UI界面
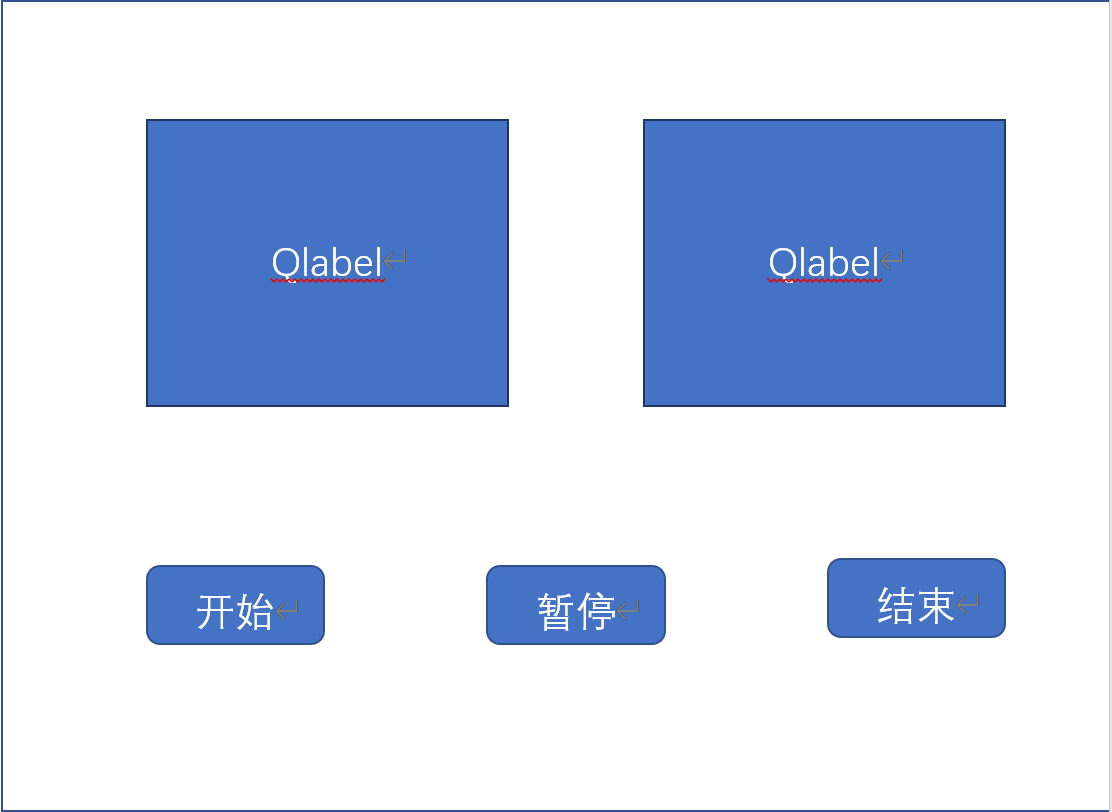
功能要求
1. 两个线程同时按指定顺序读取文件(图片) 需要注意线程冲突
2.读取的文件显示在主窗口的两个QLabel当中
3. 开始按钮 实现启动线程,开始更新图片
4. 暂停按钮 实现图片暂停刷新
5. 结束按钮实现停止刷新图片,退出线程
代码实现
mainwindow.h:
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include <QMessageBox>
#include <QDebug>
#include <QTimer>
#include <QSemaphore>
#include <synchapi.h>
//#include <mythread.h>
QT_BEGIN_NAMESPACE
namespace Ui { class MainWindow; }
QT_END_NAMESPACE
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr);
~MainWindow();
private:
QTimer *timer;
QSemaphore *sem1,*sem2;
QThread *pth1,*pth2;
private slots:
void cdPicture();
void on_btn_start_clicked();
void on_btn_stop_clicked();
void on_btn_end_clicked();
public:
Ui::MainWindow *ui;
};
#endif // MAINWINDOW_H
mythread.h:
#ifndef MYTHREAD_H
#define MYTHREAD_H
#include <QThread>
#include <QImage>
#include <QMessageBox>
#include <QSemaphore>
#include <mainwindow.h>
class Mythread:public QThread
{
Q_OBJECT
public:
explicit Mythread(QObject *parent,QLabel *label,QSemaphore *sem_p,QSemaphore *sem_v);
private:
static int count;
MainWindow *parent;
QLabel *label;
QSemaphore *sem_p,*sem_v;
protected:
void run();
signals:
public slots:
};
#endif // MYTHREAD_H
mainwindow.cpp:
#include "mainwindow.h"
#include "ui_mainwindow.h"
#include "mythread.h"
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
, ui(new Ui::MainWindow)
{
ui->setupUi(this);
//设置按钮样式
ui->btn_stop->setStyleSheet("border:2px;border-radius:10px;background-color:#d9d9d9;color:#ffffff");
ui->btn_end->setStyleSheet("border:2px;border-radius:10px;background-color:#d9d9d9;color:#ffffff");
ui->btn_start->setStyleSheet("border:2px;border-radius:10px;background-color:#00007f;color:#ffffff");
//初始化信号量
this->sem1=new QSemaphore(1);
this->sem2=new QSemaphore(0);
this->pth1=this->pth2=nullptr;
}
MainWindow::~MainWindow()
{
delete ui;
}
void MainWindow::cdPicture()
{
if(pth1==nullptr && pth2==nullptr)
{
pth1=new Mythread(this,this->ui->label_1,this->sem1,this->sem2);
pth2=new Mythread(this,this->ui->label_2,this->sem2,this->sem1);
}
pth1->start();
pth2->start();
}
void MainWindow::on_btn_start_clicked()
{
//设置按钮可用状态和样式
this->ui->btn_start->setEnabled(false);
this->ui->btn_end->setEnabled(true);
this->ui->btn_stop->setEnabled(true);
ui->btn_start->setStyleSheet("border:2px;border-radius:10px;background-color:#d9d9d9;color:#ffffff");
ui->btn_end->setStyleSheet("border:2px;border-radius:10px;background-color:#00007f;color:#ffffff");
ui->btn_stop->setStyleSheet("border:2px;border-radius:10px;background-color:#00007f;color:#ffffff");
timer=new QTimer(this);
connect(timer,SIGNAL(timeout()),this,SLOT(cdPicture()));
timer->start(500);
}
void MainWindow::on_btn_stop_clicked()
{
if(this->ui->btn_stop->text()=="暂停")
{
timer->stop();
this->ui->btn_stop->setText("继续");
}else{
timer->start();
this->ui->btn_stop->setText("暂停");
}
}
void MainWindow::on_btn_end_clicked()
{
//设置按钮可用状态和样式
this->ui->btn_start->setEnabled(true);
this->ui->btn_end->setEnabled(false);
ui->btn_start->setStyleSheet("border:2px;border-radius:10px;background-color:#00007f;color:#ffffff");
ui->btn_end->setStyleSheet("border:2px;border-radius:10px;background-color:#d9d9d9;color:#ffffff");
ui->btn_stop->setStyleSheet("border:2px;border-radius:10px;background-color:#d9d9d9;color:#ffffff");
this->timer->stop();
this->ui->btn_stop->setText("暂停");
this->ui->btn_stop->setEnabled(false);
this->pth1->quit();
this->pth2->quit();
delete this->pth1;
delete this->pth2;
this->pth1=nullptr;
this->pth2=nullptr;
}
mythread.cpp:
#include "mythread.h"
#include <QDebug>
#include "mainwindow.h"
#include "ui_mainwindow.h"
int Mythread::count=1;
Mythread::Mythread(QObject *parent,QLabel *label,QSemaphore *sem_p,QSemaphore *sem_v):QThread(parent)
{
this->parent=(MainWindow *)parent;
this->label=label;
this->sem_p=sem_p;
this->sem_v=sem_v;
count=1;
}
void Mythread::run()
{
sem_p->acquire(1);
QString name;
switch(count)
{
case 1:
name=":/image/1.png";
break;
case 2:
name=":/image/2.png";
break;
case 3:
name=":/image/3.png";
break;
case 4:
name=":/image/4.png";
break;
case 5:
name=":/image/5.png";
break;
case 6:
name=":/image/6.png";
break;
case 7:
name=":/image/7.png";
break;
case 8:
name=":/image/8.png";
break;
case 9:
name=":/image/9.png";
break;
case 10:
name=":/image/10.png";
break;
}
QString filename(name);
QImage* img=new QImage;
img->load(filename);
this->label->setPixmap(QPixmap::fromImage(*img));
delete img;
parent->update();
count=count%10+1;
sem_v->release(1);
}