问题描述
《炉石传说:魔兽英雄传》(Hearthstone: Heroes of Warcraft,简称炉石传说)是暴雪娱乐开发的一款集换式卡牌游戏(如下图所示)。游戏在一个战斗棋盘上进行,由两名玩家轮流进行操作,本题所使用的炉石传说游戏的简化规则如下:
* 玩家会控制一些角色,每个角色有自己的生命值和攻击力。当生命值小于等于 0 时,该角色死亡。角色分为英雄和随从。
* 玩家各控制一个英雄,游戏开始时,英雄的生命值为 30,攻击力为 0。当英雄死亡时,游戏结束,英雄未死亡的一方获胜。
* 玩家可在游戏过程中召唤随从。棋盘上每方都有 7 个可用于放置随从的空位,从左到右一字排开,被称为战场。当随从死亡时,它将被从战场上移除。
* 游戏开始后,两位玩家轮流进行操作,每个玩家的连续一组操作称为一个回合。
* 每个回合中,当前玩家可进行零个或者多个以下操作:
1) 召唤随从:玩家召唤一个随从进入战场,随从具有指定的生命值和攻击力。
2) 随从攻击:玩家控制自己的某个随从攻击对手的英雄或者某个随从。
3) 结束回合:玩家声明自己的当前回合结束,游戏将进入对手的回合。该操作一定是一个回合的最后一个操作。
* 当随从攻击时,攻击方和被攻击方会同时对彼此造成等同于自己攻击力的伤害。受到伤害的角色的生命值将会减少,数值等同于受到的伤害。例如,随从 X 的生命值为 H X、攻击力为 A X,随从 Y 的生命值为 H Y、攻击力为 A Y,如果随从 X 攻击随从 Y,则攻击发生后随从 X 的生命值变为 H X - A Y,随从 Y 的生命值变为 H Y - A X。攻击发生后,角色的生命值可以为负数。
本题将给出一个游戏的过程,要求编写程序模拟该游戏过程并输出最后的局面。
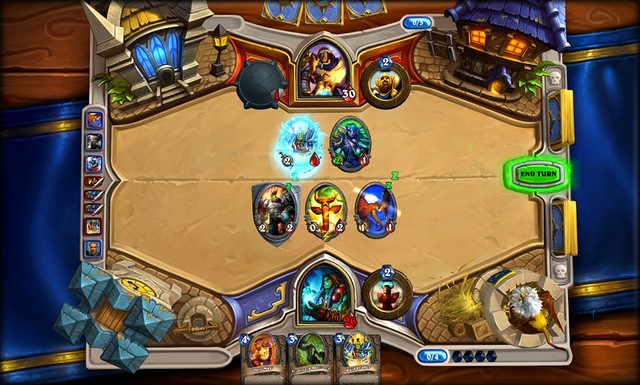
* 玩家会控制一些角色,每个角色有自己的生命值和攻击力。当生命值小于等于 0 时,该角色死亡。角色分为英雄和随从。
* 玩家各控制一个英雄,游戏开始时,英雄的生命值为 30,攻击力为 0。当英雄死亡时,游戏结束,英雄未死亡的一方获胜。
* 玩家可在游戏过程中召唤随从。棋盘上每方都有 7 个可用于放置随从的空位,从左到右一字排开,被称为战场。当随从死亡时,它将被从战场上移除。
* 游戏开始后,两位玩家轮流进行操作,每个玩家的连续一组操作称为一个回合。
* 每个回合中,当前玩家可进行零个或者多个以下操作:
1) 召唤随从:玩家召唤一个随从进入战场,随从具有指定的生命值和攻击力。
2) 随从攻击:玩家控制自己的某个随从攻击对手的英雄或者某个随从。
3) 结束回合:玩家声明自己的当前回合结束,游戏将进入对手的回合。该操作一定是一个回合的最后一个操作。
* 当随从攻击时,攻击方和被攻击方会同时对彼此造成等同于自己攻击力的伤害。受到伤害的角色的生命值将会减少,数值等同于受到的伤害。例如,随从 X 的生命值为 H X、攻击力为 A X,随从 Y 的生命值为 H Y、攻击力为 A Y,如果随从 X 攻击随从 Y,则攻击发生后随从 X 的生命值变为 H X - A Y,随从 Y 的生命值变为 H Y - A X。攻击发生后,角色的生命值可以为负数。
本题将给出一个游戏的过程,要求编写程序模拟该游戏过程并输出最后的局面。
#include<iostream>
#include<vector>
#include<cstring>
#include<string>
using namespace std;
int main(){
int total;
string s[1000];
int oper[1000][4];
memset(oper,0,sizeof(oper));
int h1=30,h2=30;
vector<vector<int> > att1,att2;
vector<int> temp(2);
int i,j,k,op;
int flag=1,die=0;
int location,size1,size2;
int atc,df;
cin>>total;
for(i=0;i<total;i++){
cin>>s[i];
if(strcmp(s[i].c_str(),"summon")==0){
oper[i][0]=1;
for(j=1;j<=3;j++){
cin>>oper[i][j];
}
}
else if(strcmp(s[i].c_str(),"attack")==0){
oper[i][0]=2;
for(j=1;j<=2;j++)
cin>>oper[i][j];
}
else{
oper[i][0]=3;
}
getchar();
}
for(int op=0;op<total;op++){//第op个操作
if(oper[op][0]==1){//summon
location=oper[op][1]-1;
if(flag==1){//player1
size1=att1.size();
if((location+1)>size1){
att1.resize(size1+1);
att1[location].resize(2);
att1[location][0]=oper[op][2];
att1[location][1]=oper[op][3];
}
else{
att1.insert(att1.begin()+location,temp);
att1[location][0]=oper[op][2];
att1[location][1]=oper[op][3];
}
}else{//player2
size2=att2.size();
if((location+1)>size2){
att2.resize(size2+1);
att2[location].resize(2);
att2[location][0]=oper[op][2];
att2[location][1]=oper[op][3];
}
else{
att2.insert(att2.begin()+location,temp);
att2[location][0]=oper[op][2];
att2[location][1]=oper[op][3];
}
}
}
else if(oper[op][0]==2){//attck
if(flag==1){//player1
atc=oper[op][1];//1的act
df=oper[op][2];//2的df
if(df==0){//打英雄
h2-=att1[atc-1][0];
if(h2<=0){
die=2;
break;
}
}
else{//打随从
att2[df-1][1]-=att1[atc-1][0];
att1[atc-1][1]-=att2[df-1][0];
if(att1[atc-1][1]<=0){
att1.erase(att1.begin()+atc-1);
}
if(att2[df-1][1]<=0){
att2.erase(att2.begin()+df-1);
}
}
}else{//player2
atc=oper[op][1];//2的act
df=oper[op][2];//1的df
if(df==0){//打英雄
h1-=att2[atc-1][0];
if(h1<=0){
die=1;
break;
}
}
else{//打随从
att1[df-1][1]-=att2[atc-1][0];
att2[atc-1][1]-=att1[df-1][0];
if(att2[atc-1][1]<=0){
att2.erase(att2.begin()+atc-1);
}
if(att1[df-1][1]<=0){
att1.erase(att1.begin()+df-1);
}
}
}
}
else{//end
if(flag==1){//player1
flag=2;
}else{//player2
flag=1;
}
}
}
if(die==0){
cout<<0<<endl;
}
else if(die==1){
cout<<-1<<endl;
}
else{
cout<<1<<endl;
}
cout<<h1<<endl;
cout<<att1.size();
for(i=0;i<att1.size();i++){
cout<<" "<<att1[i][1];
}
cout<<endl;
cout<<h2<<endl;
cout<<att2.size();
for(i=0;i<att2.size();i++){
cout<<" "<<att2[i][1];
}
cout<<endl;
/*
cout<<"1随从"<<endl;
for(i=0;i<att1.size();i++){
for(j=0;j<att1[i].size();j++){
cout<<att1[i][j]<<" ";
}
cout<<endl;
}
cout<<"2随从"<<endl;
for(i=0;i<att2.size();i++){
for(j=0;j<att2[i].size();j++){
cout<<att2[i][j]<<" ";
}
cout<<endl;
}
*/
return 0;
}
后来才发现考虑了一些冗余的情况,不过代码没改了。