阅读导航
-
本文背景
-
代码实现
-
本文参考
-
源码
1. 本文背景
WPF中垂直导航菜单大家应该都常用,本文介绍使用MVVM的方式怎么绑定菜单,真的很简单。
使用 .Net Core 3.1 创建名为 “MenuMVVM” 的WPF模板项目,添加两个Nuget库:MaterialDesignThemes和MaterialDesignColors。
MaterialDesign控件库
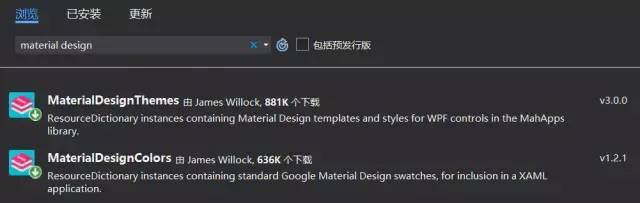
解决方案目录结构:
MenuMVVM
Views
MainView.xaml
MainView.xaml.cs
ViewModels
MainViewModel.cs
Modles
ItemCount.cs
MenuItem.cs
App.xaml
2.1 引入MD控件样式
文件【App.xaml】,在StartupUri中设置启动的视图【Views/MainView.xaml】,并在【Application.Resources】节点增加MD控件4个样式文件
-
x:Class="MenuMVVM.App"
-
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
-
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
-
StartupUri="Views/MainView.xaml">
-
>
-
>
-
>
-
Source="pack://application:,,,/MaterialDesignThemes.Wpf;component/Themes/MaterialDesignTheme.Dark.xaml" />
-
Source="pack://application:,,,/MaterialDesignThemes.Wpf;component/Themes/MaterialDesignTheme.Defaults.xaml" />
-
Source="pack://application:,,,/MaterialDesignColors;component/Themes/Recommended/Primary/MaterialDesignColor.Blue.xaml" />
-
Source="pack://application:,,,/MaterialDesignColors;component/Themes/Recommended/Accent/MaterialDesignColor.LightBlue.xaml" />
-
-
-
-
2.2 Models
两个简单的菜单实体类:
2.2.1 菜单新文件信息
文件【ItemCount.cs】,定义菜单项右侧的新文件显示个数及显示背景色:
-
using System.Windows.Media;
-
namespace MenuMVVM.Models
-
{
-
public class ItemCount
-
{
-
public Brush Color { get; private set; }
-
public int Value { get; private set; }
-
public ItemCount(Brush color, int value)
-
{
-
Color = color;
-
Value = value;
-
}
-
}
-
}
2.2.2 菜单项信息
文件【MenuItem.cs】,定义菜单项展示的名称、图片、新文件信息:
-
using MaterialDesignThemes.Wpf;
-
using System;
-
namespace MenuMVVM.Models
-
{
-
public class MenuItem
-
{
-
public String Name { get; private set; }
-
public PackIconKind Icon { get; private set; }
-
public ItemCount Count { get; private set; }
-
public MenuItem(String name, PackIconKind icon, ItemCount count)
-
{
-
Name = name;
-
Icon = icon;
-
Count = count;
-
}
-
}
-
}
其中菜单项图标使用MD控件自带的字体图标库,通过枚举【PackIconKind】可以很方便的使用,该库提供的字体图标非常丰富,目前有4836个(枚举值有7883个), 下面是最后几个:
-
//
-
// 摘要:
-
// List of available icons for use with MaterialDesignThemes.Wpf.PackIcon.
-
//
-
// 言论:
-
// All icons sourced from Material Design Icons Font - https://materialdesignicons.com/
-
// - in accordance of https://github.com/Templarian/MaterialDesign/blob/master/license.txt.
-
public enum PackIconKind
-
{
-
.
-
.
-
.
-
ZodiacPisces = 4832,
-
HoroscopePisces = 4832,
-
ZodiacSagittarius = 4833,
-
HoroscopeSagittarius = 4833,
-
ZodiacScorpio = 4834,
-
HoroscopeScorpio = 4834,
-
ZodiacTaurus = 4835,
-
HoroscopeTaurus = 4835,
-
ZodiacVirgo = 4836,
-
HoroscopeVirgo = 4836
-
}
2.3 ViewModels
文件【MainViewModel.cs】,只定义了简单的几个属性:窗体展示Logo、菜单绑定列表。属性定义比较简单,因为视图MainView.xaml展示内容不多:
-
using MaterialDesignThemes.Wpf;
-
using MenuMVVM.Models;
-
using System.Collections.Generic;
-
using System.Windows.Media;
-
namespace MenuMVVM.ViewModels
-
{
-
public class MainViewModel
-
{
-
public string Logo { get; set; }
-
public List LeftMenus { get; set; }
-
public MainViewModel()
-
{
-
Logo = "https://img.dotnet9.com/logo-foot.png";
-
LeftMenus = new List();
-
LeftMenus.Add(new MenuItem("图片", PackIconKind.Image, new ItemCount(Brushes.Black, 2)));
-
LeftMenus.Add(new MenuItem("音乐", PackIconKind.Music, new ItemCount(Brushes.DarkBlue, 4)));
-
LeftMenus.Add(new MenuItem("视频", PackIconKind.Video, new ItemCount(Brushes.DarkGreen, 7)));
-
LeftMenus.Add(new MenuItem("文档", PackIconKind.Folder, new ItemCount(Brushes.DarkOrange, 9)));
-
}
-
}
-
}
2.4 Views
文件【MainView.xaml】作为唯一的视图,只有31行布局代码,显示了一个Logo、菜单列表:
-
x:Class="MenuMVVM.Views.MainView"
-
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
-
xmlns:materialDesign="http://materialdesigninxaml.net/winfx/xaml/themes"
-
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
-
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
-
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
-
mc:Ignorable="d"
-
Title="Dotnet9" Height="600" Width="1080" Background="#FF36235F" MouseLeftButtonDown="Window_MouseLeftButtonDown"
-
WindowStyle="None" ResizeMode="NoResize" WindowStartupLocation="CenterScreen">
-
>
-
Width="200" HorizontalAlignment="Left" Background="#FF472076">
-
Height="150" Background="White">
-
Source="{Binding Logo}"/>
-
-
ItemsSource="{Binding LeftMenus}">
-
>
-
>
-
Orientation="Horizontal" Height="30">
-
Kind="{Binding Path=Icon}" Width="20" Height="20" VerticalAlignment="Center"/>
-
Text="{Binding Path=Name}" Margin="20 0" FontSize="15" VerticalAlignment="Center"/>
-
VerticalAlignment="Center">
-
Width="30" Height="15" RadiusY="7.15" RadiusX="7.15" Fill="{Binding Path=Count.Color}" Stroke="White" StrokeThickness="0.7"/>
-
Text="{Binding Path=Count.Value}" HorizontalAlignment="Center" VerticalAlignment="Center" FontSize="9"/>
-
-
-
-
-
-
-
-
文件【MainView.xaml.cs】作为视图【MainView.xaml】的后台,绑定ViewModel,并实现鼠标左键拖动窗体功能:
-
using MenuMVVM.ViewModels;
-
using System.Windows;
-
namespace MenuMVVM.Views
-
{
-
///
-
/// 演示主窗体,只用于简单的绑定ListView控件
-
///
-
public partial class MainView : Window
-
{
-
public MainView()
-
{
-
this.DataContext = new MainViewModel();
-
InitializeComponent();
-
}
-
private void Window_MouseLeftButtonDown(object sender, System.Windows.Input.MouseButtonEventArgs e)
-
{
-
DragMove();
-
}
-
}
-
}
3.本文参考
-
视频一:C# WPF Design UI: Navigation Drawer Model View View Mode,配套源码:MenuMVVM。
4.源码
文中代码已经全部给出,图片使用站长网站外链,可直接Copy代码,按解决方案目录组织代码文件即可运行,另附原作者视频及源码,见【3.本文参考】。