一、 stack的介绍和使用
1. stack的介绍
stack
的文档介绍
翻译:
1. stack
是一种容器适配器,专门用在具有后进先出操作的上下文环境中,其删除只能从容器的一端进行元素的插入与提取操作。
2. stack
是作为容器适配器被实现的,容器适配器即是对特定类封装作为其底层的容器,并提供一组特定的成员函数来访问其元素,将特定类作为其底层的,元素特定容器的尾部(
即栈顶
)
被压入和弹出。
3. stack
的底层容器可以是任何标准的容器类模板或者一些其他特定的容器类,这些容器类应该支持以下操作:
- empty:判空操作
- back:获取尾部元素操作
- push_back:尾部插入元素操作
- pop_back:尾部删除元素操作
4.
标准容器
vector
、
deque
、
list
均符合这些需求,默认情况下,如果没有为
stack
指定特定的底层容器,默认情况下使用deque
。
栈和队列严格来说已经不是容器,而是容器适配器。
二、queue的介绍和使用
1. queue的介绍
queue
的文档介绍
翻译:
1.
队列是一种容器适配器,专门用于在
FIFO
上下文
(
先进先出
)
中操作,其中从容器一端插入元素,另一端 提取元素。
2.
队列作为容器适配器实现,容器适配器即将特定容器类封装作为其底层容器类,
queue
提供一组特定的成员函数来访问其元素。元素从队尾入队列,从队头出队列。
3.
底层容器可以是标准容器类模板之一,也可以是其他专门设计的容器类。该底层容器应至少支持以下操作:
- empty:检测队列是否为空
- size:返回队列中有效元素的个数
- front:返回队头元素的引用
- back:返回队尾元素的引用
- push_back:在队列尾部入队列
- pop_front:在队列头部出队列
4.
标准容器类
deque
和
list
满足了这些要求。默认情况下,如果没有为
queue
实例化指定容器类,则使用标准容器deque
。
三、什么是适配器
适配器是一种设计模式
(
设计模式是一套被反复使用的、多数人知晓的、经过分类编目的、代码设计经验的总结)
,该种模式是将一个类的接口转换成客户希望的另外一个接口。
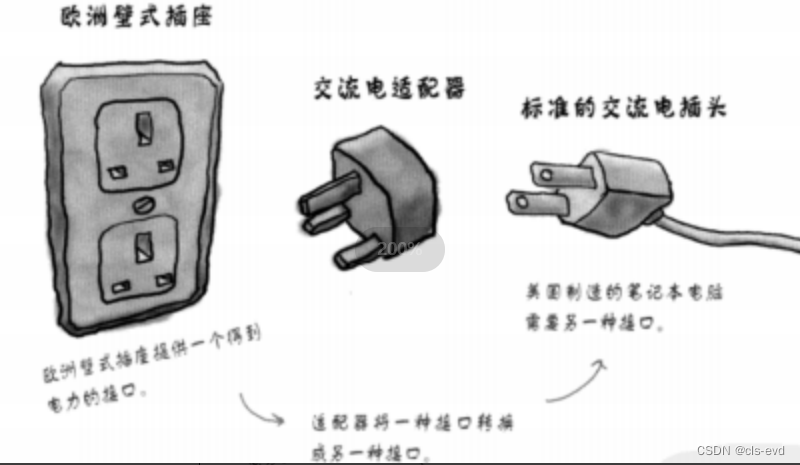
STL
标准库中
stack
和
queue
的底层结构
虽然
stack
和
queue
中也可以存放元素,但在
STL
中并没有将其划分在容器的行列,而是将其称为
容器适配
器
,这是因为
stack
和队列只是对其他容器的接口进行了包装,
STL
中
stack
和
queue
默认使用
deque。
四、stack的模拟实现
早期写一个栈底层可以用vector,同样也可以使用list,但是这样就写死了,只能用vector或者list。不能灵活的适配,比如说:手机充电器,只要都是typeC口的,10瓦的你可以用,20瓦,40瓦的你同样可以用。类比到这里,就相当于10瓦的你可以用,20瓦的,40瓦的...你就不可以用了,所以非常不灵活。![]()
所以我们就可以增加一个模板参数Container,只要你这个Container支持push_back和pop_back就可以了
你想要是数组栈或者是链栈都可以,想用啥用啥。
模拟实现
namespace pxl
{
//deque<T> 就是一个缺省值
template<class T,class Container=deque<T>>
class stack
{
public:
bool empty() const
{
return con_.empty();
}
size_t size() const
{
return con_.size();
}
const T& top()
{
return con_.back();
}
void push(const T& x)
{
con_.push_back(x);
}
void pop()
{
con_.pop_back();
}
private:
Container con_;
};
void test()
{
//stack<int, std::list<int>> s2;
//stack<int, std::vector<int>> s;
stack<int> s;
s.push(1);
s.push(2);
s.push(3);
s.push(4);
while (!s.empty())
{
cout << s.top() << " ";
s.pop();
}
cout << endl;
}
}
测试:
适配器传string可以不可以呢?
也可以。因为string同样支持这些的接口。但是会发生问题。string可以存储整形,因为int char都是整形,只不过存储的时候会发生截断,int4字节,char1字节,截断就会导致溢出。刚才只是巧合
或者改成string就直接报错了
五、queue的模拟实现
namespace pxl
{
template<class T, class Container = deque<T>>
class queue
{
public:
bool empty() const
{
return con_.empty();
}
size_t size() const
{
return con_.size();
}
const T& back() const
{
return con_.back();
}
const T& front() const
{
return con_.front();
}
void push(const T& x)
{
con_.push_back(x);
}
void pop()
{
con_.pop_front();
}
private:
Container con_;
};
void test_queue()
{
queue<int,list<int>> s;
s.push(1);
s.push(2);
s.push(3);
s.push(400);
while (!s.empty())
{
cout << s.front() << " ";
s.pop();
}
cout << endl;
}
}
测试
queue的适配器就不能用vector了,因为vector没push_front这样的接口
![]()