登录页面
1.登录页面编码
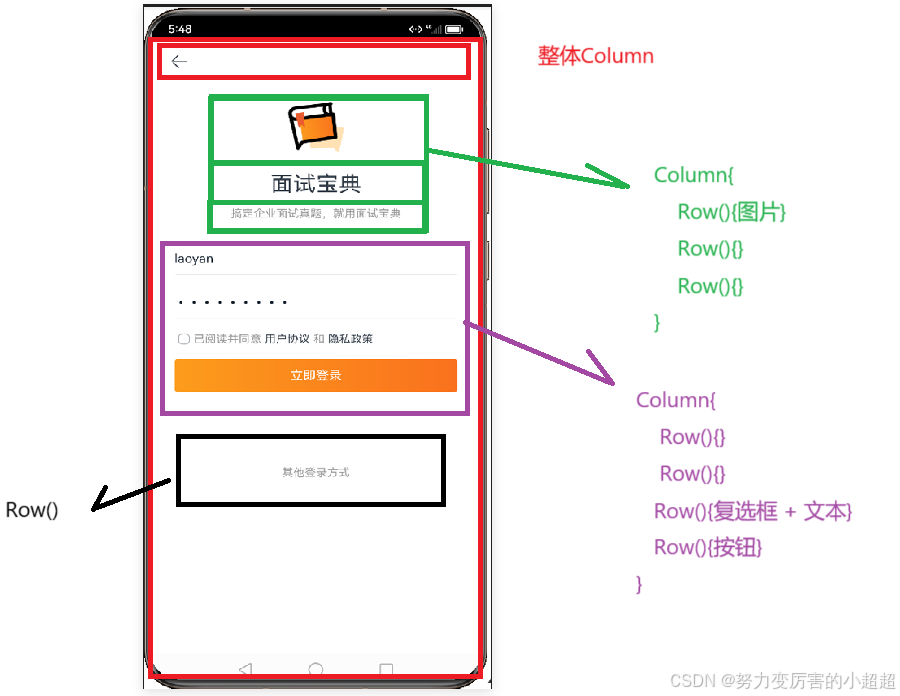
@Extend(TextInput) function customStyle(){
.backgroundColor('#fff')
.border({width:{bottom:0.5},color:'#e4e4e4'})
.borderRadius(1)
.placeholderColor('#c3c3c5')
.caretColor('#fa711d')
}
@Entry
@Component
struct LoginPage {
build() {
Column(){
Column(){
Image($r('app.media.avatar'))
.width(120)
.aspectRatio(1)
Text('面试宝典')
.fontSize(28)
.margin({bottom:15})
Text('搞定企业面试真题,就用面试宝典')
.fontSize(15)
.fontColor('gray')
}
Column({space:15}){
TextInput({placeholder:'请输入账号'})
.customStyle()
TextInput({placeholder:'请输入密码'})
.customStyle()
Row(){
Checkbox()
.selectedColor('red')
.width(14)
.aspectRatio(1)
.shape(CheckBoxShape.ROUNDED_SQUARE)
Text('已阅读并同意')
.fontSize(14)
.fontColor('gray')
Text(' 用户协议 ')
.fontSize(14)
Text('和')
.fontSize(14)
.fontColor('gray')
Text(' 隐私政策')
.fontSize(14)
}
.width('100%')
Button({type:ButtonType.Normal,stateEffect:false}){
Text('立即登录')
.fontColor('#fff')
}
.width('100%')
.backgroundColor('transparent')
.borderRadius(4)
.height(44)
.linearGradient({
direction:GradientDirection.Right,
colors: [['#FC9B1C',0],['#FA711D',1]]
})
}
.padding({
top:30,
bottom:80
})
Column(){
Text('其它登录方式')
.fontSize(14)
.fontColor('gray')
}
}
.width('100%')
.height('100%')
.padding(15)
}
}
2.是否勾选的逻辑
import promptAction from '@ohos.promptAction';
@Extend(TextInput) function customStyle(){
.backgroundColor('#fff')
.border({width:{bottom:0.5},color:'#e4e4e4'})
.borderRadius(1)
.placeholderColor('#c3c3c5')
.caretColor('#fa711d')
}
@Entry
@Component
struct LoginPage {
@State isAgree:boolean = false;
login(){
if(!this.isAgree){
promptAction.showToast({
message: '请阅读条款',
duration: 5000
})
} else {
promptAction.showToast({
message: '登录成功',
duration: 5000
})
}
}
build() {
Column(){
Button({type:ButtonType.Normal,stateEffect:false}){
Text('立即登录')
.fontColor('#fff')
}
.width('100%')
.backgroundColor('transparent')
.borderRadius(4)
.height(44)
.linearGradient({
direction:GradientDirection.Right,
colors: [['#FC9B1C',0],['#FA711D',1]]
})
.onClick(() => {
this.login();
})
}
.width('100%')
.height('100%')
.padding(15)
}
}
Checkbox(){
}
.onchange((value)=>{
this.isAggree = value
})
3.密码框
TextInput({placeholder:'请输入密码'})
.customStyle()
.type(InputType.Password)
4.获取数据
import promptAction from '@ohos.promptAction';
@Extend(TextInput) function customStyle(){
.backgroundColor('#fff')
.border({width:{bottom:0.5},color:'#e4e4e4'})
.borderRadius(1)
.placeholderColor('#c3c3c5')
.caretColor('#fa711d')
}
@Entry
@Component
struct LoginPage {
@State isAgree:boolean = false;
@State username:string = '';
@State password:string = '';
login(){
if(!this.isAgree){
promptAction.showToast({
message: '请阅读条款',
duration: 5000
})
return;
}
let reg = /^[a-zA-Z0-9_]{3,10}$/
if(reg.test(this.username) == false){
promptAction.showToast({
message: '用户名输入格式错误',
duration: 5000
})
return;
}
let reg_pwd = /^[a-zA-Z0-9_]{6,}$/
if(reg_pwd.test(this.password) == false){
promptAction.showToast({
message: '密码输入格式错误',
duration: 5000
})
return;
}
promptAction.showToast({
message: '登录成功'+ this.username + '--->'+ this.password,
duration: 5000
})
}
build() {
Column(){
Column({space:15}){
TextInput({placeholder:'请输入账号'})
.customStyle()
.onChange((value)=>{
this.username = value
})
TextInput({placeholder:'请输入密码'})
.customStyle()
.type(InputType.Password)
.onChange((value)=>{
this.password = value
})
Row(){
Checkbox()
.selectedColor('red')
.width(14)
.aspectRatio(1)
.shape(CheckBoxShape.ROUNDED_SQUARE)
.onChange((value)=>{
this.isAgree = value
})
}
.width('100%')
Button({type:ButtonType.Normal,stateEffect:false}){
Text('立即登录')
.fontColor('#fff')
}
.width('100%')
.backgroundColor('transparent')
.borderRadius(4)
.height(44)
.linearGradient({
direction:GradientDirection.Right,
colors: [['#FC9B1C',0],['#FA711D',1]]
})
.onClick(() => {
this.login();
})
}
}
.width('100%')
.height('100%')
.padding(15)
}
}