目录
一、常见关键字
1.1、关键字typeof
typedef
顾名思义是类型定义,这里应该理解为类型重命名。
typedef unsigned int uint;
typedef struct Node
{
int data;
struct Node* next;
}Node;
int main()
{
//unsigned int num = 0; (1)
uint num2 = 1;
//struct Node n; (1)
Node n2;
return 0;
}
1.2关键字static
在
C
语言中:
static
是用来修饰变量和函数的
1.
修饰局部变量
-
称为静态局部变量
2.
修饰全局变量
-
称为静态全局变量
3.
修饰函数
-
称为静态函数
1.2.1 修饰局部变量
1.2.2 修饰全局变量-称为静态全局变量
没有加static时
加了ststic
static修饰全局变量的时候这个全局变量的外部链接属性就变成了内部链接属性。
其他源文件(.c)就不能再使用到这个全局变量了。
1.2.3 修饰函数-称为静态函数
一个函数被static修饰,使得这个函数只能在本源文件内使用,不能在其他源文件内使用
1.3 register----寄存器
#include <stdio.h>
int main()
{
//寄存器变量
register int num = 3;//建议: 3存放在寄存中
return 0;
}
二、#define 定义常量和宏
2.1 #define 定义常量
2.2 #define 定义宏
三、指针
指针的基础示范
指针变量的大小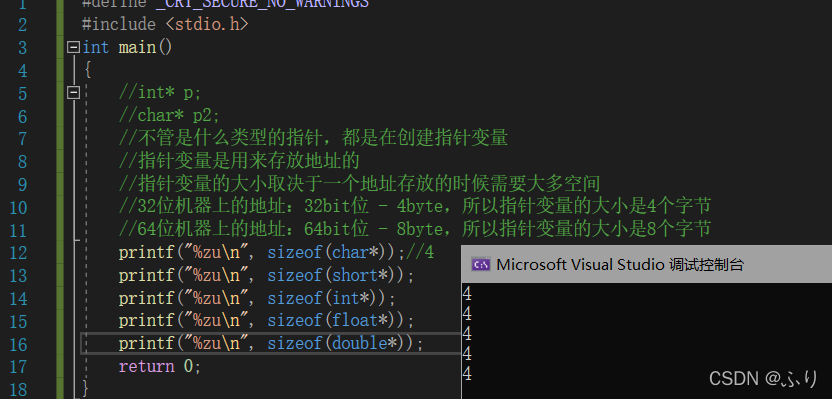
四、结构体
结构体就是把单一的类型组合在一起
C语言就给了自定义类型的能力自定义类型中有一种叫 : 结构体struct
4.1结构体书写基础方式
#include <stdio.h>
//学生
struct Stu
{
//结构体成员
char name[20];
int age;
char sex[10];
char tele[12];
};
int main()
{
struct Stu s = {"zhangsan", 20, "nan", "15596668862"};
//结构体对象.成员名
printf("%s %d %s %s\n", s.name, s.age, s.sex, s.tele);
return 0;
}
4.2结构体书写指针方式,函数输出
#include <stdio.h>
//学生
struct Stu
{
//结构体成员
char name[20];
int age;
char sex[10];
char tele[12];
};
void print(struct Stu* ps)//下方的自定义的print函数
{
printf("%s %d %s %s\n", (*ps).name, (*ps).age, (*ps).sex, (*ps).tele);//指针
//->
//结构体指针变量->成员名
printf("%s %d %s %s\n", ps->name, ps->age, ps->sex, ps->tele);
}
int main()
{
struct Stu s = {"zhangsan", 20, "nan", "15596668862"}; //结构体
print(&s);//函数
return 0;
}