基本操作 原题
#include<iostream>
using namespace std;
class ABC {
public:
// ERROR **********found**********
ABC() {a=0; b=0; c=0;}
ABC(int aa, int bb, int cc);
void Setab() {++a,++b;}
int Sum() {return a+b+c;}
private:
int a,b;
const int c;
};
ABC::ABC(int aa, int bb, int cc):c(cc) {a=aa; b=bb;}
int main()
{
ABC x(1,2,3), y(4,5,6);
ABC z,*w=&z;
w->Setab();
// ERROR **********found**********
int s1=x.Sum()+y->Sum();
cout<<s1<<' ';
// ERROR **********found**********
int s2=s1+w.Sum();
cout<<s2<<endl;
return 0;
}

基本操作 答案
#include<iostream>
using namespace std;
class ABC {
public:
// ERROR **********found**********
ABC():c(0) {a=0; b=0;}
ABC(int aa, int bb, int cc);
void Setab() {++a,++b;}
int Sum() {return a+b+c;}
private:
int a,b;
const int c;
};
ABC::ABC(int aa, int bb, int cc):c(cc) {a=aa; b=bb;}
int main()
{
ABC x(1,2,3), y(4,5,6);
ABC z,*w=&z;
w->Setab();
// ERROR **********found**********
int s1=x.Sum()+y.Sum();
cout<<s1<<' ';
// ERROR **********found**********
int s2=s1+w->Sum();
cout<<s2<<endl;
return 0;
}
简单应用 原题
#include <iostream>
using namespace std;
class Entry { //链接栈的结点
public:
Entry* next; // 指向下一个结点的指针
int data; // 结点数据
//**********found**********
Entry(Entry* n, int d) : _______________________, data(d) { }
};
class Stack {
Entry* top;
public:
Stack() : top(0) { }
~Stack()
{
while (top != 0)
{
Entry* tmp = top;
//**********found**********
top = _______________________; // 让top指向下一个结点
delete tmp;
}
}
void push(int data) // 入栈
//push函数是把入栈数据放入新结点中,并使之成为栈顶结点,原来的结点成为新结点的下一个结点
{
//**********found**********
top = new Entry(_______________________, data);
}
int pop()
{
if (top == 0) return 0;
//**********found**********
int result = _______________________; // 保存栈顶结点中的数据
top = top->next;
return result;
}
};
int main()
{
int a[] = { 0, 1, 2, 3, 4, 5, 6, 7, 8, 9 };
Stack s;
int i = 0;
for (i = 0; i < 10; i++) {
cout << a[i] << ' ';
s.push(a[i]);
}
cout << endl;
for (i = 0; i < 10; i++) {
cout << s.pop() << ' ';
}
cout << endl;
return 0;
}
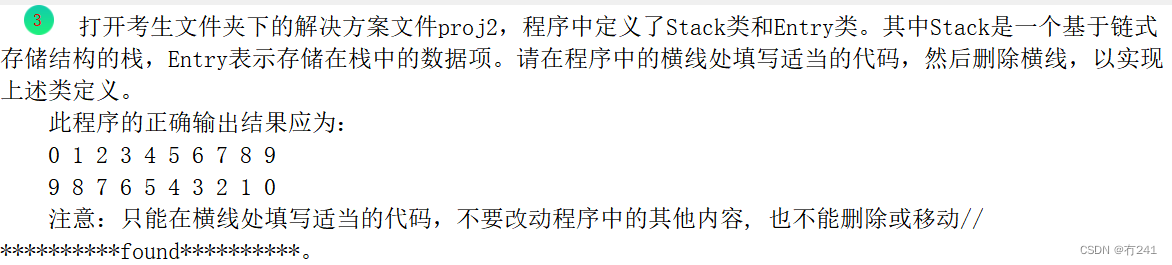
简单应用 答案
#include <iostream>
using namespace std;
class Entry { //链接栈的结点
public:
Entry* next; // 指向下一个结点的指针
int data; // 结点数据
//**********found**********
Entry(Entry* n, int d) : next(n), data(d) { }
};
class Stack {
Entry* top;
public:
Stack() : top(0) { }
~Stack()
{
while (top != 0)
{
Entry* tmp = top;
//**********found**********
top = top->next; // 让top指向下一个结点
delete tmp;
}
}
void push(int data) // 入栈
//push函数是把入栈数据放入新结点中,并使之成为栈顶结点,原来的结点成为新结点的下一个结点
{
//**********found**********
top = new Entry(top, data);
}
int pop()
{
if (top == 0) return 0;
//**********found**********
int result = top->data; // 保存栈顶结点中的数据
top = top->next;
return result;
}
};
int main()
{
int a[] = { 0, 1, 2, 3, 4, 5, 6, 7, 8, 9 };
Stack s;
int i = 0;
for (i = 0; i < 10; i++) {
cout << a[i] << ' ';
s.push(a[i]);
}
cout << endl;
for (i = 0; i < 10; i++) {
cout << s.pop() << ' ';
}
cout << endl;
return 0;
}
综合应用 原题
//main.cpp
#include<iostream>
#include "Array.h"
using namespace std;
//由a和b带回数组a中最小的两个值
template<class Type>
void Array<Type>::MinTwo(Type& x1, Type& x2) const { //补充完整函数体的内容
a[0]<=a[1]? (x1=a[0],x2=a[1]): (x1=a[1],x2=a[0]);
//********333********
//********666********
}
int main() {
int s1[8]={29,20,33,12,18,66,25,14};
Array<int> d1(s1,8);
int i,a,b;
d1.MinTwo(a,b);
cout<<d1.Length()<<endl;
for(i=0;i<7;i++) cout<<d1[i]<<", "; cout<<d1[7]<<endl;
cout<<a<<", "<<b<<endl;
writeToFile(".\\"); //不用考虑此语句的作用
return 0;
}
//Array.h
#include<iostream>
#include<string>
#include<cstdlib>
using namespace std;
template<class Type>
class Array { //数组类
public:
Array(Type b[], int mm):size(mm) { //构造函数
if(size<2) {cout<<"数组长度太小,退出运行!"; exit(1);}
a=new Type[size];
for(int i=0; i<size; i++) a[i]=b[i];
}
~Array() {delete []a;} //析构函数
void MinTwo(Type& x1, Type& x2) const; //由x1和x2带回数组a中最小的两个值
int Length() const{ return size;} //返回数组长度
Type operator [](int i)const { //下标运算符重载为成员函数
if(i<0 || i>=size) {cout<<"下标越界!"<<endl; exit(1);}
return a[i];
}
private:
Type *a;
int size;
};
void writeToFile(string path); //不用考虑此语句的作用
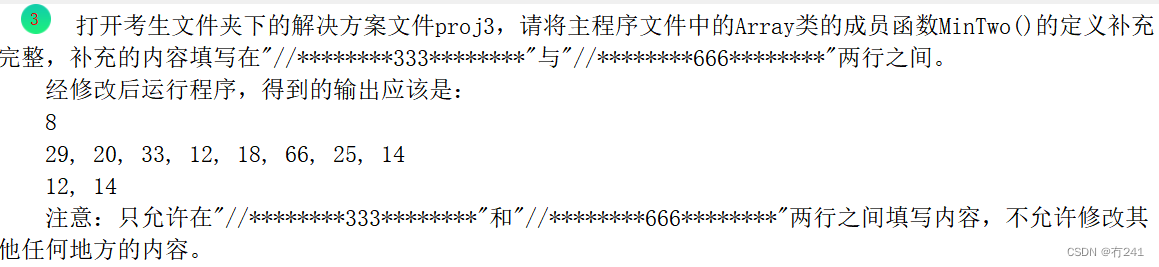
综合应用 答案
#include<iostream>
#include "Array.h"
using namespace std;
//由a和b带回数组a中最小的两个值
template<class Type>
void Array<Type>::MinTwo(Type& x1, Type& x2) const { //补充完整函数体的内容
a[0]<=a[1]? (x1=a[0],x2=a[1]): (x1=a[1],x2=a[0]);
//********333********
for(int i=1;i<size;i++)
{
a[i]<x1 ? (x2=x1, x1=a[i]) : (x2=x2<a[i]?x2:a[i]);
}
//********666********
}
int main() {
int s1[8]={29,20,33,12,18,66,25,14};
Array<int> d1(s1,8);
int i,a,b;
d1.MinTwo(a,b);
cout<<d1.Length()<<endl;
for(i=0;i<7;i++) cout<<d1[i]<<", "; cout<<d1[7]<<endl;
cout<<a<<", "<<b<<endl;
writeToFile(".\\"); //不用考虑此语句的作用
return 0;
}