一、案例简介
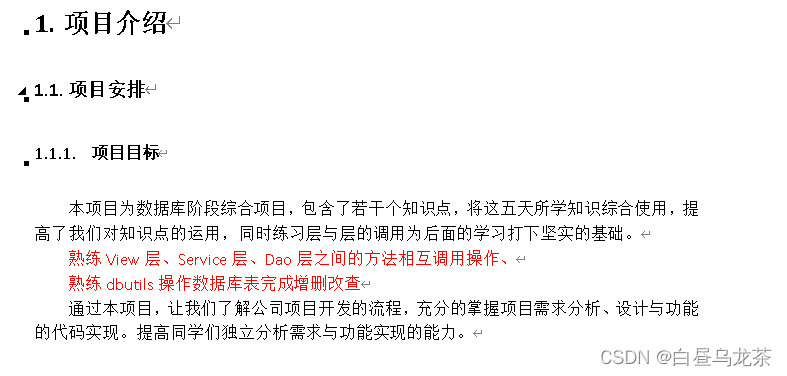
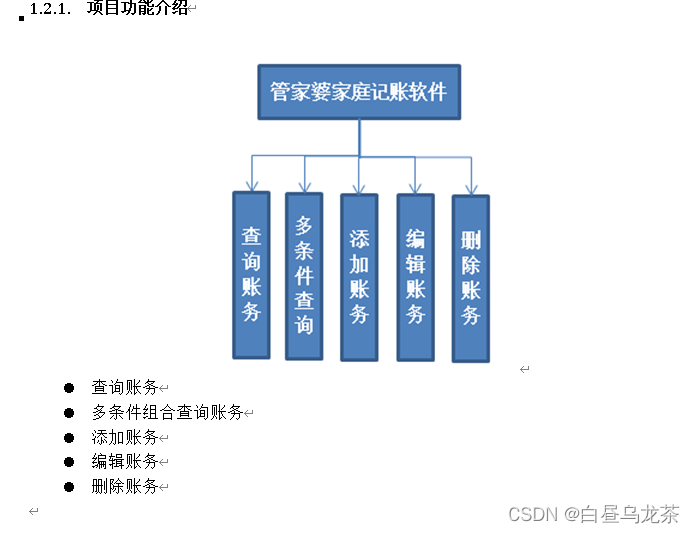
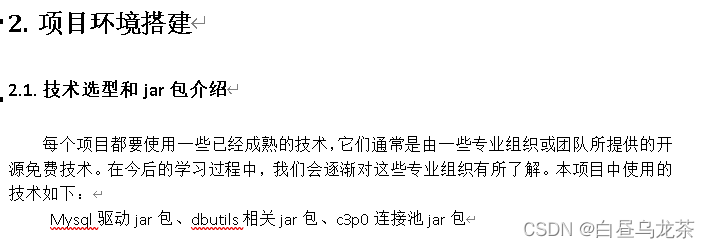
二、环境搭建
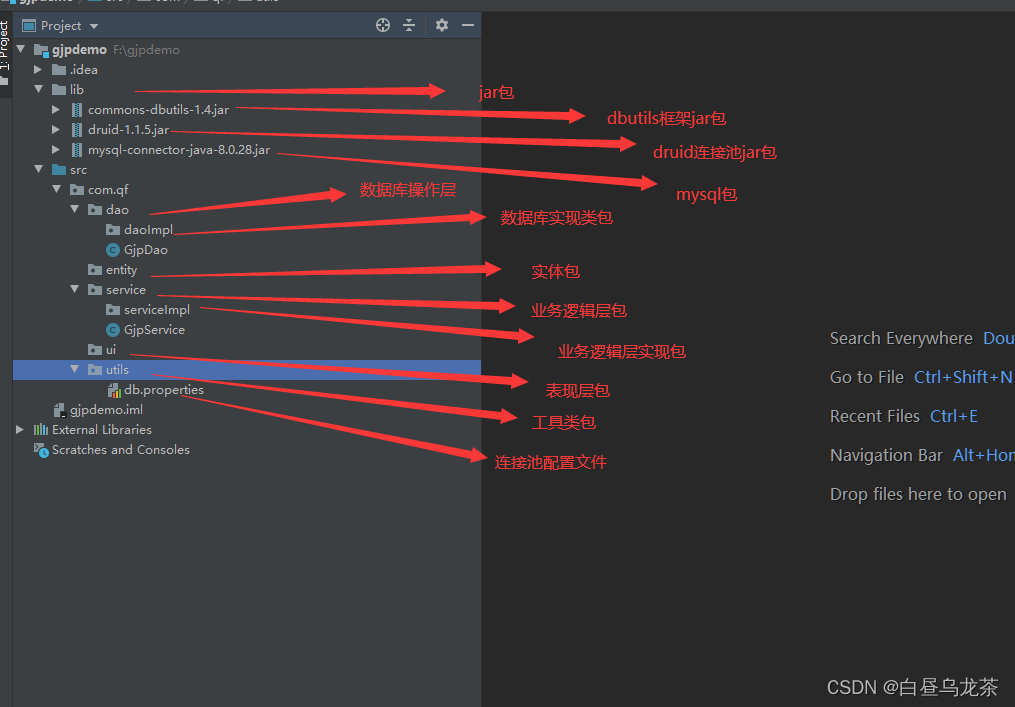
三、代码
配置文件:
# druid.properties文件的配置
driverClassName=com.mysql.cj.jdbc.Driver
url=jdbc:mysql://localhost:3306/gjpdb
username=root
password=123456
# 初始化连接数量
initialSize=5
# 最大连接数
maxActive=10
# 最大超时时间
maxWait=3000
工具类:
package com.qf.utils;
import com.alibaba.druid.pool.DruidDataSourceFactory;
import org.apache.commons.dbutils.QueryRunner;
import javax.sql.DataSource;
import java.util.Properties;
public enum DBUtils {
DB;
//定义QueryRunner对象
public QueryRunner qr;
DBUtils(){
try {
//实例化Properties对象
Properties pro = new Properties();
//加载配置文件
pro.load(DBUtils.class.getResourceAsStream("db.properties"));
//获取数据源对象
DataSource ds = DruidDataSourceFactory.createDataSource(pro);
//实例化QueryRunner对象
qr = new QueryRunner(ds);
} catch (Exception e) {
e.printStackTrace();
}
}
}
dao接口:
package com.qf.dao;
import com.qf.entity.Gjp;
import java.util.List;
public interface GjpDao {
//添加账务
int addGjp(Gjp gjp);
//查询账务-按时间
List<Gjp> selectTime(String startTime,String endTime);
//查询账务-查询所有
List<Gjp> selectAll();
//编辑账务
int updataGjp(Gjp gjp);
//删除账务
int deleteGjp(int zwid);
}
dao实现类:
package com.qf.dao.daoImpl;
import com.qf.dao.GjpDao;
import com.qf.entity.Gjp;
import com.qf.utils.DBUtils;
import org.apache.commons.dbutils.QueryRunner;
import org.apache.commons.dbutils.handlers.BeanListHandler;
import java.sql.SQLException;
import java.util.Date;
import java.util.List;
public class GjpDaoImpl implements GjpDao{
//实例化QueryRunner对象
private QueryRunner qr = DBUtils.DB.qr;
@Override
public int addGjp(Gjp gjp) {
int num = 0;
try {
//编写SQL语句
String sql = "insert into gjp(flname,money,zhangHu,createtime,description) values(?,?,?,?,?)";
//给占位符赋值
Object [] objects = {gjp.getFlname(),gjp.getMoney(),gjp.getZhanghu(),gjp.getCreatetime(),gjp.getDescription()};
//提交SQL语句
num = qr.update(sql, objects);
} catch (SQLException e) {
e.printStackTrace();
}
return num;
}
@Override
public List<Gjp> selectTime(String startTime, String endTime) {
List<Gjp> gjpList = null;
try {
//编写SQL语句
String sql = "select * from gjp where createtime>=? and createtime<=?";
//给占位符赋值
Object [] objects={startTime,endTime};
//提交SQL语句
gjpList = qr.query(sql, new BeanListHandler<Gjp>(Gjp.class), objects);
} catch (SQLException e) {
e.printStackTrace();
}
return gjpList;
}
@Override
public List<Gjp> selectAll() {
List<Gjp> gjpList = null;
try {
//编写SQL语句
String sql = "select * from gjp";
//提交SQL语句
gjpList = qr.query(sql, new BeanListHandler<Gjp>(Gjp.class));
} catch (SQLException e) {
e.printStackTrace();
}
return gjpList;
}
@Override
public int updataGjp(Gjp gjp) {
int num = 0;
try {
//编写SQL语句
String sql = "update gjp set flname=?,money=?,zhanghu=?,createtime=?,description=? where zwid=?";
//给占位符赋值
Object [] objects={gjp.getFlname(),gjp.getMoney(),gjp.getZhanghu(),gjp.getCreatetime(),gjp.getDescription(),gjp.getZwid()};
//提交SQL语句
num = qr.update(sql, objects);
} catch (SQLException e) {
e.printStackTrace();
}
return num;
}
@Override
public int deleteGjp(int zwid) {
int num=0;
try {
//编写SQL语句
String sql = "delete from gjp where zwid=?";
Object [] objects={zwid};
//提交SQL语句
num = qr.update(sql,objects);
} catch (SQLException e) {
e.printStackTrace();
}
return num;
}
}
service接口:
package com.qf.service;
import com.qf.entity.Gjp;
import java.util.List;
public interface GjpService {
//添加账务
int addGjp(Gjp gjp);
//查询账务-按时间
List<Gjp> selectTime(String startTime, String endTime);
//查询账务-查询所有
List<Gjp> selectAll();
//编辑账务
int updataGjp(Gjp gjp);
//删除账务
int deleteGjp(int zwid);
}
service实现类:
package com.qf.service.serviceImpl;
import com.qf.dao.GjpDao;
import com.qf.dao.daoImpl.GjpDaoImpl;
import com.qf.entity.Gjp;
import com.qf.service.GjpService;
import java.util.List;
public class GjpServiceImpl implements GjpService{
//实例化daoImpl对象
private GjpDao gjpDao = new GjpDaoImpl();
@Override
public int addGjp(Gjp gjp) {
return gjpDao.addGjp(gjp);
}
@Override
public List<Gjp> selectTime(String startTime, String endTime) {
return gjpDao.selectTime(startTime,endTime);
}
@Override
public List<Gjp> selectAll() {
return gjpDao.selectAll();
}
@Override
public int updataGjp(Gjp gjp) {
return gjpDao.updataGjp(gjp);
}
@Override
public int deleteGjp(int zwid) {
return gjpDao.deleteGjp(zwid);
}
}
GjpUtils类:
package com.qf.ui;
import com.qf.entity.Gjp;
import com.qf.service.GjpService;
import com.qf.service.serviceImpl.GjpServiceImpl;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.List;
import java.util.Scanner;
public class GjpUtils {
//实例化GjpService对象
private GjpService gjpService = new GjpServiceImpl();
//实例化Scanner
Scanner input = new Scanner(System.in);
//增
public void addGjp() throws ParseException {
System.out.print("请输入类型:");
String flname = input.next();
System.out.print("请输入账户:");
String zhanghu = input.next();
System.out.print("请输入金额:");
Double money = input.nextDouble();
System.out.print("请输入时间:");
String time=input.next();
System.out.print("请输入说明:");
String description = input.next();
//实例化gjp对象
Gjp g = new Gjp(flname,money,zhanghu,new SimpleDateFormat("yyyy-MM-dd").parse(time),description);
//调用方法
int num = gjpService.addGjp(g);
//判断是否添加成功
if (num>0){
System.out.println("添加成功");
}else {
System.out.println("添加失败");
}
}
//按时间查询
public void selectTime(){
System.out.println("请输入起始时间");
String startTime = input.next();
System.out.println("请输入结束时间");
String endTime = input.next();
//调用方法
List<Gjp> gjpList = gjpService.selectTime(startTime, endTime);
//遍历集合
for (Gjp g:gjpList){
System.out.println(g);
}
}
//全部查询
public void selectAll(){
//调用方法
List<Gjp> gjpList = gjpService.selectAll();
//遍历集合
for (Gjp g:gjpList){
System.out.println(g);
}
}
//编辑账务
public void updateGjp() throws ParseException {
System.out.print("请输入ID:");
int zwid = input.nextInt();
System.out.print("请输入新类别:");
String flname = input.next();
System.out.print("请输入新账户:");
String zhanghu=input.next();
System.out.print("请输入新金额:");
double money = input.nextDouble();
System.out.print("请输入新时间:");
String time = input.next();
System.out.print("请输入新描述:");
String description = input.next();
//实例化Gjp对象
Gjp g = new Gjp(zwid,flname,money,zhanghu,new SimpleDateFormat("yyyy-MM-dd").parse(time),description);
//调用方法
int num = gjpService.updataGjp(g);
//判断
if (num>0){
System.out.println("编辑成功");
}else {
System.out.println("编辑失败");
}
}
//删除账务
public void deleteGjp(){
System.out.print("请输入ID:");
int zwid = input.nextInt();
//调用方法
int num = gjpService.deleteGjp(zwid);
System.out.println(num);
//判断
if (num>0){
System.out.println("删除成功");
}else {
System.out.println("删除失败");
}
}
}
UIView类:
package com.qf.ui;
import java.text.ParseException;
import java.util.Scanner;
public class UIView {
public static void main(String[] args) throws ParseException {
//实例化GjpUtils对象
GjpUtils gu = new GjpUtils();
boolean flag = true;
Scanner in = new Scanner(System.in);
while (flag) {
System.out.println("---------------管家婆家庭记账软件---------------");
System.out.println("1.添加账务 2.编辑账务 3.删除账务 4.查询账务 5.退出系统");
System.out.println("请输入要操作的功能序号[1-5]:");
int op = in.nextInt();
switch (op) {
case 1:
gu.addGjp();
break;
case 2:
gu.updateGjp();
break;
case 3:
gu.deleteGjp();
break;
case 4:
System.out.println("1.查询所有记录 2.按时间条件查询");
int selectId = in.nextInt();
if (selectId==1){
gu.selectAll();
}else if (selectId==2){
gu.selectTime();
}else {
System.out.println("请输入正确选项");
}
break;
case 5:
System.out.println("再见!");
flag = false;
break;
default:
System.out.println("输入错误!");
}
}
}
}