实验内容
编写一个对输入的英文单词按照字典排序的程序,当在一个文本域输入若干个英文字单词,单击字典排序按钮,另一个文本域 对输入的英文单词进行排序;当按清空按钮,另一个文本区域的内容同时清空。要求通过Swing组件完成本程序的编写。
程序源码
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.StringTokenizer;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JTextArea;
public class MyFrame{
public static void main(String args[]) {
new MyFra();
}
}
class MyFra extends JFrame //继承JFrame 类,实现构建一个窗口的功能和特性
{
JButton buttonRight; //右边的按钮
JTextArea textRight; //右边的文本框
JButton buttonLeft; //左边的按钮
JTextArea textLeft; //左边的文本框
MyFra() //构造函数
{
buttonLeft = new JButton("字典排序");
buttonRight = new JButton("清空");
textLeft = new JTextArea();
textRight = new JTextArea();
this.setLayout(null); //设置为空布局,准确地定位组件在容器的位置和大小
this.add(textLeft);
this.setBounds(200, 200, 600, 400); //设置窗口在屏幕上的位置和大小
textLeft.setBounds(50, 20, 100, 200); //设置左边文本框的位置和大小
textLeft.setVisible(true); //窗口可见
this.add(textRight);
textRight.setBounds(200, 20, 100, 200); //设置右边文本框的位置和大小
textRight.setVisible(true);
textRight.setEditable(false); //右边文本框不可编辑
this.add(buttonLeft);
buttonLeft.setBounds(50, 250, 100, 20); //设置左边按钮的位置和大小
buttonLeft.setVisible(true);
buttonLeft.addActionListener(new ActionListener() //获得监视器
{
public void actionPerformed(ActionEvent e) //此方法对事件做出处理
{
sort(); //按左边按钮进行排序
}
});
this.add(buttonRight);
buttonRight.setBounds(200, 250, 100, 20); //设置右边按钮的位置和大小
buttonRight.setVisible(true);
buttonRight.addActionListener(new ActionListener() //获得监视器
{
public void actionPerformed(ActionEvent e)
{
clear(); //按右边按钮执行清空操作
}
});
setVisible(true);
validate(); //确保组件具有有效的布局
}
private void clear() //清空
{
textLeft.setText("");
textRight.setText("");
}
private void sort() //排序
{
String[] words = getWords(); //获取单词
dictionary(words); //调用dictionary函数将其排序
for (int i = 0; i < words.length; i++)
{
if (words[i].equals(null))
{
return;
}
System.out.println(words[i]);
textRight.append(words[i] + "\n"); //在右边文本框输出排好的单词
}
}
private void dictionary(String[] words)//冒泡法将数组中的字符串按字典序排序
{
for (int i = 0; i < words.length; i++)
{
if (words[i].equals(null))
{
return;
}
for (int j = i + 1; j < words.length; j++)
{
if (words[i].compareTo(words[j]) > 0)
{
String temp = words[i];
words[i] = words[j];
words[j] = temp;
}
}
}
}
private String[] getWords()
{
String left = textLeft.getText(); //输入到左边文本框
StringTokenizer token = new StringTokenizer(left, "\n");
//使用StringTokenizer类,其中分割标记为'/n'
int count = token.countTokens(); //得到计数变量的值
String[] words = new String[count];
int i = 0;
while (token.hasMoreTokens()) //控制循环,获取目标字符串
{
String current = token.nextToken();
if (current.matches("\\p{Alpha}+")) //使用正则表达式匹配字母
{
words[i] = current;
i++;
}
}
String[] result = new String[i];
for (int j = 0; j < i; j++)
{
result[j] = words[j];
}
return result; //将过滤掉的字符串数组返回
}
}
程序运行结果
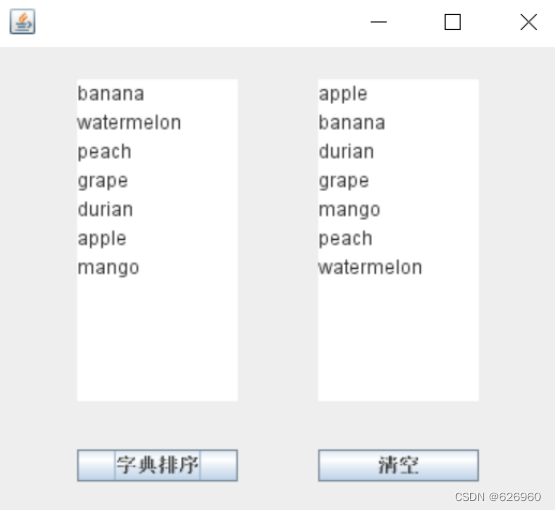
总结
MyFrame继承自JFrame类从而实现构建一个窗口的功能和特性。对于MyFrame 类,其包含的组建分别来自JButton和JTextArea两个类,从而在窗口中构建了一个可编辑的文本框和一个可输出结果的文本框,并完成两个可执行操作的按钮。布局采用 null 布局,所有组建均在构造函数中初始化并添加其位置和事件监听器。
通过 clear方法执行清空操作,通过sort方法调用dictionary和getWords两个方法来共同完成对左边文本框中内容的处理。其中getWords方法可以将左边文本框中的单词按行划分并过滤非纯字母字符串后放入一个字符串数组中返回,dictionary方法可将传入的字符串数组进行排序。
事件处理也是需要我们着重学习的内容,当我们操作键盘或鼠标时会发生事件,程序可以做出相应的响应,用户定义事件发生时程序应做出何种反映即可,比如该实验点击按钮后程序执行了清空或排序操作。
此次实验使我们了解了常用组件的属性和功能,并掌握了事件的处理机制,对我们以后设计图形用户界面有很大的帮助。