1. 使用传统JDBC方法与Model 1模型通过JSP页面访问数据库。
【步骤1】在MySQL数据库test中创建books表,其结构如下:
创建数据库MySQL代码与插入数据代码
drop database if exists web_test;
create database web_test character set utf8mb4;
use web_test;
create table books(
book_id varchar(10) not null,
title varchar(50),
author varchar(20),
publisher varchar(40),
price float
);
insert into books values ('204', 'Head First Servlets & JSP', 'Bryan Basham', '中国电力出版社', 98);
insert into books values ('201', 'Servlets与JSP核心教程', 'Hall Marty', '清华大学出版社', 45);
insert into books values ('202', 'Tomcat与Java Web 开发技术详解', '孙卫琴', '机械工业出版社', 45);
insert into books values ('203', 'JSP 应用开发技术', '柳永坡', '人民邮电出版社', 52);
insert into books values ('205', 'J2EE 1.4 编程指南', 'Spielman Sue', '电子工业出版社', 68);
【步骤2】创建模型:编写名称为BookBean.java的JavaBean用于封装图书的信息;编写BookDAO.java,采用传统JDBC方法创建数据库连接,对图书信息进行查询。
DBUtil的代码
package org.example.utils;
import com.mysql.jdbc.jdbc2.optional.MysqlDataSource;
import javax.sql.DataSource;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
public class DBUtil {
private static volatile DataSource DS;
private static DataSource getDataSource() {
if (DS == null) {
synchronized (DBUtil.class) {
if (DS == null) {
MysqlDataSource dataSource = new MysqlDataSource();
dataSource.setURL("jdbc:mysql://localhost:3306/web_test");
dataSource.setUser("root");//账号
dataSource.setPassword("142516");
dataSource.setUseSSL(false);//不设置不会出现问题,但会出现红色警告
//数据库的编码格式,可以设置为UTF-8,或是utf8
dataSource.setCharacterEncoding("utf8");
DS = dataSource;
}
}
}
return DS;
}
//获取数据库连接
public static Connection getConnection() {
try {
return getDataSource().getConnection();
} catch (SQLException e) {
throw new RuntimeException("获取数据库连接异常", e);
}
}
//释放连接
public static void close(java.sql.Connection c, Statement s, ResultSet rs) {
try {
if(rs != null) rs.close();
if(s != null) s.close();
if(c != null) c.close();
} catch (SQLException e) {
throw new RuntimeException("jdbc释放资源出错", e);
}
}
}
上面这部分需要改一下
BookBean代码
package org.example.beans;
public class BookBean {
private String bookId;
private String title;
private String author;
private String publisher;
private double price;
public String getBookId() {
return bookId;
}
public void setBookId(String bookId) {
this.bookId = bookId;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public String getPublisher() {
return publisher;
}
public void setPublisher(String publisher) {
this.publisher = publisher;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
}
BookDao代码
package org.example.dao;
import org.example.beans.BookBean;
import org.example.utils.DBUtil;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.util.ArrayList;
import java.util.List;
public class BookDao {
public static List<BookBean> queryBooks() {
Connection c = null;
PreparedStatement ps = null;
ResultSet rs = null;
try {
c = DBUtil.getConnection();
String sql = "select * from books";
ps = c.prepareStatement(sql);
rs = ps.executeQuery();
List<BookBean> books = new ArrayList<>();
while (rs.next()) {
BookBean b = new BookBean();
b.setBookId(rs.getString("book_id"));
b.setAuthor(rs.getString("author"));
b.setTitle(rs.getString("title"));
b.setPublisher(rs.getString("publisher"));
b.setPrice(rs.getDouble("price"));
books.add(b);
}
return books;
} catch (Exception e) {
throw new RuntimeException("查询books出错", e);
} finally {
DBUtil.close(c, ps, rs);
}
}
}
【步骤3】创建兼具视图与控制器功能的JSP页面:编写displayBooks.jsp页面,使用<jsp:useBean>动作调用BookDAO查询所有图书的信息,以表格的形式显示所有图书的书号、书名、作者、价格和出版社名称。
displayBooks.jsp
<%@ page import="java.util.*" %>
<%@ page import="org.example.beans.*" %>
<%@ page contentType="text/html;charset=utf-8" %>
<jsp:useBean id="book" class="org.example.dao.BookDao" scope="session"/>
<html>
<head>
<title>displayBooks</title>
<style>
td{
text-align: center;
}
</style>
</head>
<body>
<table width="500" height="256" border="1" align="center">
<tr>
<th scope="col">bookid</th>
<th scope="col">title</th>
<th scope="col">author</th>
<th scope="col">publisher</th>
<th scope="col">price</th>
</tr>
<%
List<BookBean> books = book.queryBooks();
int len = books.size();
for (int i = 0; i < len; i++) {
String book_id = books.get(i).getBookId();
String title = books.get(i).getTitle();
String author = books.get(i).getAuthor();
String publisher = books.get(i).getPublisher();
double price = books.get(i).getPrice();
%>
<tr>
<td><%=book_id%> </td>
<td><%=title%></td>
<td><%=author%></td>
<td><%=publisher%></td>
<td><%=price%></td>
</tr>
<% } %>
</table>
</body>
</html>
2. 基于实验内容1创建的数据库表和模型,采用MVC设计模式,通过数据源和DAO对象访问数据库。其中JavaBeans实现模型与数据库访问与操作,Servlet实现控制器,JSP页面实现视图。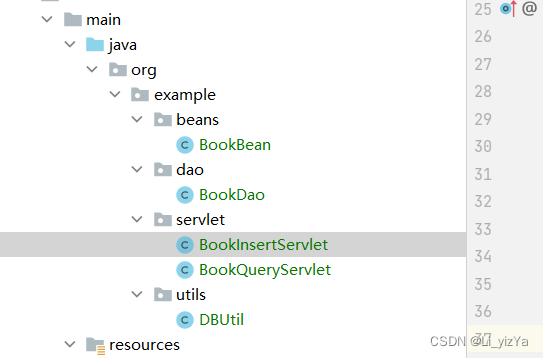
- 模型包括2个JavaBean:BookBean用于存放图书信息,BookDAO用于访问数据库。
BookBean.java(BookBean与上面的BookBean一样)
package org.example.beans;
import java.io.Serializable;
/**
* Created with IntelliJ IDEA.
* Description:
* User: Li_yizYa
* Date: 2023—04—24
* Time: 17:57
*/
//@SuppressWarnings("serial")
public class BookBean {
private String bookId;
private String title;
private String author;
private String publisher;
private double price;
public String getBookId() {
return bookId;
}
public void setBookId(String bookId) {
this.bookId = bookId;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public String getPublisher() {
return publisher;
}
public void setPublisher(String publisher) {
this.publisher = publisher;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
}
BookDao.java(BookDao在上面的java文件中额外加了几个方法)
package org.example.dao;
import org.example.beans.BookBean;
import org.example.utils.DBUtil;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.util.ArrayList;
import java.util.List;
/**
* Created with IntelliJ IDEA.
* Description:
* User: Li_yizYa
* Date: 2023—04—24
* Time: 18:13
*/
public class BookDao {
public static List<BookBean> queryBooks() {
Connection c = null;
PreparedStatement ps = null;
ResultSet rs = null;
try {
c = DBUtil.getConnection();
String sql = "select * from books";
ps = c.prepareStatement(sql);
rs = ps.executeQuery();
List<BookBean> books = new ArrayList<>();
while (rs.next()) {
BookBean b = new BookBean();
b.setBookId(rs.getString("book_id"));
b.setAuthor(rs.getString("author"));
b.setTitle(rs.getString("title"));
b.setPublisher(rs.getString("publisher"));
b.setPrice(rs.getDouble("price"));
books.add(b);
}
return books;
} catch (Exception e) {
throw new RuntimeException("查询books出错", e);
} finally {
DBUtil.close(c, ps, rs);
}
}
//根据序号查询
public static BookBean queryById(String id) {
Connection c = null;
PreparedStatement ps = null;
ResultSet rs = null;
BookBean b = null;
try {
c = DBUtil.getConnection();
String sql = "select * from books where book_id = ?";
ps = c.prepareStatement(sql);
ps.setString(1, id);
rs = ps.executeQuery();
while (rs.next()) {
b = new BookBean();
b.setBookId(rs.getString("book_id"));
b.setAuthor(rs.getString("author"));
b.setTitle(rs.getString("title"));
b.setPublisher(rs.getString("publisher"));
b.setPrice(rs.getDouble("price"));
}
return b;
} catch (Exception e) {
throw new RuntimeException("查询books出错", e);
} finally {
DBUtil.close(c, ps, rs);
}
}
//根据书名查询
public static BookBean queryByTitle(String title) {
Connection c = null;
PreparedStatement ps = null;
ResultSet rs = null;
BookBean b = null;
try {
c = DBUtil.getConnection();
String sql = "select * from books where title = ?";
ps = c.prepareStatement(sql);
ps.setString(1, title);
rs = ps.executeQuery();
while (rs.next()) {
b = new BookBean();
b.setBookId(rs.getString("book_id"));
b.setAuthor(rs.getString("author"));
b.setTitle(rs.getString("title"));
b.setPublisher(rs.getString("publisher"));
b.setPrice(rs.getDouble("price"));
}
return b;
} catch (Exception e) {
throw new RuntimeException("查询books出错", e);
} finally {
DBUtil.close(c, ps, rs);
}
}
//根据作者查询
public static BookBean queryByAuthor(String author) {
Connection c = null;
PreparedStatement ps = null;
ResultSet rs = null;
BookBean b = null;
try {
c = DBUtil.getConnection();
String sql = "select * from books where author = ?";
ps = c.prepareStatement(sql);
ps.setString(1, author);
rs = ps.executeQuery();
while (rs.next()) {
b = new BookBean();
b.setBookId(rs.getString("book_id"));
b.setAuthor(rs.getString("author"));
b.setTitle(rs.getString("title"));
b.setPublisher(rs.getString("publisher"));
b.setPrice(rs.getDouble("price"));
}
return b;
} catch (Exception e) {
throw new RuntimeException("查询books出错", e);
} finally {
DBUtil.close(c, ps, rs);
}
}
//插入数据
public static int insert(BookBean book) {
Connection c = null;
PreparedStatement ps = null;
try {
c = DBUtil.getConnection();
String sql = "insert into books values (?, ?, ?, ?, ?);";
ps = c.prepareStatement(sql);
ps.setString(1, book.getBookId());
ps.setString(2, book.getTitle());
ps.setString(3, book.getAuthor());
ps.setString(4, book.getPublisher());
ps.setDouble(5, book.getPrice());
return ps.executeUpdate();
} catch (Exception e) {
throw new RuntimeException("插入books出错", e);
} finally {
DBUtil.close(c, ps, null);
}
}
}
- 控制器包括2个Servlet:BookQueryServlet根据请求参数查询图书信息、BookInsertServlet用来向数据库中插入一条图书信息。
BookQueryServlet.java
package org.example.servlet;
import org.example.beans.BookBean;
import org.example.dao.BookDao;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
/**
* Created with IntelliJ IDEA.
* Description:
* User: Li_yizYa
* Date: 2023—04—24
* Time: 20:12
*/
@WebServlet("/query")
public class BookQueryServlet extends HttpServlet {
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
resp.setCharacterEncoding("utf-8");
String selection = req.getParameter("selection");
String content = req.getParameter("content");
BookBean book = null;
if (selection.equals("book_id")) {
book = BookDao.queryById(content);
} else if (selection.equals("title")) {
book = BookDao.queryByTitle(content);
} else {
book = BookDao.queryByAuthor(content);
}
if(book!=null){
req.getSession().setAttribute("book", book);
RequestDispatcher view = req.getRequestDispatcher("display.jsp");
view.forward(req, resp);
}else{
RequestDispatcher view = req.getRequestDispatcher("errorPage.jsp");
view.forward(req, resp);
}
}
}
BookInsertServlet.java
package org.example.servlet;
import org.example.beans.BookBean;
import org.example.dao.BookDao;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.Date;
/**
* Created with IntelliJ IDEA.
* Description:
* User: Li_yizYa
* Date: 2023—04—24
* Time: 20:12
*/
@WebServlet("/insert")
public class BookInsertServlet extends HttpServlet {
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
req.setCharacterEncoding("utf-8");
resp.setContentType("text/html;charset=utf-8");
BookBean book = new BookBean();
book.setBookId(req.getParameter("bookId"));
book.setTitle(req.getParameter("title"));
book.setAuthor(req.getParameter("author"));
book.setPublisher(req.getParameter("publisher"));
book.setPrice(Double.parseDouble(req.getParameter("price")));
System.out.println(book.getTitle());
int ret = BookDao.insert(book);
String respContent = "插入失败";
if (ret == 1) {
respContent = "插入成功";
}
PrintWriter out = resp.getWriter();
out.println("<HTML>");
out.println(" <BODY><center>");
out.println("<h2>"+respContent + "</h2>");
out.println(" </center> </BODY>");
out.println("</HTML>");
}
}
- 视图包括4个JSP页面:bookQuery.jsp显示图书查询表单的页面、bookInsert.jsp显示收集图书信息表单的页面、display.jsp显示查询结果页面、errorPage.jsp显示查询错误页面。
bookQuery.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<head>
<title>bookQuery</title>
</head>
<body>
<form action="query" method="post">
请做选择:<select name="selection">
<option value="book_id">序号</option>
<option value="title">书名</option>
<option value="author">作者</option>
</select>
<br>
查询内容:<input type="text" name="content">
<input type="submit" value="查询">
</form>
</body>
</html>
bookInsert.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<jsp:useBean id="book" class="org.example.beans.BookBean" scope="session"/>
<html>
<head>
<title>bookInsert</title>
</head>
<body>
<h3>请输入图书信息:</h3>
<form action="insert" method="post">
<table>
<tr><td>书号</td> <td><input type="text" name="bookId" ></td></tr>
<tr><td>书名</td><td><input type="text" name="title"></td></tr>
<tr><td>作者</td><td><input type="text" name="author" ></td></tr>
<tr><td>出版社</td><td><input type="text" name="publisher" ></td></tr>
<tr><td>单价</td><td><input type="text" name="price" ></td></tr>
<tr><td><input type="submit" value="确定" ></td>
<td><input type="reset" value="重置" ></td>
</tr>
</table>
</form>
</body>
</html>
display.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<jsp:useBean id="book" class="org.example.beans.BookBean" scope="session"/>
<jsp:setProperty name="book" property="*"/>
<html>
<head>
<title>display</title>
</head>
<body>
书号:<jsp:getProperty name="book" property="bookId"/><br><br>
书名:<jsp:getProperty name="book" property="title"/><br><br>
作者:<jsp:getProperty name="book" property="author"/><br><br>
出版社:<jsp:getProperty name="book" property="publisher"/><br><br>
价格:<jsp:getProperty name="book" property="price"/><br><br>
</body>
</html>
errorPage.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>errorPage</title>
</head>
<body>
Sorry,未查询到您需要的图书!
</body>
</html>
3. DAO设计模式练习。
【步骤1】:使用root用户登录MySQL的test数据库,创建customer表,包括custName、email、phone等字段,设计CustomerBean传输对象,使其实现java.io.Serializable接口。
创建数据库代码
create table customer(
custName varchar(20),
email varchar(40),
phone varchar(20)
);
CustomerBean.java
package org.example.beans;
import java.io.Serializable;
/**
* Created with IntelliJ IDEA.
* Description:
* User: Li_yizYa
* Date: 2023—04—24
* Time: 21:49
*/
@SuppressWarnings("serial")
public class CustomerBean implements Serializable {
private String custName;
private String email;
private String phone;
public String getCustName() {
return custName;
}
public void setCustName(String custName) {
this.custName = custName;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
}
【步骤2】:创建CustomerDAO类实现数据库访问逻辑,通过该类可以获得一个连接对象,对数据库进行查询、插入、修改和删除操作,最后关闭指定的对象。
package org.example.dao;
import org.example.beans.BookBean;
import org.example.beans.CustomerBean;
import org.example.utils.DBUtil;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
/**
* Created with IntelliJ IDEA.
* Description:
* User: Li_yizYa
* Date: 2023—04—24
* Time: 21:57
*/
public class CustomerDAO {
public static CustomerBean queryByName(String name) {
Connection c = null;
PreparedStatement ps = null;
ResultSet rs = null;
try {
System.out.println(name);
c = DBUtil.getConnection();
String sql = "select * from customer where custName = ?";
ps = c.prepareStatement(sql);
ps.setString(1, name);
rs = ps.executeQuery();
CustomerBean customer = null;
while (rs.next()) {
customer = new CustomerBean();
customer.setCustName(rs.getString("custName"));
customer.setEmail(rs.getString("email"));
customer.setPhone(rs.getString("phone"));
}
return customer;
} catch (Exception e) {
throw new RuntimeException("查询customer出错", e);
} finally {
DBUtil.close(c, ps, rs);
}
}
//查询数据
public static List<CustomerBean> queryCustomer() {
Connection c = null;
PreparedStatement ps = null;
ResultSet rs = null;
try {
c = DBUtil.getConnection();
String sql = "select * from customer";
ps = c.prepareStatement(sql);
rs = ps.executeQuery();
List<CustomerBean> customers = new ArrayList<>();
while (rs.next()) {
CustomerBean customer = new CustomerBean();
customer.setCustName(rs.getString("custName"));
customer.setEmail(rs.getString("email"));
customer.setPhone(rs.getString("phone"));
customers.add(customer);
}
return customers;
} catch (Exception e) {
throw new RuntimeException("查询customer出错", e);
} finally {
DBUtil.close(c, ps, rs);
}
}
//插入数据
public static int insert(CustomerBean customer) {
Connection c = null;
PreparedStatement ps = null;
try {
c = DBUtil.getConnection();
String sql = "insert into customer values (?, ?, ?);";
ps = c.prepareStatement(sql);
ps.setString(1, customer.getCustName());
ps.setString(2, customer.getEmail());
ps.setString(3, customer.getPhone());
return ps.executeUpdate();
} catch (Exception e) {
throw new RuntimeException("插入books出错", e);
} finally {
DBUtil.close(c, ps, null);
}
}
//根据name删除数据
public static int delete(String name) {
Connection c = null;
PreparedStatement ps = null;
try {
c = DBUtil.getConnection();
String sql = "delete from customer where custName=?";
ps = c.prepareStatement(sql);
ps.setString(1, name);
return ps.executeUpdate();
} catch (SQLException e) {
throw new RuntimeException("删除数据库图片出错", e);
} finally {
DBUtil.close(c, ps, null);
}
}
//修改数据
public static int update(CustomerBean customer) {
Connection c = null;
PreparedStatement ps = null;
try {
c = DBUtil.getConnection();
String sql = "update customer set custName=?,email=?,phone=? where custName = ?";
ps = c.prepareStatement(sql);
ps.setString(1, customer.getCustName());
ps.setString(2, customer.getEmail());
ps.setString(3, customer.getPhone());
ps.setString(4, customer.getCustName());
return ps.executeUpdate();
} catch (SQLException e) {
throw new RuntimeException("修改数据异常", e);
} finally {
DBUtil.close(c, ps, null);
}
}
}
【步骤3】:创建insertCustomer.jsp页面,通过一个表单录入向数据库中插入的数据。
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>insertCustomer</title>
</head>
<body>
<h3>请输入顾客信息:</h3>
<form action = "insertCustomer" method = "post">
<table>
<tr><td>姓名</td> <td><input type="text" name="custName" ></td></tr>
<tr><td>邮箱</td><td><input type="text" name="email"></td></tr>
<tr><td>电话</td><td><input type="text" name="phone" ></td></tr>
<tr><td><input type="submit" value="确定" ></td>
<td><input type="reset" value="重置" ></td>
</tr>
</table>
</form>
</body>
</html>
【步骤4】:创建InsertCustomerServlet.java控制器,调用CustomerDAO对象和传输对象,将数据插入到数据库中。
package org.example.servlet;
import org.example.beans.BookBean;
import org.example.beans.CustomerBean;
import org.example.dao.BookDao;
import org.example.dao.CustomerDAO;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
/**
* Created with IntelliJ IDEA.
* Description:
* User: Li_yizYa
* Date: 2023—04—24
* Time: 21:56
*/
@WebServlet("/insertCustomer")
public class InsertCustomerServlet extends HttpServlet {
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
req.setCharacterEncoding("utf-8");
resp.setContentType("text/html;charset=utf-8");
CustomerBean customer = new CustomerBean();
customer.setCustName(req.getParameter("custName"));
customer.setEmail(req.getParameter("email"));
customer.setPhone(req.getParameter("phone"));
int ret = CustomerDAO.insert(customer);
String respContent = "插入失败";
if (ret == 1) {
respContent = "插入成功";
}
PrintWriter out = resp.getWriter();
out.println("<HTML>");
out.println(" <BODY><center>");
out.println("<h2>"+respContent + "</h2>");
out.println("<a href=\"insertCustomer.jsp\">返回添加页面</a>");
out.println("<a href=\"showCustmer.jsp\">返回显示页面</a>");
out.println(" </center> </BODY>");
out.println("</HTML>");
}
}
【步骤5】:创建showCustmer.jsp,显示所有客户的信息,每一条客户信息后面增加修改和删除超链接,编写ActionServlet.java控制器,调用DAO和传输对象实现对客户信息的删除和修改功能。
showCustmer.jsp
<%@ page import="java.util.*" %>
<%@ page import="org.example.beans.*" %>
<%@ page contentType="text/html;charset=utf-8" %>
<jsp:useBean id="customer" class="org.example.dao.CustomerDAO" scope="session"/>
<html>
<head>
<title>showCustmer</title>
<style>
td{
text-align: center;
}
</style>
</head>
<body>
<table width="500" height="256" border="1">
<tr>
<th scope="col">客户姓名</th>
<th scope="col">邮箱</th>
<th scope="col">电话号</th>
<th scope="col">操作</th>
</tr>
<%
List<CustomerBean> customers = customer.queryCustomer();
int len = customers.size();
for (int i = 0; i < len; i++) {
String custName = customers.get(i).getCustName();
String email = customers.get(i).getEmail();
String phone = customers.get(i).getPhone();
%>
<tr>
<td><%=custName%> </td>
<td><%=email%></td>
<td><%=phone%></td>
<td>
<a href="update.jsp?name=<%= custName %>">修改</a>
<a href="delete1?name=<%= custName %>">删除</a>
</td>
</tr>
<% } %>
</table>
</body>
</html>
ActionServlet.java
package org.example.servlet;
import org.example.beans.CustomerBean;
import org.example.dao.CustomerDAO;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
/**
* Created with IntelliJ IDEA.
* Description:
* User: Li_yizYa
* Date: 2023—04—24
* Time: 22:21
*/
@WebServlet({"/delete1", "/update1"})
public class ActionServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
String uri = req.getRequestURI();
if (uri.endsWith("/delete1")) {
delete1(req, resp);
}
if (uri.endsWith("/update1")) {
update1(req, resp);
}
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req, resp);
}
private void delete1(HttpServletRequest req, HttpServletResponse resp) throws IOException {
String name = req.getParameter("name");
int ret = CustomerDAO.delete(name);
resp.sendRedirect("showCustmer.jsp");
}
private void update1(HttpServletRequest req, HttpServletResponse resp) throws IOException {
req.setCharacterEncoding("utf-8");
resp.setContentType("text/html;charset=utf-8");
CustomerBean customer = new CustomerBean();
customer.setCustName(req.getParameter("custName"));
customer.setEmail(req.getParameter("email"));
customer.setPhone(req.getParameter("phone"));
System.out.println(customer.getCustName());
int ret = CustomerDAO.update(customer);
String respContent = "修改失败";
if (ret == 1) {
respContent = "修改成功";
}
PrintWriter out = resp.getWriter();
out.println("<HTML>");
out.println(" <BODY><center>");
out.println("<h2>"+respContent + "</h2>");
out.println("<a href=\"showCustmer.jsp\">返回显示页面</a>");
out.println(" </center> </BODY>");
out.println("</HTML>");
}
}