updateItemCat")
public SysResult updateItemCat(@RequestBody ItemCat itemCat) {
itemCatService.updateItemCat(itemCat);
return SysResult.success();
}
@DeleteMapping("/deleteItemCat")
public SysResult deleteItemCat(ItemCat itemCat) {
itemCatService.deleteItemCat(itemCat);
return SysResult.success();
}
}