1.原理图解
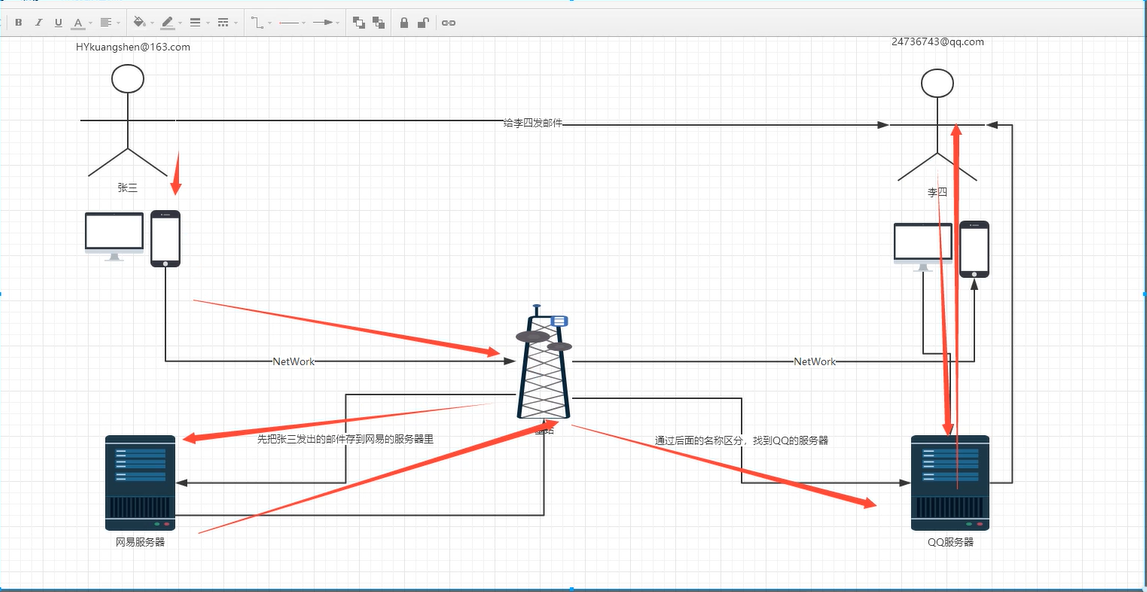
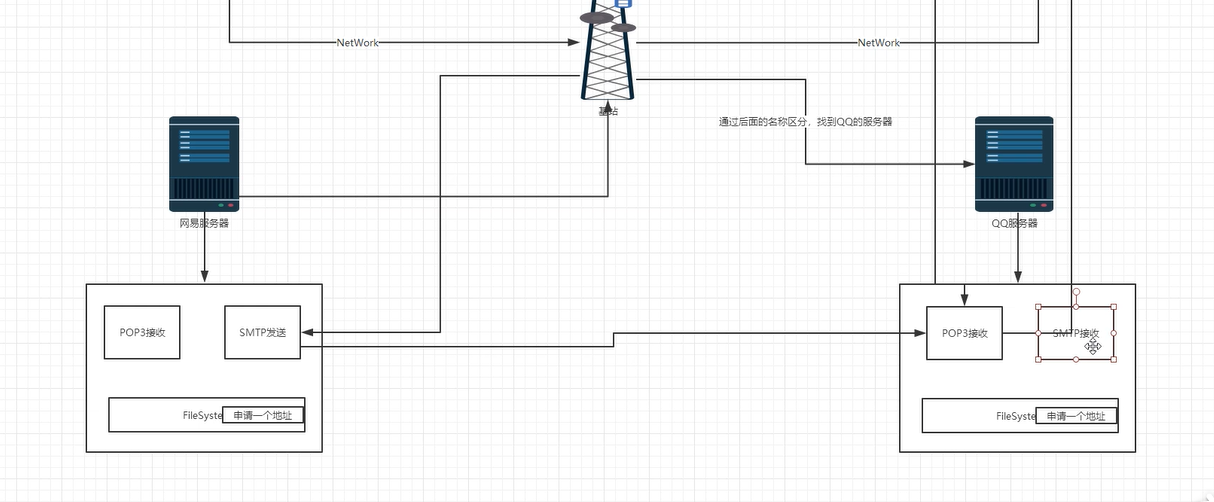
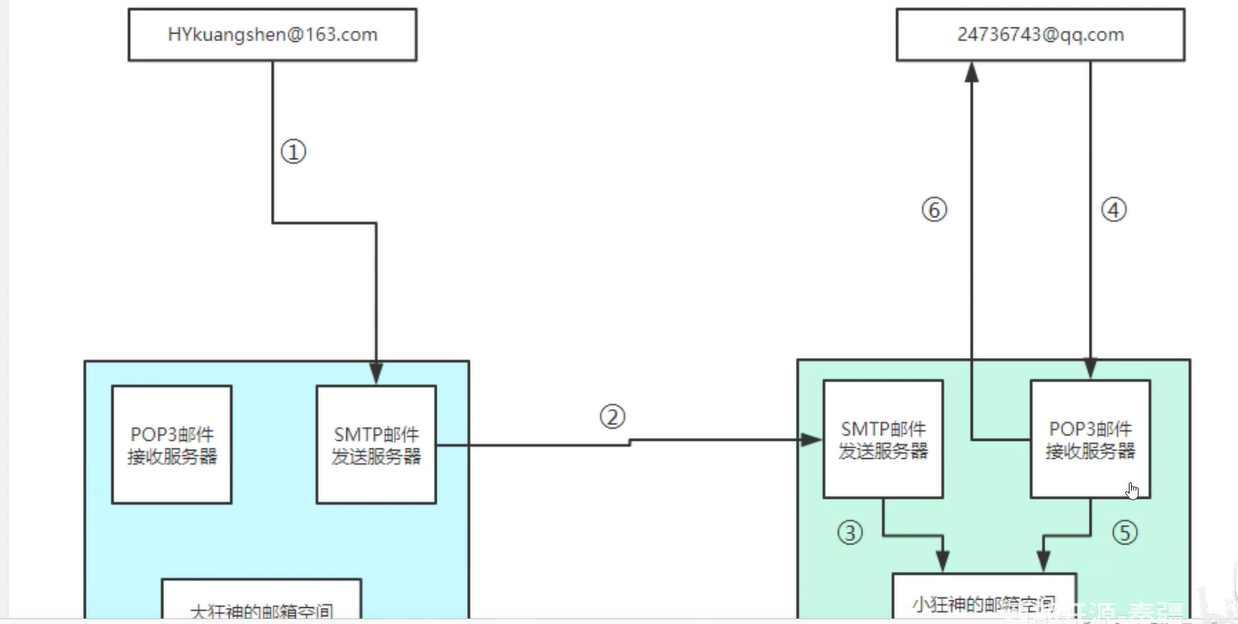
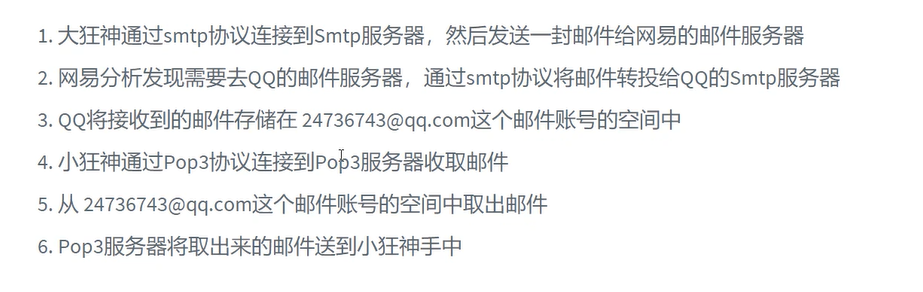

<!-- https://mvnrepository.com/artifact/javax.mail/mail -->
<dependency>
<groupId>javax.mail</groupId>
<artifactId>mail</artifactId>
<version>1.4.7</version>
</dependency>
<!-- https://mvnrepository.com/artifact/javax.activation/activation -->
<dependency>
<groupId>javax.activation</groupId>
<artifactId>activation</artifactId>
<version>1.1.1</version>
</dependency>
原理图:
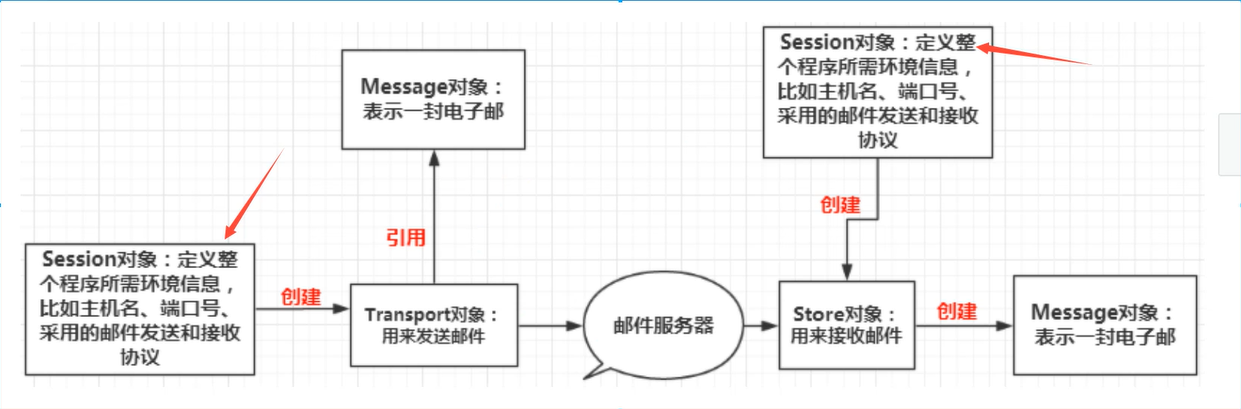
2.简单的邮件发送
实施条件:
1.两个jar包
mail.jar
activation.jar
代码实现:
//发送一封简单邮箱
public class Mail01 {
public static void main(String[] args) throws Exception {
Properties prop = new Properties();
prop.setProperty("mail.host", "smtp.qq.com"); //设置QQ邮件服务器
prop.setProperty("mail.transport.protocol", "smtp"); //邮件发送协议
prop.setProperty("mail.smtp.auth", "true"); //需要验证用户名和密码
//关于QQ邮箱,还要设置SSL加密,加上以下代码
MailSSLSocketFactory sf = new MailSSLSocketFactory();
prop.put("mail.smtp.ssl.enable", "true");
prop.put("mail.smtp.socketFactory.class", "javax.net.ssl.SSLSocketFactory");
prop.put("mail.smtp.ssl.socketFactory", sf);
//使用JavaMail发送邮件的5个步骤
//1.创建定义这个应用程序所需的环境消息的 Session对象
//QQ才有!其他邮箱不需要
Session session = Session.getDefaultInstance(prop, new Authenticator() {
@Override
protected PasswordAuthentication getPasswordAuthentication() {
//发送人邮件名、授权码
return new PasswordAuthentication("2082767195@qq.com", "bbvlmdhentfiehfi");
}
});
//开启Session的debug模式, 这样就可以查看到程序发送Email的运行状态
session.setDebug(true);
//2.通过Session得到transport对象
Transport ts = session.getTransport();
//3.使用邮箱的用户名和授权码连上服务器
ts.connect("smtp.qq.com", "2082767195@qq.com", "bbvlmdhentfiehfi");
//4.创建邮件:写邮件
//创建文件对象(需要传递Session)
MimeMessage message = new MimeMessage(session);
//指明邮件的发件人
message.setFrom(new InternetAddress("2082767195@qq.com"));
//指明邮件的发件人, 现在发件人和收件人是一样的, 那就是自己给自己发
message.setRecipient(Message.RecipientType.TO, new InternetAddress("2082767195@qq.com"));
//邮件的标题
message.setSubject("只包含文本的简单邮件");
//邮件的文本内容
message.setContent("身伤易逝,情伤难合", "text/html;charset=UTF-8");
//5.发送邮件
ts.sendMessage(message, message.getAllRecipients());
//6.关闭连接
ts.close();
}
}
3.网站注册发送邮件实现
实施条件:
MimeBodPart类
表示一个MIME消息, 它与MimeMessage类一样都是从Part接口继承过来。
MimeMultipart类
抽象类Multipart的实现子类, 他用来3组合多个MIME消息。一个MimeMultipart对象可以包含多个代表MIME消息的MimeBodyPart对象。
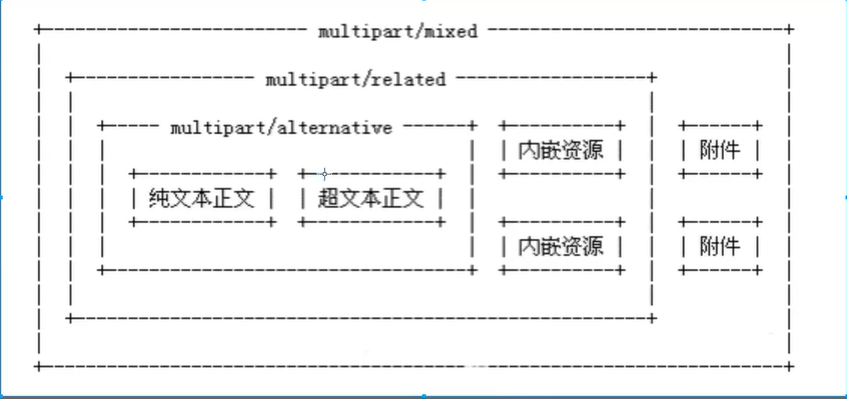
代码实现:
//发送一封复杂邮箱
public class Mail02 {
public static void main(String[] args) throws Exception {
Properties prop = new Properties();
prop.setProperty("mail.host", "smtp.qq.com"); //设置QQ邮件服务器
prop.setProperty("mail.transport.protocol", "smtp"); //邮件发送协议
prop.setProperty("mail.smtp.auth", "true"); //需要验证用户名和密码
//关于QQ邮箱,还要设置SSL加密,加上以下代码
MailSSLSocketFactory sf = new MailSSLSocketFactory();
prop.put("mail.smtp.ssl.enable", "true");
prop.put("mail.smtp.socketFactory.class", "javax.net.ssl.SSLSocketFactory");
prop.put("mail.smtp.ssl.socketFactory", sf);
//使用JavaMail发送邮件的5个步骤
//1.创建定义这个应用程序所需的环境消息的 Session对象
//QQ才有!其他邮箱不需要
Session session = Session.getDefaultInstance(prop, new Authenticator() {
@Override
protected PasswordAuthentication getPasswordAuthentication() {
//发送人邮件名、授权码
return new PasswordAuthentication("2082767195@qq.com", "bbvlmdhentfiehfi");
}
});
//开启Session的debug模式, 这样就可以查看到程序发送Email的运行状态
session.setDebug(true);
//2.通过Session得到transport对象
Transport ts = session.getTransport();
//3.使用邮箱的用户名和授权码连上服务器
ts.connect("smtp.qq.com", "2082767195@qq.com", "bbvlmdhentfiehfi");
//4.创建邮件:写邮件
MimeMessage message = new MimeMessage(session);
//指明邮件的发件人
message.setFrom(new InternetAddress("2082767195@qq.com"));
//指明邮件的发件人, 现在发件人和收件人是一样的, 那就是自己给自己发
message.setRecipient(Message.RecipientType.TO, new InternetAddress("2082767195@qq.com"));
//邮件的标题
message.setSubject("只包含附件的复杂邮件");
//准备图片数据
MimeBodyPart image = new MimeBodyPart();
//图片经过数据处理…… DataHandler: 数据处理
DataHandler dh = new DataHandler(new FileDataSource("src/resource/厚涂画风.jpg"));
image.setDataHandler(dh);//在我们的Body中放入这个处理的图片数据
image.setContentID("bz.jpg");//给图片设置一个ID(名字), 我们在后面可以使用!
//准备正文数据
MimeBodyPart text = new MimeBodyPart();
text.setContent("这是一封邮件正文图片<img src='cid:bz.jpg'>的邮件", "text/html;charset=UTF-8");
//附件
MimeBodyPart enclosure = new MimeBodyPart();
enclosure.setDataHandler(new DataHandler(new FileDataSource("src/resource/1.txt")));
enclosure.setFileName("fj.txt");//附件设置名字
//描述数据关系
MimeMultipart mm = new MimeMultipart();
mm.addBodyPart(text);
mm.addBodyPart(image);
mm.setSubType("related");
//拼装好正文内容设置为主体
MimeBodyPart contentText = new MimeBodyPart();
contentText.setContent(mm);
//拼接附件
MimeMultipart allFile = new MimeMultipart();
allFile.addBodyPart(enclosure);//附件
allFile.addBodyPart(contentText);//正文
allFile.setSubType("mixed");//正文和附件都存在邮件中, 所有类型设置为mixed
//设置到消息中, 保存修改
message.setContent(allFile);
message.saveChanges();
//5.发送邮件
ts.sendMessage(message, message.getAllRecipients());
//6.关闭连接
ts.close();
}
}