目录
2.2 boolean equals(Object anObject) 方法:按照字典序(字符大小的顺序)比较
2.3 int compareTo(String s) 方法: 按照字典序进行比较
2.4.int compareToIgnoreCase(String str) 方法:与compareTo方式相同,但是忽略大小写比较
1.字符串的构造
我们先来简单认识一下,String的构造方式
public static void main(String[] args) {
// 使用常量串构造
String a1 = "hello,there";
System.out.println(a1);
// 直接newString对象
String a2 = new String("hello,there");
System.out.println(a2);
// 使用字符数组进行构造
char[] array = {'h', 'e', 'l', 'l', 'o', 't', 'h', 'e', 'r', 'e'};
String a3 = new String(array);
System.out.println(a3);
}
public static void main(String[] args) {
// a1和a2引用的是不同对象 a1和a3引用的是同一对象
String a1 = "hello";
String a2 = "there";
String a3 = a1;
// 获取字符串长度---输出5
System.out.println(a1.length());
// 如果字符串长度为0,返回true,否则返回false
System.out.println(a1.isEmpty());
}
2.String对象的比较
2.1 '==' 比较是否引用同一个对象
public static void main(String[] args) {
int a = 10;
int b = 20;
int c = 10;
// 对于基本类型变量,==比较两个变量中存储的值是否相同
System.out.println(a == b);
System.out.println(a == c);
// 对于引用类型变量,==比较两个引用变量引用的是否为同一个对象
String a1 = new String("hello,there");
String a2 = new String("hello,there");
String a3 = new String("hello");
String a4 = a1;
System.out.println(a1 == a2);
System.out.println(a2 == a3);
System.out.println(a4 == a1);
}
对于内置类型,
==
比较的是变量中的值;对于引用类型
==
比较的是引用中的地址。
2.2 boolean equals(Object anObject) 方法:按照字典序(字符大小的顺序)比较
String
类重写了父类
Object
中
equals
方法,
Object
中
equals
默认按照
==
比较,
String
重写
equals
方法
public static void main(String[] args) {
String a1 = new String("hi");
String a2 = new String("hi");
String a3 = new String("hi");
//a1、a2、a3引用的是三个不同对象,因此==比较结果全部为false
System.out.println(a1 == a2);
System.out.println(a2 == a3);
// equals比较:String对象中的逐个字符
// 虽然a1与a2引用的不是同一个对象,但是两个对象中放置的内容相同,因此输出true
// a1与a3引用的不是同一个对象,而且两个对象中内容也不同,因此输出false
System.out.println(a1.equals(a2));
System.out.println(a2.equals(a3));
}
2.3 int compareTo(String s) 方法: 按照字典序进行比较
与
equals
不同的是,
equals
返回的是
boolean
类型,而
compareTo
返回的是
int
类型。具体比较方式:
1.
先按照字典次序大小比较,如果出现不等的字符,直接返回这两个字符的大小差值
2.
如果前
k
个字符相等
(k
为两个字符长度最小值
)
,返回值两个字符串长度差值
public static void main(String[] args) {
String a1 = "zxc";
String a2 = "zx";
String a3 = "zxc";
String a4 = "zxcvbn";
// 不同输出字符差值-1
System.out.println(a1.compareTo(a2));
// 相同输出 0
System.out.println(a1.compareTo(a3));
// 前k个字符完全相同,输出长度差值 -3
System.out.println(a1.compareTo(a4));
}
2.4.int compareToIgnoreCase(String str) 方法:与compareTo方式相同,但是忽略大小写比较
public static void main(String[] args) {
String a1 = "zxc";
String a2 = "zx";
String a3 = "ZXc";
String a4 = "zxcvbn";
// 不同输出字符差值-1
System.out.println(a1.compareToIgnoreCase(a2));
// 相同输出 0
System.out.println(a1.compareToIgnoreCase(a3));
// 前k个字符完全相同,输出长度差值 -3 }
System.out.println(a1.compareToIgnoreCase(a4));
}
3. 字符串查找
方法
|
功能
|
char charAt(int index)
|
返回
index
位置上字符,如果
index
为负数或者越界,抛出 IndexOutOfBoundsException异常
|
int indexOf(int ch)
|
返回
ch
第一次出现的位置,没有返回
-1
|
int indexOf(int ch,
int fromIndex)
|
从
fromIndex
位置开始找
ch
第一次出现的位置,没有返回
-1
|
int indexOf(String str)
|
返回
str
第一次出现的位置,没有返回
-1
|
int indexOf(String str,
int fromIndex)
|
从
fromIndex
位置开始找
str
第一次出现的位置,没有返回
-1
|
int lastIndexOf(int ch)
|
从后往前找,返回
ch
第一次出现的位置,没有返回
-1
|
int lastIndexOf(int ch, int
fromIndex)
|
从
fromIndex
位置开始找,从后往前找
ch
第一次出现的位置,没有返
回
-1
|
int lastIndexOf(String str)
|
从后往前找,返回
str
第一次出现的位置,没有返回
-1
|
int lastIndexOf(String str, int fromIndex)
|
从
fromIndex
位置开始找,从后往前找
str
第一次出现的位置,没有返回-1
|
第一个例子演示 :返回index位置上字符,如果index为负数或者越界,
抛出 IndexOutOfBoundsException异常
正确演示
public static void main(String[] args) {
String a1 = "aabbccddeeffgg";
System.out.println(a1.charAt(3));
}
报错演示
public static void main(String[] args) {
String a1 = "aabbccddeeffgg";
System.out.println(a1.charAt(15));
}
第二个例子演示:返回ch第一次出现的位置,没有返回-1
public static void main(String[] args) {
String a1 = "aabbccddeeffgg";
System.out.println(a1.indexOf('d'));
System.out.println(a1.indexOf('z'));
}
第三个例子演示:从fromIndex位置开始找ch第一次出现的位置,没有返回-1
public static void main(String[] args) {
String a1 = "aabbccddeeffggffeeddccbbaa";
System.out.println(a1.indexOf("c",14));
System.out.println(a1.indexOf("f",40));
}
第四个例子演示:返回str第一次出现的位置,没有返回-1
public static void main(String[] args) {
String a1 = "aabbccddeeffggffeeddccbbaa";
System.out.println(a1.indexOf("cc"));
System.out.println(a1.indexOf("zz"));
}
第五个例子演示:从fromIndex位置开始找str第一次出现的位置,没有返回-1
public static void main(String[] args) {
String a1 = "aabbccddeeffggffeeddccbbaa";
System.out.println(a1.indexOf("aa",20));
System.out.println(a1.indexOf("zz",10));
}
第六个例子演示:从后往前找,返回ch第一次出现的位置,没有返回-1
public static void main(String[] args) {
String a1 = "aabbccddeeffggffeeddccbbaa";
System.out.println(a1.lastIndexOf("b"));
System.out.println(a1.lastIndexOf("z"));
}
第七个例子演示:从fromIndex位置开始找,从后往前找ch第一次出现的位置,
没有返回-1
public static void main(String[] args) {
String a1 = "aabbccddeeffggffeeddccbbaa";
System.out.println(a1.lastIndexOf("b",18));
System.out.println(a1.lastIndexOf("z",10));
}
第八个例子演示:从后往前找,返回str第一次出现的位置,没有返回-1
public static void main(String[] args) {
String a1 = "aabbccddeeffggffeeddccbbaa";
System.out.println(a1.lastIndexOf("bb"));
System.out.println(a1.lastIndexOf("zz"));
}
第九个例子演示:
从
fromIndex
位置开始找,从后往前找
str
第一次出现的位置,
没有返回-1
public static void main(String[] args) {
String a1 = "aabbccddeeffggffeeddccbbaa";
System.out.println(a1.lastIndexOf("bb",18));
System.out.println(a1.lastIndexOf("zz",10));
}
4 .转化
4.1.数值和字符串转化
class Student {
public String name;
public int age;
public Student(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return "Student{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
}
public class Test {
public static void main(String[] args) {
String str = String.valueOf(123);
System.out.println(str);
String str2 = String.valueOf(true);
System.out.println(str2);
String s4 = String.valueOf(new Student("zhangsan", 22));
System.out.println(s4);
System.out.println("=============");
//int val1 = Integer.parseInt("123");
int val1 = Integer.valueOf("123");
System.out.println(val1 + 1);
double val2 = Double.parseDouble("12.25");
System.out.println(val2);
}
4.2大小写转换
public static void main(String[] args) {
String s1 = "hello";
String s2 = "THERE";
//小写转大写
System.out.println(s1.toUpperCase());
//大写转小写
System.out.println(s2.toLowerCase());
}
4.3.字符串转数组
public static void main(String[] args) {
String s3 = "hello";
//字符串转数组
char[] ch = s3.toCharArray();
for (int i = 0; i < ch.length; i++) {
System.out.println(ch[i]);
}
System.out.println();
//数组转字符串
String s4 = new String(ch);
System.out.println(s4);
}
4.4格式化
public static void main(String[] args) {
String s1 = String.format("%d-%d-%d",2022,9,3);
System.out.println(s1);
}
5.字符串的替换
使用一个指定的新的字符串替换掉已有的字符串数据
方法
|
功能
|
String replaceAll(String regex, String replacement)
|
替换所有的指定内容
|
String replaceFirst(String regex, String replacement)
|
替换首个内容
|
第一个例子演示
public static void main(String[] args) {
String str = "hello,there";
System.out.println(str.replaceAll("l","_"));
}
第二个例子演示
public static void main(String[] args) {
String str = "hello,there";
System.out.println(str.replaceFirst("l","B"));
}
注意事项: 由于字符串是不可变对象, 替换不修改当前字符串, 而是产生一个新的字符串.
6.字符串拆分
可以将一个完整的字符串按照指定的分隔符划分为若干个子字符串。
拆分是特别常用的操作. 一定要重点掌握.
另外有些特殊字符作为分割符可能无法正确切分, 需要加上转义
.
方法
|
功能
|
String[] split(String regex)
|
将字符串全部拆分
|
String[] split(String regex, int limit)
|
将字符串以指定的格式,拆分为
limit
组
|
第一个例子演示
public static void main(String[] args) {
String str = "You are not prepared";
String[] result = str.split(" ");//按照空格拆分
for (String s : result) {
System.out.println(s);
}
}
第二个例子演示
public static void main(String[] args) {
String str = "You are not prepared";
String[] result = str.split(" ", 2);
for (String s : result) {
System.out.println(s);
}
}
拆分IP地址
public static void main(String[] args) {
String str = "2017.520.13.14";
String[] result = str.split("\\.");
for (String s : result) {
System.out.println(s);
}
}
注意事项
:
1. 字符"|","*","+"都得加上转义字符,前面加上 "\\" .
2. 而如果是 "\" ,那么就得写成 "\\\\" .
3. 如果一个字符串中有多个分隔符,可以用"|"作为连字符.
多次拆分
public static void main(String[] args) {
String str = "name=lisi&age=38";
String[] result = str.split("&");
for (int i = 0; i < result.length; i++) {
String[] temp = result[i].split("=");
System.out.println(temp[0] + " = " + temp[1]);
}
}
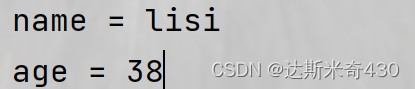
7 字符串截取
方法
|
功能
|
String substring(int beginIndex)
|
从指定索引截取到结尾
|
String substring(int beginIndex, int endIndex)
|
截取部分内容
|
例子演示
public static void main(String[] args) {
String str = "hellothere";
System.out.println(str.substring(5));
System.out.println(str.substring(0, 5));
}
注意事项
:
1. 索引从0开始
2. 注意前闭后开区间的写法, substring(0, 5) 表示包含 0 号下标的字符, 不包含 5 号下标
其他方法:trim()方法的使用
public static void main(String[] args) {
String str = " Hello There ";
System.out.println("["+str+"]");
System.out.println("["+str.trim()+"]");
}
trim 会去掉字符串开头和结尾的空白字符(空格, 换行, 制表符等).