import javax.swing.*;
import javax.swing.plaf.FontUIResource;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.Enumeration;
/**
* 继承窗体类,实现动作监听接口
*/
public class Calculator extends JFrame implements ActionListener {
static Calculator frm = new Calculator();
// 使用JPanel容器,放置组件及其面板
static JPanel pan1 = new JPanel();
static JPanel pan2 = new JPanel();
// 文本框组件
static JTextField tf = new JTextField();
// 按钮组件
static JButton[] b = new JButton[10];
static JButton bp, ba, bs, bm, bd, be, bc, br;
// 文本框内容
String expression = "";
public static void main(String[] args) {
// 设置界面字体样式、大小
InitGlobalFont(new Font("微软雅黑", Font.PLAIN, 24));
for (int i = 0; i <= 9; i++) {
b[i] = new JButton("" + i);
}
bp = new JButton(".");
ba = new JButton("+");
bs = new JButton("-");
bm = new JButton("*");
bd = new JButton("/");
be = new JButton("=");
bc = new JButton("C");
br = new JButton("Backspace");
// 设置窗体的标题
frm.setTitle("简易计算器");
// 设置用户界面上的屏幕组件的格式布局,默认为流式布局
frm.setLayout(null);
// 设置组件大小
frm.setSize(500, 450);
// 此窗体不可随意调整大小
frm.setResizable(false);
// 采用指定的行数、列数将容器分割成多个网格
GridLayout grid1 = new GridLayout(4, 4);
GridLayout grid2 = new GridLayout(1, 2);
pan1.setLayout(grid1);
pan2.setLayout(grid2);
// 设置JFrame组件中的x、y坐标,组件宽度、高度
tf.setBounds(45, 30, 400, 50);
pan1.setBounds(45, 105, 400, 200);
pan2.setBounds(45, 305, 400, 60);
// 设置文本框背景
tf.setBackground(Color.white);
// 设置文本框字体、样式、大小
tf.setFont(new Font("微软雅黑",Font.PLAIN,24));
// 设置文本框内容对齐方式
tf.setHorizontalAlignment(JTextField.RIGHT);
pan1.add(b[7]);
pan1.add(b[8]);
pan1.add(b[9]);
pan1.add(bd);
pan1.add(b[4]);
pan1.add(b[5]);
pan1.add(b[6]);
pan1.add(bm);
pan1.add(b[1]);
pan1.add(b[2]);
pan1.add(b[3]);
pan1.add(bs);
pan1.add(bp);
pan1.add(b[0]);
pan1.add(be);
pan1.add(ba);
pan2.add(bc);
pan2.add(br);
frm.add(tf);
frm.add(pan1);
frm.add(pan2);
// 添加动作侦听器,接收发自此按钮的动作事件
for (int i = 0; i <= 9; i++) {
b[i].addActionListener(frm);
}
bd.addActionListener(frm);
bm.addActionListener(frm);
bs.addActionListener(frm);
ba.addActionListener(frm);
bc.addActionListener(frm);
br.addActionListener(frm);
bp.addActionListener(frm);
be.addActionListener(frm);
// 设置用户在此窗体上发起 "close" 时,使用 System exit方法退出应用程序
frm.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// 数据模型已经构造好了,允许JVM可以根据数据模型执行paint方法开始画图并显示到屏幕上了
frm.setVisible(true);
}
// 字体设置
private static void InitGlobalFont(Font font) {
FontUIResource fontRes = new FontUIResource(font);
for (Enumeration keys = UIManager.getDefaults().keys(); keys.hasMoreElements(); ) {
Object key = keys.nextElement();
Object value = UIManager.get(key);
if (value instanceof FontUIResource) {
UIManager.put(key, fontRes);
}
}
}
// 重写接口ActionListener里面定义的一个抽象方法
@Override
public void actionPerformed(ActionEvent e) {
if ("=".equals(e.getActionCommand())) {
expression = Calculate.count(expression);
// 获取文本框文本内容
tf.setText(expression);
} else if ("C".equals(e.getActionCommand())) {
expression = "";
tf.setText("0");
} else if ("Backspace".equals(e.getActionCommand())) {
expression = expression.substring(0, expression.length() - 1);
tf.setText(expression);
} else {
expression += e.getActionCommand();
tf.setText(expression);
}
}
}
import java.util.Stack;
public class Calculate {
public static Stack<String> num = new Stack<String>();
public static Stack<String> operator = new Stack<String>();
// 计算
public static void work() {
String s1 = num.pop();
String s2 = num.pop();
// 初始化字符串
float num1 = Float.parseFloat(s1);
float num2 = Float.parseFloat(s2);
String op = operator.pop();
float num3;
switch (op) {
case "+":
num3 = num1 + num2;
num.push(num3 + "");
break;
case "-":
num3 = num1 - num2;
num.push(num3 + "");
break;
case "*":
num3 = num1 * num2;
num.push(num3 + "");
break;
case "/":
num3 = num1 / num2;
num.push(num3 + "");
break;
}
}
// 从文本框中返回输入的字符串
public static String count(String s) {
int i = 0;
while (i < s.length()) {
// 从字符串中获取字符
switch (s.charAt(i)) {
case '+':
case '-':
while (!operator.empty()) {
work();
}
operator.push(s.charAt(i) + "");
i++;
break;
case '*':
case '/':
while (!operator.empty() && (operator.peek().equals("*") || operator.peek().equals("/"))) {
work();
System.out.println("Ok");
}
operator.push(s.charAt(i) + "");
i++;
break;
default:
String number = "";
for (; i < s.length() && (s.charAt(i) >= '0' && s.charAt(i) <= '9' || s.charAt(i) == '.'); i++) {
number = number + s.charAt(i);
}
num.push(number);
}
}
while (!operator.empty()) {
work();
}
return num.peek();
}
}
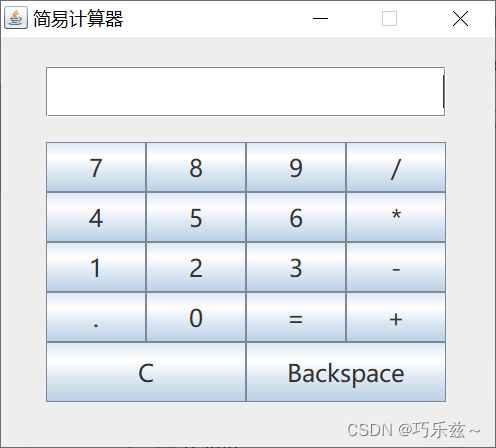