顺序表
顺序表可以分为:1.静态顺序表:用定长数组创建
2.动态顺序表:使用动态开辟的数组存储;(创建在堆);
特点:1.连续的物理空间
2.数据必须是从头开始,依次存储。
1.静态顺序表
1.1.宏定义和结构定义、
#define MAX_SIZE 10
typedef int SQDataType; //定义出顺序表存储的数据类型
typedef struct SeqList
{
SQDataType a[MAX_SIZE]; //SQDataType表示数据类型
int size; //有效数据的个数
}SL;
1.2.增删查改接口函数
//顺序表初始化函数
void SeqListInit(SL*ps);
{
memset(ps->a,0,sizeof(SQDataType)*N);
ps->size=0;
}
//SQDatatype表示数组的数据类型
//尾插函数
void SeqListPushback(SL*ps,SQDataType x)
{
assert(ps);
if(ps->size>=MAX_SIZE)
{
return;
}
ps->a[ps->size]=x;
ps->size++;
}
//头插函数
void SeqListpushfront(SL*ps,SQDataType x)
{
assert(ps);
if(ps->size>=MAX_SIZE)
{
return;
}
for(int i=size;i>0;i--)
{
ps->a[i]=ps->a[i-1];
}
ps->a[0]=x;
ps->size++;
}
//头删函数
void SeqListPopback(SL*ps)
{
assert(ps);
if(ps->size==0)
return ;
for(int i=0;i<sz;i++)
{
ps->a[i]=ps->a[i+1];
}
ps->size--;
}
//尾删函数
void SeqListPopfront(SL*ps)
{
assert(ps);
if(ps->size==0)
return ;
ps->a[ps-size-1]=0;
ps->size--;
}
2.动态顺序表
2.1宏定义和结构体定义
typedef int QDataType; //定义出顺序表存储的数据类型
typedef struct SeqList
{
QDataType *a; //SQDataType表示数据类型
int size; //有效数据的个数
int capacity //最大容量
}SL;
2.2初始化和接口函数
void SeqListInit(SL*ps)
{
ps->a=NULL;
PS->size=0;
ps->capacity=4;
}
//检查容量函数
void SeqListCheckCapacity(SL*ps)
{
if(ps->size==ps->capacity)
{
QDataType*tmp=(QDataType*)realloc(ps->a,ps->capacity*2*sizeof(QDataType));
if(tmp==NULL){
printf("realloc fail\n");
exit(-1);
}
else
{
ps->a=tmp;
ps->capacity*=2;
}
}
}
//尾插接口
void SeqListPushback(SL*ps,SQDataType x)
{
assert(ps);
SeqListCheckCapacity(ps);
ps->a[ps->size]=x;
ps->size++;
}
//头插函数
void SeqListpushfront(SL*ps,SQDataType x)
{
assert(ps);
SeqListCheckCapacity(ps);
int end=ps->size-1;
while(end>=0)
{
ps->a[end+1]=ps->a[end];
end--;
}
ps->a[0]=x;
ps->size++;
}
//头删函数
void SeqListPopfront(SL*ps)
{
assert(ps->size>0);
int start=1;
while(start<ps->size)
{
ps->a[start-1]=ps->a[start];
start++;
}
ps->size--;
}
//尾删函数
void SeqListPopback(SL*ps)
{
assert(ps->size>0);
ps->a[ps->size-1]={0};
ps->size--;
}
//随机插入
void SeqListInsert(SL*ps,int pos,SQDataType x)
{
assert(ps&&pos<ps->size);
SeqListCheckCapacity(ps);
int end=ps->size-1;
while(end>=pos)
{
ps->a[end+1]=ps->a[end];
end--;
}
ps->a[pos]=x;
ps->size++;
}
//随机删除
void SeQListErase(SL*ps,int pos)
{
assert(ps&&pos<ps->size-1&&ps->size>0);
int start=pos+1;
while(pos<ps->size)
{
ps->a[start]=ps->a[start+1];
start++;
}
ps->size--;
}
//查询接口
int SeQListFind(SL*ps,SQDataType x)
{
for(int i=0;i<ps->size;i++)
{
if(ps->a[i]==x)
{
return i;
}
}
}
//修改接口
void SeQListModify(SL*ps,int pos,SQDataType x)
{
assert(pos<ps->size);
ps->a[pos]=x;
}
//打印接口
void SeQListPrint(SL*ps)
{
for(int i=0;i<ps->sz;i++)
{
printf("%d ",ps->a[i]);
}
}
2.3.动态顺序表
void menu()
{
printf("***************************************\n");
printf("1.尾插数据, 2.头插数据\n");
printf("3.尾删数据, 4.头删数据\n");
printf("5.随机插入数据, 6.随机删除数据\n");
printf("7.打印数据, -1.退出\n");
printf("请输入你的操作选项\n");
printf("***************************************\n");
}
int main()
{
SL s;
SeqListInit(&s);
int option=0;
int x =0
while(option!=1)
{
menu();
scanf("%d",&option);
switch(option)
{
case 1:
printf("请输入你要插入的数据,以-1结束\n");
do{
scanf("%d",&x);
if(x!=1-)
{
SeqListPushback(SL*ps,SQDataType x);
}
}while(x!=-1);
break;
case 2:
printf("请输入你要插入的数据,以-1结束\n");
do{
scanf("%d",&x);
if(x!=1-)
{
SeqListpushfront(SL*ps,SQDataType x);
}
}while(x!=-1);
break;
case 3:
SeqListPopback(SL*ps);
break;
case 4:
SeqListPopfront(SL*ps);
break;
case 5:
printf("请输入你要插入的数据,以-1结束\n");
do{
scanf("%d",&x);
if(x!=1-)
{
int pos=0;
printf("请输入插入的位置\n");
scanf("%d",&pos);
SeqListInsert(SL*ps,int pos,SQDataType x);
}
}while(x!=-1); -
break;
case 6:
int pos=0;
printf("请输入删除的位置\n");
scanf("%d",&pos);
SeQListErase(SL*ps,int pos);
break;
case 7:
SeQListPrint(SL*ps);
break;
}
}
今日例题
题目1: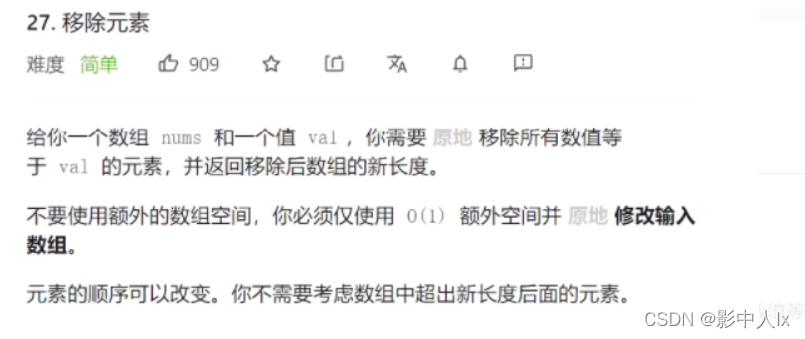
//双指针
int removeElement(int* nums,int numSize,int val)
{
int src=0;
int dst=0;
while(src<numSize&&dst<numSize)
{
if(num[src]!=val)
{
nums[dst++]=nums[src++];
}
else
{
src++;
}
}
return dst;
}
题目2: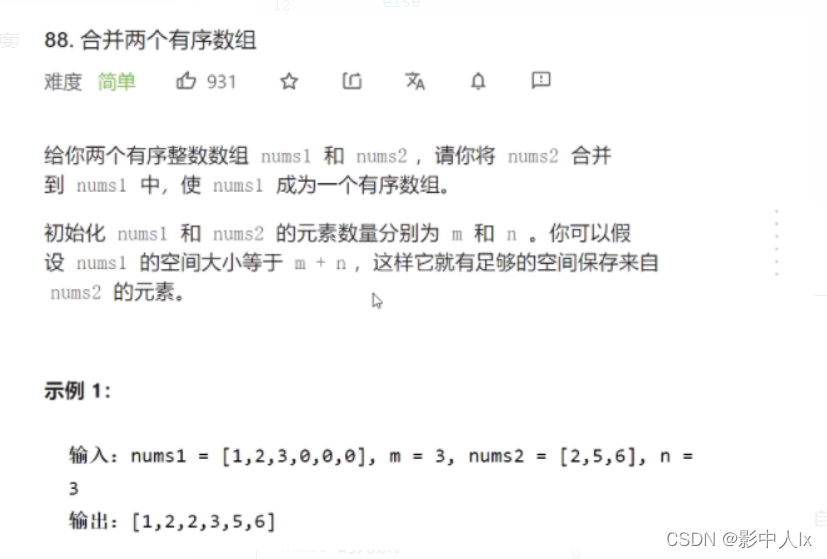
//归并,从后往前归并
//双指针
void memrge(int*nums1,int nums1Size,int m,int*nums2,int nums2Size,int n)
{
int end1=m-1;
int end2=n-1;
int end=m+n-1;
while(end1>=0&&end2>=0&&end>=0)
{
if(nums1[end1]>=nums2[end2])
{
nums1[end]=nums1[end1];
end1--;
end--;
}
else
{
nums1[end]=nums2[end2];
end2--;
end--
}
}
while(end>=0)
{
nums1[end]=nums[end2];
end--;
end2--
}
}