八、按钮组QButton
8.1 命令按钮QPushButton
按钮或命令按钮或许是任何图形用户界面中最常用的 Widget。按下 (点击) 按钮以命令计算机履行某些动作或回答问题。典型按钮有 OK、申请、取消、关闭、是、否 及 帮助。
命令按钮为矩形且通常显示描述其动作的文本标签。可以通过在文本首选字符前面加上 & 和号来指定快捷键。
我们这次创建QMainWindow工程
mainwindow.h 头文件
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include<QPushButton>
QT_BEGIN_NAMESPACE
namespace Ui { class MainWindow; }
QT_END_NAMESPACE
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr);
~MainWindow();
private:
Ui::MainWindow *ui;
QPushButton *pb1,*pb2;
private slots:
void pushbutton1_clicked();
void pushbutton2_clicked();
};
#endif // MAINWINDOW_H
我们先引入QPushButton头文件,然后定义两个QPushButton对象,既然要用按钮,当然得需要信号与槽,所以我们定义两个命令按钮槽函数。
mainwindow.cpp
首先我们先设置窗口位置
this->setGeometry(300,150,500,300);
起初的窗口位置是
设置后的位置是
void QWidget::setGeometry(int x, int y, int w, int h):
从窗体的(x,y)坐标开始,显示一个宽为w,长为h的控件。
然后我们实例化两个命令按钮并且设置位置
pb1=new QPushButton("命令按钮1",this);
pb2=new QPushButton("命令按钮2",this);
pb1->setGeometry(20,20,150,30);
pb2->setGeometry(20,90,150,50);
QPushButton的构造函数:
在官方文档中显示QPushButton有三个构造函数
1. QPushButton::QPushButton(const QIcon &icon, const QString &text, QWidget *parent = nullptr):构造一个带有图标、文本和父按钮的按钮。注意,您还可以将QPixmap对象作为图标传递(这要归功于c++提供的隐式类型转换)。
2. QPushButton::QPushButton(const QString &text, QWidget *parent = nullptr):用文本类text构造一个按钮。
3. QPushButton::QPushButton(QWidget *parent = nullptr):构造一个没有文本的按钮
实现槽函数
connect(pb1,SIGNAL(clicked()),this,SLOT(pushbutton1_clicked()));
connect(pb2,SIGNAL(clicked()),this,SLOT(pushbutton2_clicked()));
void MainWindow::pushbutton1_clicked()
{
this->setStyleSheet("QMainWindow {background-color:rgba(255,255,0,100%);}");
}
void MainWindow::pushbutton2_clicked()
{
this->setStyleSheet("QMainWindow {background-color:rgba(255,0,0,100%);}");
}
点击命令按钮1
点击命令按钮2
setStyleSheet的用法在第一篇哦!
但是得注意的是在用setStyleSheet的时候一定要跟上哪个窗体
比如
this->setStyleSheet("QMainWindow {background-color:rgba(255,255,0,100%);}");
这表明在QMainWindow窗体中用到背景颜色。
完整代码
#include "mainwindow.h"
#include "ui_mainwindow.h"
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
, ui(new Ui::MainWindow)
{
ui->setupUi(this);
//设置运行窗口的位置
this->setGeometry(300,150,500,300);
//实例化两个命令按钮并设置位置
pb1=new QPushButton("命令按钮1",this);
pb2=new QPushButton("命令按钮2",this);
pb1->setGeometry(20,20,150,30);
pb2->setGeometry(20,90,150,50);
connect(pb1,SIGNAL(clicked()),this,SLOT(pushbutton1_clicked()));
connect(pb2,SIGNAL(clicked()),this,SLOT(pushbutton2_clicked()));
}
MainWindow::~MainWindow()
{
delete ui;
}
void MainWindow::pushbutton1_clicked()
{
this->setStyleSheet("QMainWindow {background-color:rgba(255,255,0,100%);}");
}
void MainWindow::pushbutton2_clicked()
{
this->setStyleSheet("QMainWindow {background-color:rgba(255,0,0,100%);}");
}
8.2 工具按钮QToolButton
工具按钮是能对特定命令 (或选项) 提供快速访问的特殊按钮。
通常,工具按钮的创建是在新的 QAction 实例被创建采用 QToolBar::addAction () 或现有动作被添加到工具栏采用 QToolBar::addAction ()。 按如任何其它 Widget 的相同方式构造工具按钮,并将它们同其它小部件一起排列在布局中是可能的。
工具按钮的一种经典用法是选择工具;例如,绘图程序中的 pen 工具。这将通过使用 QToolButton 作为切换按钮 来实现 (见 setCheckable ()).
QToolButton 支持自动上升。在自动上升模式下,按钮仅当鼠标指到它时才绘制 3D 框架。特征会自动打开当按钮用于 QToolBar 。改变它采用 setAutoRaise ()。
工具按钮的图标被设为 QIcon .这样就可以为禁用状态和活动状态指定不同的像素图。禁用的像素图在按钮的功能不可用时使用。当按钮自动升起时,将显示活动的像素图,因为鼠标指针悬停在其上。
按钮外观和尺度的调节采用 setToolButtonStyle () 和 setIconSize ().当在 QMainWindow 中使用时,该按钮会自动调整到 QMainWindow 的设置(请参阅 QMainWindow::setToolButtonStyle () 和 QMainWindow : :setIconSize ())。工具按钮还可以显示箭头符号,而不是图标,该符号使用 arrowType 指定。
工具按钮可以在弹出菜单中提供其他选项。可以使用 setMenu () 设置弹出菜单。使用 setPopupMode () 配置可用于具有菜单集的工具按钮的不同模式。默认模式是延迟弹出模式,有时与Web浏览器中的“后退”按钮一起使用。按住按钮一段时间后,会弹出一个菜单,显示可能跳转到的页面列表。超时取决于样式,请参阅 QStyle::SH_ToolButton_PopupDelay 。
我们这次创建QMainWindow工程
mainwindow.h
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include<QToolBar>
#include<QToolButton>
#include<QStyle>
#include<QApplication>
#include<QIcon>
QT_BEGIN_NAMESPACE
namespace Ui { class MainWindow; }
QT_END_NAMESPACE
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr);
~MainWindow();
private:
Ui::MainWindow *ui;
QToolBar *tbr;
QToolButton *tbn;
};
#endif // MAINWINDOW_H
mainwindow.cpp
我们先创建QToolBar对象,并设置位置大小显示在窗口。
this->setGeometry(300,150,500,300);
tbr=new QToolBar(this);
tbr->setGeometry(20,20,200,50);
我们可以看到出现一个面板。
既然我们用到工具按钮,那我们应该先设置一下按钮的图标。
QStyle *s=QApplication::style();
QIcon i=s->standardIcon(QStyle::SP_TitleBarContextHelpButton);
QStyle *QApplication::style():
返回应用程序的样式对象。
QIcon QStyle::standardIcon(QStyle::StandardPixmap standardIcon, const QStyleOption *option = ..., const QWidget *widget = ...) const :
返回给定标准图标的图标。
标准图标是一个标准的像素图,它可以遵循一些现有的GUI风格或指导方针。option参数可用于传递定义适当图标时所需的额外信息。widget参数是可选的,也可用于帮助确定图标。
enum QStyle::StandardPixmap:这个枚举描述可用的标准像素图。标准像素图是可以遵循某些现有GUI风格或指导方针的像素图。(也就是系统自带的)
常量 | 值 | 描述 |
---|---|---|
QStyle::SP_TitleBarMinButton | 1 | 最小化标题栏上的按钮(例如,在QMdiSubWindow)。 |
QStyle::SP_TitleBarMenuButton | 0 | 标题栏上的菜单按钮。 |
QStyle::SP_TitleBarMaxButton | 2 | 标题栏上的最大化按钮。 |
QStyle::SP_TitleBarCloseButton | 3 | 标题栏上的关闭按钮。 |
QStyle::SP_TitleBarNormalButton | 4 | 标题栏上的正常(恢复)按钮。 |
QStyle::SP_TitleBarShadeButton | 5 | 标题栏上的阴影按钮。 |
QStyle::SP_TitleBarUnshadeButton | 6 | 取消标题栏上的阴影按钮。 |
QStyle::SP_TitleBarContextHelpButton | 7 | 标题栏上的上下文帮助按钮。 |
QStyle::SP_MessageBoxInformation | 9 | “信息”图标。 |
QStyle::SP_MessageBoxWarning | 10 | “警告”图标。 |
QStyle::SP_MessageBoxCritical | 11 | “关键”图标 |
QStyle::SP_MessageBoxQuestion | 12 | “问题”图标。 |
QStyle::SP_DesktopIcon | 13 | 请‘’打开桌面‘’上的图标 |
QStyle::SP_TrashIcon | 14 | “垃圾”图标。 |
QStyle::SP_ComputerIcon | 15 | “我的电脑”图标。 |
QStyle::SP_DriveFDIcon | 16 | 软盘图标。 |
QStyle::SP_DriveHDIcon | 17 | 硬盘图标 |
QStyle::SP_DriveCDIcon | 18 | CD图标。 |
QStyle::SP_DriveDVDIcon | 19 | DVD图标。 |
QStyle::SP_DriveNetIcon | 20 | 网络图标。 |
QStyle::SP_DirHomeIcon | 55 | 主目录图标。 |
QStyle::SP_DirOpenIcon | 21 | 打开目录图标。 |
QStyle::SP_DirClosedIcon | 22 | 已关闭的目录图标。 |
QStyle::SP_DirIcon | 37 | 目录图标。 |
QStyle::SP_DirLinkIcon | 23 | 链接到目录图标。 |
QStyle::SP_FileIcon | 24 | The file icon. |
QStyle::SP_FileLinkIcon | 25 | The link to file icon. |
QStyle::SP_FileDialogStart | 28 | The "start" icon in a file dialog. |
QStyle::SP_FileDialogEnd | 29 | The "end" icon in a file dialog. |
QStyle::SP_FileDialogToParent | 30 | The "parent directory" icon in a file dialog. |
QStyle::SP_FileDialogNewFolder | 31 | The "create new folder" icon in a file dialog. |
QStyle::SP_FileDialogDetailedView | 32 | The detailed view icon in a file dialog. |
QStyle::SP_FileDialogInfoView | 33 | The file info icon in a file dialog. |
QStyle::SP_FileDialogContentsView | 34 | The contents view icon in a file dialog. |
QStyle::SP_FileDialogListView | 35 | The list view icon in a file dialog. |
QStyle::SP_FileDialogBack | 36 | The back arrow in a file dialog. |
QStyle::SP_DockWidgetCloseButton | 8 | Close button on dock windows (see also QDockWidget). |
QStyle::SP_ToolBarHorizontalExtensionButton | 26 | Extension button for horizontal toolbars. |
QStyle::SP_ToolBarVerticalExtensionButton | 27 | Extension button for vertical toolbars. |
QStyle::SP_DialogOkButton | 38 | Icon for a standard OK button in a QDialogButtonBox. |
QStyle::SP_DialogCancelButton | 39 | Icon for a standard Cancel button in a QDialogButtonBox. |
QStyle::SP_DialogHelpButton | 40 | Icon for a standard Help button in a QDialogButtonBox. |
QStyle::SP_DialogOpenButton | 41 | Icon for a standard Open button in a QDialogButtonBox. |
QStyle::SP_DialogSaveButton | 42 | Icon for a standard Save button in a QDialogButtonBox. |
QStyle::SP_DialogCloseButton | 43 | Icon for a standard Close button in a QDialogButtonBox. |
QStyle::SP_DialogApplyButton | 44 | Icon for a standard Apply button in a QDialogButtonBox. |
QStyle::SP_DialogResetButton | 45 | Icon for a standard Reset button in a QDialogButtonBox. |
QStyle::SP_DialogDiscardButton | 46 | Icon for a standard Discard button in a QDialogButtonBox. |
QStyle::SP_DialogYesButton | 47 | Icon for a standard Yes button in a QDialogButtonBox. |
QStyle::SP_DialogNoButton | 48 | Icon for a standard No button in a QDialogButtonBox. |
QStyle::SP_ArrowUp | 49 | Icon arrow pointing up. |
QStyle::SP_ArrowDown | 50 | Icon arrow pointing down. |
QStyle::SP_ArrowLeft | 51 | Icon arrow pointing left. |
QStyle::SP_ArrowRight | 52 | Icon arrow pointing right. |
QStyle::SP_ArrowBack | 53 | Equivalent to SP_ArrowLeft when the current layout direction isQt::LeftToRight, otherwise SP_ArrowRight. |
QStyle::SP_ArrowForward | 54 | Equivalent to SP_ArrowRight when the current layout direction isQt::LeftToRight, otherwise SP_ArrowLeft. |
QStyle::SP_CommandLink | 56 | Icon used to indicate a Vista style command link glyph. |
QStyle::SP_VistaShield | 57 | Icon used to indicate UAC prompts on Windows Vista. This will return a null pixmap or icon on all other platforms. |
QStyle::SP_BrowserReload | 58 | Icon indicating that the current page should be reloaded. |
QStyle::SP_BrowserStop | 59 | Icon indicating that the page loading should stop. |
QStyle::SP_MediaPlay | 60 | Icon indicating that media should begin playback. |
QStyle::SP_MediaStop | 61 | Icon indicating that media should stop playback. |
QStyle::SP_MediaPause | 62 | Icon indicating that media should pause playback. |
QStyle::SP_MediaSkipForward | 63 | Icon indicating that media should skip forward. |
QStyle::SP_MediaSkipBackward | 64 | Icon indicating that media should skip backward. |
QStyle::SP_MediaSeekForward | 65 | Icon indicating that media should seek forward. |
QStyle::SP_MediaSeekBackward | 66 | Icon indicating that media should seek backward. |
QStyle::SP_MediaVolume | 67 | Icon indicating a volume control. |
QStyle::SP_MediaVolumeMuted | 68 | Icon indicating a muted volume control. |
QStyle::SP_CustomBase | 0xf0000000 | Base value for custom standard pixmaps; custom values must be greater than this value. |
实例化QToolButton对象,并且修改tbn的图标,设置tbn的样式,最后把它添加到tbr面板里。
tbn=new QToolButton();
tbn->setIcon(i);
tbn->setText("系统帮助提示");
//调用setToolButtonStyle函数设置tbn样式,设置文本在图标下方
tbn->setToolButtonStyle(Qt::ToolButtonTextUnderIcon);
tbr->addWidget(tbn);
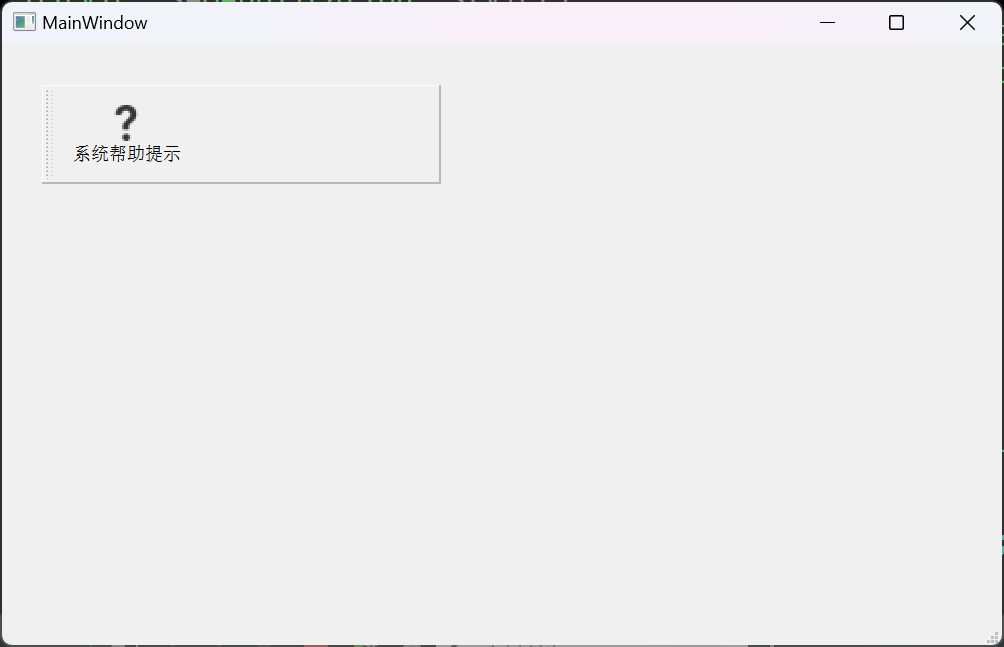
void setToolButtonStyle(Qt::ToolButtonStyle style)来源于Qt::ToolButtonStyle
此属性用于确定工具按钮是仅显示图标、仅显示文本还是仅显示图标旁边/下方的文本。
默认为Qt::ToolButtonIconOnly。
要使工具按钮的样式遵循系统设置,请将此属性设置为Qt::ToolButtonFollowStyle。在Unix上,将使用桌面环境中的用户设置。在其他平台上,Qt::ToolButtonFollowStyle仅表示图标。
QToolButton自动将此槽连接到其所在的QMainWindow中的相关信号。
ToolButtonStyle,Qt为我们准备了五种:
常量 | 值 | 描述 |
---|---|---|
Qt::ToolButtonIconOnly | 0 | 只显示图标。 |
Qt::ToolButtonTextOnly | 1 | 只显示文本。 |
Qt::ToolButtonTextBesideIcon | 2 | 文本将出现在图标旁边。 |
Qt::ToolButtonTextUnderIcon | 3 | 文本出现在图标下方。 |
Qt::ToolButtonFollowStyle | 4 | 请看 style |
最后一个style,也就是enum QStyle::StyleHint:此enum描述可用的样式提示。样式提示是一般的外观和/或感觉提示。具体看官方文档。
完整代码
#include "mainwindow.h"
#include "ui_mainwindow.h"
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
, ui(new Ui::MainWindow)
{
ui->setupUi(this);
this->setGeometry(300,150,500,300);
tbr=new QToolBar(this);
tbr->setGeometry(20,20,200,50);
//主要目的设置风格,图标是系统自带
QStyle *s=QApplication::style();
QIcon i=s->standardIcon(QStyle::SP_TitleBarContextHelpButton);
tbn=new QToolButton();
tbn->setIcon(i);
tbn->setText("系统帮助提示");
//调用setToolButtonStyle函数设置tbn样式,设置文本在图标下方
tbn->setToolButtonStyle(Qt::ToolButtonTextUnderIcon);
tbr->addWidget(tbn);
}
MainWindow::~MainWindow()
{
delete ui;
}
8.3 单选按钮QRadioButton
QRadioButton 是可以切换为开 (复选) 或关 (取消复选) 的选项按钮。 单选按钮通常按多选一呈现给用户。 在一组单选按钮中,每次仅一单选按钮可被复选; 若用户选择另一按钮,先前选中按钮切换为关。
默认情况下,单选按钮是autoExclusive。如果启用了自动独占,则属于同一父构件的单选按钮的行为就像它们是同一独占按钮组的一部分一样。如果属于同一父小部件的单选按钮需要多个独占按钮组,请将它们放入 QButtonGroup 中。
每当被切换为开 (或关) 时,按钮发射 toggled () 信号。 连接到此信号,若想要在按钮每次改变状态时触发动作。 使用 isChecked () 能查看是否选中特定按钮。
就像 QPushButton ,单选按钮显示文本和可选小图标。 图标的设置采用 setIcon ()。 文本的设置可以在构造函数中或采用 setText ()。 通过在文本首选字符之前加 & 号可以指定快捷键。
我们继续创建QMainWindow工程
mainwindow.h
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include<QRadioButton>
QT_BEGIN_NAMESPACE
namespace Ui { class MainWindow; }
QT_END_NAMESPACE
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr);
~MainWindow();
private:
Ui::MainWindow *ui;
QRadioButton *rb1;
QRadioButton *rb2;
};
#endif // MAINWINDOW_H
实例化两个QRadioButton对象,并设置位置
rb1=new QRadioButton("按钮1",this);
rb2=new QRadioButton("按钮2",this);
rb1->setGeometry(20,20,150,40);
rb2->setGeometry(20,80,150,40);
设置单选按钮默认值
rb1->setChecked(true);
rb2->setChecked(false);
void setChecked(bool):
此属性保存按钮是否被选中
只有可检查按钮可以被选中。缺省情况下,该按钮处于未选中状态。
完整代码
#include "mainwindow.h"
#include "ui_mainwindow.h"
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
, ui(new Ui::MainWindow)
{
ui->setupUi(this);
this->setGeometry(300,150,500,300);
this->setStyleSheet("QMainWindow {background-color:rgba(255,255,0,100%);}");
rb1=new QRadioButton("按钮1",this);
rb2=new QRadioButton("按钮2",this);
rb1->setGeometry(20,20,150,40);
rb2->setGeometry(20,80,150,40);
rb1->setChecked(true);
rb2->setChecked(false);
}
MainWindow::~MainWindow()
{
delete ui;
}
8.4 复选框QCheckBox
QCheckBox 是可以被切换为开 (复选) 或切换为关 (取消复选) 的选项按钮。复选框通常用于表示可以被启用或禁用的 (不影响其它) 应用程序特征。可以实现不同行为类型。例如, QButtonGroup 可以用于逻辑分组复选按钮,允许独占复选框。不管怎样, QButtonGroup 不提供任何视觉表示。
以下图像进一步阐明独占复选框和非独占复选框之间的差异。
每当复选框被复选或清零时,它发射信号 stateChanged ()。连接到此信号,若想要在复选框每次改变状态时触发动作。可以使用 isChecked () 查询复选框是否被复选。
除通常的被复选和取消复选状态外,QCheckBox 还可选提供第 3 种无变化状态指示。每当需要赋予用户既不复选也不取消复选的复选框选项时,这很有用。若需要这种第 3 状态,启用它采用 setTristate (),和使用 checkState () 查询当前触发状态。
就像 QPushButton ,复选框显示文本,及可选小图标。图标的设置是采用 setIcon ()。文本的设置可以在构造函数中或采用 setText ()。通过在首选字符之前加 & 号可以指定快捷键。
首先创建一个QMainWindow工程。
mainwindow.h
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include<QCheckBox>
QT_BEGIN_NAMESPACE
namespace Ui { class MainWindow; }
QT_END_NAMESPACE
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr);
~MainWindow();
private:
Ui::MainWindow *ui;
QCheckBox *cb;
private slots:
void checkboxstate(int state);
};
#endif // MAINWINDOW_H
mainwindow.cpp
设置任意窗口背景颜色,然后实例化cb对象并设置位置。
this->setGeometry(400,300,500,300);
this->setStyleSheet("QMainWindow {background-color:rgba(255,100,0,100%);}");
cb=new QCheckBox(this);
cb->setGeometry(30,50,250,50);
可以发现出现一个小方框,这个小方块点击是一个对钩
初始化三态状态,默认是Checked状态
cb->setCheckState(Qt::Checked);
cb->setText("初始状态:Checked状态");
cb->setTristate();
选中状态:Checked
未选中状态:Unchecked
半选中状态:PartiallyChecked
void QCheckBox::setCheckState(Qt::CheckState state):
将复选框的复选状态设置为state。如果不需要三状态支持,还可以使用QAbstractButton::setChecked(),它接受一个布尔值。
void setTristate(bool y = true):
此属性保留复选框是否为三状态复选框,默认值为false,即复选框只有两种状态。
如果要开启三态状态,这个函数是必须有的。
接下来实现槽函数,使得选中三种状态是改变复选框的标题。
connect(cb,SIGNAL(stateChanged(int)),this,SLOT(checkboxstate(int)));
void MainWindow::checkboxstate(int state)
{
switch(state)
{
case Qt::Checked:
cb->setText("Checked");
break;
case Qt::Unchecked:
cb->setText("Unchecked");
break;
case Qt::PartiallyChecked:
cb->setText("PartiallyChecked");
break;
default:
break;
}
}
对于enum Qt::CheckState:此枚举描述可检查项、控件和小部件的状态。
常量 | 值 | 描述 |
---|---|---|
Qt::Unchecked | 0 | 该项目未被选中 |
Qt::PartiallyChecked | 1 | 项目被部分检查。如果检查了层次模型中的一些(但不是全部)子项,则可以对其进行部分检查 |
Qt::Checked | 2 | 项目被选中。 |
stateChange是状态改变信号,该信号一旦触发,就会检测到QCheckBox的状态,如果状态改变,就把该状态的值传给槽函数。
完整代码
#include "mainwindow.h"
#include "ui_mainwindow.h"
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
, ui(new Ui::MainWindow)
{
ui->setupUi(this);
this->setGeometry(400,300,500,300);
this->setStyleSheet("QMainWindow {background-color:rgba(255,100,0,100%);}");
cb=new QCheckBox(this);
cb->setGeometry(30,50,250,50);
//初始化三态状态
cb->setCheckState(Qt::Checked);
cb->setText("初始状态:Checked状态");
cb->setTristate();
connect(cb,SIGNAL(stateChanged(int)),this,SLOT(checkboxstate(int)));
}
MainWindow::~MainWindow()
{
delete ui;
}
void MainWindow::checkboxstate(int state)
{
switch(state)
{
case Qt::Checked:
cb->setText("Checked");
break;
case Qt::Unchecked:
cb->setText("Unchecked");
break;
case Qt::PartiallyChecked:
cb->setText("PartiallyChecked");
break;
default:
break;
}
}
8.5 命令链接按钮QCommandLinkButton
命令链接是由 Windows Vista 引入的新控件。它的预期用途类似于单选按钮的用途,因为它用于在一组互斥选项之间进行选择。命令链接按钮不应单独使用,而应用作向导和对话框中单选按钮的替代方法,并使按下“下一步”按钮变得多余。外观通常类似于平面按钮的外观,但它除了允许使用普通按钮文本外,还允许使用描述性文本。默认情况下,它还将带有一个箭头图标,指示按控件将打开另一个窗口或页面。
mainwindow.h
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include<QCommandLinkButton>
#include<QDesktopServices> //引入桌面服务
#include<QUrl>
QT_BEGIN_NAMESPACE
namespace Ui { class MainWindow; }
QT_END_NAMESPACE
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr);
~MainWindow();
private:
Ui::MainWindow *ui;
QCommandLinkButton *clb;
private slots:
void clbcliked();
};
#endif // MAINWINDOW_H
mainwindow.cpp
首先创建QCommandLinkButton对象并设置位置。
this->setGeometry(400,300,500,300);
this->setStyleSheet("QMainWindow {background-color:rgba(255,100,0,100%);}");
clb=new QCommandLinkButton("test01","test02",this);
clb->setGeometry(50,100,250,60);
我们来看一下它的构造函数
接下来实现点击按钮后自动打开百度。
connect(clb,SIGNAL(clicked()),this,SLOT(clbcliked()));
void MainWindow::clbcliked()
{
QDesktopServices::openUrl(QUrl("www.baidu.com"));
}
我们进行点击
就打开百度了。
bool QDesktopServices::openUrl(const QUrl &url):
在用户桌面环境的适当Web浏览器中打开给定的url,如果成功则返回true;否则返回false。
如果URL是对本地文件的引用(也就是说,URL方案是“file”),那么它将用合适的应用程序而不是Web浏览器打开。
这个我们先初步了解即可。
完整代码
#include "mainwindow.h"
#include "ui_mainwindow.h"
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
, ui(new Ui::MainWindow)
{
ui->setupUi(this);
this->setGeometry(400,300,500,300);
this->setStyleSheet("QMainWindow {background-color:rgba(255,100,0,100%);}");
clb=new QCommandLinkButton("test01","test02",this);
clb->setGeometry(50,100,250,60);
connect(clb,SIGNAL(clicked()),this,SLOT(clbcliked()));
}
MainWindow::~MainWindow()
{
delete ui;
}
void MainWindow::clbcliked()
{
QDesktopServices::openUrl(QUrl("www.baidu.com"));
}
8.6 按钮盒QDialogButtonBox
对话框和消息框通常在符合该平台界面指导方针的布局中,呈现按钮。始终不变,不同平台的对话框拥有不同布局。QDialogButtonBox 允许开发者向其添加按钮,并将自动为用户桌面环境使用适当布局。
大多数对话框按钮遵循某些角色。这些角色包括:
- 接受或拒绝对话框。
- 寻求帮助。
- 在对话框自身上履行动作 (譬如:重置字段或应用更改)。
还可以使用其他方法关闭对话框,这可能会导致破坏性结果。
大多数对话框都有几乎可以被认为是标准的按钮(例如“确定”和“取消 ”按钮)。有时以标准方式创建这些按钮很方便。
mainwindow.h
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include<QPushButton>
#include<QDebug>
#include<QDialogButtonBox>
QT_BEGIN_NAMESPACE
namespace Ui { class MainWindow; }
QT_END_NAMESPACE
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr);
~MainWindow();
private:
Ui::MainWindow *ui;
QDialogButtonBox *dbb;
QPushButton *pb;
private slots:
void dbbclicked(QAbstractButton *);
};
#endif // MAINWINDOW_H
mainwindow.cpp
实例化QDialogButtonBox对象,并创建一个取消按钮。
this->setGeometry(0,0,800,600);
dbb=new QDialogButtonBox(this);
dbb->setGeometry(300,200,200,30);
dbb->addButton(QDialogButtonBox::Cancel);
dbb->button(QDialogButtonBox::Cancel)->setText("取 消");
*QDialogButtonBox::button(QDialogButtonBox::StandardButton which) const:
返回与标准按钮对应的QPushButton,如果此按钮框中不存在标准按钮,则返回0。
void QDialogButtonBox::addButton(QAbstractButton *button, QDialogButtonBox::ButtonRole role(角色)):
将给定 按钮添加到具有指定角色 的按钮框中。如果角色无效,则不会添加该按钮。
如果已添加该按钮,则会将其删除,然后使用新角色再次添加。
注意: 按钮框取得按钮的所有权。
另请参阅 removeButton () 和 clear ()。
QPushButton *QDialogButtonBox:: addButton (const QString & text , QDialogButtonBox::ButtonRole role role ):
使用给定的文本 创建一个按钮,将其添加到指定角色 的按钮框中,并返回相应的按钮。如果角色无效,则不会创建任何按钮,并返回零。
另请参阅 removeButton () 和 clear ()。
QPushButton *QDialogButtonBox:: addButton (QDialogButtonBox::StandardButton button ):
将标准按钮 添加到按钮框中(如果有效),并返回一个按钮。如果按钮无效,则不会将其添加到按钮框中,并返回零。
另请参阅 removeButton () 和 clear ()。
对于QDialogButtonBox::ButtonRole的值有:
对于QDialogButtonBox::StandardButton的值有:
实现槽函数
connect(dbb,SIGNAL(clicked(QAbstractButton*)),this,SLOT(dbbclicked(QAbstractButton*)));
void MainWindow::dbbclicked(QAbstractButton *bt)
{
if(bt==dbb->button(QDialogButtonBox::Cancel))
{
qDebug()<<"取消"<<endl;
}
else if(bt==pb)
{
qDebug()<<"自定义"<<endl;
}
}
完整代码
#include "mainwindow.h"
#include "ui_mainwindow.h"
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
, ui(new Ui::MainWindow)
{
ui->setupUi(this);
this->setGeometry(0,0,800,600);
dbb=new QDialogButtonBox(this);
dbb->setGeometry(300,200,200,30);
dbb->addButton(QDialogButtonBox::Cancel);
dbb->button(QDialogButtonBox::Cancel)->setText("取 消");
pb=new QPushButton("自定义");
dbb->addButton(pb,QDialogButtonBox::ActionRole);
connect(dbb,SIGNAL(clicked(QAbstractButton*)),this,SLOT(dbbclicked(QAbstractButton*)));
}
MainWindow::~MainWindow()
{
delete ui;
}
void MainWindow::dbbclicked(QAbstractButton *bt)
{
if(bt==dbb->button(QDialogButtonBox::Cancel))
{
qDebug()<<"取消"<<endl;
}
else if(bt==pb)
{
qDebug()<<"自定义"<<endl;
}
}