文章目录
- 类的6个默认成员函数
- 构造函数
- 析构函数
- 拷贝构造函数
- 赋值操作符重载
- 默认拷贝构造与赋值运算符重载
- const成员函数
- 取地址及const取地址操作符重载
一、类的6个默认成员函数
如果一个类中没有任何成员,则这个类被称为空类。则编译器会自动生成6个默认成员函数。
默认成员函数:用户没有显示实现,编译器会生成的成员函数被称为默认成员函数。
class Date{ };
二、构造函数
构造函数是特殊的成员函数,需要注意的是,构造函数的虽然名称叫构造,但是需要注意的是构造函数的主要任务并不是开空间创建对象,而是初始化对象。
其特征如下:
1. 函数名与类名相同。
2. 无返回值。
3. 对象实例化时编译器自动调用对应的构造函数。
4. 构造函数可以重载。
#include<iostream>
using namespace std;
class Date
{
public:
// 1.无参构造函数
Date()
{}
// 2.带参构造函数
Date(int year, int month, int day)
{
_year = year;
_month = month;
_day = day;
}
private:
int _year;
int _month;
int _day;
};
void TestDate()
{
Date d1; // 调用无参构造函数
Date d2(2015, 1, 1); // 调用带参的构造函数
// 注意:如果通过无参构造函数创建对象时,对象后面不用跟括号,否则就成了函数声明
// 以下代码的函数:声明了d3函数,该函数无参,返回一个日期类型的对象
Date d3();
}
int main()
{
TestDate();
return 0;
}
5.如果类中没有显式定义构造函数,则C++编译器会自动生成一个无参的默认构造函数,一旦用户显式定义编译器将不再生成。
#include<iostream>
using namespace std;
typedef int DataType;
class Stack
{
public:
Stack(size_t capacity )
{
_array = (DataType*)malloc(sizeof(DataType)* capacity);
if (NULL == _array)
{
perror("malloc申请空间失败!!!");
return;
}
_capacity = capacity;
_size = 0;
}
void Push(DataType data)
{
// CheckCapacity();
_array[_size] = data;
_size++;
}
private:
DataType* _array;
int _capacity;
int _size;
};
class MyQueue {
public:
void push(int x) {}
//...
private:
size_t _size = 0;
Stack _st1;
Stack _st2;
};
int main()
{
//
// MyQueue 用默认生成构造函数就挺好,不需要显示写
MyQueue q;//如果此时已经有了构造函数则编译器不会再生成默认的构造函数,此时调用发现没有合适的默认构造函数
return 0;
}
6.无参的构造函数和全缺省的构造函数都称为默认构造函数,并且默认构造函数只能有一个。注意:无参构造函数、全缺省构造函数、我们没写编译器默认生成的构造函数,都可以认为是默认成员函数。
默认构造函数:1、编译器自动生成的构造函数2、全缺省构造函数3、无参构造函数
C++把类型分成内置类型(基本类型)和自定义类型。内置类型就是语法已经定义好的类型:如
int/char...,自定义类型就是我们使用class/struct/union自己定义的类型。
#include<iostream>
using namespace std;
class Time
{
public:
Time()
{
cout << "Time()" << endl;
_hour = 0;
_minute = 0;
_second = 0;
}
private:
int _hour;
int _minute;
int _second;
};
class Date
{
private:
// 基本类型(内置类型)
int _year;
int _month;
int _day;
// 自定义类型
Time _t;
};
int main()
{
Date d;//默认生成构造函数,a:内置类型成员不做处理 b:自定义类型成员回去调用他的默认构造函数
return 0;
}
三、析构函数
析构函数:与构造函数功能相反,析构函数不是完成对象的销毁,局部对象销毁工作是由编译器完成的。而对象在销毁时会自动调用析构函数,完成类的一些资源清理工作。
特性 :
析构函数是特殊的成员函数。
其特征如下:
1. 析构函数名是在类名前加上字符 ~。
2. 无参数无返回值。
3. 一个类有且只有一个析构函数。若未显式定义,系统会自动生成默认的析构函数。
4. 对象生命周期结束时,C++编译系统系统自动调用析构函数。
#include<iostream>
#include<cassert>
using namespace std;
typedef int DataType;
class SeqList
{
public:
SeqList(int capacity = 10)
{
_pData = (DataType*)malloc(capacity * sizeof(DataType));
assert(_pData);
_size = 0;
_capacity = capacity;
}
~SeqList()
{
if (_pData)
{
free(_pData); // 释放堆上的空间
_pData = NULL; // 将指针置为空
_capacity = 0;
_size = 0;
}
}
private:
int* _pData;
size_t _size;
size_t _capacity;
};
int main()
{
SeqList q;
return 0;
}
四、 拷贝构造函数
构造函数
:
只有单个形参
,该形参是对本
类类型对象的引用
(
一般常用
const
修饰
)
,在用已存在的类类型对象创建新对象时由编译器自动调用。
特征
拷贝构造函数也是特殊的成员函数,其特征如下:
1. 拷贝构造函数是构造函数的一个重载形式。
2. 拷贝构造函数的参数只有一个且必须使用引用传参,使用传值方式会引发无穷递归调用。
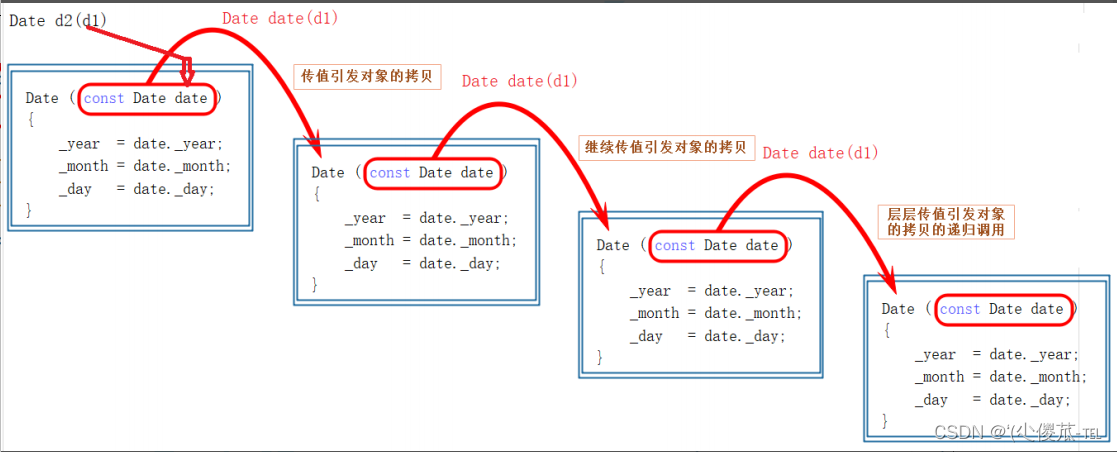
3. 若未显示定义,系统生成默认的拷贝构造函数。
默认的拷贝构造函数对象按内存存储按字节序完成拷
贝,这种拷贝我们叫做浅拷贝,或者值拷贝。
#include<iostream>
using namespace std;
class Date
{
public:
Date(int year = 1, int month = 1, int day = 1) {
_year = year;
_month = month;
_day = day;
}
Date(Date& d) {
_year = d._year;
_month = d._month;
_year = d._day;
}
private:
int _year = 1;
int _month = 1;
int _day = 1;
};
int main()
{
Date d1(2023, 3, 6);
Date d2(d1);
return 0;
}
五、赋值运算符重载
运算符重载
C++为了增强代码的可读性引入了运算符重载,运算符重载是具有特殊函数名的函数,也具有其返回值类型,函数名字以及参数列表,其返回值类型与参数列表与普通的函数类似。
函数名字为:关键字operator后面接需要重载的运算符符号。
函数原型:返回值类型 operator操作符(参数列表)
注意:
1、不能通过连接其他符号来创建新的操作符:比如operator@
2、重载操作符必须有一个类类型或者枚举类型的操作数。
3、用于内置类型的操作符,其含义不能改变,例如:内置的整型+,不 能改变其含义。
4、作为类成员的重载函数时,其形参看起来比操作数数目少1成员函数的操作符有一个默认的形参this,限定为第一个形参。
5、.* 、:: 、sizeof 、?: 、. 注意以上5个运算符不能重载
赋值运算符重载
赋值运算符主要有四点:
1. 参数类型
2. 返回值
3. 检测是否自己给自己赋值
4. 返回*this
5. 一个类如果没有显式定义赋值运算符重载,编译器也会生成一个,完成对象按字节序的值拷贝。
//#include<iostream>
//
//using namespace std;
//
//class A
//{
//public :
// A(int a = 0) {
// cout << "A(int a=0)->" << _a << endl;
// }
// ~A()
// {
// cout << "~A()->" << _a << endl;
// }
//
//private:
// int _a;
//};
//int main()
//{
// A aa1(1);
// A aa2(aa1);
//
//
// return 0;
//}
#include<iostream>
using namespace std;
class Date {
public:
int GetMonthDay(int year, int month) {
static int days[13] = { 0,31,28,31,30,31,30,31,31,30,31,30,31 };
int day = days[month];
if (month == 2 && ((year % 4 == 0 && year % 100 != 0) || (year % 400 == 0))) {
day += 1;
}
return day;
}
//构造函数被频繁调用,所以直接放在类里面定义作为inline
Date(int year = 1900, int month = 1, int day = 1) {
_year = year;
_month = month;
_day = day;
}
Date(const Date& d) {
_year = d._year;
_month = d._month;
_year = d._day;
}
Date& operator=(const Date& d) {
if (this != &d) {
_year = d._year;
_month = d._month;
_day = d._day;
return *this;
}
}
bool operator==(const Date& d) {
return _year == d._year && _month == d._month && _day == d._day;
}
bool operator!=(const Date& d) {
return !(*this == d);
}
bool operator>(const Date& d) {
if ((_year > d._year) || (_year == d._year && _month > d._month) || (_year == d._year && _month == d._month && _day == d._day))
return true;
else
return false;
}
bool operator>=(const Date& d) {
return (*this > d) || (*this == d);
}
bool operator<(const Date& d) {
return !(*this >= d);
}
bool operator<=(const Date& d) {
return !(*this > d);
}
Date operator+(int day) {
Date ret = *this;
ret += day;
return ret;
}
Date& operator+=(int day) {
if (day < 0) {
return *this -= -day;
}
_day += day;
while (_day > GetMonthDay(_year, _month)) {
_day -= GetMonthDay(_year, _month);
++_month;
if (_month == 13) {
_year++;
_month = 1;
}
}
return *this;
}
//根据函数重载来区分前置++和后置++
//不加参数为前置++
Date& operator++() {
*this += 1;
return *this;
}
Date operator++(int day) {
Date tmp(*this);
*this += 1;
return tmp;
}
Date operator-(int day) {
Date ret = *this;
ret -= day;
return ret;
}
Date& operator-=(int day) {
if (day < 0) {
return *this += -day;
}
_day -= day;
while (_day <= 0) {
--_month;
if (_month == 0) {
--_year;
_month = 12;
}
_day += GetMonthDay(_year, _month);
}
return *this;
}
Date& operator--() {
return *this -= 1;
}
Date operator--(int) {
Date tmp(*this);
*this -= 1;
return tmp;
}
int operator-(const Date& d) {
Date max = *this;
Date min = d;
int flag = 1;
if (*this < d) {
max = d;
min = *this;
flag = -1;
}
int n = 0;
while (min != max) {
++min;
++n;
}
return n * flag;
}
void Print()
{
cout << _year << "/" << _month << "/" << _day << endl;
}
friend ostream& operator<<(ostream& out, const Date& d);
friend istream& operator>>(istream& in, const Date& d);
private:
int _year;
int _month;
int _day;
};
inline ostream& operator<<(ostream& out,const Date& d)
{
out << d._year << "年" << d._month << "月" << d._day << "日" << endl;
return out;
}
inline istream& operator>>(istream& in, const Date& d)
{
in >> d._year >> d._month >> d._day;
return in;
}
void TestDate1()
{
Date d1(2022, 7, 24);
d1 += 4;
d1.Print();
d1 += 40;
d1.Print();
d1 += 400;
d1.Print();
++d1;
d1.Print();
d1++;
d1.Print();
}
int main()
{
TestDate1();
return 0;
}
六、const成员函数
const
修饰类的成员函数
将const修饰的类成员函数称之为const成员函数,const修饰类成员函数,实际修饰该成员函数隐含的this指针,表明在该成员函数中不能对类的任何成员进行修改。
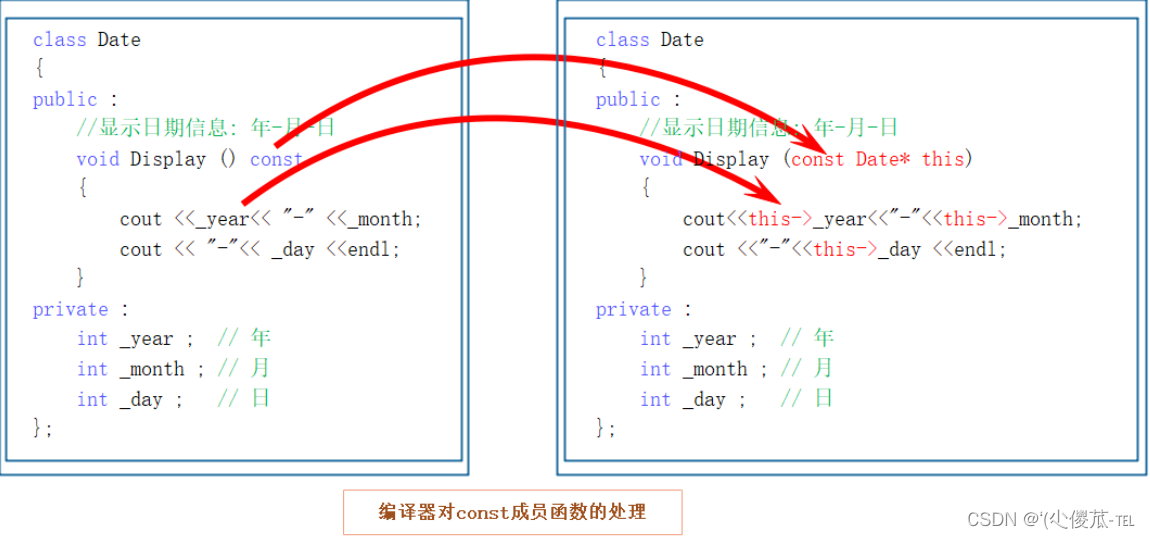
#include<iostream>
using namespace std;
class Date
{
public:
Date(int year = 1900, int month = 1, int day = 1)
{
_year = year;
_month = month;
_day = day;
}
void Display()
{
cout << "Display ()" << endl;
cout << "year:" << _year << endl;
cout << "month:" << _month << endl;
cout << "day:" << _day << endl << endl;
}
void Display() const
{
cout << "Display () const" << endl;
cout << "year:" << _year << endl;
cout << "month:" << _month << endl;
cout << "day:" << _day << endl << endl;
}
private:
int _year; // 年
int _month; // 月
int _day; // 日
};
void Test()
{
Date d1(2023,2,8);
d1.Display();
const Date d2(2023,25);
d2.Display();
}
int main()
{
Test();
return 0;
}
取地址及
const
取地址操作符重载
这两个运算符一般不需要重载,使用编译器生成的默认取地址的重载即可,只有特殊情况,才需要重载,比如想让别人获取到指定的内容!
class Date
{
public:
Date* operator&()
{
return this;
}
const Date* operator&()const
{
return this;
}
private:
int _year; // 年
int _month; // 月
int _day; // 日
};