第19题 删除链表的倒数第N个结点
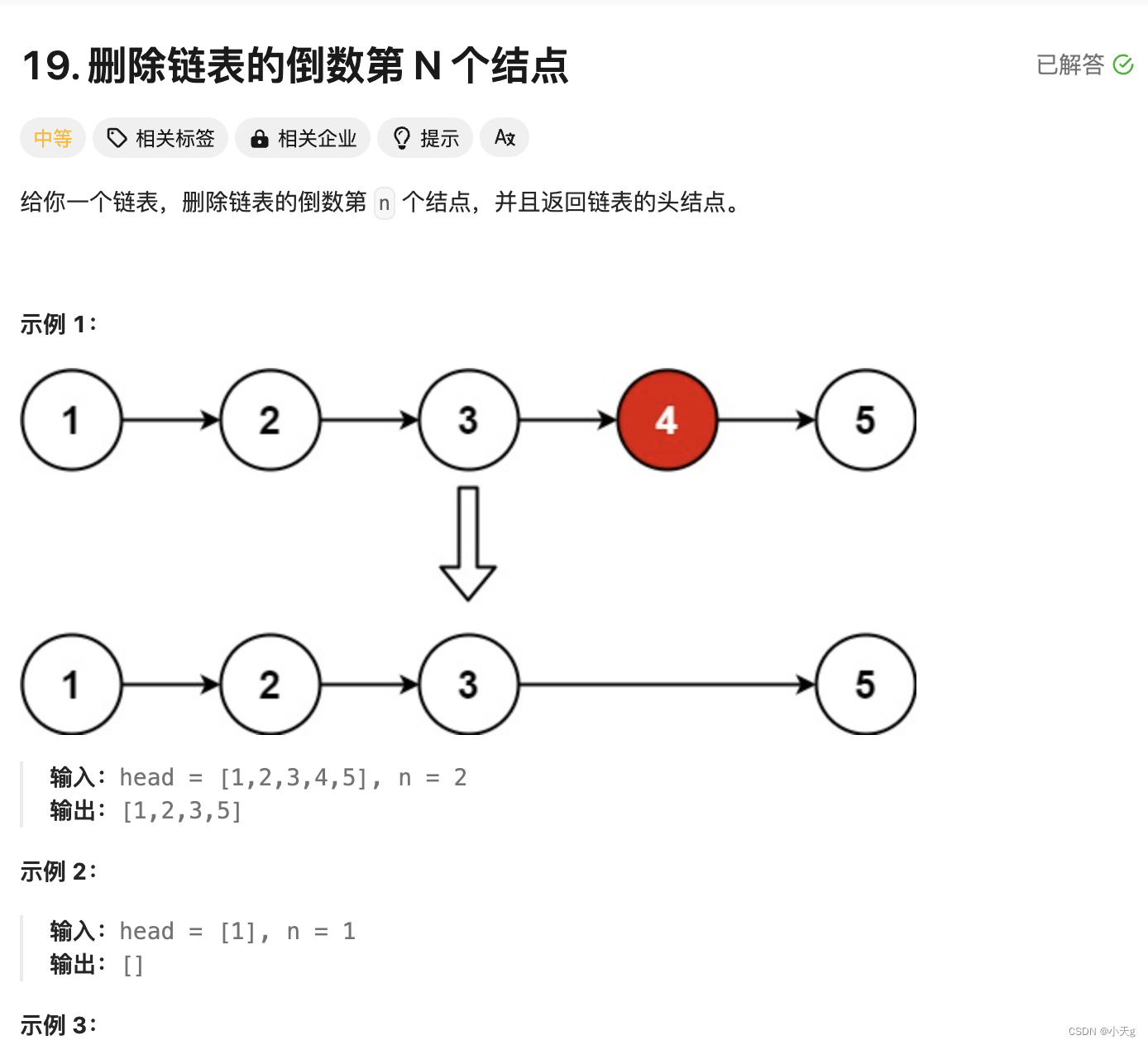
public ListNode removeNthFromEnd(ListNode head, int n) {
if (head == null || n == 0) return head;
ListNode next = head;
int count = 0;
while (next != null){
next = next.next;
count++;
}
if (count < n) return head;
if (count == n) return head.next;
ListNode pre = head;
for (int i = 0; i < count - n - 1; i++) {
pre = pre.next;
}
pre.next = pre.next.next;
return head;
}
第24题 两两交换链表中的节点
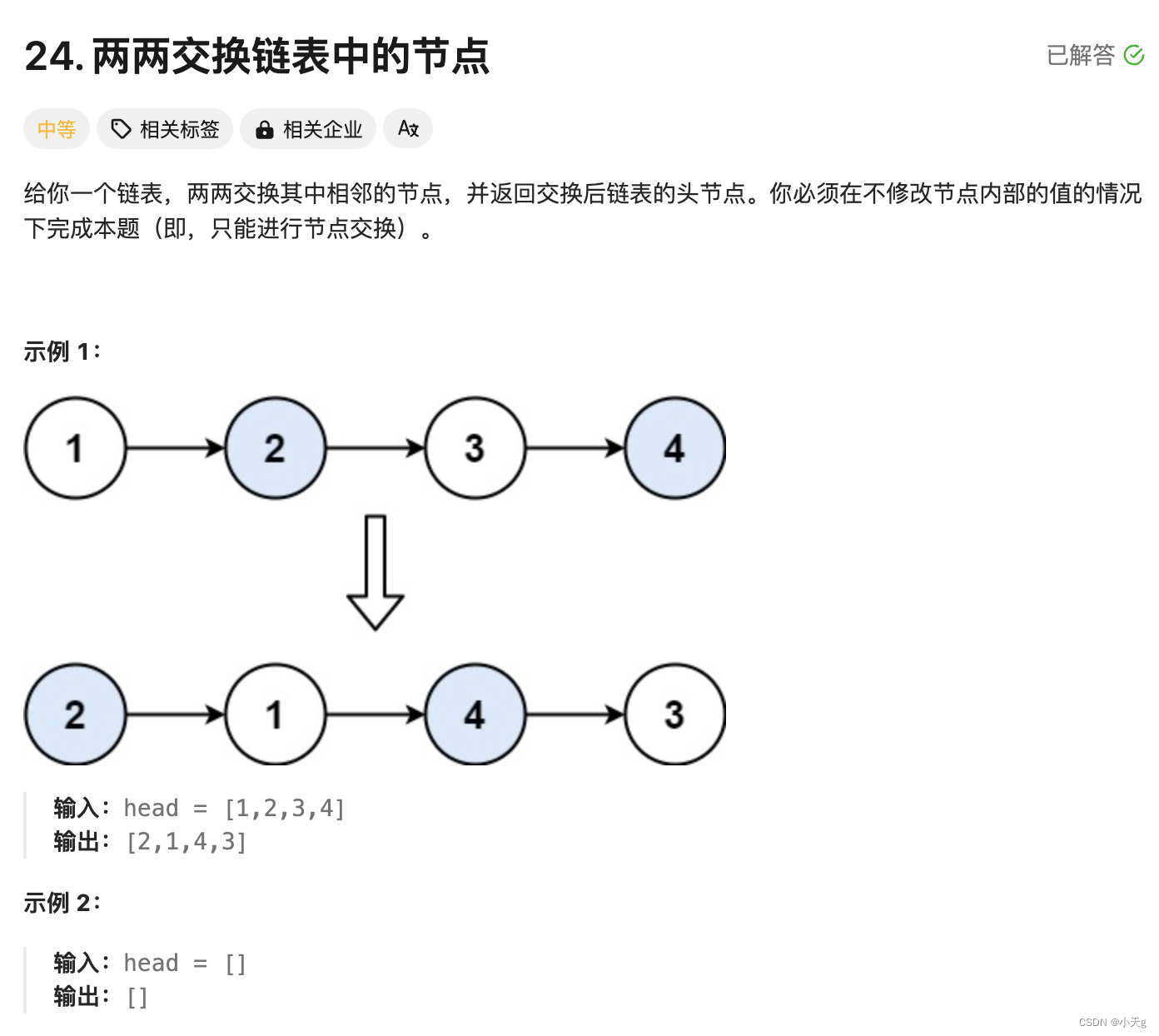
public ListNode swapPairs(ListNode head) {
if(head == null || head.next == null) return head;
ListNode p = head;
ListNode q = head.next;
ListNode pre = head;
head = q;
while (p.next != null) {
pre.next = q;
p.next = q.next;
q.next = p;
if(p.next != null){
pre = p;
p = p.next;
q = p.next;
}
}
return head;
}
第142题 环形链表II
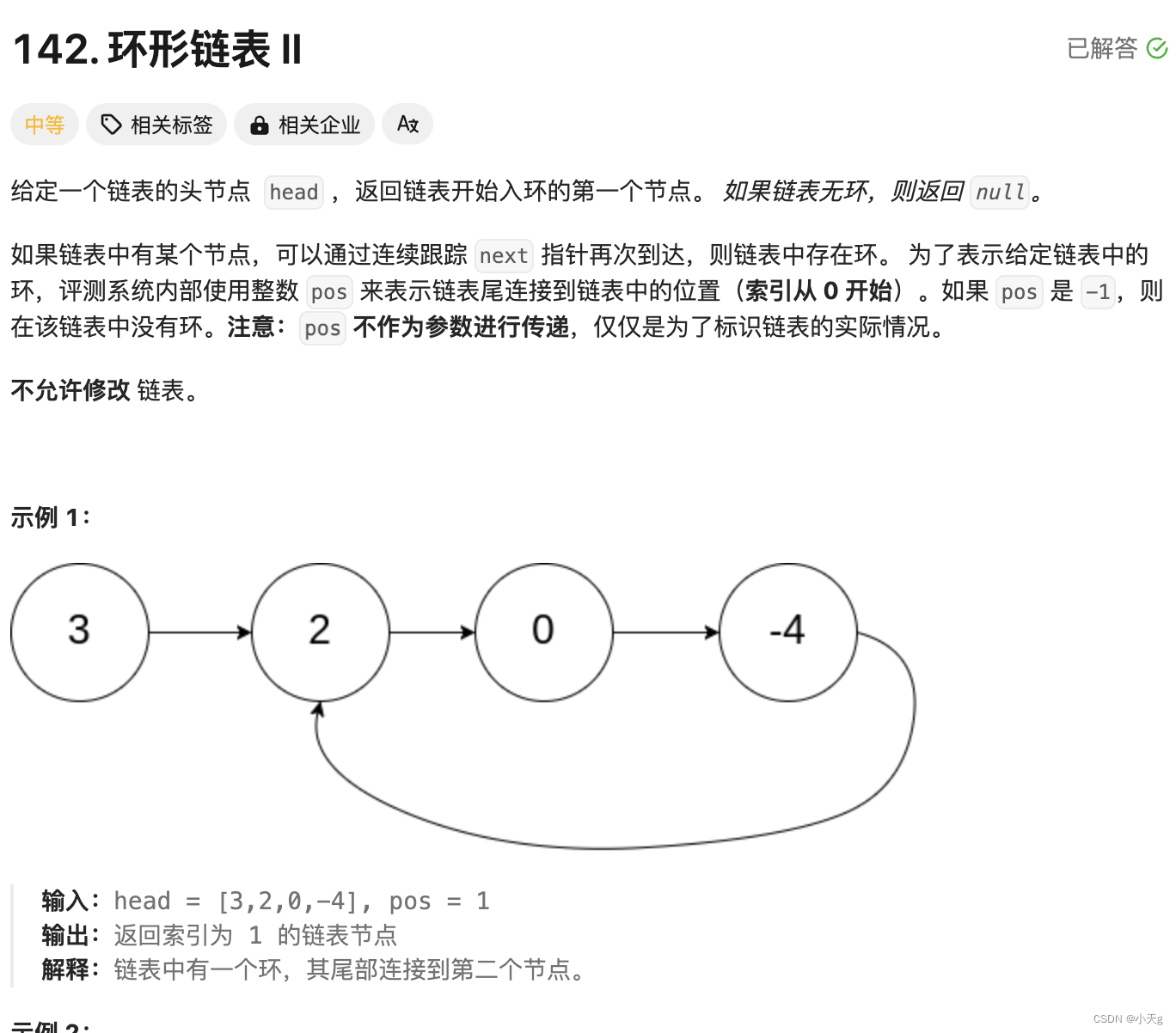
public ListNode detectCycle(ListNode head) {
if(head == null) return null;
HashSet<ListNode> listNodes = new HashSet<>();
while (head != null){
listNodes.add(head);
if(listNodes.contains(head.next)){
return head.next;
}
head = head.next;
}
return head;
}
第203题 移除链表元素
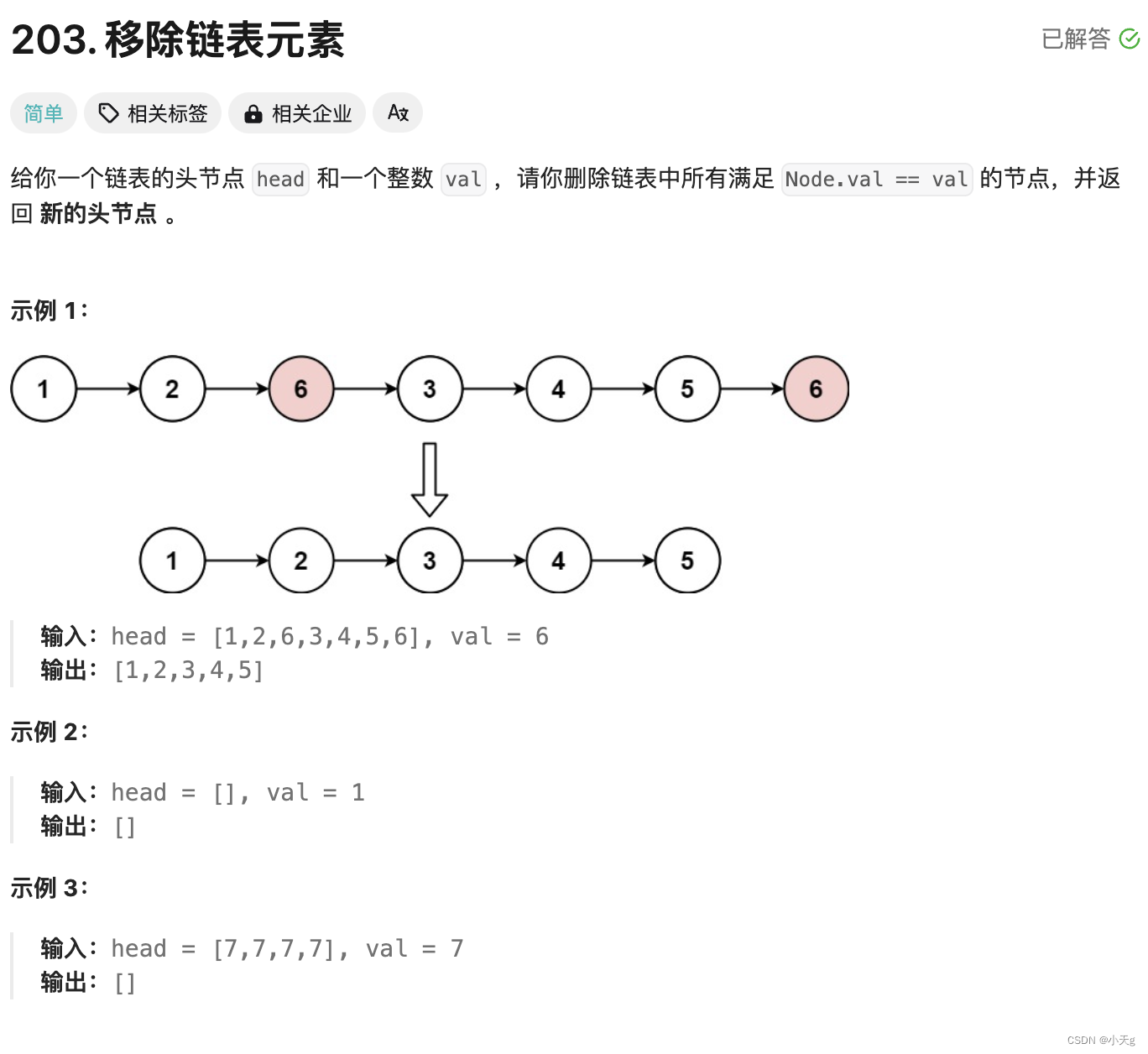
public class ListNode {
int val;
ListNode next;
ListNode() {}
ListNode(int val) { this.val = val; }
ListNode(int val, ListNode next) { this.val = val; this.next = next; }
}
public ListNode removeElements(ListNode head, int val) {
while(head != null && head.val == val){
head = head.next;
}
if(head == null) return head;
ListNode nextNode = head.next;
ListNode preNode = head;
while (nextNode != null){
if (nextNode.val == val){
preNode.next = nextNode.next;
}else {
preNode = nextNode;
}
nextNode = nextNode.next;
}
return head;
}
第206题 反转链表
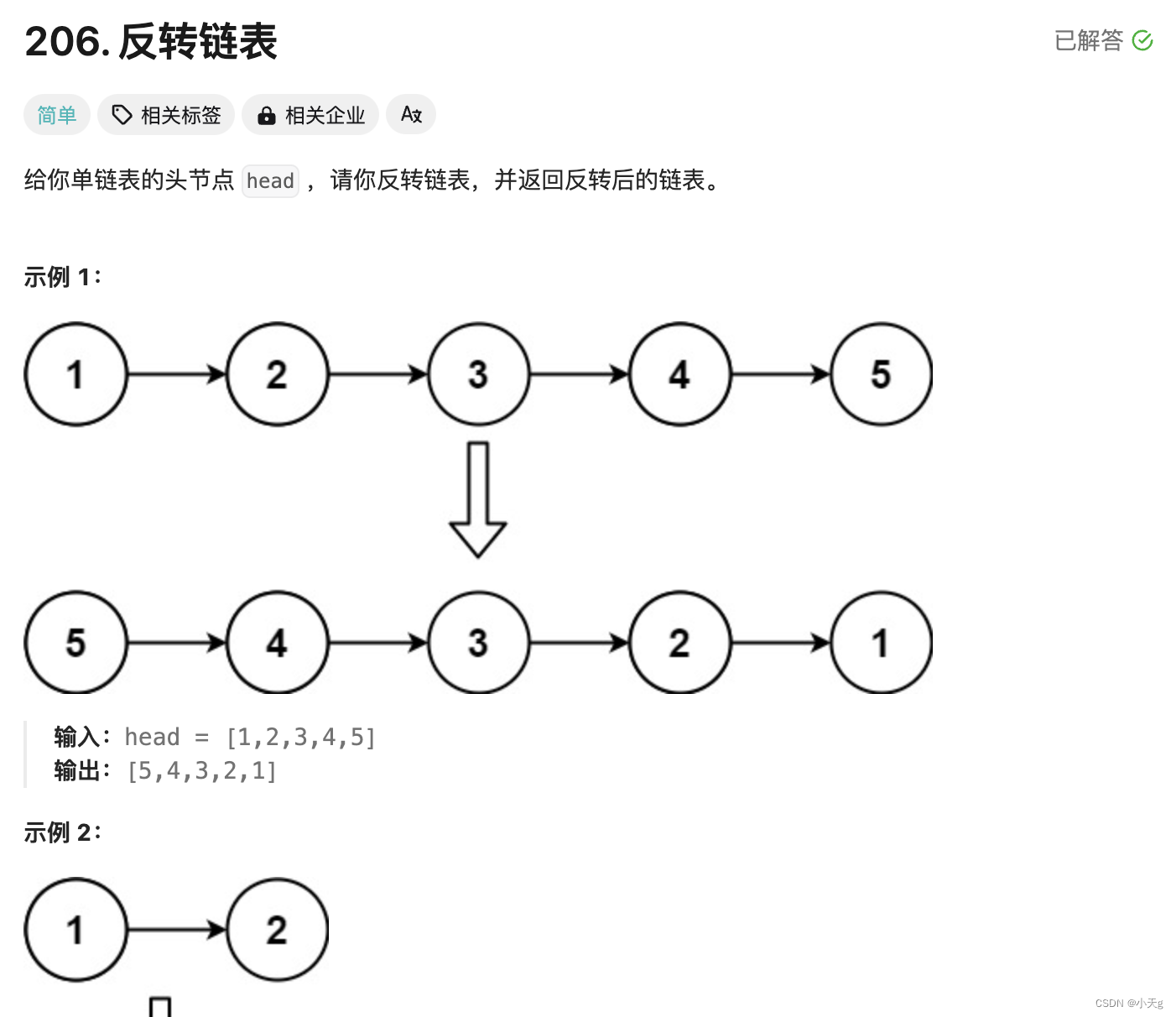
class ListNode {
int val;
ListNode next;
ListNode() {}
ListNode(int val) { this.val = val; }
ListNode(int val, ListNode next) { this.val = val; this.next = next; }
}
public ListNode reverseList(ListNode head) {
if(head == null) return null;
ListNode newHead = null;
while (head != null){
ListNode listNode = new ListNode(head.val);
listNode.next = newHead;
newHead = listNode;
head = head.next;
}
return newHead;
}
第707题 设计链表
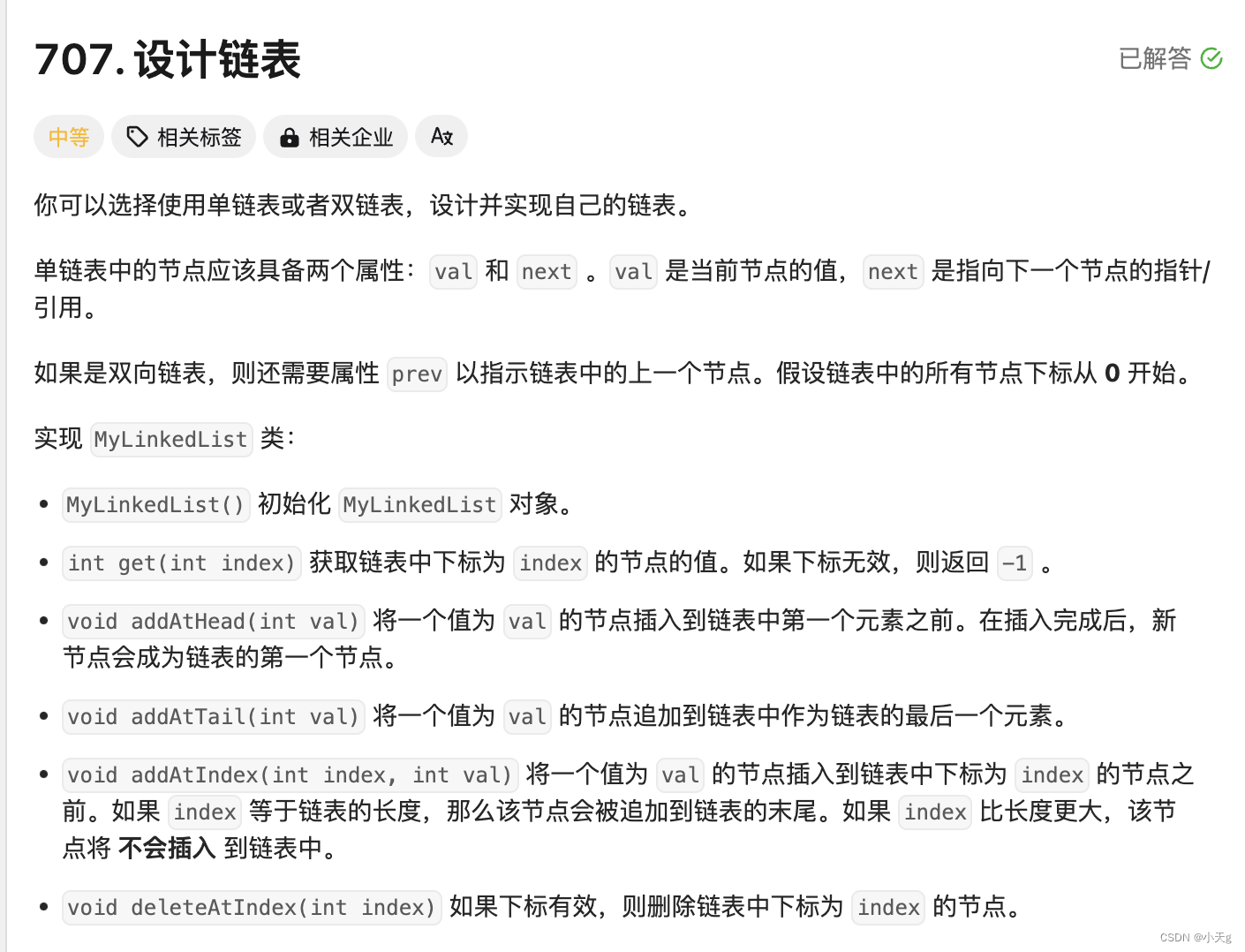
class ListNode {
int val;
ListNode next;
ListNode() {}
ListNode(int val) { this.val = val; }
ListNode(int val, ListNode next) { this.val = val; this.next = next; }
}
public class MyLinkedList {
private ListNode head;
private int length;
public MyLinkedList() {
}
public int getListLength(){
ListNode next = head;
int count = 0;
while (next != null){
next = next.next;
count++;
}
return count;
}
public int get(int index) {
int listLength = getListLength();
if (index+1 > listLength) return -1;
ListNode next = head;
for (int i = 0; i < index; i++) {
next = next.next;
}
return next.val;
}
public void addAtHead(int val) {
ListNode listNode = new ListNode(val);
listNode.next = head;
head = listNode;
}
public void addAtTail(int val) {
ListNode next = head;
while (next != null && next.next != null){
next = next.next;
}
ListNode listNode = new ListNode(val);
if (head == null){
head = listNode;
}else {
next.next = listNode;
}
}
public void addAtIndex(int index, int val) {
int listLength = getListLength();
if(listLength == index) {
addAtTail(val);
return;
}
if(index == 0) {
addAtHead(val);
return;
}
if(index > listLength){
return;
}
ListNode next = head;
for (int i = 0; i < index - 1; i++) {
next = next.next;
}
ListNode listNode = new ListNode(val);
listNode.next = next.next;
next.next = listNode;
}
public void deleteAtIndex(int index) {
int listLength = getListLength();
if(index+1 > listLength){
return;
}
if(index == 0){
head = head.next;
return;
}
ListNode next = head;
for (int i = 0; i < index - 1; i++) {
next = next.next;
}
next.next = next.next.next;
}
}
面试题02.07 链表相交
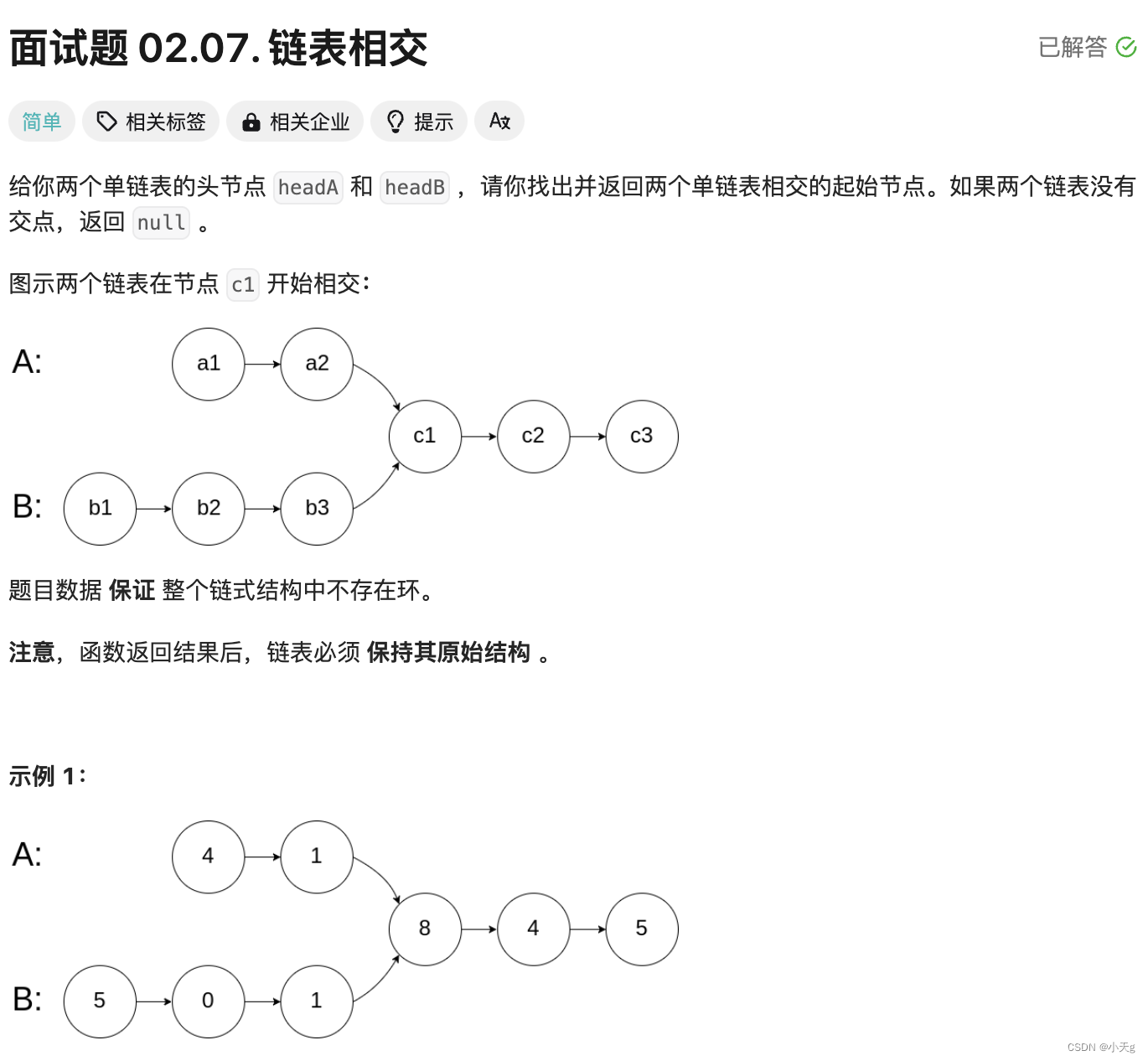
public class Solution {
public ListNode getIntersectionNode(ListNode headA, ListNode headB) {
if(headA == null || headB == null) return null;
Stack<ListNode> AStack = new Stack<>();
Stack<ListNode> BStack = new Stack<>();
ListNode p = headA;
ListNode q = headB;
while (p != null){
AStack.push(p);
p = p.next;
}
while (q != null){
BStack.push(q);
q = q.next;
}
p = AStack.pop();
q = BStack.pop();
if(p != q) return null;
ListNode pre = p;
while (p == q){
pre = p;
if (!AStack.isEmpty()){
p = AStack.pop();
}else {
break;
}
if(!BStack.isEmpty()){
q = BStack.pop();
}else {
break;
}
}
return pre;
}