复制以下代码,直接跑就行啦
效果图如下
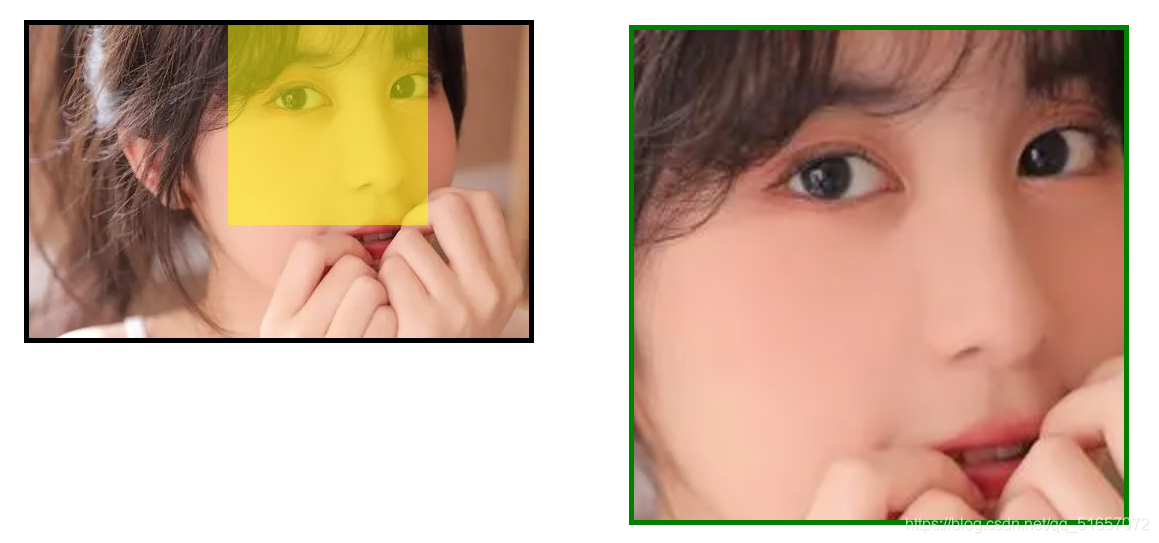
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
.boxOne {
position:relative;
width: 510px;
height: 323px;
border:5px solid #000000;
margin: 100px 0 0 100px;
}
.boxTwo {
display: none;
position: absolute;
top: 0;
left: 0;
width: 200px;
height: 200px;
background-color: yellow;
opacity: .5;
cursor: move;
}
.boxThree {
display: none;
position:absolute;
top: 0;
left: 600px;
width: 500px;
height: 500px;
border: 5px solid green;
overflow: hidden;
}
.boxThree .bigImg {
width:1000px;
height:626px;
position:absolute;
top: 0;
left: 0;
}
</style>
</head>
<body>
<div class="boxOne">
<img class="smallImg" src="https://img1.baidu.com/it/u=954496120,1621506021&fm=26&fmt=auto&gp=0.jpg" alt="">
<div class="boxTwo"> </div>
<div class="boxThree">
<img class="bigImg" src="https://img1.baidu.com/it/u=954496120,1621506021&fm=26&fmt=auto&gp=0.jpg" alt="">
</div>
</div>
<script>
window.onload = function () {
var boxOne = document.querySelector('.boxOne');
var boxTwo = document.querySelector('.boxTwo');
var boxThree = document.querySelector('.boxThree');
var smallImg = document.querySelector('.smallImg');
var bigImg = document.querySelector('.bigImg');
boxOne.onmouseover = function () {
boxTwo.style.display = 'block';
boxThree.style.display = 'block';
}
boxOne.onmouseout = function () {
boxTwo.style.display = 'none';
boxThree.style.display = 'none';
}
boxOne.onmousemove = function (e) {
//鼠标在boxOne里面的坐标 = 鼠标在页面的坐标 - 当前盒子(.boxOne)到父盒子的距离
var x = e.pageX - this.offsetLeft;
var y = e.pageY - this.offsetTop;
//让鼠标在boxTwo这个盒子内中间 = x/y - boxTwo盒子的二分之一
var boxTwoX = x - boxTwo.offsetWidth / 2;
var boxTwoY = y - boxTwo.offsetHeight / 2;
//boxTwo的最大移动距离 = boxOne的宽度/高度 - boxTwo的宽度/高度 10是两个边框5px
var boxTwoMaxX = boxOne.offsetWidth - boxTwo.offsetWidth - 10;
var boxTwoMaxY = boxOne.offsetHeight - boxTwo.offsetHeight - 10;
//如果boxTwoX(鼠标的坐标为0 的话,boxTwo盒子的宽度/高度会出现在盒子外面) 当boxTwoX小于0,就等于0
//如果boxTwoX(鼠标的坐标超出最大移动距离的话,boxTwo盒子的宽度/高度会出现在盒子外面) 当boxTwoX大于最大移动距离,就等于他
if (boxTwoX <= 0) {
boxTwoX = 0;
}else if (boxTwoX >= boxTwoMaxX) {
boxTwoX = boxTwoMaxX
}
if (boxTwoY <= 0) {
boxTwoY = 0;
}else if (boxTwoY >= boxTwoMaxY) {
boxTwoY = boxTwoMaxY
}
//最后把值赋给绝对定位的boxTwo
boxTwo.style.left = boxTwoX + 'px';
boxTwo.style.top = boxTwoY + 'px';
//相对于右边的boxThree盒子的操作
var bigImgMaxX = boxThree.offsetWidth - bigImg.offsetWidth - 10;
var bigImgMaxY = boxThree.offsetHeight - bigImg.offsetHeight - 10;
//右边boxThree的img移动距离 = boxTwo的移动距离 * boxThree的img最大移动距离 / boxTwo最大移动距离
var bigImgX = boxTwoX * bigImgMaxX / boxTwoMaxX;
var bigImgY = boxTwoY * bigImgMaxY / boxTwoMaxY;
bigImg.style.left = bigImgX + 'px';
bigImg.style.top = bigImgY + 'px';
}
}
</script>
</body>
</html>