一系列环境配置参考第一弹的
内容:写了一个简单的后台管理系统页面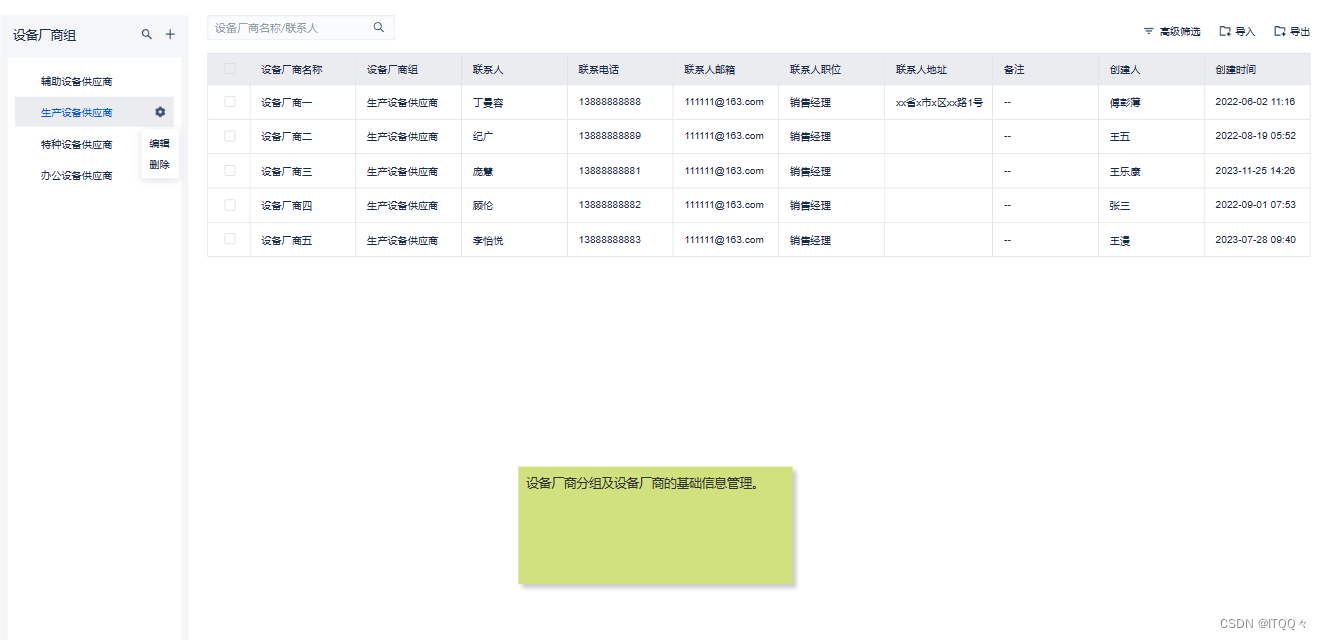
Controller层
DeviceFactoryGroup(设备组)
/**
* Created with IntelliJ IDEA.
*
* @Author: syq
* @Date: 2024/06/28/17:19
* @Description:DeviceFactoryGroup设备组控制层
* update操作id和name都不能为空
* add操作name不能为空
*/
@RestController
@RequestMapping("deviceFactoryGroup")
public class DeviceFactoryGroupController {
@Autowired
private DeviceFactoryGroupService deviceFactoryGroupService;
@GetMapping("findAllByName")
public Result findAllByName(String name){
List<DeviceFactoryGroup> factoryGroups = deviceFactoryGroupService.findAllByName(name);
// 判断是否有数据,健壮性
if (factoryGroups.size() == 0 || factoryGroups == null){
return Result.failed("暂无数据");
}else {
return Result.success(factoryGroups);
}
}
@PostMapping("add")
public Result add(@RequestBody DeviceFactoryGroup deviceFactoryGroup){
// 页面效果图需要显示,所以名称不能为空
if (!StringUtils.hasText(deviceFactoryGroup.getName())){
return Result.failed("名称不能为空");
}
deviceFactoryGroupService.add(deviceFactoryGroup);
return Result.success("添加成功",deviceFactoryGroup);
}
// /deviceFactoryGroup/deleteById/9在地址上写
@DeleteMapping("deleteById/{id}")
public Result deleteById(@PathVariable Integer id){
if(deviceFactoryGroupService.deleteById(id)){
return Result.success("删除成功",null);
}else {
return Result.failed("删除失败");
}
}
// 可以 ---GetMapping请求请求行传参 ---PostMapping请求体传参也可以
// @PostMapping(value = "deleteById/id")
// public Result deleteById(Integer id){
// if(deviceFactoryGroupService.deleteById(id)){
// return Result.success("删除成功",null);
// }else {
// return Result.failed("删除失败");
// }
// }
// GetMapping请求请求行传参 --- PostMapping不行
// @GetMapping(value = "deleteById/{id}")
// public Result deleteById(@PathVariable Integer id){
// if(deviceFactoryGroupService.deleteById(id)){
// return Result.success("删除成功",null);
// }else {
// return Result.failed("删除失败");
// }
// }
@PutMapping("update")
public Result update(@RequestBody DeviceFactoryGroup deviceFactoryGroup){
if(deviceFactoryGroupService.update(deviceFactoryGroup)){
return Result.success("修改成功",null);
}else{
return Result.failed("修改失败");
}
}
}
DeviceFactory(设备)
@RestController
@RequestMapping("devicefactory")
public class DeviceFactoryController {
@Autowired
private DeviceFactoryService deviceFactoryService;
@PostMapping("add")
public Result add(@RequestBody DeviceFactory deviceFactory){
deviceFactoryService.add(deviceFactory);
return Result.success("添加成功",deviceFactory);
}
// groupId 是设备组的id keyword是设备厂商名称或者是联系人名
@GetMapping("findByGroupId")
public Result findByGroupId(Integer groupId,String keyword){
List<DeviceFactory> deviceFactories = deviceFactoryService.findByGroupId(groupId,keyword);
if(deviceFactories == null || deviceFactories.size() == 0){
return Result.failed("暂无数据");
}else {
return Result.success(deviceFactories);
}
}
// @GetMapping("findByGroupId")----错误写法
// public Result findByGroupId(@RequestBody Integer groupId,String keyword){
// List<DeviceFactory> deviceFactories = deviceFactoryService.findByGroupId(groupId,keyword);
// if(deviceFactories == null || deviceFactories.size() == 0){
// return Result.failed("暂无数据");
// }else {
// return Result.success(deviceFactories);
// }
//
// }
}
Service和Impl
DeviceFactoryGroup(设备组)
public interface DeviceFactoryGroupService {
List<DeviceFactoryGroup> findAllByName(String name);
boolean add(DeviceFactoryGroup deviceFactoryGroup);
boolean deleteById(Integer id);
boolean update(DeviceFactoryGroup deviceFactoryGroup);
}
----------------------------------------------------------------
@Service("deviceFactoryGroupService")
public class DeviceFactoryGroupServiceImpl implements DeviceFactoryGroupService {
@Autowired
private DeviceFactoryGroupMapper deviceFactoryGroupMapper;
@Override
public List<DeviceFactoryGroup> findAllByName(String name) {
return deviceFactoryGroupMapper.findAllByName(name);
}
@Override
public boolean add(DeviceFactoryGroup deviceFactoryGroup) {
return deviceFactoryGroupMapper.add(deviceFactoryGroup);
}
@Override
public boolean deleteById(Integer id) {
return deviceFactoryGroupMapper.deleteById(id);
}
@Override
public boolean update(DeviceFactoryGroup deviceFactoryGroup) {
if(deviceFactoryGroup.getId() == null){
throw new RuntimeException("修改失败,id不能为空");
}
if(deviceFactoryGroup.getName() == null){
throw new RuntimeException("修改失败,name不能为空");
}
return deviceFactoryGroupMapper.update(deviceFactoryGroup);
}
}
DeviceFactory(设备)
public interface DeviceFactoryService {
boolean add(DeviceFactory deviceFactory);
List<DeviceFactory> findByGroupId(Integer groupId, String keyword);
}
---------------------------------------------------------
@Service("deviceFactoryService")
public class DeviceFactoryServiceImpl implements DeviceFactoryService {
@Autowired
private DeviceFactoryMapper deviceFactoryMapper;
@Override
public boolean add(DeviceFactory deviceFactory) {
deviceFactory.setCreateId(1);
deviceFactory.setUpdateId(1);
return deviceFactoryMapper.add(deviceFactory);
}
@Override
public List<DeviceFactory> findByGroupId(Integer groupId, String keyword) {
return deviceFactoryMapper.findByGroupId(groupId,keyword);
}
}
mapper层及其实现mapper.xml
DeviceFactoryGroup(设备组)
public interface DeviceFactoryGroupMapper extends BaseMapper<DeviceFactoryGroup>{
List<DeviceFactoryGroup> findAllByName(String name);
}
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.itqq.mapper.DeviceFactoryGroupMapper">
<select id="findAllByName" resultType="DeviceFactoryGroup">
SELECT * FROM device_factory_group
<where>
<if test="name != null and name != ''">name LIKE CONCAT('%',#{name},'%')</if>
</where>
</select>
<!-- useGeneratedKeys="true"
当它的值为 "true" 时,指示MyBatis在执行插入语句后应该获取由数据库自动生成的主键(如自增列)的值。 -->
<!-- keyProperty="id" 与 useGeneratedKeys="true" 配合使用
"id" 指的是Java实体类中的属性名,这个属性用来接收从数据库生成并返回的主键值。
例如,如果你有一个用户类 User,其中包含一个主键属性 id,那么设置 keyProperty="id"
就意味着MyBatis会自动将新插入记录的ID填充到 User 对象的 id 属性中-->
<!-- parameterType="DeviceFactoryGroup"
是MyBatis映射文件中 <insert>, <update>, <delete>, <select> 等标签的一个属性,
用于指定传入SQL语句的参数类型。这个属性告诉MyBatis如何解析传入的方法参数,即将Java对象映射到SQL语句中的参数。-->
<insert id="add" useGeneratedKeys="true" keyProperty="id" parameterType="DeviceFactoryGroup">
insert into device_factory_group values (null , #{name})
</insert>
<delete id="deleteById" >
delete from device_factory_group where id = #{id}
</delete>
<update id="update" parameterType="DeviceFactoryGroup">
update device_factory_group
set
name = #{name}
where id = #{id}
</update>
</mapper>
DeviceFactory(设备)
public interface DeviceFactoryMapper extends BaseMapper <DeviceFactory> {
List<DeviceFactory> findByGroupId(@Param("groupId") Integer groupId,@Param("keyword") String keyword);
}
user表是之前一个项目中的表,不想要可以删掉--无影响
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.itqq.mapper.DeviceFactoryMapper">
<insert id="add" keyProperty="id" useGeneratedKeys="true">
insert into device_factory values
(
null , #{name},#{relationName},#{relationPhone},
#{relationEmail},#{relationJob},#{relationAddress},
#{remark},#{deviceFactoryGroupId},#{createId},
now(),#{updateId},now()
);
</insert>
<select id="findByGroupId" resultType="DeviceFactory">
SELECT
df.* ,dfg.name device_factory_group_name,u.username create_name
from device_factory df
LEFT JOIN device_factory_group dfg on df.deviceFactoryGroupId = dfg.id
LEFT JOIN user u on df.createId = u.id
<where>
deviceFactoryGroupId = #{groupId}
<if test="keyword != null and keyword != ''">
AND (
df.`name` like CONCAT('%',#{keyword},'%')
or
df.relation_Name like CONCAT('%',#{keyword},'%')
)
</if>
</where>
</select>
</mapper>
BaseMapper
public interface BaseMapper <E>{
List<E> findAll();
E findById(Integer id);
boolean add(E e);
boolean update(E e);
boolean deleteById(Integer id);
}
学习的新内容
配置一个异常处理控制,这样service抛异常的时候就会被捕获,打印错误信息.Exception一种错误类型,也可以定义其他的错误类型,向下包含
//@ResponseBody
//@ControllerAdvice
@RestControllerAdvice
public class ErrorController {
// Exception.class 当出现Exception类型的异常时,会自动进入到这个异常类,返回报错信息
// service就不用写try...catch...
@ExceptionHandler(Exception.class)
public Result error1(Exception exception){
return Result.failed(exception.getMessage());
}
}
例如:会被 ErrorController捕获
@Override
public boolean update(DeviceFactoryGroup deviceFactoryGroup) {
if(deviceFactoryGroup.getId() == null){
throw new RuntimeException("修改失败,id不能为空");
}
if(deviceFactoryGroup.getName() == null){
throw new RuntimeException("修改失败,name不能为空");
}
return deviceFactoryGroupMapper.update(deviceFactoryGroup);
}
StringUtils.hasText(deviceFactoryGroup.getName() )和supplierGroup .getName() == null || supplierGroup.getName() == ""的区别
StringUtils.hasText(deviceFactoryGroup.getName())
:这个方法来自Apache Commons Lang库,它会检查给定字符串是否有非空白内容。这意味着如果字符串为null
、空字符串(""
)或者只包含空白字符(如空格、制表符等),此方法都会返回false
。只有当字符串中至少有一个非空白字符时,它才返回true
。而
supplierGroup.getName() == null || supplierGroup.getName() == ""
:这个表达式直接在代码中进行了逻辑检查,它检查字符串是否为null
或空字符串(""
)。这个表达式不会关心字符串中是否只包含空白字符(例如,一个只包含空格的字符串会通过这个检查)。因此,如果你希望同时排除
null
、空字符串以及只含空白字符的字符串,StringUtils.hasText(...)
是更合适的选择。如果你只关心排除null
和空字符串,则后者可能已经足够。但在很多应用场景下,两者可能被用作类似目的,只是StringUtils.hasText()
提供了更全面的“空值”检查。
上述接口均经过Apifox测试通过。自学记录!!!