1. 什么是MyBatis?
MyBatis是⼀款优秀的 持久层 框架,⽤于简化JDBC的开发
总结:简单来说 MyBatis 是更简单完成程序和数据库交互的框架,也就是更简单的操作和读取数据库⼯具
2.MyBatis⼊⻔
1. 准备⼯作(创建springboot⼯程、数据库表准备、实体类)
2. 引⼊Mybatis的相关依赖,配置Mybatis(数据库连接信息)
3. 编写SQL语句(注解/XML)
4. 测试
2.1 准备⼯作
2.1.1 创建⼯程
创建springboot⼯程,并导⼊ mybatis的起步依赖、mysql的驱动包
PS:Mybatis 是⼀个持久层框架, 具体的数据存储和数据操作还是在MySQL中操作的, 所以需要添加 MySQL驱动
项⽬⼯程创建完成后,⾃动在pom.xml⽂件中,导⼊Mybatis依赖和MySQL驱动依赖
1 <!--Mybatis 依赖包-->
2 <dependency>
3 <groupId>org.mybatis.spring.boot</groupId>
4 <artifactId>mybatis-spring-boot-starter</artifactId>
5 <version>2.3.1</version>
6 </dependency>
7 <!--mysql驱动包-->
8 <dependency>
9 <groupId>com.mysql</groupId>
10 <artifactId>mysql-connector-j</artifactId>
11 <scope>runtime</scope>
12 </dependency>
2.2 配置数据库连接字符串
Mybatis中要连接数据库,需要数据库相关参数配置
• MySQL驱动类
• 登录名
• 密码
• 数据库连接字符串
如果是application.yml⽂件, 配置内容如下:
1 # 数据库连接配置
2 spring:
3 datasource:
4 url: jdbc:mysql://127.0.0.1:3306/mybatis_test?
characterEncoding=utf8&useSSL=false
5 username: root
6 password: root
7 driver-class-name: com.mysql.cj.jdbc.Driver
如果是application.properties⽂件, 配置内容如下:
1 #驱动类名称
2 spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
3 #数据库连接的url
4 spring.datasource.url=jdbc:mysql://127.0.0.1:3306/mybatis_test?
characterEncoding=utf8&useSSL=false
5 #连接数据库的⽤⼾名
6 spring.datasource.username=root
7 #连接数据库的密码
8 spring.datasource.password=root
2.3 写持久层代码
//示例
@Mapper
public interface UserInfoMapper {
//查询所有⽤⼾
@Select("select username, `password`, age, gender, phone from userinfo")
public List<UserInfo> queryAllUser();
}
- Mybatis的持久层接⼝规范⼀般都叫 XxxMapper
- @Mapper注解:表⽰是MyBatis中的Mapper接⼝
2.4 单元测试
9 @SpringBootTest
10 class DemoApplicationTests {
11
12 @Autowired
13 private UserInfoMapper userInfoMapper;
14
15 @Test
16 void contextLoads() {
17 List<UserInfo> userInfoList = userInfoMapper.queryAllUser();
18 System.out.println(userInfoList);
19 }
20 }
-
测试类上添加了注解 @SpringBootTest,该测试类在运⾏时,就会⾃动加载Spring的运⾏环境.
-
@Autowired这个注解, 注⼊我们要测试的类, 就可以开始进⾏测试了
3. MyBatis的基础操作
3.1 打印⽇志
- 在Mybatis当中我们可以借助⽇志, 查看到sql语句的执⾏、执⾏传递的参数以及执⾏结果
1 mybatis:2 configuration: # 配置打印 MyBatis ⽇志3 log-impl: org.apache.ibatis.logging.stdout.StdOutImpl
1 # 指定 mybatis 输出⽇志的位置 , 输出控制台2 mybatis.configuration.log-impl = org.apache.ibatis.logging.stdout.StdOutImpl
3.2 参数传递
1 @Select("select username, `password`, age, gender, phone from userinfo whereid= 4 ")2 UserInfo queryById ();
- 但是这样的话, 只能查找id=4 的数据, 所以SQL语句中的id值不能写成固定数值,需要变为动态的数值
- 解决⽅案:在queryById⽅法中添加⼀个参数(id),将⽅法中的参数,传给SQL语句 使⽤ #{} 的⽅式获取⽅法中的参数
#{id}
是一个占位符,表示id
变量的值将在运行时替换进来
1 @Select("select username, `password`, age, gender, phone from userinfo whereid= #{id} ")2 UserInfo queryById (Integer id);
如果mapper接⼝⽅法形参只有⼀个普通类型的参数,#{…} ⾥⾯的属性名可以随便写,如:#{id}、# {value}。建议和参数名保持⼀致
添加测试⽤例
1 @Test2 void queryById () {3 UserInfo userInfo = userInfoMapper.queryById( 4 );4 System.out.println(userInfo);5 }
3.3 增(Insert)
insert into userinfo (username, `password`, age, gender, phone) values("zhaoliu","zhaoliu", 19 , 1 ,"18700001234")
@Insert("insert into userinfo (username, `password`, age, gender, phone)values (#{username},#{password},#{age},#{gender},#{phone})")Integer insert (UserInfo userInfo);
1 @Test2 void insert () {3 UserInfo userInfo = new UserInfo ();4 userInfo.setUsername( "zhaoliu" );5 userInfo.setPassword( "zhaoliu" );6 userInfo.setGender( 2 );7 userInfo.setAge( 21 );8 userInfo.setPhone( "18612340005" );9 userInfoMapper.insert(userInfo);10 }
@Options(useGeneratedKeys = true, keyProperty = "id")@Insert("insert into userinfo (username, age, gender, phone) values(# {userinfo.username},#{userinfo.age},#{userinfo.gender},#{userinfo.phone})")Integer insert ( @Param("userinfo") UserInfo userInfo);
1 @Test2 void insert () {3 UserInfo userInfo = new UserInfo ();4 userInfo.setUsername( "zhaoliu" );5 userInfo.setPassword( "zhaoliu" );6 userInfo.setGender( 2 );7 userInfo.setAge( 21 );8 userInfo.setPhone( "18612340005" );9 Integer count = userInfoMapper.insert(userInfo);10 System.out.println( " 添加数据条数 :" +count + ", 数据 ID:" + userInfo.getId());11 }
3.4 删(Delete)
@Delete("delete from userinfo where id = #{id}")void delete (Integer id);
3.5 改(Update)
1 @Update("update userinfo set username=#{username} where id=#{id}")2 void update (UserInfo userInfo);
3.6 查(Select)
1 @Select("select id, username, `password`, age, gender, phone, delete_flag,create_time, update_time from userinfo")2 List<UserInfo> queryAllUser ();
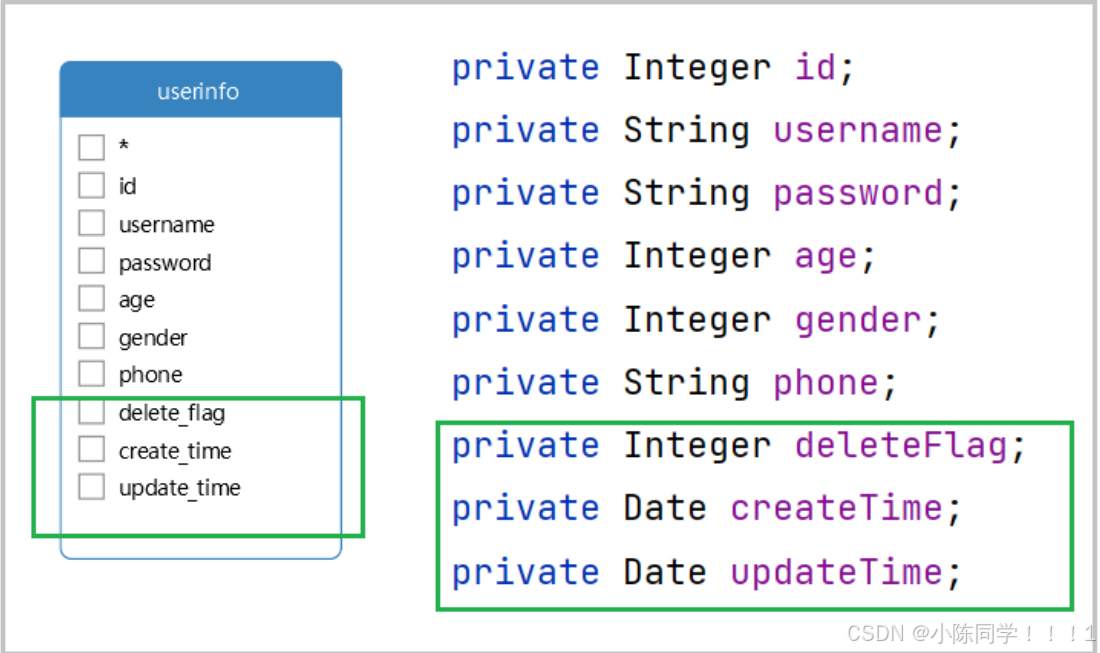
3.6.1 起别名
@Select("select id, username, `password`, age, gender, phone, delete_flag asdeleteFlag, " + "create_time as createTime, update_time as updateTime from userinfo")public List<UserInfo> queryAllUser ();
PS:SQL语句太⻓时, 使⽤加号 + 进⾏字符串拼接
3.6.2 结果映射
1 @Select("select id, username, `password`, age, gender, phone, delete_flag,create_time, update_time from userinfo")2 @Results({3 @Result(column = "delete_flag",property = "deleteFlag"),4 @Result(column = "create_time",property = "createTime"),5 @Result(column = "update_time",property = "updateTime")6 })7 List<UserInfo> queryAllUser ();
column
属性:指定数据库查询结果集中的列名。property
属性:指定 Java 对象中的属性名,这个属性会接收column
属性指定的列的值。
如果其他SQL, 也希望可以复⽤这个映射关系, 可以给这个Results定义⼀个名称
3.6.3 开启驼峰命名(推荐)
- 通常数据库列使⽤蛇形命名法进⾏命名(下划线分割各个单词), ⽽ Java 属性⼀般遵循驼峰命名法约定.
- 为了在这两种命名⽅式之间启⽤⾃动映射,需要将 mapUnderscoreToCamelCase 设置为 true。
-
驼峰命名规则: abc_xyz => abcXyz• 表中字段名:abc_xyz• 类中属性名:abcXyz
4. MyBatis XML配置⽂件
- 使⽤Mybatis的注解⽅式,主要是来完成⼀些简单的增删改查功能. 如果需要实现复杂的SQL功能,建 议使⽤XML来配置映射语句,也就是将SQL语句写在XML配置⽂件中
-
MyBatis XML的⽅式需要以下两步
4.1 配置连接字符串和MyBatis
1 # 数据库连接配置2 spring:3 datasource:4 url: jdbc:mysql://127.0.0.1:3306/mybatis_test?characterEncoding=utf8&useSSL=false5 username: root6 password: root7 driver-class-name: com.mysql.cj.jdbc.Driver8 # 配置 mybatis xml 的⽂件路径,在 resources/mapper 创建所有表的 xml ⽂件9 mybatis:10 mapper-locations: classpath:mapper/**Mapper.xml
4.2 写持久层代码
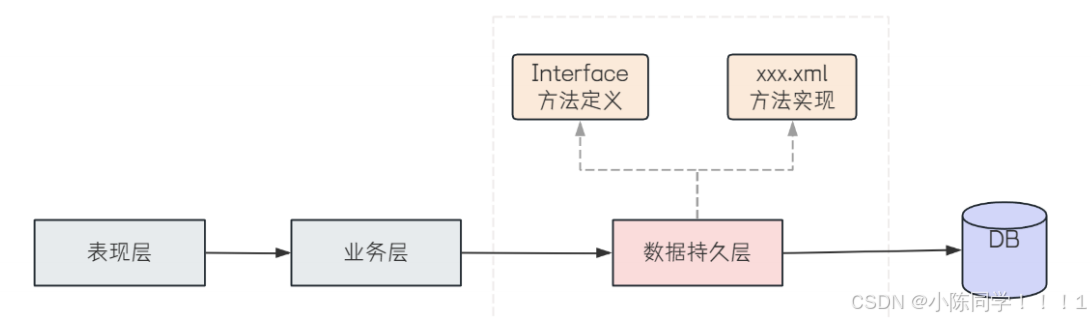
4.2.1 添加 mapper 接⼝
@Mapperpublic interface UserInfoXMlMapper {List<UserInfo> queryAllUser ();}
MyBatis 的固定 xml 格式:
1 <?xml version= "1.0" encoding= "UTF-8" ?>2 <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN""http://mybatis.org/dtd/mybatis-3-mapper.dtd" >3 < mapper namespace = "com.example.demo.mapper.UserInfoMapper" >45 </ mapper >
具体实现:
1 <?xml version= "1.0" encoding= "UTF-8" ?>2 <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN""http://mybatis.org/dtd/mybatis-3-mapper.dtd" >3 < mapper namespace = "com.example.demo.mapper.UserInfoXMlMapper" >4 < select id = "queryAllUser" resultType = "com.example.demo.model.UserInfo" >5 select username,`password`, age, gender, phone from userinfo6 </ select >7 </ mapper >
4.3 增删改查操作
4.3.1 增(Insert)
Integer insertUser (UserInfo userInfo);
< insert id = "insertUser" >insert into userinfo (username, `password`, age, gender, phone) values (#{username}, #{password}, #{age},#{gender},#{phone})</ insert >
4.3.2 删(Delete)
Integer deleteUser (Integer id);
UserInfoMapper.xml实现:
1 < delete id = "deleteUser" >2 delete from userinfo where id = #{id}3 </ delete >
4.3.3 改(Update)
Integer updateUser (UserInfo userInfo);
UserInfoMapper.xml实现
< update id = "updateUser" >update userinfo set username=#{username} where id=#{id}</ update >
4.3.4 查(Select)
1 < select id = "queryAllUser" resultType = "com.example.demo.model.UserInfo" >2 select id, username,`password`, age, gender, phone, delete_flag,create_time, update_time from userinfo3 </ select >
5. 其他查询操作
5.1 多表查询
5.1.1 准备⼯作
(表就不展示了,看代码理解代码即可)
5.1.2 数据查询
1 SELECT2 ta.id,3 ta.title,4 ta.content,5 ta.uid,6 tb.username,7 tb.age, 8 tb.gender9 FROM10 articleinfo ta11 LEFT JOIN userinfo tb ON ta.uid = tb.id12 WHERE13 ta.id = 1
接⼝定义:
1 import com.example.demo.model.ArticleInfo;2 import org.apache.ibatis.annotations.Mapper;34 @Mapper5 public interface ArticleInfoMapper {67 @Select("SELECTta.id,ta.title,ta.content,ta.uid,tb.username,tb.age,tb.gender " +8 "FROM articleinfo ta LEFT JOIN userinfo tb ON ta.uid = tb.id " +9 "WHERE ta.id = #{id}")10 ArticleInfo queryUserByUid (Integer id);11 }