效果如图(动态效果自行执行)
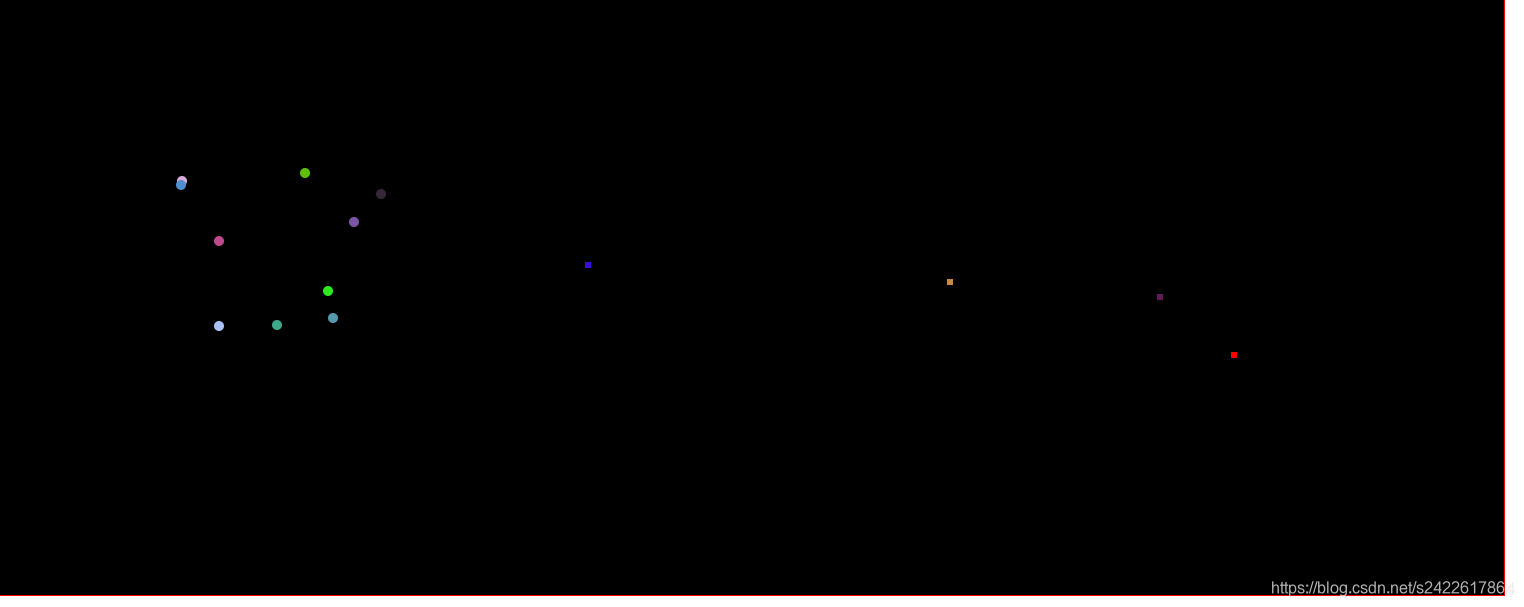
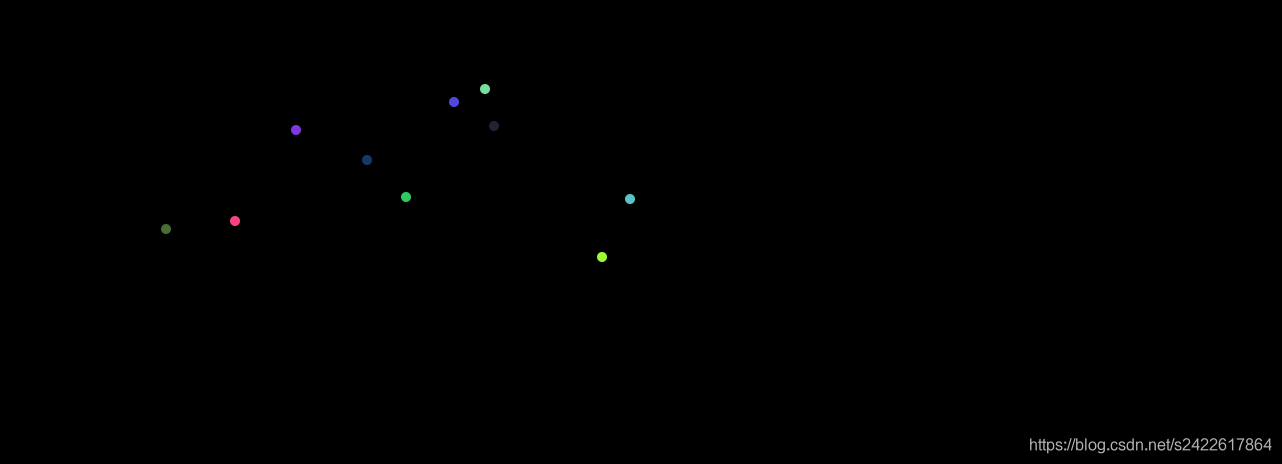
代码如下直接cv即可(欢迎指正)
不懂的地方也可以评论问我
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
#container {
width: 80%;
height: 600px;
border: 1px red solid;
position: relative;
margin: 20px auto;
cursor: pointer;
background: black;
}
.fire {
background: red;
position: absolute;
bottom: 0px;
width: 6px;
height: 6px;
}
.small-fire {
width: 10px;
height: 10px;
position: absolute;
border-radius: 50%;
}
</style>
</head>
<body>
<div id="container">
</div>
<script>
var oCont = document.querySelector('#container');
oCont.onclick = function (e) {
var pos = {};
pos.x = e.offsetX;
pos.y = e.offsetY;
new Fire(oCont, pos);
};
function Fire(oCont, pos) {
this.oCont = oCont;
this.pos = pos;
var oDivB = document.createElement('div');
oDivB.className = 'fire';
oDivB.style.left = this.pos.x + 'px';
oDivB.style.backgroundColor = this.getColor();
this.oCont.append(oDivB);
var that = this;
this.move(oDivB, { top: this.pos.y }, function () {
oDivB.remove();
that.smallFire();
})
}
Fire.prototype.smallFire = function () {
for (var i = 0; i < 12; i++) {
var oDivS = document.createElement('div');
oDivS.className = 'small-fire';
oDivS.style.backgroundColor = this.getColor();
this.oCont.append(oDivS);
oDivS.style.top = this.pos.y + 'px';
oDivS.style.left = this.pos.x + 'px';
var left = this.rand(0, this.oCont.offsetWidth - oDivS.offsetWidth);
var top = this.rand(0, this.oCont.offsetHeight - oDivS.offsetHeight)
that = this;
(function (ele) {
that.move(ele, {
left: left,
top: top
}, function () {
ele.remove();
});
})(oDivS)
}
}
Fire.prototype.move = function (fireObj, json, callBack) {
var times = '';
clearInterval(times);
that = this;
times = setInterval(() => {
for (var attr in json) {
var nowSize = parseInt(that.getStyle(fireObj, attr));
var speed = (json[attr] - nowSize) / 7;
speed = speed > 0 ? Math.ceil(speed) : Math.floor(speed);
fireObj.style[attr] = speed + nowSize + 'px';
if (json[attr] == nowSize) {
clearInterval(times);
callBack();
}
}
}, 30);
}
Fire.prototype.getColor = function () {
var str = '#';
for (var i = 0; i < 6; i++) {
str += (Math.round(Math.random() * 16)).toString(16);
}
return str;
}
Fire.prototype.getStyle = function (ele, attr) {
if (ele.currentStyle) {
return ele.currentStyle[attr];
} else {
return getComputedStyle(ele, false)[attr];
}
}
Fire.prototype.rand = function (min, max) {
return parseInt(Math.random() * (max - min) + min);
}
</script>
</body>
</html>