一、基于注解的Spring AOP的五种增强处理
1. 前置增强处
//定义增强处理
/**
* 1、定义前置增强处理
* @Before注解表示该方法是一个前置增强处理 在目标方法执行之前调用
* 属性value:定义该增强处理切入的位置(切入点)
* execution(* com.cxsw.spring.service.impl.UserServiceImpl.*(..))
* UserServiceImpl实现类下的所有方法
* execution(* com.cxsw.spring.service.impl.*.*(..))
* com.cxsw.spring.service.impl包下所有的类的所有方法都执行增强处理
*/
@Before(value = "execution(* com.cxsw.spring.service.impl.*.*(..))")
public void beforeAdvice(JoinPoint jp) {
System.out.println("基于Spring AOP的前置增强处理.....");
}
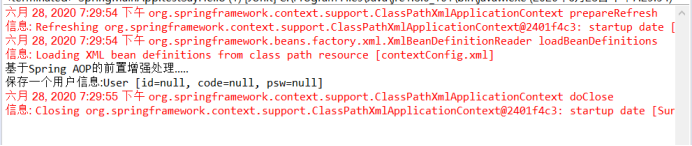
2.后置增强处理
/**
* 2、定义普通的后置增强处理
* @After表示该方法是一个后置增强处理 在目标方法执行之后被调用(织入) 不管目标方法是否正常结束 都会被织入
*/
@After(value = "execution(* com.cxsw.spring.service.impl.*.*(..))")
public void afterAdvice() {
System.out.println("基于Spring AOP的后置增强处理.....");
}
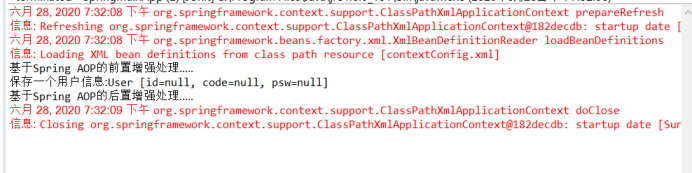
3.后置返回增强处理
/**
* 3、后置返回增强处理
* @AfterReturning表示该方法是一个后置返回增强处理 在目标方法正常结束后被注入 如果,目标方法发生异常 不会被织入
*/
@AfterReturning(value="execution(* com.cxsw.spring.service.impl.*.*(..))")
public void afterReturnAdvice() {
System.out.println("基于Spring AOP的后置返回增强处理.....");
}
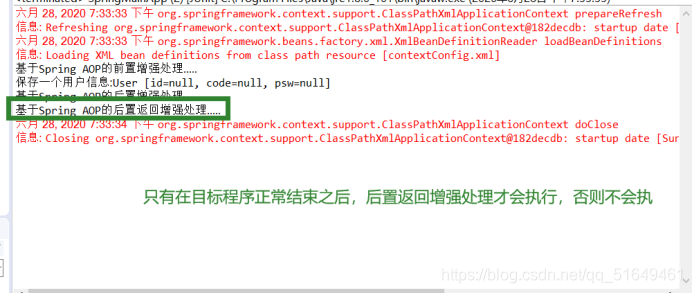
4.异常返回增强处理
/**
* 4、异常返回增强处理
* @AfterThrowing表示该方法是一个异常返回增强处理 只有在目标方法发生异常时被织入
*/
@AfterThrowing(value="execution(* com.cxsw.spring.service.impl.*.*(..))")
public void afterThrowAdvice() {
System.out.println("基于Spring AOP的异常返回增强处理.....");
}
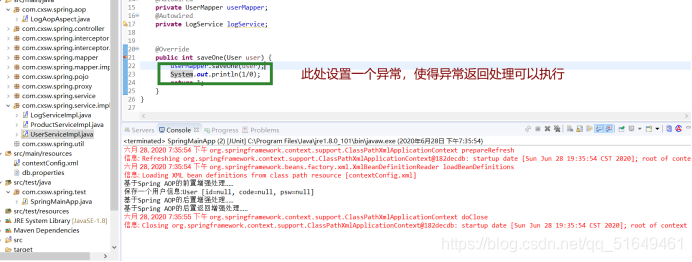
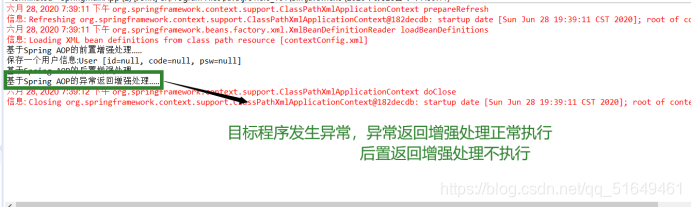
5.环绕增强处理
/**
* 5、环绕增强处理
* 如果该方法返回类型为void或者返回为null,会抛出异常:
* Null return value from advice does not match primitive return type for:
* public abstract int com.cxsw.spring.service.UserService.saveOne(com.cxsw.spring.pojo.User)
*该方法的返回值为目标方法的返回值
* 需要在该增强处理方法中手动调用目标方法 否则目标方法是不会被执行的。通过以下方式调用目标方法:
* 给该方法添加参数:ProceedingJoinPoint pt
* pt.proceed();//调用目标方法
*
* @Around表示该方法是一个环绕增强处理,可以在执行目标方法之前织入 也可以在执行目标方法之后织入
* 可以理解成@Before + @AfterReturning的总和
*
*
*/
@Around(value="execution(* com.cxsw.spring.service.impl.*.*(..))")
public Object aoundAdvice(ProceedingJoinPoint pt) {
System.out.println("基于Spring AOP的环绕增强前置处理.....");
Object res = null;
try {
res=pt.proceed();//调用目标方法 返回目标方法的返回结果
} catch (Throwable e) {
e.printStackTrace();
}
System.out.println("基于Spring AOP的环绕增强后置处理.....");
return res;
}
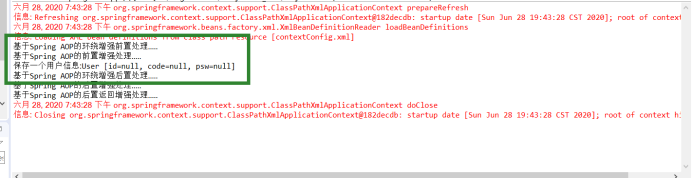
二、基于xml的Spring AOP的五种增强处理
package com.cxsw.spring.aop;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.Signature;
import org.aspectj.lang.annotation.After;
import org.aspectj.lang.annotation.AfterReturning;
import org.aspectj.lang.annotation.AfterThrowing;
import org.aspectj.lang.annotation.Around;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.aspectj.lang.annotation.Pointcut;
import org.springframework.stereotype.Component;
/**
* 定义日志的切面
* @author Administrator
*/
@Component
public class LogAopAspect {
public void beforeAdvice(JoinPoint jp) {
//1、获取目标方法的参数
Object[] args=jp.getArgs();
//2、获取目标方法名称
Signature st=jp.getSignature();
String methodName=st.getName();
System.out.println("基于Spring AOP的前置增强处理....."+args[0]+" "+methodName);
}
public void afterAdvice() {
System.out.println("基于Spring AOP的后置增强处理.....");
}
public void afterReturnAdvice() {
System.out.println("基于Spring AOP的后置返回增强处理.....");
}
public void afterThrowAdvice() {
System.out.println("基于Spring AOP的异常返回增强处理.....");
}
public Object aoundAdvice(ProceedingJoinPoint pt) {
System.out.println("基于Spring AOP的环绕增强前置处理.....");
Object res = null;
try {
res=pt.proceed();//调用目标方法 返回目标方法的返回结果
} catch (Throwable e) {
e.printStackTrace();
}
System.out.println("基于Spring AOP的环绕增强后置处理.....");
return res;
}
}
<!--aop的基于xml的配置 -->
<!--注册一个切面bean -->
<bean id="mylogAop" class="com.cxsw.spring.aop.LogAopAspect"/>
<aop:config>
<!--定义切点表达式 -->
<aop:pointcut expression="execution(* com.cxsw.spring.service.impl.*.*(..))" id="myPointCut"/>
<!--定义切面 -->
<aop:aspect id="myAop" ref="mylogAop">
<!--定义增强处理 -->
<aop:before method="beforeAdvice" pointcut-ref="myPointCut"/>
<aop:after method="afterAdvice" pointcut-ref="myPointCut"/>
<aop:after-returning method="afterReturnAdvice" pointcut-ref="myPointCut"/>
<aop:after-throwing method="afterThrowAdvice" pointcut-ref="myPointCut"/>
<aop:around method="aoundAdvice" pointcut-ref="myPointCut"/>
</aop:aspect>
</aop:config>
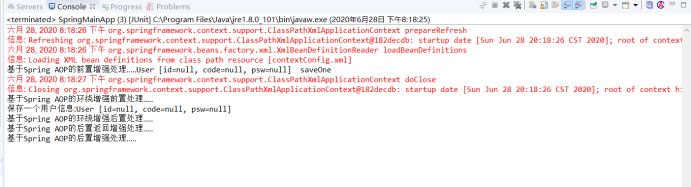