1. 从文件中读取数据
1.1. 读取整个文件
在同目录下创建textA文本文件
123
456
789
a. open函数:要以任何方式去使用文件,都需要先打开文件,它接受一个参数——要打开文件的名称。 open()返回一个表示文件的对象。
b. 关键字with在不再需要访问文件后将其关闭。
c. 使用read()方法可以读取文件中的内容,并将其作为一个长字符串存储在变量中。read()到达文件末尾时返回一个空字符串。
with open('textA') as text:
contents = text.read()
print(contents)
# 123
# 456
# 789
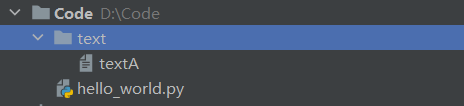
1.1.1 文件路径
使用相对路径
with open('text/textA') as text:
contents = text.read()
print(contents)
# 123
# 456
# 789
使用绝对路径
file_path = 'D:\Code\\text\\textA'
with open(file_path) as obj:
contents = obj.read()
print(contents.rstrip())
# 123
# 456
# 789
1.1.2 逐行读取
file_path = 'D:\Code\\text\\textA'
with open(file_path) as obj:
for line in obj:
print(line.rstrip())
# 123
# 456
# 789
1.1.3 创建一个包含文件各行内容的列表
注意:使用方法readlines,从文件中读取每一行,将其存储在一个列表中。
file_path = 'D:\Code\\text\\textA'
with open(file_path) as obj:
list = obj.readlines()
for line in list:
print(line.rstrip())
print(list)
# 123
# 456
# 789
# ['123\n', '456\n', '789']
1.2. 使用文件的内容
file_path = 'D:\Code\\text\\textA'
with open(file_path) as file:
file_list = file.readlines()
str = ''
for line in file_list:
str += line.rstrip()
print(str)
print(len(str))
# 123456789
# 9
1.2.1 使用replace修改文件中的内容
textA
I like cat!
The cat is very cute!
hello_world.py
file_path = 'D:\Code\\text\\textA'
with open(file_path) as file:
contents = file.read()
print(contents.replace('cat', 'dog'))
# I like dog!
# The dog is very cute!
2. 写入文件
注意:
open函数可接受两个实参,一个是要打开文件的名称,另一个是以什么样的模式打开这个文件。其中,模式有:r(读取模式)、w(写入模式)、a(附加模式)、r+(能够读取和写入文件的模式)。如果省略模式实参,将默认以只读模式打开文件。
如果写入的文件不存在,则open函数将创建这个文件;如果写入的文件是存在的,则python在返回文件对象前会清空该文件。
文件对象的方法——write,用于将字符串写入文件。
file_name = 'D:\Code\\text\\textA'
with open(file_name, 'w') as file_object:
file_object.write('I love python!')
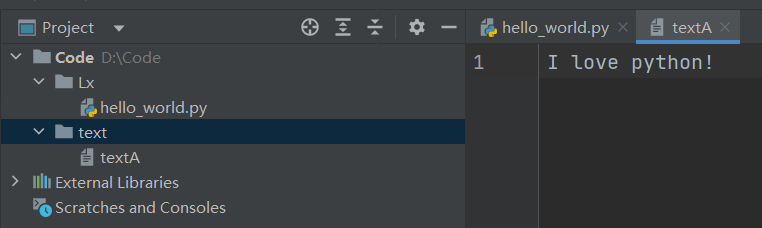
2.1. 写入多行
注意:加换行符
file_name = 'D:\Code\\text\\textA'
with open(file_name, 'w') as file_object:
file_object.write('I love python!\nFighting!')
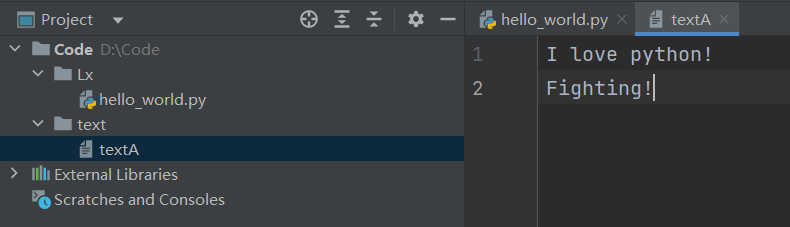
2.2. 附加到文件
注意:
若要给文件添加内容,而不是覆盖原有的内容,则可用附加模式a打开文件。
若指定的文件不存在,则会创建一个空文件。
该方法添加的内容都在文件末尾。
hello_world.py
file_name = 'D:\Code\\text\\textA'
with open(file_name, 'a') as file_object:
file_object.write('come on\n')
file_object.write('If you can, you can.')
textA
I love python!
Fighting!
come on
If you can, you can.
2.3. 习题
1.访客:编写一个程序,提示用户输入其名字;用户作出响应后,将其名字写入到文件guest中。
hello_world.py
username = input('Please input your name: ')
file_name = 'D:\Code\\text\\guest'
with open(file_name, 'a') as file_object:
file_object.write(username.title() + '\n')
guest
Ann
Bob
2.访客名单:编写一个while循环,提示用户输入其名字。用户输入其名字后,在屏幕上打印一句问候语,并将一条访问记录添加到文件guest_book中。确保这个文件中的每条记录都独占一行。
hello_world.py
file_name = 'D:\Code\\text\guest'
def write_fun(str, file_name):
with open(file_name, 'a') as file_object:
file_object.write(str + '\n')
while True:
username = input('Please input your name: ')
str = 'Hello,' + username.title()
print(str)
write_fun(str, file_name)
# Please input your name: tom
# Hello,Tom
# Please input your name: bob
# Hello,Bob
# Please input your name:
guest
Hello,Tom
Hello,Bob
3. 异常
Python使用称为异常的特殊对象来去处理程序执行期间发生的错误。当发生让Python不知所措的错误时,会创建一个异常对象。如果你编写了处理异常的代码,则程序继续运行;若没有对异常进行处理,则程序停止,并显示traceback,里面包含有关异常的报告。
3.1. 处理ZeroDivisionError异常
print(2/0)
显示:
Traceback (most recent call last):
File "D:\Code\hello_world.py", line 1, in <module>
print(2/0)
ZeroDivisionError: division by zero
注意:这里的ZeroDivisionError是一个异常对象,我们可以根据这种信息对程序进行修改,告诉Python发生错误时,该怎么处理。
3.1.1 使用try-except代码块
注意:
若try代码块中的代码执行起来没有问题,则跳过except代码块
若try代码块中代码执行起来有问题,则运行except中的代码块
若try-except代码块后面还有代码,则将继续执行下去
try:
print(2 / 0)
except ZeroDivisionError:
print("you can't divide by zero!")
# you can't divide by zero!
3.2. try-except-else代码块
工作原理:
try:将可能引发异常的代码放入try代码块中
else:存放仅在try代码块成功执行时才需要运行的代码
except:处理引发指定的异常时的情况
print("Enter 'q' to quit.")
while True:
a = input('Please input a number: ')
if a == 'q':
break
b = input('Please input a number: ')
if b == 'q':
break
try:
result = int(a) / int(b)
except ZeroDivisionError:
print("You can't divide by zero!")
else:
print(result)
# Enter 'q' to quit.
# Please input a number: 5
# Please input a number: 0
# You can't divide by zero!
# Please input a number: 8
# Please input a number: 4
# 2.0
# Please input a number: q
3.3. 处理FileNotFoundError异常
示例:不存在a这个文件
file_name = 'a.txt'
try:
with open(file_name) as file_obj:
contents = file_obj.read()
except FileNotFoundError:
print(file_name + ' is not exist!')
else:
print(contents)
# a.txt is not exist!
3.3.1 分析文本
注意:split方法可将字符串生成列表
a
Tom is my best friend, and I like him.
hello_world.py
file_name = 'D:\Code\lx\\a'
try:
with open(file_name) as file_obj:
contents = file_obj.read()
except FileNotFoundError:
print(file_name + ' is not exist!')
else:
words = contents.split()
print(len(words))
# 9
3.3.2 使用多个文件
a
Tom is my best friend, and I like him.
b
Jerry is my best friend.
hello_world.py
def count_word(file_name):
'''文件单词数量'''
try:
with open(file_name) as file_obj:
contents = file_obj.read()
except FileNotFoundError:
print(file_name + ' is not exist!')
else:
words = contents.split()
print(len(words))
file_list = ['D:\Code\lx\\a', 'D:\Code\lx\\b', 'D:\Code\lx\\c']
for file in file_list:
count_word(file)
# 9
# 5
# D:\Code\lx\c is not exist!
3.3.3 失败时不做处理
注意:使用pass语句,可以让Python什么都不做。
def count_word(file_name):
'''文件单词数量'''
try:
with open(file_name) as file_obj:
contents = file_obj.read()
except FileNotFoundError:
pass
else:
words = contents.split()
print(len(words))
file_list = ['D:\Code\lx\\a', 'D:\Code\lx\\b', 'D:\Code\lx\\c']
for file in file_list:
count_word(file)
# 9
# 5
3.4. 补充count方法
注意:count方法用来确定特定的单词或短语在字符串中出现了多少次
str = 'dog dog dog cat cat dogdog'
print(str.count('dog'))
# 5
4. 存储数据
可以使用模块json来存储数据,以JSON格式存储的数据也可以与使用其他编程语言的人分享
4.1. 使用json.dump()和json.load()
json.dump用来存储数据,json.load将数据读取到内存中的程序,使用时需先导入json模块
json.dump(要存储的数据,可用于存储数据的文件对象)
使用文件扩展名.json指出文件存储的数据为JSON格式
1.使用json.dump将数字列表存储到文件number_list.json中
hello_world.py
import json
numbers = [1, 2, 3]
with open('number_list.json', 'w') as file_obj:
json.dump(numbers, file_obj)
number_list.json
[1, 2, 3]
2.使用json.load将列表读取到内存中
import json
with open('number_list.json') as file_obj:
contents = json.load(file_obj)
print(contents)
# [1, 2, 3]
4.2. 保存和读取用户生成的数据
hello_world.py
import json
file_name = 'username_list.json'
try:
with open(file_name) as file_obj:
username = json.load(file_obj)
except FileNotFoundError:
username = input("What's your name? ")
with open(file_name, 'w') as file_obj:
json.dump(username, file_obj)
print("We'll remember you at the next time," + username + '.')
else:
print(username.title() + ', welcome back!')
# What's your name? bob
# We'll remember you at the next time,bob.
# Bob, welcome back!
username_list.json
"bob"
5. 重构
将代码划分为一系列完成具体工作的函数,这一过程称为重构。重构让代码更清晰、更易于理解以及更容易扩展。
hello_world.py
import json
file_name = 'username_list.json'
def get_stored_user():
'''若存储了用户名,则使用它'''
try:
with open(file_name) as file_obj:
username = json.load(file_obj)
except FileNotFoundError:
return None
else:
return username
def get_new_user():
'''存储新用户'''
username = input("What's your name? ")
with open(file_name, 'w') as file_obj:
json.dump(username, file_obj)
return username
def greet_user():
'''问候用户'''
username = get_stored_user()
if username:
print(username.title() + ', welcome back!')
else:
username = get_new_user()
print("We'll remember you at the next time," + username + '.')
greet_user()
# What's your name? bob
# We'll remember you at the next time,bob.
# Bob, welcome back!
username_list.json
"bob"