一.plsql基本语法,记录类型
plsql块的构成
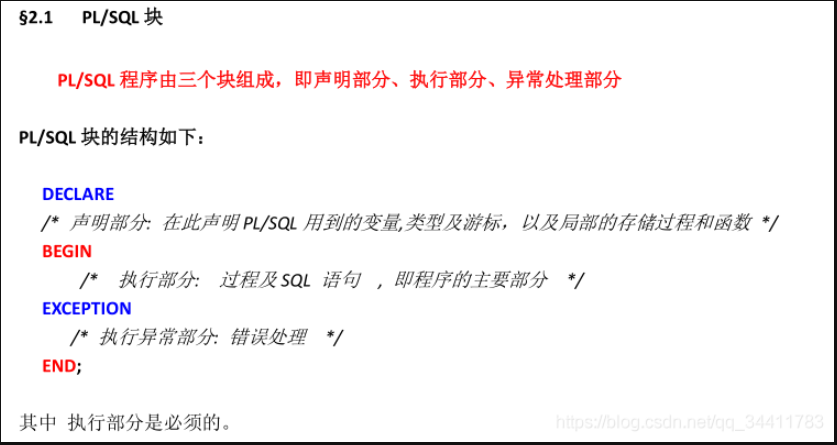
输出一个helloworld
首先要在sql中 set serveroutput on
begin
dbms_output.put_line(‘helloworld’);
end;
命名规则
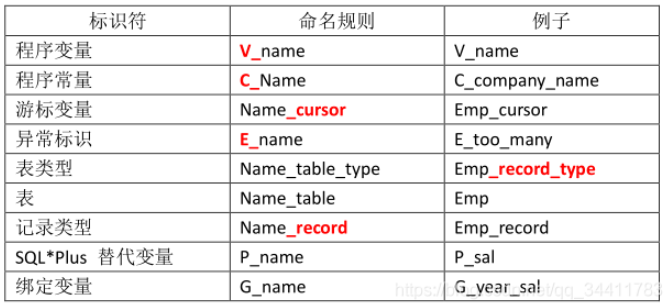
–查询100号员工的工资
declare
--声明变量
v_sal number(10,2);
v_email varchar2(20);
v_hire_date date;
begin
--sql语句的操作:select。。。into。。from。。where
select salary,email,hire_date into v_sal,v_email,v_hire_date
from employees where employee_id=100;
--打印
dbms_output.put_line(v_sal||’,’||v_email||’.’||v_hire_date);
end;
into前后要一一对应,声明的变量范围不能小
其中可以动态的获取表中的数据类型:v_sal employees.salary%type;
2. 记录类型
记录类型是把 逻辑相关 的数据作为一个单元存储起来 ,称作 PL/SQL RECORD 的域(FIELD) ,其作用是存放互不相同但逻辑相关的信息。
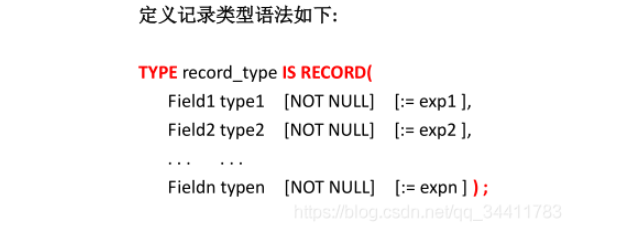
declare
–声明一个记录类型
type emp_record is record(
v_sal employees.salary%type ,
v_email employees.email%type ,
v_hire_date date
);
–定义一个记录类型的成员变量
v_emp_record emp_record ;
begin
–sql语句的操作:select。。。into。。from。。where
select salary,email,hire_date into v_emp_record
from employees where employee_id=100;
–打印
dbms_output.put_line(v_emp_record.v_sal||’,’||v_emp_record.v_email||’,’||v_emp_record.v_hire_date);
end;
plsql中赋值的表达式时 := v_salary number(10,2) := 0;
使用 %rowtype
这样就能把整个表的列定义了
declare
--声明一个记录类型的变量
v_emp_record employees%rowtype;
begin
--通过 select … into … 语句为变量赋值
select * into v_emp_record
from employees
where employee_id = 186;
– 打印变量的值
dbms_output.put_line(v_emp_record.last_name || ', ’ || v_emp_record.email || ', ’ ||
v_emp_record.salary || ', ’ || v_emp_record.job_id || ', ’ ||
v_emp_record.hire_date);
end;
通过变量实现DELETE、INSERT、UPDATE等操作
declare
v_emp_id employees.employee_id%type;
begin
v_emp_id := 109;
delete from employees
where employee_id = v_emp_id;
–commit;
end;
plsql的知识框架
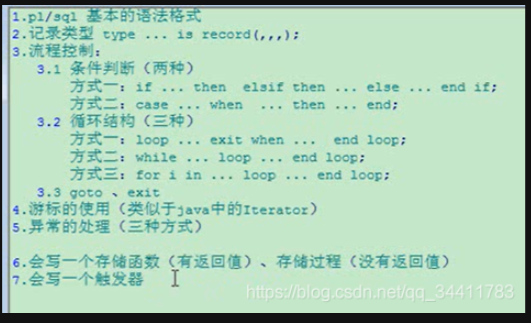
二.plsql流程控制
1.条件语句
1.1 if条件语句
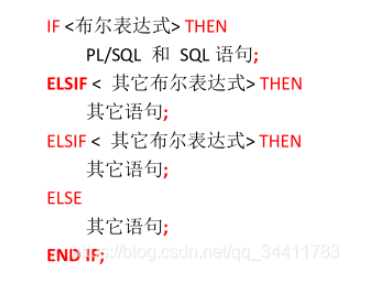
注意elsif不能写成elseif
语句后面有 ;
最后是end if;
then后面可以赋值
1.2 case表达式
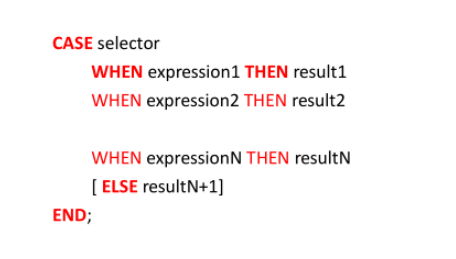
语句后面没有 ;
最后是end;
when后面不能写范围,只能写具体的
then后面不可以赋值不能使用dbms_output.put_line
–查询出 150号 员工的工资, 若其工资大于或等于 10000 则打印 ‘salary >= 10000’;
–若在 5000 到 10000 之间, 则打印 ‘5000<= salary < 10000’; 否则打印 ‘salary < 5000’
第一种方法
declare
v_salary employees.salary%type;
begin
select salary into v_salary
from employees
where employee_id=150;
if (v_salary>=10000) then dbms_output.put_line(‘salary >= 10000’);
elsif(v_salary>=5000 ) then dbms_output.put_line(‘5000<= salary < 10000’);
else dbms_output.put_line(‘salary < 5000’);
end if;
end;
第二种方法
declare
v_salary employees.salary%type;
v_msg varchar2(50);
begin
select salary into v_salary
from employees
where employee_id=150;
v_msg :=
case trunc(v_salary/5000) when 0 then ‘salary<5000’
when 1 then ‘5000<= salary < 10000’
else ‘salary>=10000’
end;
dbms_output.put_line(v_salary||’,’||v_msg);
end;
2.循环
2.1 简单循环loop
loop
要执行的语句 ;
exit when<条件语句>;
循环语句;
end loop;
2.2 while循环
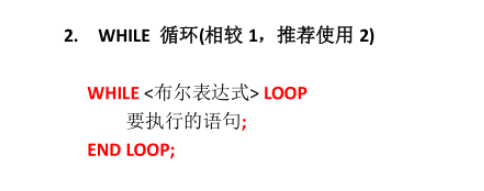
2.3 数字式循环
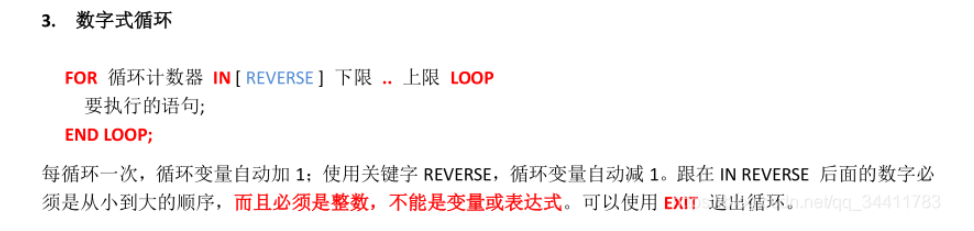
使用循环语句打印 1 - 100.(三种方式)
1).
LOOP … EXIT WHEN … END LOOP
declare
--初始化条件
v_i number(3) := 1;
begin
loop
--循环体
dbms_output.put_line(v_i);
--循环条件
exit when v_i = 100;
--迭代条件
v_i := v_i + 1;
end loop;
end;
-----------------
-----------------
-----------------
2).
WHILE … LOOP … END LOOP
declare
--初始化条件
v_i number(3) := 1;
begin
--循环条件
while v_i <= 100 loop
--循环体
dbms_output.put_line(v_i);
--迭代条件
v_i := v_i + 1;
end loop;
end;
-----------------
-----------------
-----------------
3).
begin
for i in 1 ..100 loop
dbms_output.put_line(i);
end loop;
end;
3.循环和判断结合的例子
综合使用 if, while 语句, 打印 1 - 100 之间的所有素数
(素数: 有且仅用两个正约数的整数, 2, 3, 5, 7, 11, 13, …).
declare
v_flag number(1):=1;
v_i number(3):=2;
v_j number(2):=2;
begin
while (v_i<=100) loop
while v_j <= sqrt(v_i) loop
if (mod(v_i,v_j)=0) then v_flag:= 0;
end if;
v_j :=v_j +1;
end loop;
if(v_flag=1) then dbms_output.put_line(v_i);
end if;
v_flag :=1;
v_j := 2;
v_i :=v_i +1;
end loop;
end;
(法二)使用for循环实现1-100之间的素数的输出 其中还加入了goto
declare
--标记值, 若为 1 则是素数, 否则不是
v_flag number(1) := 0;
begin
for i in 2 .. 100 loop
v_flag := 1;
for j in 2 .. sqrt(i) loop
if i mod j = 0 then
v_flag := 0;
goto label;
end if;
end loop;
<<label>>
if v_flag = 1 then
dbms_output.put_line(i);
end if;
end loop;
end;