IO流小记
流的分类(要求掌握
)
- 按操作
数据单位
不同分为:(byte)字节流(8 bit),(char)字符流(16 bit) - 按数据流的
流向
不同分为:输入流,输出流 - 按流的
角色
的不同分为:节点流,处理流
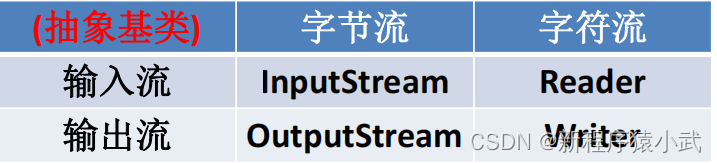
- 理解
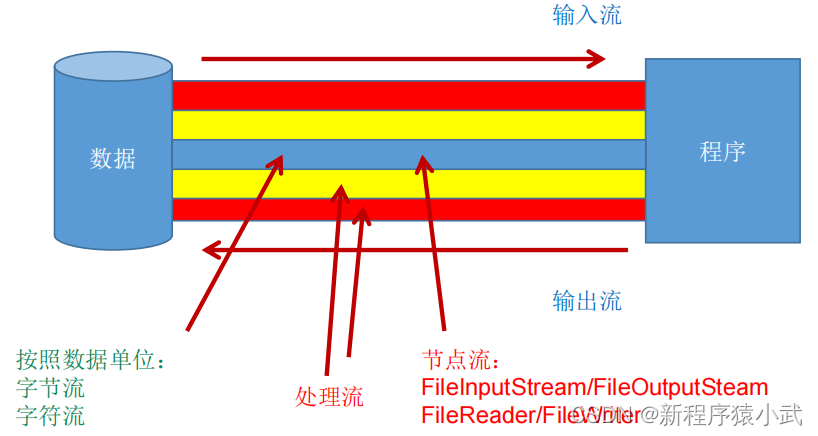
IO流体系
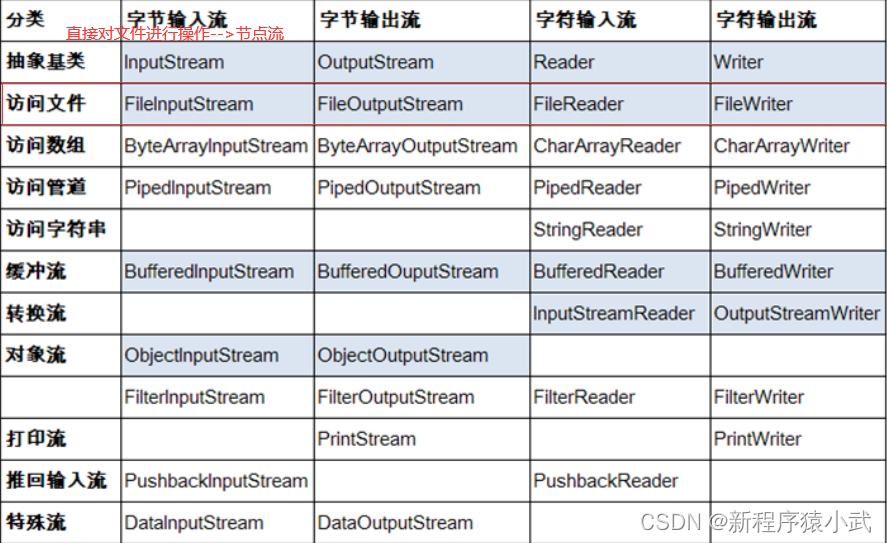
具体代码应用
FileReader
@Test
public void testFileReader() {
FileReader fr = null;
try {
File file = new File("hello.txt");
fr = new FileReader(file);
int data;
while ((data = fr.read()) != -1) {
System.out.print((char) data);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (fr != null) {
fr.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
@Test
public void testFileReader1() {
FileReader fr = null;
try {
File file = new File("hello.txt");
fr = new FileReader(file);
char[] charbuffer = new char[5];
int len;
while ((len = fr.read(charbuffer)) != -1) {
for (int i = 0; i < len; i++) {
System.out.print(charbuffer[i]);
}
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
FileWriter
@Test
public void testFileWriter() {
FileWriter fw = null;
try {
File file = new File("hello1.txt");
fw = new FileWriter(file);
fw.write("I am Iron Man~\n");
} catch (Exception e) {
e.printStackTrace();
}finally{
try {
if (fw != null) {
fw.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
FileInputStream与FileOutputStream
- 字节流操作字节,比如:.mp3,.avi,.rmvb,mp4,.jpg,.doc,.ppt
- 字符流操作字符,只能操作普通文本文件。最常见的文本文
件:.txt,.java,.c,.cpp 等语言的源代码。尤其注意.doc,excel,ppt这些不是本文件
。
@Test
public void testFileInputStreamAndFileOutputStream(){
FileInputStream fi = null;
FileOutputStream fo = null;
try {
File file1 = new File("梦想相册.png");
File file2 = new File("梦想相册1.png");
fi = new FileInputStream(file1 );
fo = new FileOutputStream(file2);
byte[] bbuff = new byte[1024];
int len;
while ((len = fi.read(bbuff)) != -1) {
fo.write(bbuff, 0, len);
}
} catch (Exception e) {
e.printStackTrace();
}finally{
if(fo != null){
try {
fo.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(fi != null){
try {
fi.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
处理流之一:缓冲流的使用
@Test
public void BufferedTest(){
FileInputStream fi = null;
FileOutputStream fo = null;
BufferedInputStream bis = null;
BufferedOutputStream bos = null;
try {
File file1 = new File("梦想相册.png");
File file2 = new File("梦想相册1.png");
fi = new FileInputStream(file1);
fo = new FileOutputStream(file2);
bis = new BufferedInputStream(fi);
bos = new BufferedOutputStream(fo);
byte[] bbuff = new byte[1024];
int len;
while ((len = bis.read(bbuff)) != -1) {
bos.write(bbuff, 0, len);
}
} catch (Exception e) {
e.printStackTrace();
}finally{
if(bos != null){
try {
bos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(bis != null){
try {
bis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void test(){
BufferedReader br = null;
BufferedWriter bw = null;
try {
br = new BufferedReader(new FileReader(new File("hello.txt")));
bw = new BufferedWriter(new FileWriter(new File("hello3.txt")));
char[] cbuf = new char[1024];
int len;
while ((len = br.read(cbuf)) != -1) {
bw.write(cbuf, 0, len);
}
} catch (Exception e) {
e.printStackTrace();
}finally{
if(bw != null){
try {
bw.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(br != null){
try {
br.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}